Maximizing Code Quality: Taming PMD, FindBugs, and Checkstyle
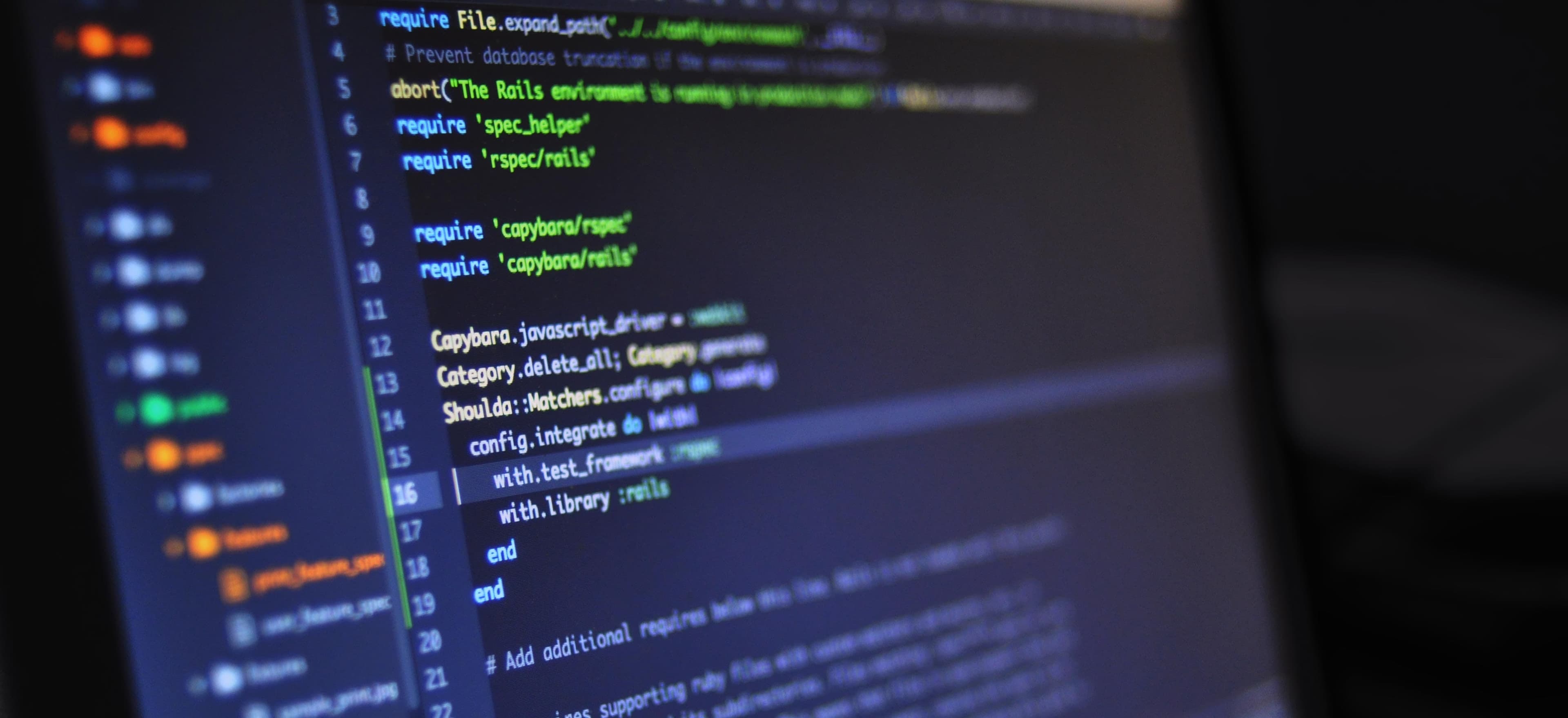
- Published on
Maximizing Code Quality: Taming PMD, FindBugs, and Checkstyle
In the world of software development, ensuring high code quality is not just an added bonus—it’s a necessity. Poor code quality can lead to bugs, increased maintenance costs, and hindered team productivity. Fortunately, tools like PMD, FindBugs, and Checkstyle can help developers spot issues early and enforce coding standards. In this article, we'll dive into each of these tools, explore their unique functionalities, and illustrate how they can be effectively integrated into your development workflow.
Understanding the Tools
PMD
PMD is a static code analysis tool that scans Java source code to identify common programming flaws, such as potential bugs, dead code, and suboptimal coding practices. It comes with a set of built-in rules and allows developers to create custom rules tailored to their specific needs.
FindBugs
FindBugs is another static analysis tool focused on detecting bugs in Java programs. It analyzes Java bytecode to identify issues such as null pointer dereferences, infinite recursive loops, and thread synchronization problems. It's a great complement to PMD as it digs deeper into potential runtime issues.
Checkstyle
Checkstyle offers a different approach by focusing on coding style and formatting. Instead of identifying bugs, it checks adherence to coding conventions. This can include anything from naming conventions, whitespace, and indentation to comprehensive Java-style guidelines.
Why Use These Tools?
Utilizing PMD, FindBugs, and Checkstyle leads to several benefits:
- Early Bug Detection: Identifying issues at an early stage minimizes debugging time.
- Maintainability: High-quality code is easier to understand, modify, and extend over time.
- Consistency: Enforcing coding standards across a team helps ensure that everyone writes code in a similar manner.
- Skill Development: These tools can help developers learn best practices.
Setting Up the Tools
To get started with PMD, FindBugs, and Checkstyle, we will integrate them into a typical Java project. Below is a step-by-step guide.
Step 1: Configuring PMD
-
Add PMD to Your Project: Start by adding the PMD dependency in your Maven
pom.xml
.<dependency> <groupId>net.sourceforge.pmd</groupId> <artifactId>pmd-core</artifactId> <version>6.38.0</version> </dependency>
-
Create a PMD Rules File: Create a
ruleset.xml
file that defines the rules you want to enforce. Here is an example rule that checks for unused local variables.<ruleset xmlns="http://pmd.sourceforge.net/ruleset_2_0_0.dtd"> <rule ref="rulesets/java/unnecessary.xml/UnusedLocalVariable"/> </ruleset>
-
Run PMD from Command Line: You can run PMD using the following command:
mvn pmd:pmd
By defining rules in the above XML and running the tool, you can catch problems early in the development cycle before they affect other parts of your application.
Step 2: Integrating FindBugs
-
Add FindBugs to Your Project: For Maven projects, you can add FindBugs as follows:
<dependency> <groupId>org.codehaus.mojo.findbugs</groupId> <artifactId>findbugs-maven-plugin</artifactId> <version>3.0.5</version> </dependency>
-
Configure the Plugin: Add the FindBugs plugin configuration to your
pom.xml
.<build> <plugins> <plugin> <groupId>org.codehaus.mojo</groupId> <artifactId>findbugs-maven-plugin</artifactId> <version>3.0.5</version> <configuration> <xmlOutput>true</xmlOutput> <failOnError>true</failOnError> </configuration> </plugin> </plugins> </build>
-
Running FindBugs: Execute the following command from your terminal:
mvn findbugs:check
By doing so, you can easily detect potential bugs related to Java applications based on the bytecode. It's crucial to review the reports generated by FindBugs, as they often point out critical issues that could lead to runtime failures.
Step 3: Configuring Checkstyle
-
Add Checkstyle to Your Project: You can include Checkstyle in your
pom.xml
like this:<dependency> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-checkstyle-plugin</artifactId> <version>2.17</version> </dependency>
-
Define Your Configuration: Here is an example
checkstyle.xml
file. This configuration checks for tab usage and ensures that javadoc comments are present for each public method.<!DOCTYPE module PUBLIC "-//Checkstyle//DTD Checkstyle Configuration 1.3//EN" "https://checkstyle.org/dtds/configuration_1_3.dtd"> <module name="Checker"> <module name="TreeWalker"> <module name="WhitespaceAround"/> <module name="JavadocType"/> </module> </module>
-
Running Checkstyle: You can run Checkstyle with the following command:
mvn checkstyle:check
The output will contain details about coding standard violations, giving you an idea of how you can improve code style across your project.
Best Practices for Test Integration
Continuous Integration (CI)
Integrating PMD, FindBugs, and Checkstyle into your CI pipeline makes running these tools an automatic part of your build process. This ensures that every commit meets your quality standards before being merged.
Code Review
Incorporating these tools into your code review process adds an additional layer of scrutiny. For example, if a developer submits code that doesn't pass PMD checks, it should be sent back for revision.
My Closing Thoughts on the Matter
Maximizing code quality is an ongoing process that requires the right tools and approaches. By effectively harnessing the power of PMD, FindBugs, and Checkstyle, you can put your Java projects on a solid foundation, reducing errors and improving readability.
Remember, the goal of using these tools is not to create obstacles but to foster a better coding environment. Embrace feedback, iterate on your processes, and watch as your code quality soars.
For further reading, check the official documentation for PMD and FindBugs. These resources can provide you with more nuanced configurations tailored to your project's needs.
By adopting these practices, you will contribute to a healthier codebase and, ultimately, a more successful development journey. Happy coding!
Checkout our other articles