Securing Java JAAS: Troubleshooting Form-Based Authentication
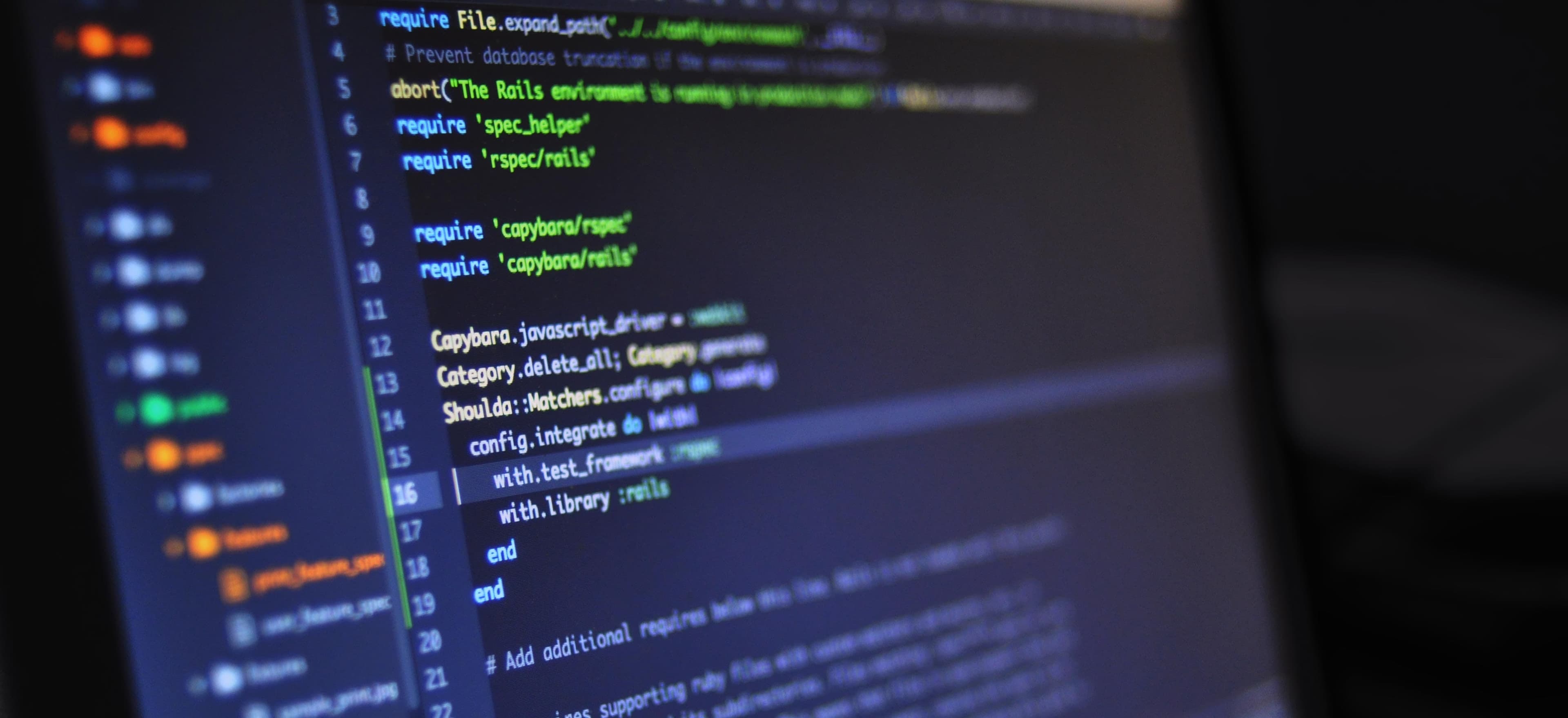
- Published on
Securing Java JAAS: Troubleshooting Form-Based Authentication
Java Authentication and Authorization Service (JAAS) is a robust framework that allows developers to add authentication and authorization features to their Java application. One common use case is implementing form-based authentication, which offers a more user-friendly way for users to log in to an application via a web-based form. However, as with any technology, problems may arise, requiring troubleshooting. In this blog post, we'll explore the intricacies of form-based authentication using JAAS and delve into common issues and how to resolve them.
Understanding Form-Based Authentication in JAAS
What is Form-Based Authentication?
Form-based authentication is an approach that provides users with a graphical interface to enter their login credentials. This method enhances usability compared to traditional login methods, such as basic authentication, where users must enter credentials in a pop-up window.
The Role of JAAS
JAAS is part of the Java platform and allows developers to separate the authentication logic from the application code. This modular approach improves code maintainability and enables businesses to leverage existing authentication mechanisms more efficiently.
In a typical JAAS setup, the authentication process involves three main components:
- Login Modules: These modules are responsible for authenticating users. They can access various data sources, such as databases or LDAP servers, to perform this action.
- Subject: A representation of the authenticated user, detailing their identities and associated permissions.
- Login Configuration: This configuration specifies which login modules are used and how they should be combined.
Setting Up Form-Based Authentication
To understand how to troubleshoot form-based authentication in JAAS effectively, let’s take a look at how to set it up:
Sample Application Setup
- Dependencies: You need the following dependencies in your
pom.xml
file for Maven:
<dependency>
<groupId>javax.security.auth</groupId>
<artifactId>jaas</artifactId>
<version>1.0</version>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>4.0.1</version>
<scope>provided</scope>
</dependency>
- Creating the Login Module:
Here's an example of a simple JAAS login module:
import javax.security.auth.callback.*;
import javax.security.auth.spi.LoginModule;
import java.util.Map;
public class MyLoginModule implements LoginModule {
private Subject subject;
private CallbackHandler callbackHandler;
private boolean succeeded;
@Override
public void initialize(Subject subject, CallbackHandler callbackHandler, Map<String, ?> sharedState, Map<String, ?> options) {
this.subject = subject;
this.callbackHandler = callbackHandler;
}
@Override
public boolean login() throws LoginException {
// Prompt user for username and password
NameCallback nameCallback = new NameCallback("Username: ");
PasswordCallback passwordCallback = new PasswordCallback("Password: ", false);
try {
callbackHandler.handle(new Callback[]{nameCallback, passwordCallback});
} catch (Exception e) {
throw new LoginException("Error retrieving credentials");
}
String username = nameCallback.getName();
String password = new String(passwordCallback.getPassword());
// Validate user credentials (this is just a placeholder)
if ("admin".equals(username) && "password".equals(password)) {
succeeded = true;
return true;
}
return false;
}
@Override
public boolean commit() throws LoginException {
if (!succeeded) {
return false;
}
// Here you would add the UserPrincipal to the subject to represent the user
// this.subject.getPrincipals().add(new UserPrincipal(username));
return true;
}
@Override
public boolean abort() throws LoginException {
return false;
}
@Override
public boolean logout() throws LoginException {
return false;
}
}
Commentary:
- The
initialize
method sets up the callback handler. - The
login
method handles user input. In a real-world application, you would retrieve valid username/password pairs from a database or a secure vault. - The
commit
method finalizes the login; this is where you could register user roles or add them to the subject for further use.
- Creating the Login Configuration (
login.config
):
Here’s an example login.config
file:
MyLoginModule com.example.MyLoginModule required;
Web XML Configuration
Assuming you're using a Java EE application, you may also want to configure your web.xml to incorporate JAAS easily.
<security-constraint>
<web-resource-collection>
<web-resource-name>Protected Area</web-resource-name>
<url-pattern>/protected/*</url-pattern>
</web-resource-collection>
<auth-constraint>
<role-name>USER</role-name>
</auth-constraint>
</security-constraint>
<login-config>
<auth-method>FORM</auth-method>
<form-login-config>
<form-login-page>/login.jsp</form-login-page>
<form-error-page>/error.jsp</form-error-page>
</form-login-config>
</login-config>
<security-role>
<role-name>USER</role-name>
</security-role>
Commentary:
- This configuration specifies that access to
/protected/*
resources is restricted to users with the "USER" role. - Upon authentication failure, users are redirected to an error page.
Common Issues and Troubleshooting Steps
1. User Credentials Not Validating
Symptoms: Users are unable to log in even with the correct credentials.
Potential Causes:
- Incorrect username/password handling in the login module.
- Issues with the underlying data source (e.g., a database, LDAP).
Troubleshooting Steps:
- Add debug logging to the login method in your
MyLoginModule
to inspect the received credentials. - Test connectivity to your data source independently.
2. Configuration Issues
Symptoms: JAAS login fails without giving a clear error message.
Potential Causes:
- Misconfigured
login.config
orweb.xml
files.
Troubleshooting Steps:
- Double-check configuration files for typos or incorrect paths.
- Ensure the
login.config
file is appropriately placed in the application's classpath.
3. CallbackHandler Null Pointer Exception
Symptoms: Application crashes with a Null Pointer Exception during login.
Potential Causes:
- CallbackHandler not properly initialized.
Troubleshooting Steps:
- Ensure that your JAAS login context is correctly set up before invoking the login method.
- Test the application to confirm that the correct callback handler is being passed to the login module.
4. Redirects and Session Issues
Symptoms: After successful login, users are redirected to the login page again.
Potential Causes:
- Session not being maintained correctly due to misconfigured session management.
Troubleshooting Steps:
- Verify server-side session handling configurations and cookie settings.
- Ensure session attributes are being correctly set and retrieved after login.
Bringing It All Together
Form-based authentication with JAAS provides a powerful mechanism for authenticating users while maintaining a simple and user-friendly approach. However, various challenges may arise during its implementation. By understanding the framework, carefully configuring your application, and employing effective troubleshooting strategies, you can resolve these issues efficiently.
For further reading on JAAS and security best practices in Java, check out Oracle's official JAAS documentation.
If you’re facing specific hurdles or have insights on JAAS that can benefit others, feel free to share your experiences in the comments below!
Checkout our other articles