Overcoming Common Gradle Issues in Spring Boot Apps
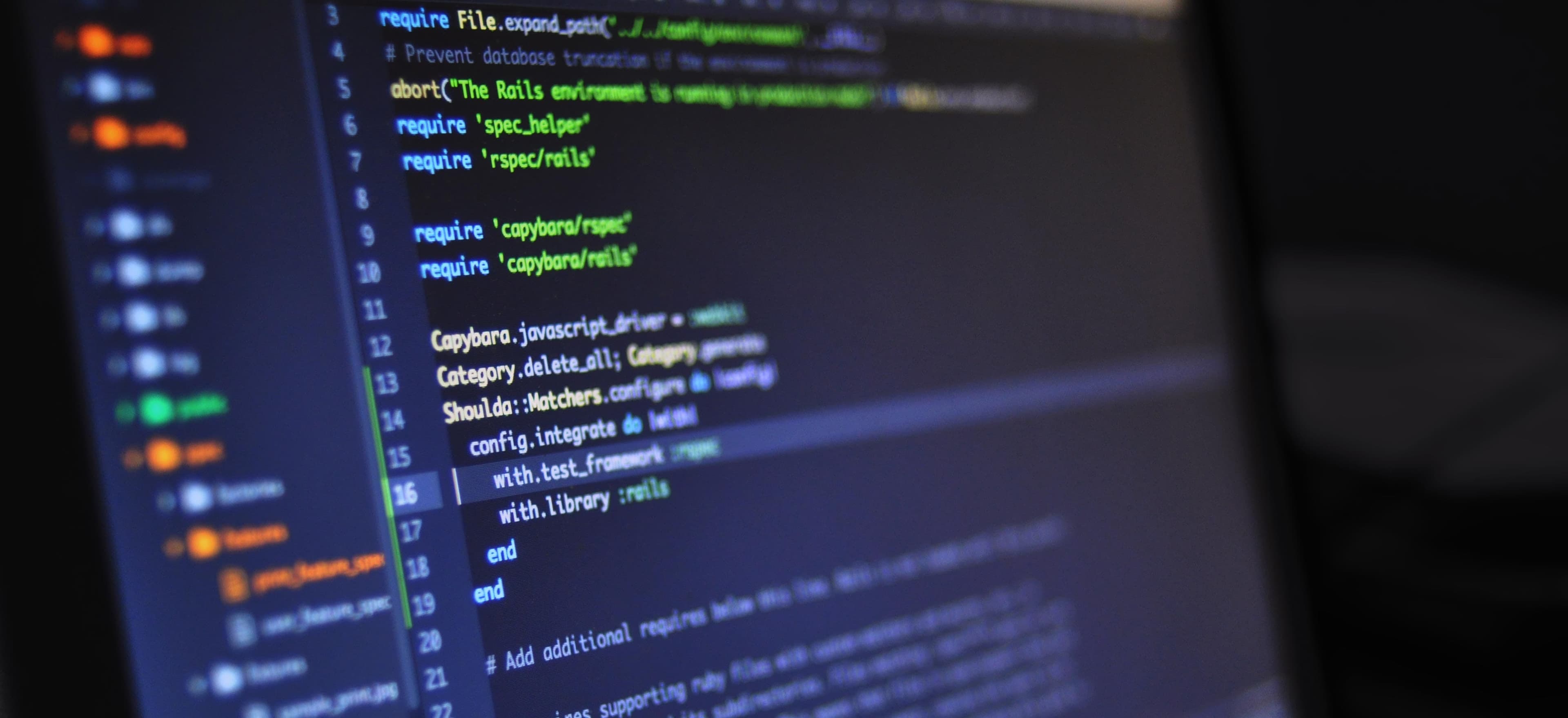
- Published on
Overcoming Common Gradle Issues in Spring Boot Apps
Gradle is a powerful build automation tool that significantly simplifies the development process of Spring Boot applications. However, like any tool, it can present challenges. In this blog post, we'll explore common issues encountered with Gradle in Spring Boot projects and provide troubleshooting tips to overcome them. We aim to enhance both your development experience and overall project performance.
Table of Contents
Understanding Gradle Basics
Before delving into issues, it’s important to understand what Gradle does. Gradle uses a Groovy (or Kotlin) based Domain Specific Language (DSL) to define the build process. Here’s a basic example of a build.gradle
file for a Spring Boot application:
plugins {
id 'org.springframework.boot' version '2.5.4'
id 'io.spring.dependency-management' version '1.0.11.RELEASE'
id 'java'
}
group = 'com.example'
version = '0.0.1-SNAPSHOT'
sourceCompatibility = '11'
repositories {
mavenCentral()
}
dependencies {
implementation 'org.springframework.boot:spring-boot-starter'
testImplementation 'org.springframework.boot:spring-boot-starter-test'
}
Why This Matters: Understanding the structure and syntax of your build.gradle
file is essential for troubleshooting issues effectively.
Common Gradle Issues
Issue 1: Dependency Conflicts
Dependency conflicts occur when different versions of the same library are included in your project. This can lead to unexpected behavior or runtime errors.
How to Detect and Resolve:
-
Dependency Tree Analysis: Use the command:
./gradlew dependencies
This will display a tree structure of your dependencies, revealing any conflicts.
-
Force a Specific Version: If you identify conflicting versions, you can force a specific version by adding this in your
dependencies
block:configurations.all { resolutionStrategy { force 'org.apache.commons:commons-lang3:3.12.0' } }
Best Practice: Regularly check for dependency updates and avoid using libraries with overlapping transitive dependencies.
Issue 2: Build Failures
Build failures can stem from various issues such as incorrect configurations, environmental problems, or even bugs in plugins.
How to Diagnose and Fix:
-
Gradle Build Scan: Leverage the Gradle build scan feature to get in-depth insights into your build process. You can run:
./gradlew build --scan
This generates a report accessible via a URL that provides detailed failure information.
-
Clean Build: Sometimes, stray files can cause problems. Always try a clean build first:
./gradlew clean build
-
Check for Updates: Ensure that you are using compatible versions of Gradle and Spring Boot. Mismatches can lead to unexpected failures.
Pro Tip: Explore the Gradle documentation or community forums like Stack Overflow if specific errors are persistent.
Issue 3: Plugin Problems
Plugins extend Gradle's functionality but can sometimes introduce issues. Common problems include incompatible versions or misconfigurations.
Solution Approaches:
-
Version Compatibility: Always check the compatibility of plugins with your Gradle version. Keep an eye on release notes for breaking changes.
-
Review Plugin Configuration: Ensure the plugins are correctly configured in your
build.gradle
. Here's how you might specify the Spring Boot plugin:plugins { id 'org.springframework.boot' version '2.5.4' id 'io.spring.dependency-management' version '1.0.11.RELEASE' id 'java-library' // Ensure you're using the correct plugin for your project type }
-
Example: Spring Boot Starter Web Plugin:
dependencies { implementation 'org.springframework.boot:spring-boot-starter-web' }
This plugin provides essential libraries for building web applications.
Why This Matters: The right plugins significantly enhance productivity and can prevent a myriad of problems just by being properly configured.
Best Practices to Prevent Issues
-
Consistent Gradle Version: Use the Gradle Wrapper to ensure everyone on your team uses the same Gradle version:
Run:
./gradlew wrapper --gradle-version=7.1.1
-
Regular Dependency Updates: Regularly check for dependency updates using tools like Dependabot or Gradle's Dependency Update plugin.
-
Continuous Integration: Incorporate CI/CD practices to catch build issues early, ensuring that your applications are always in a deployable state. Tools like Jenkins or GitHub Actions can help with this.
A Final Look
Gradle is a robust tool that, when used effectively, can significantly streamline your Spring Boot development process. Challenges are inevitable, but by understanding common pitfalls and employing the best practices discussed here, you can tackle issues head-on.
If you find you are continually facing problems, don’t hesitate to consult the Spring Boot documentation or the Gradle documentation. Staying informed and proactive is the best strategy for smooth development. Happy coding!
Checkout our other articles