Microservices vs. Monolith: Choosing the Right Architecture Challenge
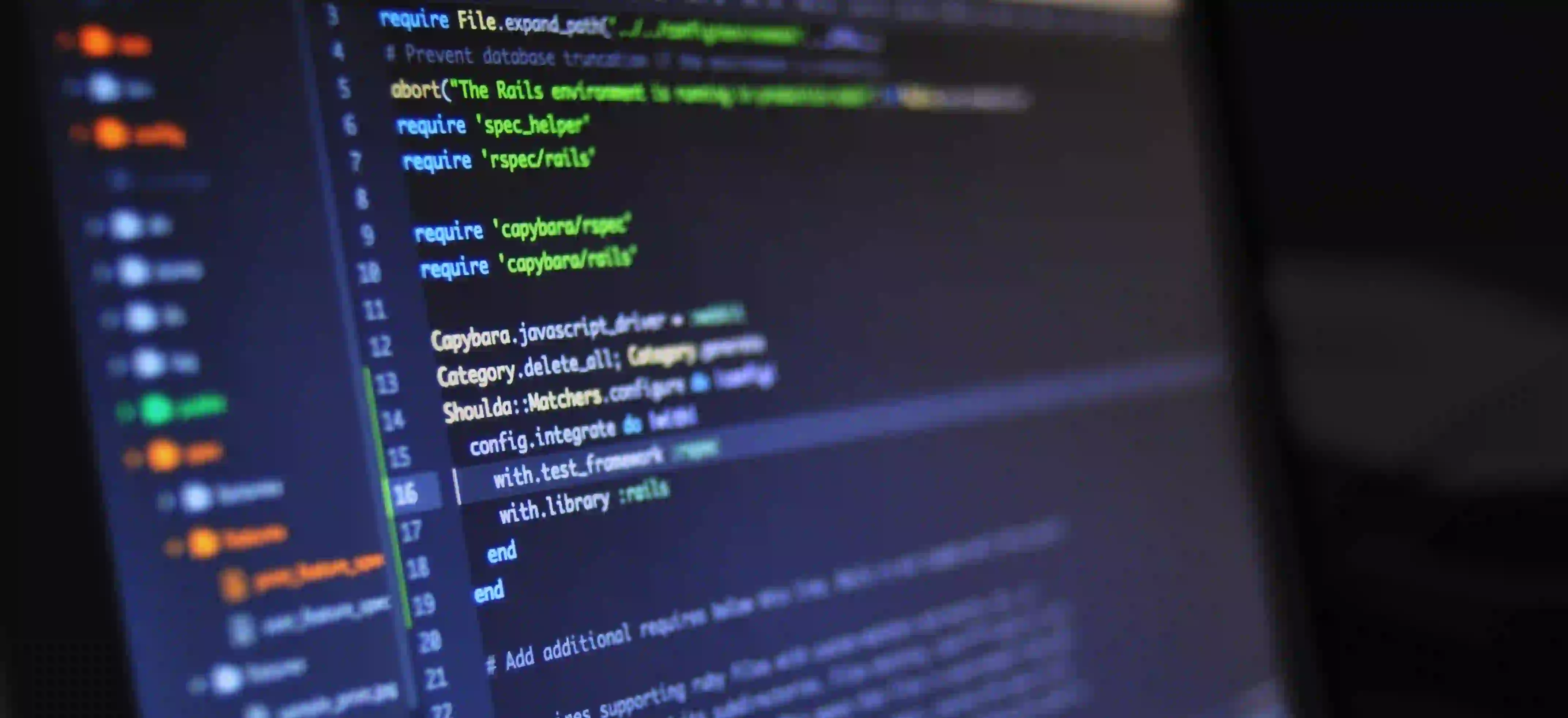
Microservices vs. Monolith: Choosing the Right Architecture Challenge
Choosing the right software architecture is crucial to the success of any application. It directly influences scalability, maintainability, and deployment speed. Among the many architectural patterns available, microservices and monolithic architectures stand out as the most popular choices.
In this blog post, we’ll compare microservices and monolithic structures, discussing their respective advantages and disadvantages. We aim to equip you with the knowledge necessary to make an informed decision for your next project.
Understanding Monolithic Architecture
Definition
A monolithic architecture refers to an application built as a single, unified unit. This means that all components—UI, business logic, database access—are packaged into one codebase.
Advantages of Monolithic Architecture
- Simplicity: One of the biggest advantages is the simplicity of deployment. There's only one compiled codebase to work with, making it easy to test and deploy.
public class MonolithicApp {
public static void main(String[] args) {
// Start the application
}
}
In this example, the MonolithicApp
class serves as a single entry point, encapsulating all application logic.
-
Performance: Because everything is processed in a single runtime environment, interactions between components are typically faster.
-
Easier Debugging: Debugging a single application is usually less complex than dealing with multiple services.
Disadvantages of Monolithic Architecture
-
Scalability Challenges: Scaling a monolithic application can be cumbersome. If one part of the application needs to scale, the entire application must do so as well.
-
Longer Deployment Times: When changes are made, the entire application must be rebuilt and redeployed, leading to longer downtimes.
-
Technology Lock-In: With a monolithic architecture, you are often tied to a single technology stack, limiting flexibility.
Understanding Microservices Architecture
Definition
Microservices architecture, on the other hand, breaks down applications into smaller, individual services that run independently and communicate over well-defined APIs.
Advantages of Microservices Architecture
- Scalability: Each service can be scaled independently based on the demand. For instance, if a particular service deals with user interactions, it can be scaled while leaving other services intact.
@RestController
@RequestMapping("/user")
public class UserService {
@GetMapping("/{id}")
public User getUser(@PathVariable String id) {
// Logic to retrieve user details
}
}
Here, the UserService
class represents a small, independent microservice focused solely on user-related operations.
-
Flexibility with Technology: Different services can be built with different programming languages or frameworks, allowing teams to use the best tools for each job.
-
Faster Deployments: Changes to a service can be made and deployed without affecting the entire application, resulting in shorter downtimes.
-
Better Fault Isolation: If one service fails, it does not necessarily bring down the entire application.
Disadvantages of Microservices Architecture
-
Complexity: The distributed nature of microservices adds complexity in communication, data management, and testing.
-
Overhead: Managing multiple services can result in operational overhead, including monitoring, logging, and managing inter-service communication.
-
Consistency Challenges: Ensuring data consistency across services can be difficult, requiring robust strategies like Distributed Transactions or eventual consistency models.
Factors to Consider When Choosing Between Microservices and Monolithic
1. Project Size and Team Structure
For smaller, less complex applications, a monolithic architecture can be sufficient. If you have a small team, simpler deployment and debugging processes allow for efficient management and development.
Conversely, for larger applications with fluctuating traffic patterns, microservices become a better choice. Their ability to handle growth without completely overhauling the architecture makes them more appropriate.
2. Development Velocity
If your team is focused on rapid development and continuous delivery, microservices will generally provide that advantage. However, if your project requires a quick go-to-market solution, a monolithic architecture might serve you better.
3. Tech Stack Diversity
Consider whether you need a variety of technologies for different components of your application. If so, microservices might be the best choice. If not, a monolithic architecture can keep things simple and coherent.
4. Deployment Frequency
How often do you need to release updates? Frequent deployments are more manageable with microservices because changes in one service do not require the redeployment of the entire application.
5. Operational Overhead
Do you have the infrastructure and support to manage multiple individual services? If not, consider a monolithic architecture.
Making the Choice
The decision between microservices and a monolith is not straightforward. It revolves primarily around the application's needs, your team's expertise, and future scalability requirements.
Here are some steps to aid your decision-making:
-
Assess your team's size and skill set: A small team might fare better in a monolithic setup, while a larger team might benefit from the flexibility offered by microservices.
-
Evaluate the application's goals: If scalability is a significant factor, microservices can offer a distinct advantage.
-
Consider future maintenance: Will your application undergo frequent changes or require rapid scaling? Microservices architecture provides more agility.
-
Analyze the existing legacy systems: If there’s an existing monolithic application, consider a gradual migration to microservices rather than a complete overhaul.
Closing Remarks
Choosing the right architecture is pivotal to your software's success. While microservices offer more flexibility and scalability, they come with added complexity. Monolithic architectures provide simplicity and performance but may struggle with scalability as applications grow.
Ultimately, there is no one-size-fits-all answer. Instead, it is essential to weigh the pros and cons tailored to your unique situation.
For deeper insights on architectural decisions, check out these resources: Microservices Best Practices and Understanding Monolithic Applications.
By understanding your requirements and limitations, you will be able to make an informed decision that will set you on the path to a successful software architecture, whether it be monolithic or microservices.