Debugging Common Issues with Spring Async Threads
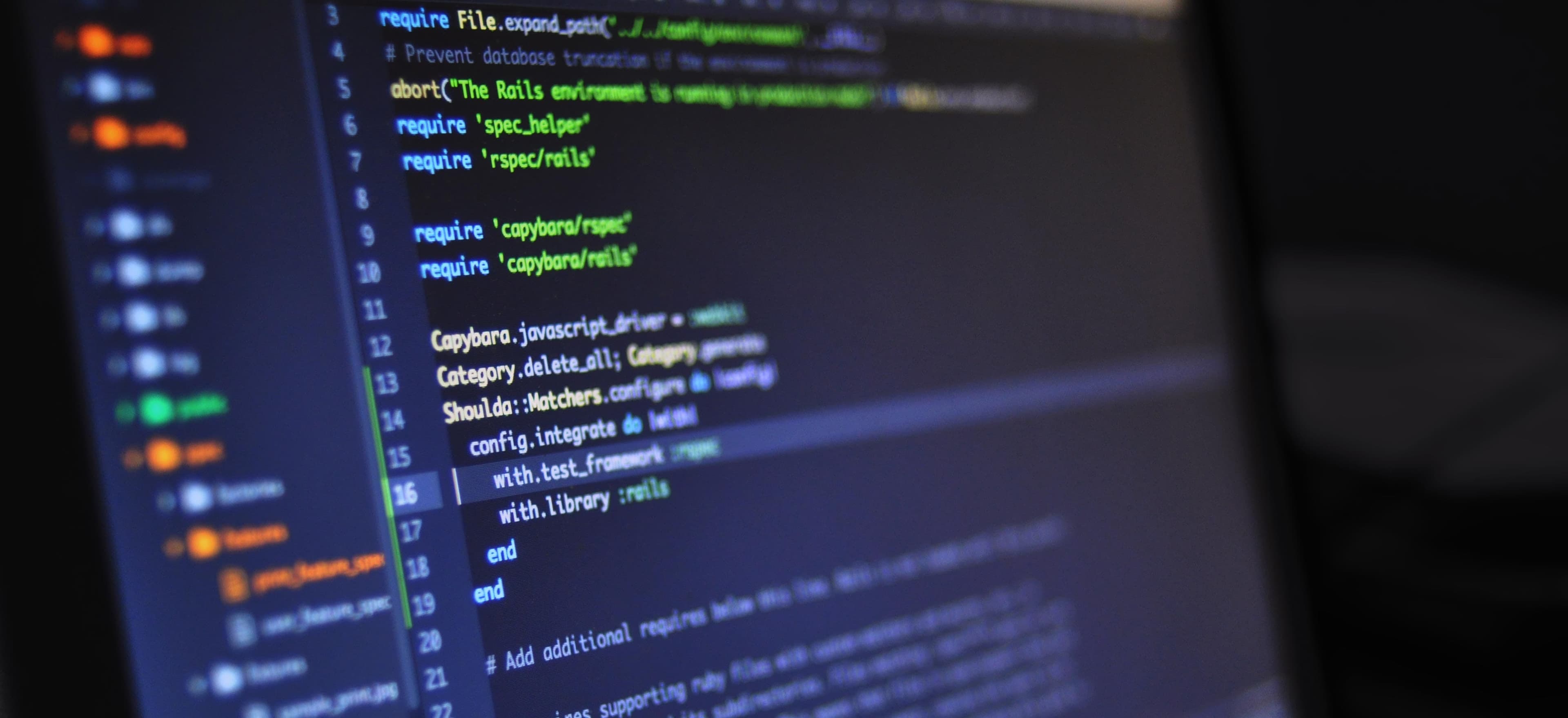
- Published on
Debugging Common Issues with Spring Async Threads
Spring Framework simplifies concurrent programming with its support for asynchronous method execution. However, the complexity of threading can often lead to challenges and bugs. In this blog post, we will delve into the common issues developers face when using Spring Async threads and how to debug them effectively.
What is Spring Async?
Spring Async leverages the @Async
annotation, which allows methods to run in a separate thread, thereby freeing up the caller thread to perform other operations. This capability is particularly useful in applications that need to perform time-consuming tasks while remaining responsive.
The Basics of @Async
To utilize the @Async
annotation, first ensure that your application is configured to support asynchronous processing.
@Configuration
@EnableAsync
public class AsyncConfig {
@Bean(name = "myAsyncExecutor")
public Executor myAsyncExecutor() {
ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor();
executor.setCorePoolSize(5);
executor.setMaxPoolSize(10);
executor.setQueueCapacity(25);
executor.initialize();
return executor;
}
}
The above code configures a thread pool with specific limits. The key is understanding that a controlled thread pool helps manage resources effectively, enhancing the performance of your application.
Common Issues with Spring Async Threads
Even seasoned developers can run into issues when working with async threads. Here are a few common pitfalls along with debugging strategies.
1. Missing @EnableAsync Annotation
One of the first hurdles you may encounter is forgetting to add the @EnableAsync
annotation on your configuration class. Failing to do so means your asynchronous methods won’t execute in a separate thread.
Debugging Tip: Always check if you've enabled the async support with:
@Configuration
@EnableAsync
public class AppConfiguration {
}
2. Thread Pool Exhaustion
Constrained thread pools can lead to performance bottlenecks. If you exceed the maximum thread pool size, subsequent requests may be queued or yield errors. Here’s how you can mitigate this:
- Monitor Your Thread Pool: Use a monitoring tool to visualize thread health.
- Adjust Settings: Base your pool size on empirical data from your application’s workload.
Here’s an adjusted configuration for a larger thread pool:
@Bean(name = "myAsyncExecutor")
public Executor myAsyncExecutor() {
ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor();
executor.setCorePoolSize(10);
executor.setMaxPoolSize(20);
executor.setQueueCapacity(50);
executor.initialize();
return executor;
}
3. Exception Handling
Another issue arises if exceptions occur in async methods. By default, exceptions thrown in asynchronous methods won’t be caught by the calling thread, leading to unhandled exceptions.
You can configure an AsyncUncaughtExceptionHandler
to manage these:
@Async
public void executeAsyncTask() {
throw new RuntimeException("Exception in async method");
}
@Async
public void asyncMethodWithException() {
try {
// Your task logic
} catch (Exception e) {
// Log the exception
System.err.println("Exception: " + e.getMessage());
}
}
It’s also essential to define a custom exception handler:
@Component
public class CustomAsyncExceptionHandler implements AsyncUncaughtExceptionHandler {
@Override
public void handleUncaughtException(Throwable throwable, Method method, Object... obj) {
System.err.println("Exception thrown in async method: " + method.getName() + " with message: " + throwable.getMessage());
}
}
4. Task Completion and Return Values
When you need return values from asynchronous tasks, it’s common to use CompletableFuture<T>
. This raises complexity but allows capturing the outcome of a future task:
@Async
public CompletableFuture<String> asyncMethodWithReturnValue() {
// Simulate a long-running task
return CompletableFuture.completedFuture("Task Complete");
}
public void initiateAsyncCall() {
CompletableFuture<String> future = asyncMethodWithReturnValue();
future.thenAccept(result -> System.out.println(result));
}
Use CompletableFuture
to manage and handle results effectively, ensuring proper synchronization between threads.
5. Context Propagation Issues
When a method is called asynchronously, the execution context (such as security context or transaction context) may not be transferred, leading to unintended behavior.
To mitigate this, consider using @Async
within the same service. If you need to ensure that the security context is always available in an asynchronous call, integrating Spring Security's SecurityContext
can be beneficial.
You can achieve context propagation using Spring’s context-holding structures, but be mindful of increased complexity.
6. Thread Safety and Resource Management
Multiple threads accessing shared resources can lead to data inconsistency. Always ensure proper synchronization and utilize thread-safe data structures to avoid potential pitfalls.
For example, using ConcurrentHashMap
ensures thread-safe access to a map shared across multiple threads:
private ConcurrentHashMap<String, String> sharedMap = new ConcurrentHashMap<>();
@Async
public void updateMap(String key, String value) {
sharedMap.put(key, value);
}
7. Testing Asynchronous Code
Testing is another vital aspect of managing async calls. Use testing frameworks such as JUnit with Mockito to validate that asynchronous calls behave as expected.
@Test
public void testAsyncMethod() throws InterruptedException {
CompletableFuture<String> future = asyncMethodWithReturnValue();
assertEquals("Task Complete", future.get());
}
Final Thoughts
Asynchronous programming is powerful yet can be tricky. By adhering to best practices and leveraging Spring's capabilities, you can efficiently manage thread execution.
Want to dive deeper? Consider checking out the official Spring documentation for comprehensive details or explore strategies on Concurrent Programming to understand the larger context.
Remember, when debugging your Spring Async threads, maintain a log of exceptions, monitor thread usability, and ensure your methods are well-structured with regard to exception handling. Follow these tips, and you'll be on your way to mastering async programming in Spring.