Mastering Form Validation Errors in Spring 3 Applications
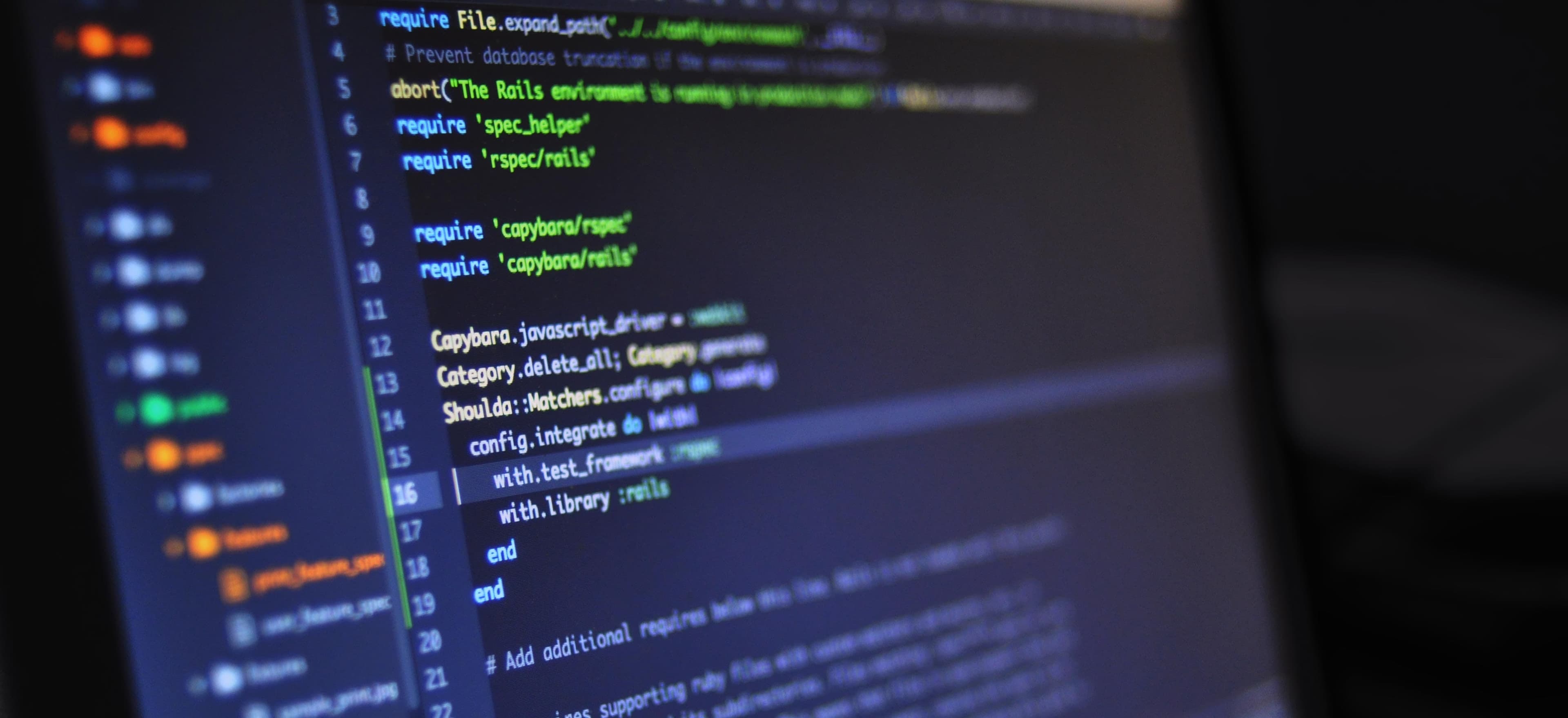
- Published on
Mastering Form Validation Errors in Spring 3 Applications
Form validation is a critical component of web applications, ensuring that user input meets certain criteria before processing it further. In Spring 3 applications, validating forms and handling feedback effectively is essential for a smooth user experience. This blog post delves into the concepts and practical implementations of form validation in Spring 3, providing you with a comprehensive guide to mastering this aspect of your applications.
Understanding the Importance of Form Validation
Before we dive into the specifics, let's discuss why form validation is essential:
-
Data Integrity: Validating user input helps maintain data integrity by ensuring that only correct data is processed.
-
User Experience: Proper validation reduces frustration by providing immediate feedback on incorrect inputs.
-
Security: Validation helps mitigate security vulnerabilities, such as SQL injection and cross-site scripting (XSS), by ensuring that unexpected or harmful data does not enter the system.
Overview of Spring 3 Validation
Spring provides a robust framework for handling form validation through the Java Bean Validation (JSR 303) API, also known as Hibernate Validator. This allows developers to utilize annotations for defining validation rules.
Key Annotations
Here are some essential annotations you will commonly use for form validation:
- @NotNull: Ensures that a property cannot be null.
- @Size: Validates the size of a string or collection.
- @Email: Checks whether the string is a valid email format.
- @Min and @Max: Validates numeric values by setting minimum and maximum limits.
These annotations, when placed on your model class fields, help define the rules for validation.
Setting Up Spring 3 Validation in Your Application
Let’s walk through the steps to set up form validation in a Spring 3 application.
Step 1: Add Dependencies
You’ll need to include the necessary dependencies in your pom.xml
if you're using Maven. If you're using a different build tool, ensure you have the required libraries.
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>3.x.x</version>
</dependency>
<dependency>
<groupId>javax.validation</groupId>
<artifactId>validation-api</artifactId>
<version>1.1.0.Final</version>
</dependency>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-validator</artifactId>
<version>5.x.x.Final</version>
</dependency>
Step 2: Create a Model Class
You’ll need a model class representing the data you want to validate. Here’s an example of a simple User class:
import javax.validation.constraints.Email;
import javax.validation.constraints.Min;
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Size;
public class User {
@NotNull(message = "Name cannot be null")
@Size(min = 2, max = 30, message = "Name must be between 2 and 30 characters")
private String name;
@NotNull(message = "Email cannot be null")
@Email(message = "Email should be valid")
private String email;
@NotNull(message = "Age cannot be null")
@Min(value = 18, message = "Age must be at least 18")
private Integer age;
// Getters and Setters
}
Step 3: Create a Controller
Next, set up a controller to handle the form submission and validation. Here’s how you can implement it:
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.validation.BindingResult;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import javax.validation.Valid;
@Controller
public class UserController {
@RequestMapping(value = "/register", method = RequestMethod.GET)
public String showForm(Model model) {
model.addAttribute("user", new User());
return "register";
}
@RequestMapping(value = "/register", method = RequestMethod.POST)
public String submitForm(@Valid @ModelAttribute("user") User user, BindingResult result, Model model) {
if (result.hasErrors()) {
return "register"; // Stay on the form page if there are errors
}
// Proceed with further processing like saving to the database
model.addAttribute("message", "User registered successfully!");
return "success";
}
}
Explanation of the Controller
- The
@Valid
annotation tells Spring to validate theuser
object. - The
BindingResult
provides access to the validation results. Ifresult.hasErrors()
returns true, it means validation failed, and you should return the user to the registration form. - If the validation is successful, you can process the data (e.g., save to a database).
Step 4: Configure Spring MVC
You need to configure your Spring application to handle validation. This configuration usually exists in web.xml
or a Java configuration file.
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/views/"/>
<property name="suffix" value=".jsp"/>
</bean>
<bean id="validator" class="org.springframework.validation.beanvalidation.LocalValidatorFactoryBean"/>
Step 5: Create JSP Pages
You can create JSP pages to capture user input and display validation messages. Below is an example of a simple registration form (register.jsp
):
<%@ taglib uri="http://www.springframework.org/tags/form" prefix="form" %>
<!DOCTYPE html>
<html>
<head>
<title>Registration Form</title>
</head>
<body>
<h2>User Registration</h2>
<form:form action="register" modelAttribute="user" method="post">
<table>
<tr>
<td>Name:</td>
<td>
<form:input path="name"/>
<form:errors path="name" cssClass="error"/>
</td>
</tr>
<tr>
<td>Email:</td>
<td>
<form:input path="email"/>
<form:errors path="email" cssClass="error"/>
</td>
</tr>
<tr>
<td>Age:</td>
<td>
<form:input path="age"/>
<form:errors path="age" cssClass="error"/>
</td>
</tr>
<tr>
<td></td>
<td>
<input type="submit" value="Register"/>
</td>
</tr>
</table>
</form:form>
</body>
</html>
Error Handling and User Feedback
Handling validation errors properly is key to improving user experience. In the above JSP, we've used <form:errors>
to display error messages directly associated with each field. This allows users to see exactly what they need to correct.
Customizing Error Messages
You can customize error messages inside your model class, which we already included in the annotations. This practice makes it easy to manage and change messages without altering the main logic.
Moreover, you can externalize the messages in a messages.properties
file to support internationalization. Simply declare your messages file in the ResourceBundleMessageSource
.
<bean id="messageSource" class="org.springframework.context.support.ResourceBundleMessageSource">
<property name="basename" value="messages"/>
</bean>
The Closing Argument
Mastering form validation in Spring 3 applications enhances both data integrity and user experience. Understanding how to set up, implement, and handle validation effectively allows developers to create robust applications. Each step, from defining validation rules in model classes to providing user feedback in views, plays a pivotal role.
For further reading on form validation in Spring, check out the official Spring Framework Documentation.
Incorporate these validation strategies into your Spring applications, and enjoy cleaner inputs with fewer errors, ultimately building a more user-friendly application. Happy coding!
Checkout our other articles