Why Testing Private Methods Can Break Your Code
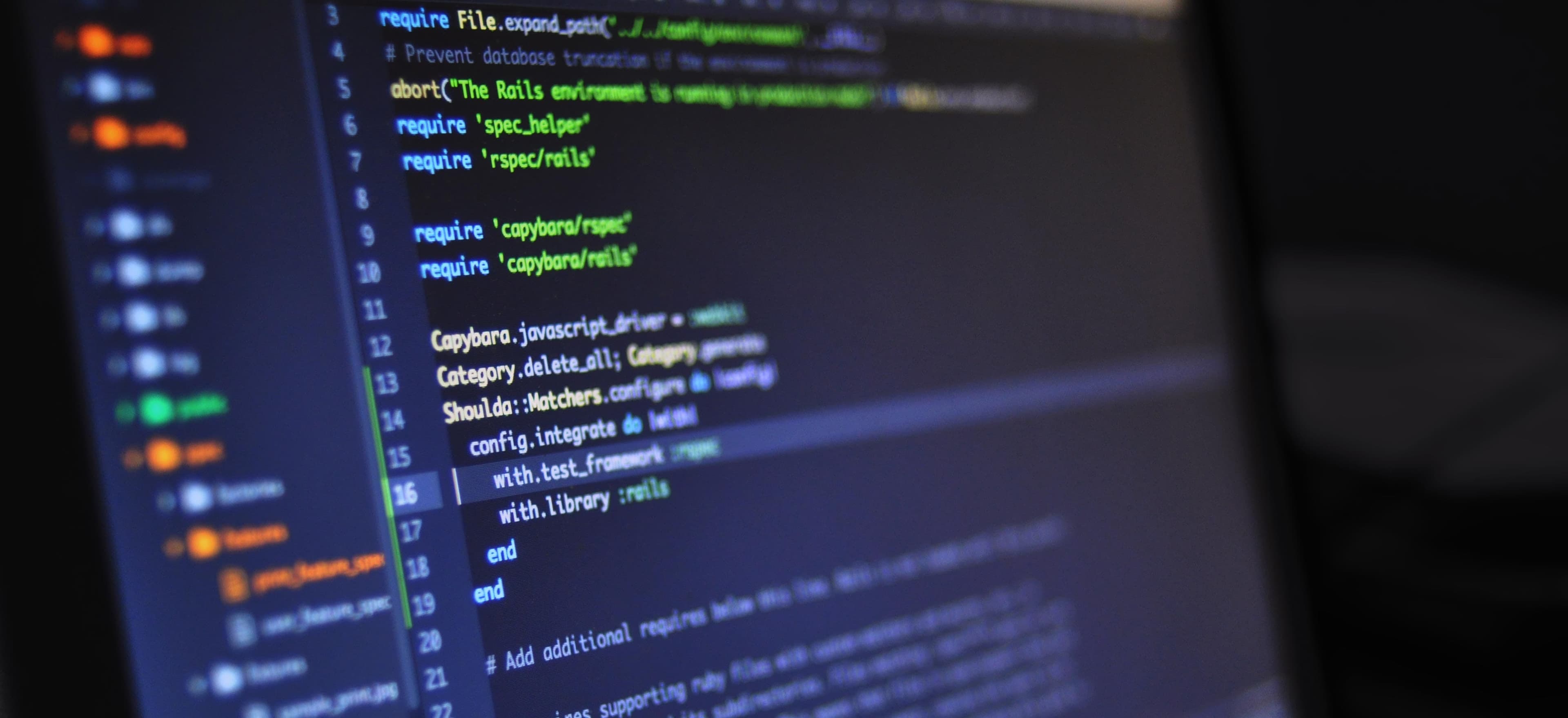
- Published on
Why Testing Private Methods Can Break Your Code
In the software development world, code reliability and maintainability are of utmost importance. One of the fundamental practices to ensure these qualities is testing. However, a common dilemma developers face is whether to test private methods. While it may seem like a practical approach to ensure high code coverage, testing private methods can lead to significant complications in your code. Let’s explore why.
Understanding the Java Class Structure
Before delving into the implications of testing private methods, it’s essential to understand the structure of a typical Java class. In Java, a class can include fields, methods, constructors, and nested classes. Here is a simple example:
public class Calculator {
private int add(int a, int b) {
return a + b;
}
public int addAndMultiply(int a, int b, int c) {
return add(a, b) * c;
}
}
The Private Method
In the above code, the add
method is a private method, meaning it is only accessible within the Calculator
class. Private methods are intended to encapsulate functionality that should not be part of the public API. The addAndMultiply
method serves as a public interface, allowing other classes to access the functionality while keeping the implementation details hidden.
The Benefits of Encapsulation
Encapsulation is a core principle of Object-Oriented Programming (OOP). It hides complex implementation details and exposes only what is necessary for the user. Testing private methods can undermine this principle, leading to a tightly coupled codebase that is more challenging to maintain and refactor. Here are some benefits of encapsulation:
- Simplifies Interfaces: Users interact with a clean interface while the underlying complexity remains hidden.
- Improved Code Maintainability: Changes in private methods do not necessarily affect external code, allowing for safer refactoring.
- Enhanced Flexibility: Private methods can be modified without breaking any external contracts.
Why Testing Private Methods is Problematic
Testing private methods directly can lead to several significant issues:
1. Breaking Encapsulation
When you write tests that directly access private methods, you violate the encapsulation principle. Here’s an example of how testing private methods can lead to a fragile test suite:
import org.junit.jupiter.api.Test;
import java.lang.reflect.Method;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@Test
public void testAdd() throws Exception {
Calculator calculator = new Calculator();
Method method = Calculator.class.getDeclaredMethod("add", int.class, int.class);
method.setAccessible(true);
int result = (int) method.invoke(calculator, 2, 3);
assertEquals(5, result);
}
}
While the above code does allow you to test the private method add
, it breaks encapsulation since add
is no longer hidden from the test. Keeping the implementation details exposed can lead to tests that are too brittle.
2. Fragile Tests
When you test private methods, any change to that method becomes a potential risk for test failures, even if the public behavior of your class remains unchanged. This results in a fragile test suite that requires continuous adjustments whenever internal implementations change.
3. Overemphasis on Implementation
Moreover, direct testing of private methods shifts the focus from testing what matters—public behavior—to how things work behind the scenes. Tests that focus on private methods often become tightly coupled to the implementation, making it harder to spot issues that impact overall functionality.
4. Increased Complexity
The complexity of your codebase increases as you introduce more tests around private methods, leading to possible confusion. This utilitarian approach may seem efficient at first. However, maintaining and understanding your tests becomes challenging over time, especially for new developers.
A Better Approach: Test Public Methods
Instead of focusing on private methods, put your energy into testing public methods. Here’s how you can effectively test the addAndMultiply
method:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@Test
public void testAddAndMultiply() {
Calculator calculator = new Calculator();
int result = calculator.addAndMultiply(2, 3, 4);
assertEquals(20, result); // Expected (2 + 3) * 4 = 20
}
}
Why This Is Better
- Focus on Behavior: By concentrating on testing what public methods do, you validate the behavior expected from a user’s perspective.
- Maintain Encapsulation: You keep your class structure intact and the details of implementation hidden, preserving the integrity of your design.
- Less Frequent Test Failures: Tests will fail only when the public contract changes, leading to fewer headaches when refactoring your code.
My Closing Thoughts on the Matter
While it may be tempting to test private methods in Java (or any programming language), it is usually not recommended. Testing private methods can break encapsulation, create fragile tests, emphasize implementation over behavior, and increase code complexity. Instead, focus on testing public methods to validate the functionality from an end-user standpoint. This practice enhances your code quality, makes refactoring easier, and generally leads to a healthier codebase.
For more in-depth information on Java testing practices, check out the official Java Testing Guide from Oracle, and consider reading "Effective Java" by Joshua Bloch for best practices in Java programming.
Remember, keep your tests intuitive and focused on testing behavior, and your code will thank you in the long run!