Understanding Garbage Collection Issues in Java Applications
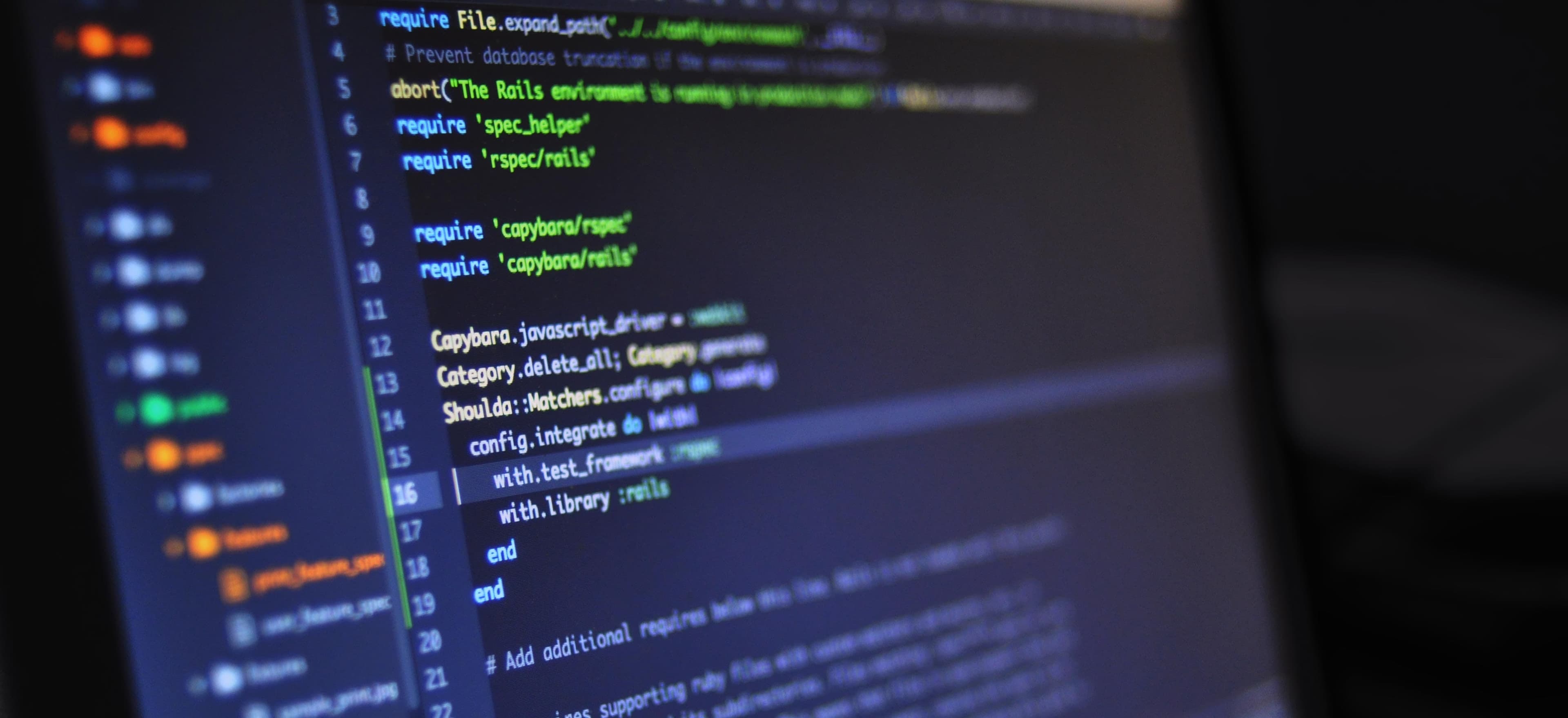
- Published on
Understanding Garbage Collection Issues in Java Applications
In the world of Java programming, memory management is a crucial aspect that developers must grasp. One of the key features Java provides is automatic garbage collection. It simplifies memory management by automatically reclaiming memory occupied by objects that are no longer in use. However, while this feature alleviates many concerns, it also introduces its own challenges. In this blog post, we'll delve into garbage collection issues in Java applications, exploring what they are, their implications, and how you can effectively manage them.
What is Garbage Collection?
Garbage Collection (GC) is the process of automatically deallocating memory that is no longer needed by a program. When objects are created in Java, they reside in the heap memory. Over time, objects can become obsolete or unreachable, meaning they can be removed from the memory to make room for new objects. The Java Virtual Machine (JVM) handles this process, which allows developers to focus more on coding than on memory management.
The Basics of How Garbage Collection Works
Java's garbage collectors employ different algorithms to identify objects that are no longer referenced. The most common algorithms are:
- Mark and Sweep: This method marks all reachable objects and then sweeps through the heap to remove the unmarked ones.
- Copying Collector: This method divides memory into two halves and copies live objects from one half to another, compacting them in the process.
- Generational GC: Java's garbage collectors usually optimize performance by implementing a generational approach, separating objects by age (young vs. old generations).
Understanding these processes is essential for optimizing your application's performance and managing memory effectively.
Common Garbage Collection Issues in Java
Garbage collection can provoke several issues that may negatively impact the performance of your Java applications. Here are a few common pitfalls:
1. Long GC Pauses
One of the most noticeable issues is long garbage collection pauses. When the JVM invokes GC, it can halt application threads to reclaim memory, especially during Full GC events.
Example
Consider a scenario in which you allocate and manipulate large objects within your program. If the objects aren't reclaimed promptly, you could encounter a Full GC, leading to extended application downtime. Here's an example of how memory allocation can lead to GC-related performance dips:
public class MemoryIntensiveTask {
public static void main(String[] args) {
int[] largeArray = new int[10000000]; // Allocate large array
// Perform operations...
// When out of scope, GC will kick in
}
}
Why it Matters: A long GC pause disrupts user experience and can lead to timeouts in services. This can greatly affect critical applications like banking systems, real-time monitoring tools, and web servers.
2. Memory Leaks
A memory leak occurs when an application holds references to objects that should be garbage collected, thereby preventing them from being reclaimed. This can gradually consume memory, leading to OutOfMemoryError exceptions.
Example
The following example illustrates how holding onto objects can create a memory leak:
import java.util.HashMap;
import java.util.Map;
public class MemoryLeakExample {
private Map<Long, Object> cache = new HashMap<>();
public void cacheObject(Long id, Object object) {
cache.put(id, object);
}
// Object is never cleared from the cache
}
Why it Matters: Memory leaks can silently accumulate over time, eventually resulting in performance degradation and application crash, particularly in long-running applications.
3. Excessive Object Creation
Efficient memory use is vital for maintaining application performance. Excessive creation of objects can ramp up garbage collection frequency, which, in turn, can lead to frequent pauses.
Example
If you're frequently creating and discarding objects in a loop, the strain on the garbage collector can be significant.
public class ExcessiveCreation {
public static void main(String[] args) {
for (int i = 0; i < 10000; i++) {
String str = new String("Example String " + i);
// Do something with str, but it becomes eligible for GC immediately
}
}
}
Why it Matters: If you need to create numerous objects, consider object pooling or singletons to mitigate the creation pressure.
Strategies for Managing Garbage Collection Issues
Now that you've seen the potential issues garbage collection can pose, let's explore strategies for managing them effectively.
1. Optimize Object Creation
Reduce Object Creation Frequency: Instead of creating new objects continuously, reuse existing objects when possible. This can significantly reduce the load on the garbage collector.
2. Use Profiler Tools
Use Java Profilers: Tools like VisualVM, Eclipse MAT, and JProfiler allow developers to analyze memory usage, track down memory leaks, and visualize garbage collection events. This insight is invaluable for debugging and optimizing your application's memory management.
3. Choose the Right Garbage Collector
Depending on your application's requirements, you may want to select a specific garbage collector that suits your needs. For instance, the G1 Garbage Collector is designed for high-throughput applications with minimal pause times. You can specify the collector while starting the JVM:
java -XX:+UseG1GC -jar YourApplication.jar
4. Tune Garbage Collection Parameters
The JVM provides extensive tuning parameters for garbage collection. For instance, you can adjust the size of the heap, set a maximum pause time goal, or even change the ratio between young and old generations. Here is how you can set up the maximum heap size:
java -Xmx1024m -Xms512m -jar YourApplication.jar
5. Avoid Static References
Avoid using large static references whenever possible. Static fields are bound to the class lifecycle and can prevent objects from being garbage collected.
6. Clean Up Resources
Ensure that resources like database connections, file handles, and network sockets are closed appropriately to facilitate memory reclamation.
7. Monitor Garbage Collection Over Time
Keep an eye on GC logs to analyze how often garbage collection occurs, types of collectors used, and overall memory usage over time. Use flags like -XX:+PrintGCDetails
to enable detailed logging.
Wrapping Up
Garbage collection is a powerful feature of Java, and while it alleviates many challenges regarding memory management, it can also introduce complications. Understanding the intricacies of garbage collection, recognizing potential issues, and employing effective strategies ensures that your Java applications run smoothly and efficiently. Whether you are building a small application or a large enterprise system, mastering these concepts will significantly enhance your application's performance and resilience.
For more in-depth information about Java garbage collection, you may find these resources useful:
- Java Garbage Collection Basics
- Understanding Java Memory Management
- Java Garbage Collection Tuning
By addressing garbage collection issues proactively, you can improve your application’s performance, ensuring that it meets both user needs and business requirements effectively. Happy coding!
Checkout our other articles