Effortless Java 8 File Processing: Tips for Common Pitfalls
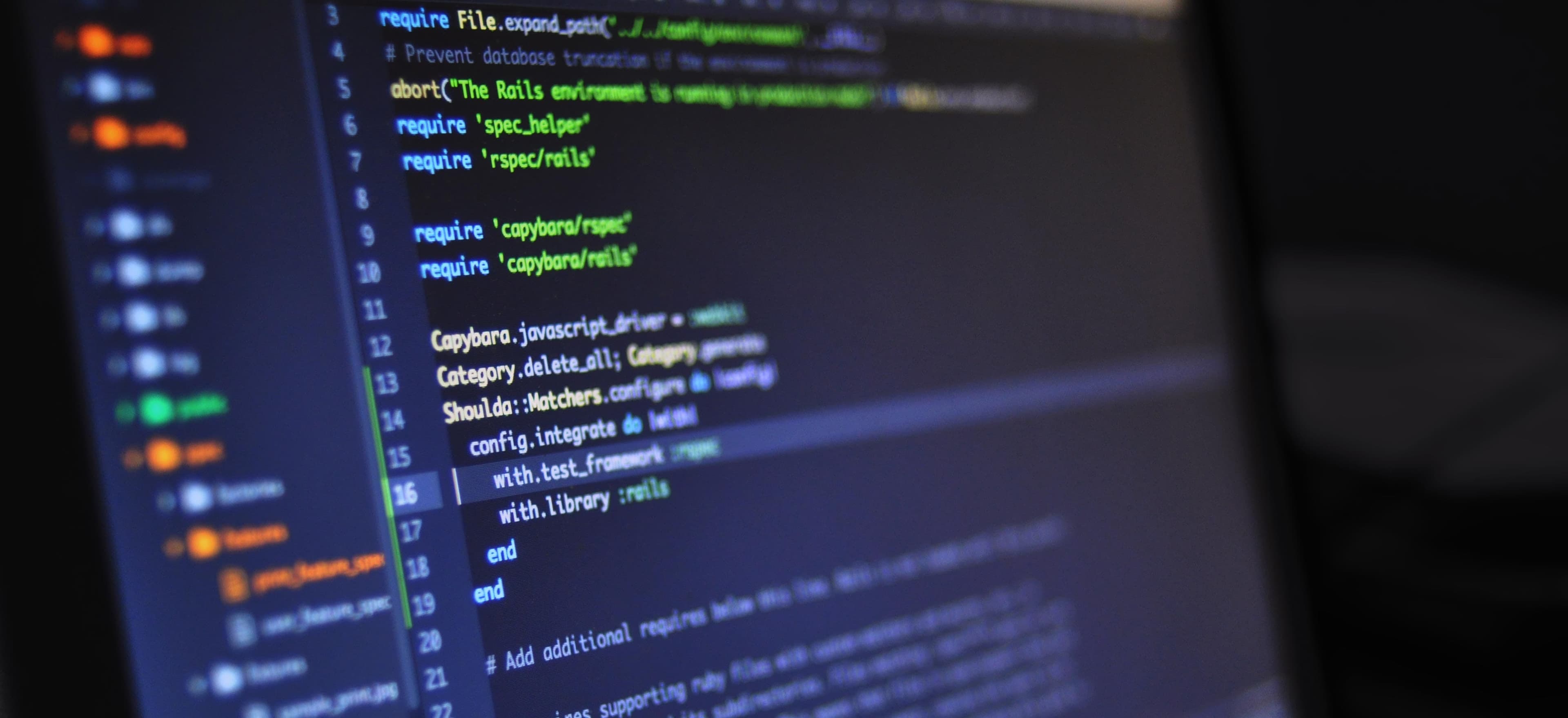
- Published on
Effortless Java 8 File Processing: Tips for Common Pitfalls
Java 8 introduced a plethora of features that fundamentally changed the way we write code, especially with the introduction of the Stream API and the enhancements to java.nio.file package. File processing, often considered a tedious task, has become easier and more intuitive. However, there are common pitfalls developers encounter, which this post aims to address.
Understanding the Basics
Before diving into common pitfalls, let's quickly review the core functionalities offered by Java 8's file processing capabilities. Java NIO (New Input/Output) allows developers to handle file and directory access efficiently. The Files class introduced many static methods to read, write, and manipulate files, while the Stream API makes it easier to process sequences of elements.
Basics of File I/O with Java 8
When working with files, it is crucial to understand how to read from and write to them effectively. Below is an example of how to read all lines from a file into a List.
import java.nio.file.Files;
import java.nio.file.Paths;
import java.io.IOException;
import java.util.List;
public class FileReadExample {
public static void main(String[] args) {
try {
List<String> lines = Files.readAllLines(Paths.get("example.txt"));
lines.forEach(System.out::println);
} catch (IOException e) {
System.err.println("Error reading the file: " + e.getMessage());
}
}
}
Commentary on the Code:
- Files.readAllLines: This method reads all lines from a file, returning them as a List. This is a straightforward way to get file content into memory.
- paths.get: This method converts a string path to a Path object.
- Error Handling: Using a try-catch block to handle potential
IOExceptions
is essential to ensure that your application doesn't crash during file operations.
Common Pitfalls in Java 8 File Processing
While working with Java 8 file processing can be straightforward, many developers hit roadblocks due to common pitfalls. Here are some of the most frequently encountered issues along with solutions.
1. Forgetting to Close Resources
Java 7 introduced the try-with-resources statement to automatically close resources, which you should always use for file processing. In Java 8, it's still relevant. Failing to close resources can lead to memory leaks and file locks.
Here's the proper usage:
import java.nio.file.Files;
import java.nio.file.Paths;
import java.io.IOException;
import java.io.BufferedReader;
public class FileReadWithResources {
public static void main(String[] args) {
try (BufferedReader reader = Files.newBufferedReader(Paths.get("example.txt"))) {
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
System.err.println("Error reading the file: " + e.getMessage());
}
}
}
Commentary on the Code:
- try-with-resources: This automatically closes the
BufferedReader
when done, preventing resource leaks. - BufferedReader: It's more efficient for reading lines from a file compared to other methods.
2. Reading Large Files into Memory
When dealing with large files, reading the entire file into memory can lead to OutOfMemoryError
. Instead, you should read files in chunks or line by line.
Below is a code snippet reading a file line by line and processing each line immediately:
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.stream.Stream;
public class FileStreamProcessing {
public static void main(String[] args) {
try (Stream<String> stream = Files.lines(Paths.get("largefile.txt"))) {
stream.filter(line -> line.contains("Java"))
.forEach(System.out::println);
} catch (IOException e) {
System.err.println("Error processing the file: " + e.getMessage());
}
}
}
Commentary on the Code:
- Files.lines: This creates a lazily populated Stream of lines from the file. Lines are read only when needed, making it suitable for large files.
- Filter: This demonstrates how to filter lines based on specific criteria (in this case, containing the word "Java").
3. Using the Wrong File Encoding
Another common pitfall is failing to specify the correct file encoding when reading text files. Java uses UTF-8 by default, but if your file uses a different encoding, you might end up with garbled text.
Here is an example of specifying the encoding explicitly:
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.List;
public class FileReadWithEncoding {
public static void main(String[] args) {
try {
List<String> lines = Files.readAllLines(Paths.get("example_utf16.txt"), StandardCharsets.UTF_16);
lines.forEach(System.out::println);
} catch (IOException e) {
System.err.println("Error reading the file: " + e.getMessage());
}
}
}
Commentary on the Code:
- StandardCharsets.UTF_16: This specifies the character encoding for reading the file, ensuring text is correctly interpreted.
Additional Resources
While the above examples cover fundamental pitfalls, there are numerous resources available for deeper insights:
- Official Java Documentation on java.nio.file offers extensive information on file operations and methodologies.
- Java 8 Stream API Documentation provides an extensive look into the capabilities offered by the Stream API.
The Closing Argument
With Java 8’s advancements in file processing, working with files has indeed become more manageable. However, vigilance is key. By recognizing common pitfalls and implementing best practices, you can ensure efficient, error-free file handling.
Don’t forget to utilize the Java 8 features to their full potential, keeping in mind resource management, file size, and encoding. Happy coding!
Checkout our other articles