Mastering Java Future: Avoiding Common Pitfalls
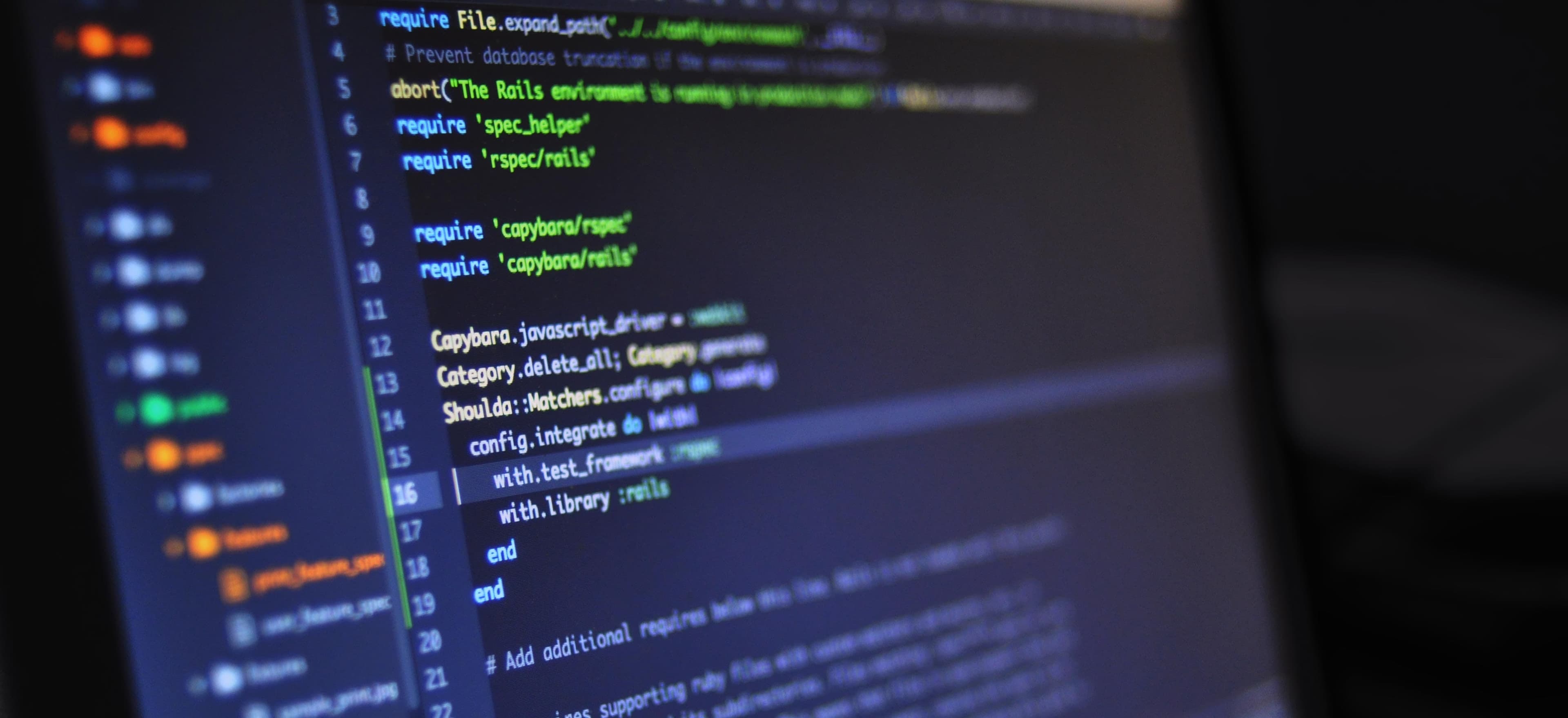
- Published on
Mastering Java Future: Avoiding Common Pitfalls
Java is renowned for its versatility and robustness, making it a favorite for developers aiming to build high-performance applications. With the introduction of concurrency features in Java, particularly the Future
interface, managing asynchronous tasks has never been easier. However, like all powerful tools, it requires careful handling. In this blog post, we will delve into the pitfalls associated with the Future
interface in Java and how to master its usage.
Understanding the Future Interface
The Future
interface, found in the java.util.concurrent
package, represents the result of an asynchronous computation. When you submit a task to an ExecutorService
, you typically receive a Future
object back. This object allows you to retrieve the result of the computation once it is complete, check if it's complete, or cancel the task if necessary.
Here’s a simple example:
import java.util.concurrent.*;
public class FutureExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newSingleThreadExecutor();
Future<Integer> future = executor.submit(() -> {
Thread.sleep(1000); // simulating long task
return 123;
});
try {
Integer result = future.get(); // this blocks until the result is available
System.out.println("Task completed with result: " + result);
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
} finally {
executor.shutdown();
}
}
}
Commentary on the Code
- Asynchronous Execution: The
submit()
method allows for asynchronous execution, which is one of the main advantages of usingFuture
. - Blocking Call: The
get()
method is a blocking call. It waits until the result is available. This could lead to deadlocks or unnecessary waits if not handled correctly. - Thread Management: Using
ExecutorService
is crucial for managing thread lifecycles efficiently.
Despite these advantages, improper management of Future
objects can lead to several pitfalls. Let’s discuss the common mistakes and how to avoid them.
Common Pitfalls of Using Future
1. Ignoring Exception Handling
One of the most significant overlooked aspects when working with Future
is exception handling. If the task fails, the exception will be encapsulated in the ExecutionException
when calling get()
. Failing to handle it appropriately could lead to silent failures in your application.
try {
Integer result = future.get();
} catch (ExecutionException e) {
// Here’s where we capture the actual exception causing the task to fail
Throwable cause = e.getCause();
System.err.println("Task failed with exception: " + cause);
}
Why This Matters: Proper exception handling allows you to understand the context of any failure and possibly recover from it or log an appropriate error message.
2. Overlooking Task Cancellation
Future provides the ability to cancel executing tasks using cancel()
, but many developers overlook this capability. Remember, cancelling a task does not guarantee its termination if it’s already running.
boolean isCancelled = future.cancel(true); // true indicates to interrupt if running
Why This Matters: Timely cancellation of tasks can prevent unnecessary resource consumption and improve application responsiveness.
3. Blocking the Main Thread
As noted earlier, calling get()
on a Future
is a blocking operation. Relying on it without considering its implications can lead to poor user experience. Instead, use it wisely by implementing timeouts.
try {
// setting a timeout of 2 seconds
Integer result = future.get(2, TimeUnit.SECONDS);
} catch (TimeoutException e) {
System.err.println("Task timed out");
} catch (ExecutionException e) {
// handle execution exception
}
Why This Matters: A timeout allows your application to remain responsive, even if a particular task takes longer than expected.
4. Using Future for Long-lived Tasks
While Future
is an excellent choice for short-lived tasks, it can become a problem for long-running tasks. Because Future
represents a single computation, managing the lifecycle and state of long-lived tasks can introduce complexity.
Instead of Future
, consider using other constructs like CompletableFuture for better flexibility and additional features such as running tasks in a chain or combining multiple tasks efficiently.
5. Not Understanding the Effects of Thread Pool Size
Using ExecutorService
improperly can lead to performance degradation. If your thread pool size is too small, tasks will queue. Conversely, too large a pool can lead to excessive context switching, resulting in poorer performance.
ExecutorService executor = Executors.newFixedThreadPool(10); // adjust based on the task demand
Why This Matters: Tuning the thread pool size according to the workload is crucial for maximizing throughput and application efficiency.
6. Inadequate Cleanup
Forgetting to shut down the ExecutorService
can lead to resource leaks and potential memory issues. Always ensure proper cleanup of your executor.
executor.shutdown(); // recommended to call this in a finally block
Why This Matters: Failing to shut down an executor can cause Java applications to hang or consume resources even after they have finished executing.
Leveraging CompletableFuture
In recent versions of Java, CompletableFuture
provides a more powerful alternative to Future
that allows for non-blocking asynchronous programming. Here's a quick example:
import java.util.concurrent.CompletableFuture;
public class CompletableFutureExample {
public static void main(String[] args) {
CompletableFuture<Integer> future = CompletableFuture.supplyAsync(() -> {
// Simulating a long running task
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
throw new IllegalStateException("Task interrupted", e);
}
return 42;
});
// If the task fails, handle the error gracefully
future.exceptionally(e -> {
System.err.println("Task failed: " + e.getMessage());
return null;
}).thenAccept(result -> System.out.println("Task completed with result: " + result));
}
}
Why Use CompletableFuture?
- Chaining: You can easily chain multiple computations together.
- Exception Handling: Built-in methods for handling exceptions.
- Non-blocking: It is designed for non-blocking computations.
For more information, check out the Java Documentation on CompletableFuture.
A Final Look
Mastering the Future
interface in Java involves understanding its nuances and potential pitfalls. By practicing careful exception handling, wisely managing cancellation and blocking calls, and tuning your thread pools, you can leverage Future
effectively. For more complex needs, consider transitioning to CompletableFuture
for a more versatile approach to asynchronous programming.
With these insights and strategies, you’ll elevate your Java programming skills, build more reliable applications, and keep common pitfalls at bay. Happy coding!
Checkout our other articles