Overcoming the Pitfalls of Generics in Software Design
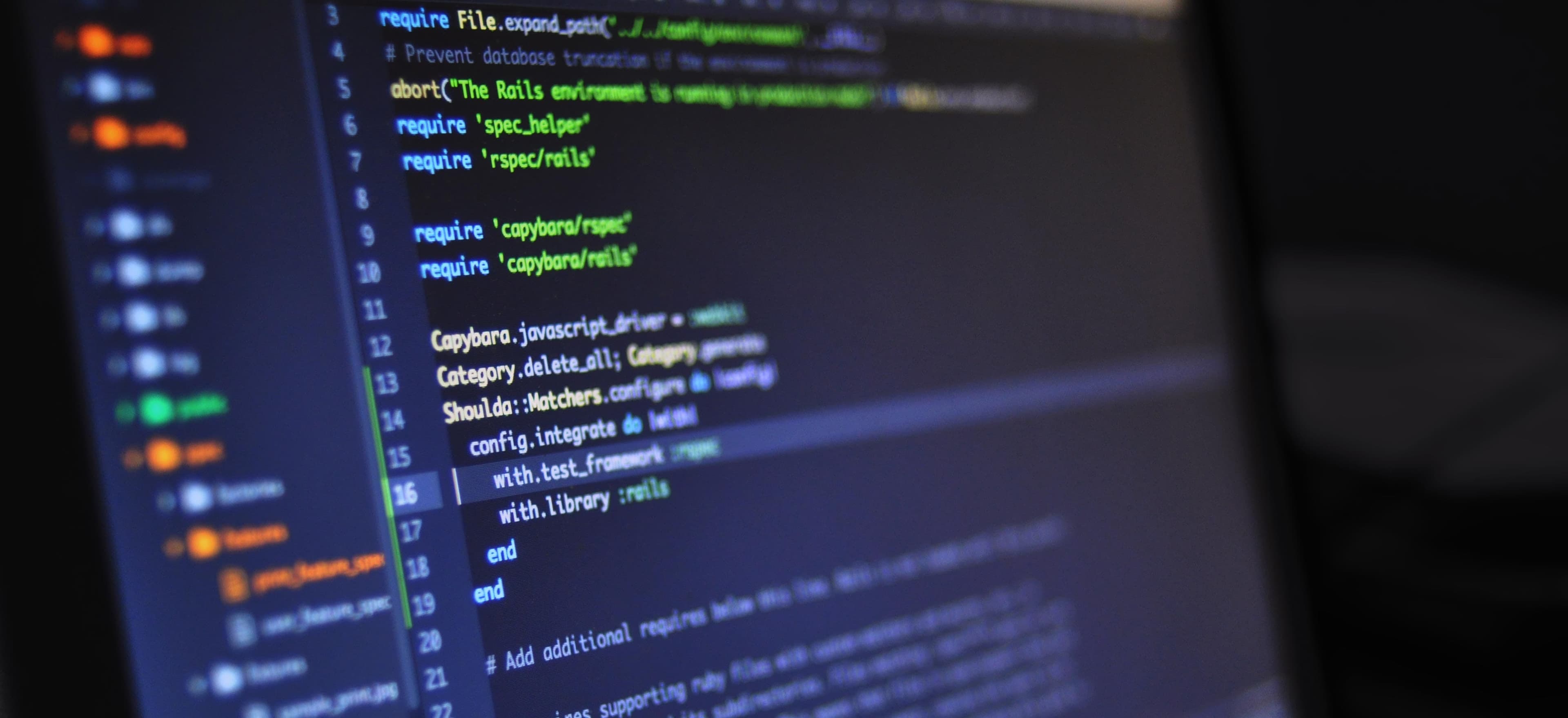
- Published on
Overcoming the Pitfalls of Generics in Software Design
Generics in Java offer a powerful way to achieve type safety and code reuse. However, they can also present challenges that, if left unaddressed, may lead to complicated code and runtime errors. In this blog post, we will explore the common pitfalls associated with generics and discuss strategies to overcome them, ensuring a smoother implementation in your software designs.
What are Generics?
Java introduced generics in version 1.5 to provide stronger type checks at compile time and to support generic programming. A generic type allows you to parameterize types, enabling classes, interfaces, and methods to operate on objects of various types while providing compile-time type safety.
Here's a quick example of how to define a simple generic class:
public class Box<T> {
private T item;
public void setItem(T item) {
this.item = item;
}
public T getItem() {
return item;
}
}
In this code snippet, T
is a type parameter that can be replaced with any object type when an instance of Box
is created. This feature allows you to create type-safe containers without sacrificing flexibility.
Common Pitfalls of Generics
1. Type Erasure
Type erasure is the process by which the Java compiler removes all generic type information during compilation. This means that generics are not available at runtime.
Consequence:
- It can be confusing, especially for developers expecting that the type information will be available at runtime.
- Certain operations, like creating generic arrays, are not allowed because of type erasure.
Solution:
Utilize collections, such as ArrayList
, instead of arrays. For example, instead of:
Box<String>[] boxes = new Box<String>[10];
Use:
List<Box<String>> boxes = new ArrayList<>();
Using collections allows more flexibility and adheres to Java's design philosophy.
2. Limitations of Wildcards
Wildcards allow for flexibility in using generics, but they come with their limitations and can lead to confusion.
Example:
Consider the following code:
public void printItems(List<?> items) {
for (Object item : items) {
System.out.println(item);
}
}
While this looks good, you cannot modify the contents of items
because of the wildcard ?
.
Solution:
To better utilize wildcards, leverage bounded wildcards when possible.
public void printNumbers(List<? extends Number> numbers) {
for (Number number : numbers) {
System.out.println(number);
}
}
This allows you to restrict the kinds of types that can be passed, ensuring that only numbers get printed, which is clearer and safer.
3. Inability to Instantiate Generic Types
You cannot create instances of generic types directly due to type erasure.
Example:
Attempting to instantiate a generic type like so will result in a compilation error:
public class GenericClass<T> {
T instance = new T(); // Compile-time error!
}
Solution:
You can create factory methods or use reflection to solve this problem.
public class GenericClass<T> {
private Class<T> type;
public GenericClass(Class<T> type) {
this.type = type;
}
public T createInstance() {
try {
return type.getDeclaredConstructor().newInstance();
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
}
By passing the Class
type to the constructor, we can create instances safely.
4. Confusion with Inheritance and Generics
When working with inheritance and generics, it’s easy to fall into the trap of thinking that subclasses can be used interchangeably with their superclass. This isn’t always true with generics.
Example:
If you have:
class Animal {}
class Dog extends Animal {}
class Box<T> {
private T item;
}
Box<Dog> dogBox = new Box<>();
Box<Animal> animalBox = dogBox; // Compile-time error!
Solution:
Use bounded wildcards to enable polymorphism when you want to create a more general usage.
public void addToAnimalBox(Box<? super Dog> animalBox) {
animalBox.add(new Dog());
}
This allows you to add a Dog
to a Box
that can hold an Animal
or any of its subtypes.
In Conclusion, Here is What Matters
Generics provide developers with the tools to optimize and enhance code quality through type safety and reusability. However, it’s vital to be aware of the common pitfalls that can arise when implementing generics in designs. By understanding the challenges of type erasure, the subtleties of wildcards, the restrictions on instantiating generic types, and the complexities of inheritance with generics, you can create more robust, maintainable code.
Always remember to use the strengths of generics to your advantage, while employing the solutions outlined in this post to mitigate their pitfalls.
Further Reading
To deepen your understanding of generics and explore more complex scenarios, consider checking out these resources:
By implementing these strategies, you'll significantly enhance your software design skills and gain confidence in using Java generics effectively. Happy coding!
Checkout our other articles