Avoiding Common Caching Pitfalls for Optimal Performance
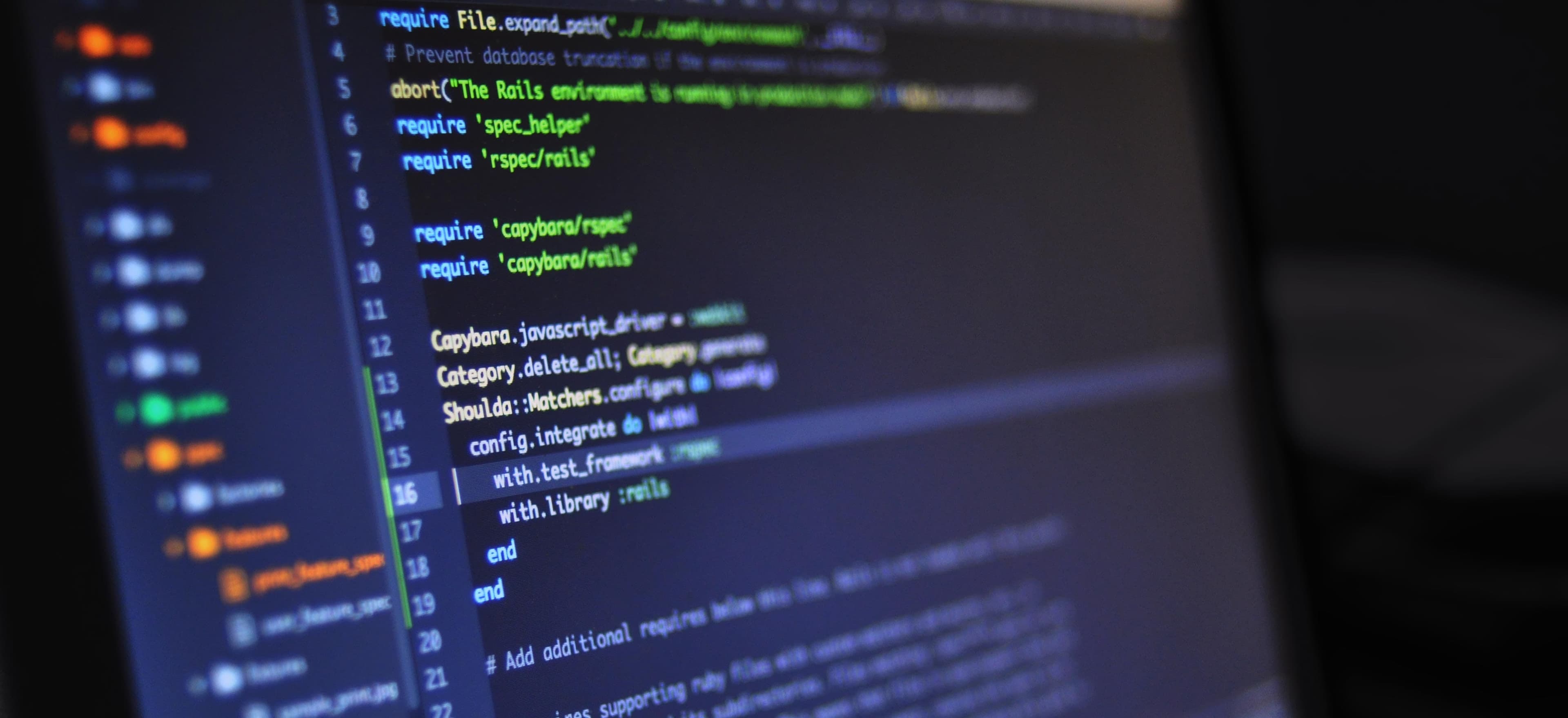
- Published on
Avoiding Common Caching Pitfalls for Optimal Performance in Java
Caching is a powerful technique used in software development to speed up application performance by storing frequently accessed data in a temporary location. When implemented correctly, it can drastically reduce database calls, decrease latency, and provide a smoother user experience. However, pitfalls abound when it comes to caching. In this blog post, we'll explore common caching mistakes made in Java applications and how to avoid them for optimal performance.
Understanding Caching
Before we delve into the pitfalls, it's essential to understand what caching is. Caching is the process of storing data in a location where it can be accessed more quickly than from its original source. In Java, this often involves storing results from expensive operations or database queries so that subsequent requests can respond faster.
The Java ecosystem provides several ways to implement caching, including:
- In-memory caches like Hazelcast or Ehcache
- HTTP caching mechanisms
- Database result caching
Each method has its pros and cons, and understanding these can help prevent performance issues down the line.
Common Caching Pitfalls
1. Not Understanding the Cache Scope
One of the most frequent mistakes developers make is not clearly defining the scope of their cache. This can lead to over-caching or under-caching.
Over-caching occurs when you store too much data in the cache, which can eventually lead to memory exhaustion:
Cache<String, User> userCache = CacheBuilder.newBuilder()
.maximumSize(1000)
.expireAfterAccess(10, TimeUnit.MINUTES)
.build();
Here, we define a maximum size of 1000 and an expiration policy of 10 minutes. These constraints help ensure we don’t consume more memory than necessary.
Under-caching, on the other hand, occurs when you limit the cache scope too severely, forcing excessive queries to the database. Always analyze performance and access patterns to determine the right scope for your caching strategy.
2. Ignoring Cache Expiration
Cache expiration is a critical aspect of caching that many developers ignore. Without proper expiry strategies in place, stale data can linger in your cache, leading to inconsistencies.
Consider using time-based expiration strategies:
CacheBuilder.newBuilder()
.expireAfterWrite(30, TimeUnit.MINUTES)
.refreshAfterWrite(10, TimeUnit.MINUTES)
.build();
Here, data is purged 30 minutes after being written to the cache and refreshed every 10 minutes. This dual strategy ensures you always have relatively fresh data without excessive database access.
3. Caching Everything
While it might be tempting to cache all the things — don’t! Not all data benefits from caching. In fact, caching large datasets, transient data, or infrequently accessed data can degrade performance rather than enhance it.
A good rule of thumb is to cache items that meet three criteria:
- The item is expensive to compute or retrieve.
- The item is used frequently.
- The item does not change often.
Use profiling tools to determine which parts of your application make expensive calls. Once identified, consider caching the results selectively.
4. Failing to Monitor Cache Performance
After implementing caching, it’s vital to monitor its performance. Without continuous observation, you may run without knowing whether the cache meets your application's needs.
Using tools like JMX or Prometheus, you can gather metrics on hit rates, evictions, and overall memory usage.
Here’s an example of how to implement JMX monitoring:
public class CacheMonitor implements NotificationEmitter {
private final Cache<String, User> userCache;
public CacheMonitor(Cache<String, User> userCache) {
this.userCache = userCache;
}
@Override
public void emitCacheStats() {
System.out.println("Cache Size: " + userCache.size());
System.out.println("Cache Hit Rate: " + userCache.stats().hitRate());
}
}
By actively monitoring these parameters, you can make data-driven decisions about when and how to adjust your cache strategy.
5. Not Handling Cache Invalidation Properly
Cache invalidation is one of the trickiest aspects of caching. It’s crucial to ensure that when data changes, the corresponding cache entries are also invalidated or updated.
Inconsistent states can cause your application to serve stale data to users. This can lead to a poor user experience, and in the worst-case scenario, financial losses or security vulnerabilities.
Here’s how you can handle cache invalidation:
public void updateUser(User user) {
userRepository.update(user);
userCache.invalidate(user.getId());
}
In this example, whenever a user is updated in the database, the corresponding cache entry is invalidated. This ensures that subsequent requests will fetch the latest data.
6. Using the Wrong Cache Implementation
Java provides several caching libraries, such as Guava Cache, Ehcache, and Caffeine. Each comes with its features, strengths, and weaknesses.
Using the wrong implementation can lead to increased complexity or poor performance. For example, Guava Cache is lightweight and suitable for in-memory caching requiring minimal setup. In contrast, Ehcache or Caffeine may be more appropriate for more extensive applications that require distributed caching.
Example of using a Caffeine cache:
Cache<String, User> userCache = Caffeine.newBuilder()
.maximumSize(10_000)
.expireAfterWrite(10, TimeUnit.MINUTES)
.build();
Choosing the appropriate caching solution for your specific use case can significantly impact your application's performance.
Closing Remarks
Caching is an essential aspect of Java applications if done correctly. To achieve optimal performance, avoid common pitfalls such as over-caching, ignoring cache expiration, caching everything, failing to monitor cache performance, mishandling invalidation, and using the wrong caching implementation.
By understanding and leveraging these techniques effectively, you can create robust caching solutions that enhance the performance of your Java applications. For further reading, you might find this comprehensive guide on application performance helpful.
Incorporate these strategies into your applications, test, monitor, and refine your caching strategies constantly. In the world of development, staying proactive is key!