Struggling with Code Clarity? Tips for Better Style!
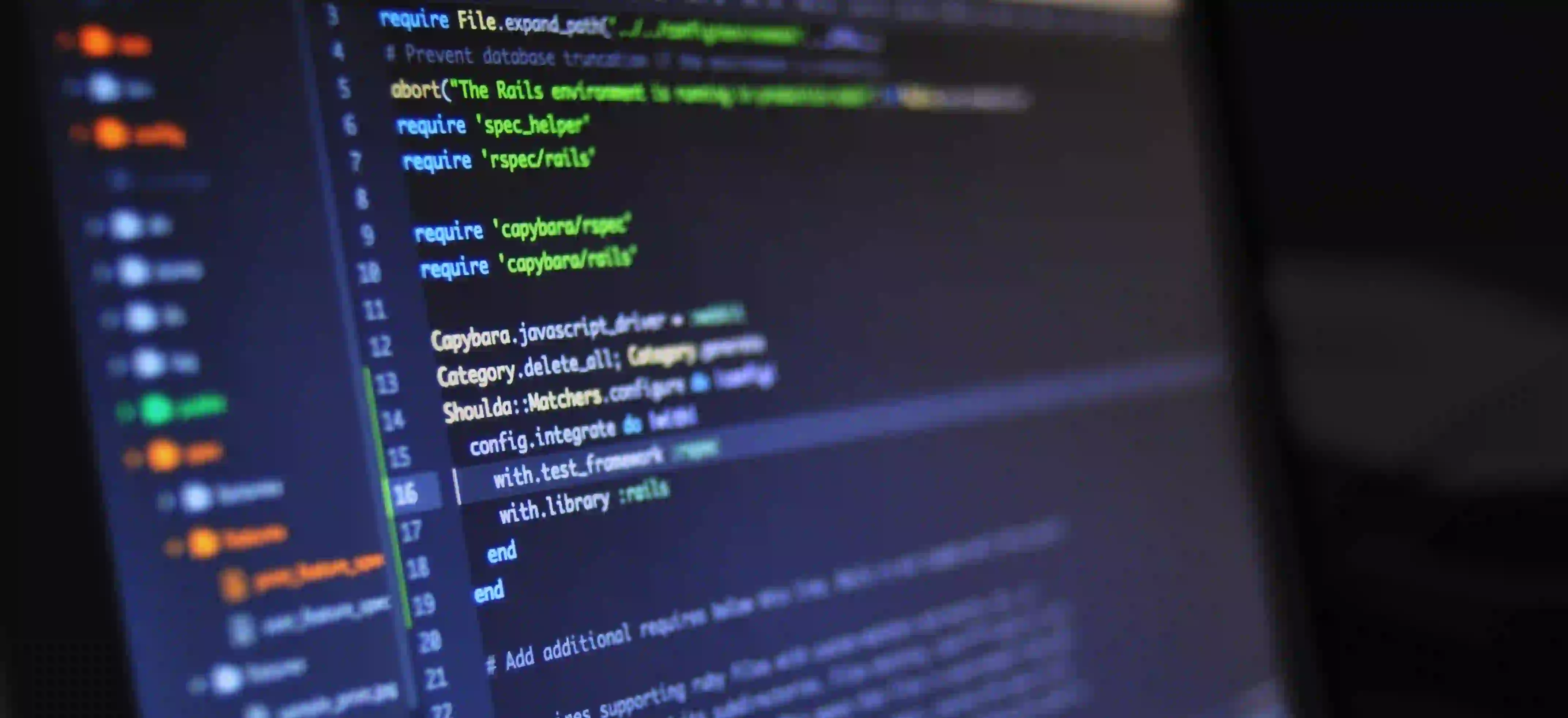
Struggling with Code Clarity? Tips for Better Style!
When it comes to software development, clarity in code is as crucial as its functionality. They say you are only as strong as your weakest link, and in programming, that link can often be tangled and convoluted code. Luckily, coding style is an area where we can significantly improve. This blog post aims to provide you with actionable tips to write more understandable and maintainable Java code.
Why is this important? Code that is clear and well-styled is easier to read, easier to maintain, and helps improve collaboration among developers.
Importance of Code Clarity
Before diving into the tips, it is essential to understand the significance of clear code. According to the Pragmatic Programmer, "good programmers write code that humans can understand." When you consider that code is often read more frequently than it is written, this becomes even more critical. Clarity leads to easier debugging, more manageable updates, and smoother team collaborations.
Key Elements of Code Clarity:
- Consistency: A consistent style makes code easier to read and understand.
- Descriptive Naming: Meaningful variable names reduce the cognitive load required to understand the code.
- Modularity: Breaking down complex systems into smaller, more manageable pieces enhances readability.
Now that we've outlined the importance of code clarity, let’s dive into some practical tips.
1. Use Meaningful Variable and Class Names
Naming conventions should always be at the forefront of a developer's mind. The right name can convey the purpose of a variable or class effectively.
Example: Bad vs Good Naming
// Bad
int a;
int b;
// Good
int numberOfUsers;
int maxLoginAttempts;
Why This Matters: Meaningful names give context. A variable named numberOfUsers
instantly informs the reader about its purpose, reducing confusion.
Java Naming Conventions
- Classes should be nouns and use CamelCase (e.g.,
UserAccount
). - Methods should be verbs and also use CamelCase (e.g.,
calculateBalance
). - Constants should be in uppercase letters with underscores (e.g.,
MAX_CONNECTIONS
).
2. Follow Consistent Indentation and Spacing
Consistency in formatting greatly enhances code readability. Whether your team prefers tabs or spaces, stick to one approach.
Example: Indentation Inconsistency
// Bad
if (isValid) {
System.out.println("Valid");
}
else {
System.out.println("Invalid");
}
// Good
if (isValid) {
System.out.println("Valid");
} else {
System.out.println("Invalid");
}
Why This Matters: Proper indentation and spacing help in visually structuring the code, making it easier to navigate.
Tools for Consistency
Consider using code style tools such as Checkstyle or PMD to enforce coding standards across your project.
3. Make Use of Comments Wisely
While code should be self-explanatory, sometimes additional context is required. Comments can bridge the gap.
Example: Effective Comments
// Calculate the user's balance after applying monthly fees
public double calculateBalance(double initialBalance) {
return initialBalance - monthlyFees;
}
Why This Matters: Comments should explain the "why" behind complex logic. Over-commenting or stating the obvious, however, can clutter your code.
Best Practices for Comments
- Use comments to explain the purpose of complex blocks.
- Avoid stating what the code itself is doing unless it's not immediately clear.
- Keep comments updated; outdated comments can lead to misunderstandings.
4. Use Consistent Error Handling
In Java, properly handling exceptions is crucial for robust applications. Consistency in your error-handling strategy can improve readability.
Example: Inconsistent Error Handling
// Bad
try {
doSomething();
} catch (IOException e) {
// log the error
}
try {
doSomethingElse();
} catch (Exception ex) {
// handle the error
}
// Good
try {
doSomething();
} catch (IOException e) {
logger.error("An IOException occurred", e);
} finally {
cleanup();
}
Why This Matters: Consistent error handling helps future developers understand the risks and mitigation strategies across the entire codebase.
Specificity Matters
Be as specific as possible in your catch blocks. Catching generic Exception
is usually considered bad practice because it can hide bugs.
5. Modularize Your Code
Rather than writing monolithic methods, break your code into smaller, reusable methods. This approach enhances readability and maintainability.
Example: Monolithic vs Modular
// Bad
public void processUser(int userId) {
// fetch user from database
// validate user
// update user status
// send notification
}
// Good
public void processUser(int userId) {
User user = fetchUser(userId);
if(validateUser(user)) {
updateUserStatus(user);
sendNotification(user);
}
}
Why This Matters: Smaller methods are easier to test and understand. Each method can be explained in terms of a single responsibility, leading to better overall code quality.
6. Embrace Java Collections Wisely
Standardizing data structures can lead to better clarity. Using Java's Collection Framework effectively can enhance the code's readability.
Example: Avoiding Arrays When Possible
// Bad
String[] users = new String[3];
// Good
List<String> users = new ArrayList<>();
Why This Matters: The List
interface provides more useful methods than standard arrays, enabling easier manipulation and improved readability.
Know When to Choose
Understand when to use a Map
, List
, or Set
. Each has its own purpose and usage, and this knowledge results in clearer code.
Closing Remarks
Clarity in code is not just an aesthetic choice; it significantly enhances software quality. By following these tips, you can write Java code that is easier to read, maintain, and evolve.
To recap:
- Use meaningful and descriptive names
- Maintain consistent formatting
- Comment wisely
- Be uniform in error handling
- Modularize your code
- Leverage Java Collections effectively
For more information and tools related to coding style, check out Oracle’s Java Coding Conventions.
Feel free to share your thoughts and experiences on writing clear code! What practices do you find most effective? Happy coding!