Mastering Apache Camel: Avoiding Connection Pooling Pitfalls
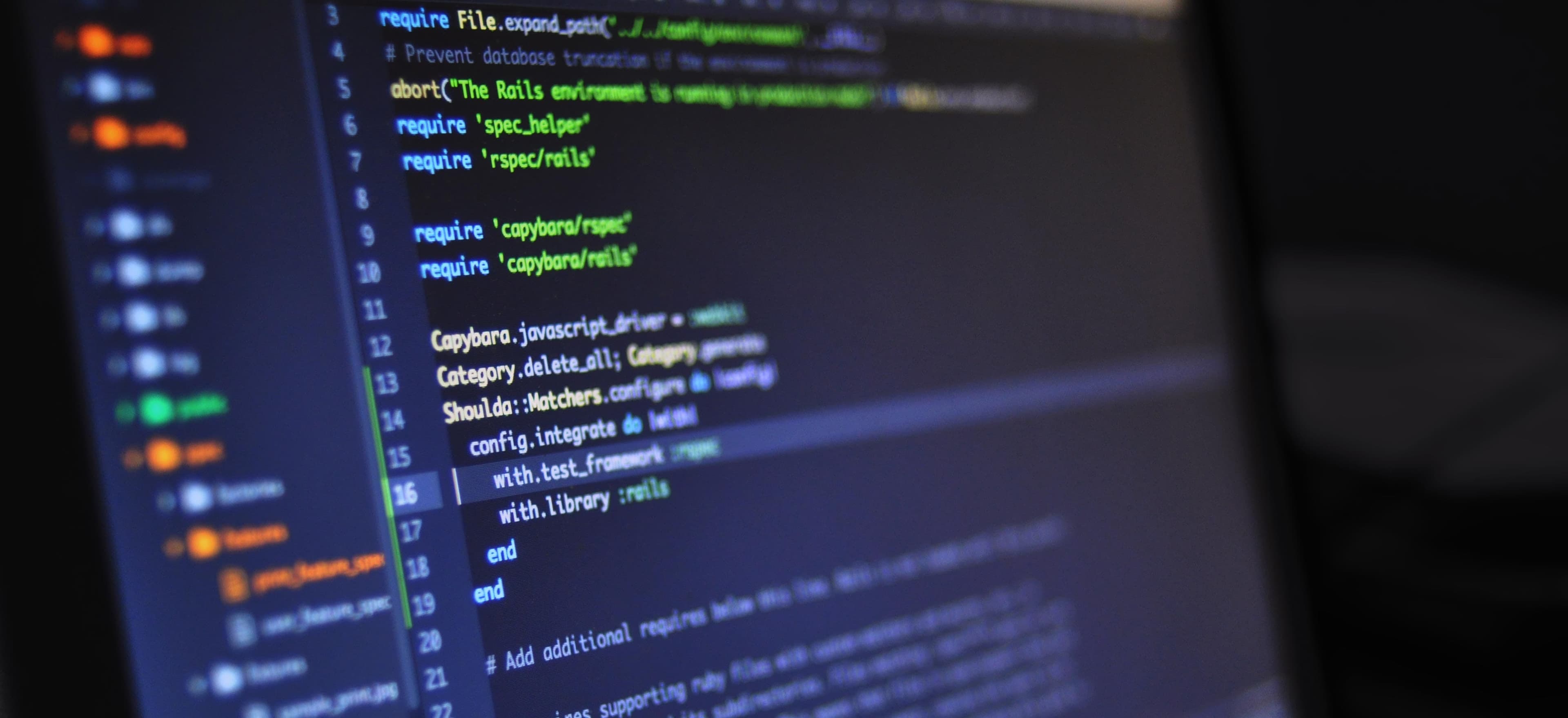
- Published on
Mastering Apache Camel: Avoiding Connection Pooling Pitfalls
Apache Camel is a powerful integration framework that simplifies the routing and mediation of various data streams. However, it also has its own set of complexities, especially when it comes to managing connections. One common challenge that developers face is connection pooling. Understanding how to effectively manage connection pools can significantly impact your application's performance and reliability. This blog post will elucidate connection pooling in Apache Camel, guide you through common pitfalls to avoid, and provide best practices to leverage its capabilities effectively.
What is Connection Pooling?
Connection pooling is a technique used to create a pool of reusable connections, which can be shared among various components of an application. Instead of opening and closing database connections frequently, a connection pool allows connections to be reused, thus enhancing performance and resource management.
In Apache Camel, various components—like JDBC, JMS, and others—rely on connection pooling. While it enhances efficiency, improper management can lead to resource exhaustion, memory leaks, and degraded performance.
The Importance of Proper Connection Management
Before diving into the pitfalls, it’s essential to grasp why connection management is crucial. Here are a few reasons:
- Performance: Decoupling the overhead of establishing new connections can boost performance.
- Resource Utilization: Avoids overloading the database or message broker with too many simultaneous connections.
- Scalability: A well-configured connection pool can handle variable loads efficiently.
Now, let’s iron out some common pitfalls associated with connection pooling in Apache Camel.
Common Pitfalls in Connection Pooling
1. Not Configuring Pool Size Correctly
One of the most significant pitfalls is failing to configure the connection pool size correctly. The default values are often insufficient for production environments, which leads to performance issues.
Why it Matters: An undersized pool can lead to ConnectionTimeoutExceptions
, while an oversized pool may result in resource contention and increased latency.
Solution: Always analyze your application's load and set appropriate min and max pool sizes.
<bean id="myDataSource" class="org.apache.commons.dbcp.BasicDataSource">
<property name="driverClassName" value="com.mysql.jdbc.Driver" />
<property name="url" value="jdbc:mysql://localhost:3306/mydb" />
<property name="username" value="user" />
<property name="password" value="password" />
<property name="initialSize" value="5" />
<property name="maxTotal" value="20" />
</bean>
2. Ignoring Connection Leaks
Connection leaks occur when an application fails to close connections under certain circumstances. This results in the depletion of available connections over time.
Why it Matters: Leaks are often hard to detect and can lead to a cascade of failures as the connection pool gets exhausted.
Solution: Always ensure that connections are closed after their use. A good practice is to use try-with-resources statements in Java, which automatically closes resources.
try (Connection conn = dataSource.getConnection()) {
// Execute your queries here
} catch (SQLException e) {
e.printStackTrace();
} // Connection is automatically closed
3. Overlooking Connection Validation Failures
A common mistake is not validating connections before use. Sometimes, connections in the pool may become stale or invalid, especially in long-running applications.
Why it Matters: Using an invalid connection can lead to unhandled exceptions and potential application crashes.
Solution: Configure the connection pool to validate connections before supplying them. Use a validationQuery
or testOnBorrow
parameter to check connection validity.
<property name="validationQuery" value="SELECT 1" />
<property name="testOnBorrow" value="true" />
4. Lack of Monitoring and Logging
Another frequent oversight is not monitoring connection pool metrics. Without metrics, it's challenging to understand the performance and health of your application regarding its connection usage.
Why it Matters: Monitoring would allow you to proactively address issues, rather than reactively troubleshooting when things go wrong.
Solution: Implement logging and monitoring tools like JMX (Java Management Extensions) to monitor connection pool statistics.
// Example of enabling JMX for a datasource
BasicDataSource dataSource = new BasicDataSource();
dataSource.setJmxName("yourJmxName");
5. Failing to Tune Timeout Values
Timeout values dictate how long the application waits for a connection from the pool. Setting these values too high may lead to user dissatisfaction, while values that are too low could result in frequent connection shortages.
Why it Matters: Proper timeout settings are crucial for user experience and system stability.
Solution: Adjust the connection timeout and validation timeout based on your application's performance benchmarks.
<property name="maxWaitMillis" value="10000" /> <!-- 10 seconds -->
<property name="maxConnectionIdleTime" value="30000" /> <!-- 30 seconds -->
Best Practices for Connection Pool Management
Addressing the pitfalls alone is not enough; implementing best practices will help you further optimize connection management in Apache Camel.
1. Use Connection Pooling Libraries
Leverage robust connection pooling libraries like HikariCP or Apache DBCP. HikariCP is known for its performance and is often favored in high-throughput applications.
<dependency>
<groupId>com.zaxxer</groupId>
<artifactId>HikariCP</artifactId>
<version>5.0.1</version>
</dependency>
2. Leverage Camel's Built-in Features
Camel provides its own methods to manage connections effectively. Use Salamander or Camel's interceptors for added flexibility in managing connections.
3. Optimize Your Queries
Always make sure that your SQL queries are optimized. Unoptimized or complex queries may consume connections longer than necessary, leading to contention in the pool.
4. Periodically Review Configuration
Your application’s needs may change over time. Review and adjust your pool of configurations periodically to adapt to any changes in load.
5. Thread Management
If you’re managing concurrent routes, make sure the thread settings are correctly configured. Unoptimized threading can lead to increased connections, overwhelming the pool.
The Closing Argument
Incorporating proper connection pooling practices in Apache Camel is vital for developing high-performing applications. By avoiding common pitfalls—like misconfiguring the connection pool size or neglecting connection validation—and following best practices, you can ensure that your application remains responsive, reliable, and scalable.
For further reading on optimizing Apache Camel routes and configurations, consider visiting the Apache Camel Documentation and exploring community discussions on platforms like Stack Overflow.
By mastering these connection pooling principles, you’ll lay a solid foundation for your integration architecture and improve the overall health of your systems. Happy coding!