Common Pitfalls in Injecting Authenticated Users in Spring MVC
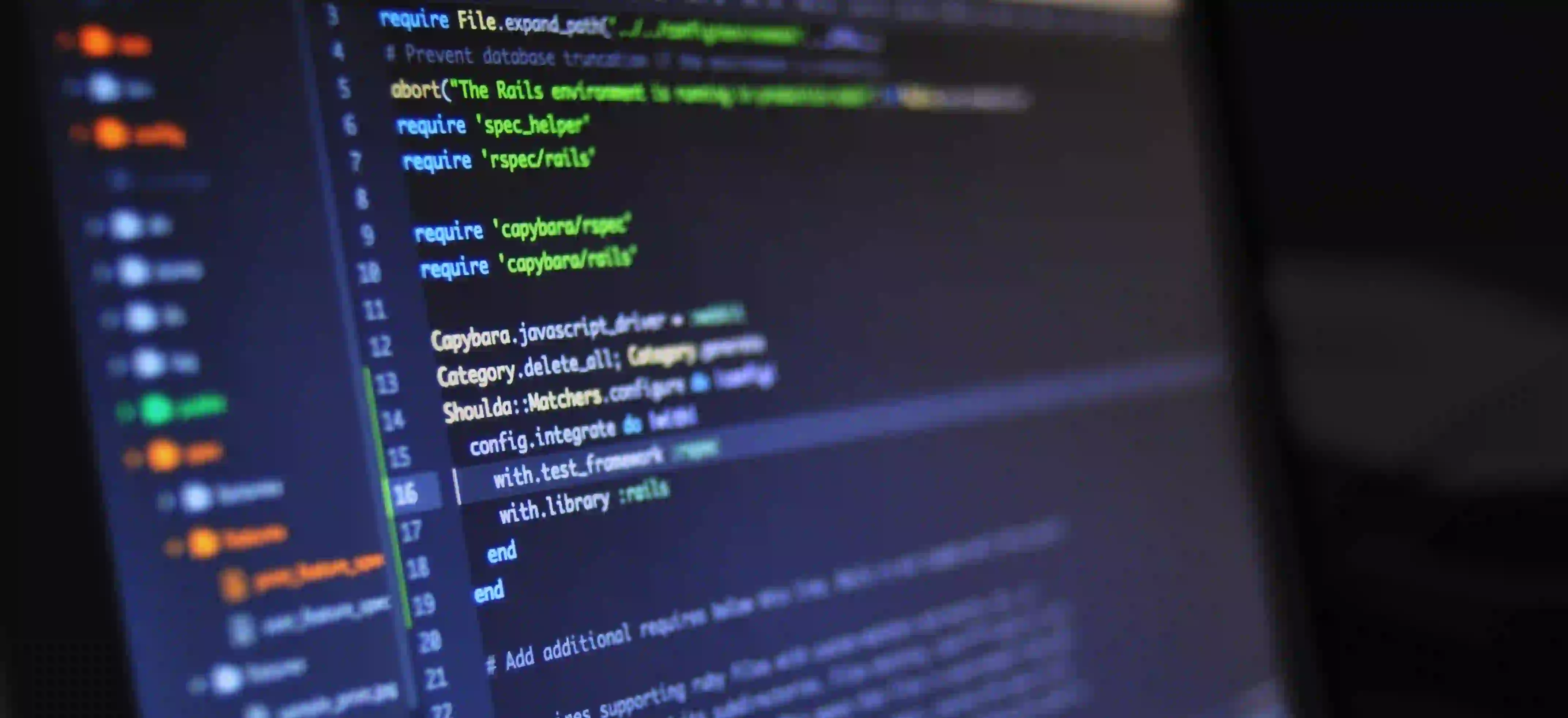
Common Pitfalls in Injecting Authenticated Users in Spring MVC
Spring MVC, one of the most popular frameworks for building web applications in Java, provides extensive support for user authentication and authorization. However, understanding how to correctly inject authenticated users into your controllers and services is crucial. Missteps in this area can lead to security vulnerabilities, application errors, and a poor user experience. In this post, we will explore the common pitfalls developers face when injecting authenticated users in Spring MVC and provide solutions to avoid these issues.
Understanding Authentication in Spring MVC
Before we dive into pitfalls, it’s essential to grasp what authentication entails in the context of Spring MVC. Authentication verifies the identity of a user, while authorization determines what authenticated users can access or do within the application.
Key Components:
- Security Context: Holds the authentication details.
- Authentication Object: Contains the user details, roles, and permissions.
- Principal: Typically the user itself, represented as a user object.
Common Pitfalls
1. Ignoring the Security Context
One of the most significant mistakes is assuming that a user is always authenticated without checking the SecurityContextHolder
.
Example:
import org.springframework.security.core.context.SecurityContextHolder;
import org.springframework.security.core.Authentication;
public User getCurrentUser() {
Authentication authentication = SecurityContextHolder.getContext().getAuthentication();
if (authentication != null && authentication.isAuthenticated()) {
return (User) authentication.getPrincipal();
}
throw new IllegalStateException("User not authenticated");
}
Why: Ignoring the Security Context may lead to null pointer exceptions if accessed improperly. Always verify that the user is authenticated before proceeding with user-specific operations.
2. Injecting User Directly into Controllers
Injecting the User
object directly into your controller methods can lead to confusion and potential errors.
Incorrect Method Signature:
@GetMapping("/profile")
public String userProfile(User user) {
// User is injected automatically but may not be the authenticated user
return "profile-view";
}
Correct Method Signature:
@GetMapping("/profile")
public String userProfile(Principal principal) {
if (principal instanceof UserDetails) {
UserDetails user = (UserDetails) principal;
// Access user details
}
return "profile-view";
}
Why: This approach ensures you're explicitly accessing the authenticated user through Principal
, reducing ambiguity in what user data is being injected.
3. Forgetting to Configure Security Properly
Misconfiguration in Spring Security settings can lead to users being unauthenticated or having insufficient permissions.
Configuration Example:
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/login").permitAll()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll();
}
}
Why: Neglecting to secure endpoints correctly can expose sensitive routes, leading to unauthorized access. Ensure that your security configurations align with your application’s user flow.
4. Not Handling Role-Based Access Control
A frequent oversight is not checking user roles when performing sensitive operations. This can allow unauthorized actions.
Example:
@GetMapping("/admin")
@PreAuthorize("hasRole('ADMIN')")
public String adminPage() {
return "admin-view";
}
Why: The @PreAuthorize
annotation provides a clean way to enforce role checks at the method level. Always protect sensitive endpoints or service calls with appropriate role checks.
5. Using Deprecated Authentication Methods
Outdated methods for handling authentication and sessions can lead to security issues. Always use the latest practices.
Example of Deprecated:
session.setAttribute("user", authenticatedUser);
Updated Approach:
Utilize the Spring Security abstraction for session management.
Authentication authentication = new UsernamePasswordAuthenticationToken(userDetails, null, userDetails.getAuthorities());
SecurityContextHolder.getContext().setAuthentication(authentication);
Why: Avoiding legacy methods keeps your application secure and compliant with the latest standards.
6. Not Testing Security Implementations
Failing to test your authentication and authorization mechanisms can lead to exploitable vulnerabilities.
Testing Best Practices:
- Unit Tests: Mock the Security Context to simulate authenticated and unauthenticated users.
- Integration Tests: Ensure all endpoints behave as expected under various security conditions.
Example of Unit Test using Mockito:
@RunWith(SpringRunner.class)
@WebMvcTest(YourController.class)
public class YourControllerTest {
@Autowired
private MockMvc mockMvc;
@MockBean
private YourService yourService;
@Test
public void testUserProfileAuthenticated() throws Exception {
SecurityContext securityContext = mock(SecurityContext.class);
Authentication authentication = mock(Authentication.class);
when(authentication.getPrincipal()).thenReturn(new User("test", "password", new ArrayList<>()));
when(securityContext.getAuthentication()).thenReturn(authentication);
SecurityContextHolder.setContext(securityContext);
mockMvc.perform(get("/profile"))
.andExpect(status().isOk());
}
}
Why: Testing ensures that your security implementation is enforced and behaves as expected.
My Closing Thoughts on the Matter
Navigating the complexities of user authentication in Spring MVC requires diligence and awareness of common pitfalls. By understanding how to properly inject authenticated users, configure security settings, and implement role-based checks, you can build more secure and robust applications.
For additional resources, consider exploring the Spring Security Documentation for comprehensive guides on configuring your security setup effectively. Stay updated with best practices to continuously protect your application against vulnerabilities.
By implementing the guidance provided in this post, you can avoid common mistakes and enhance your application’s security, providing a smooth and safe experience for your users.