Scaling Challenges in Your Java Spring Boot URL Shortener
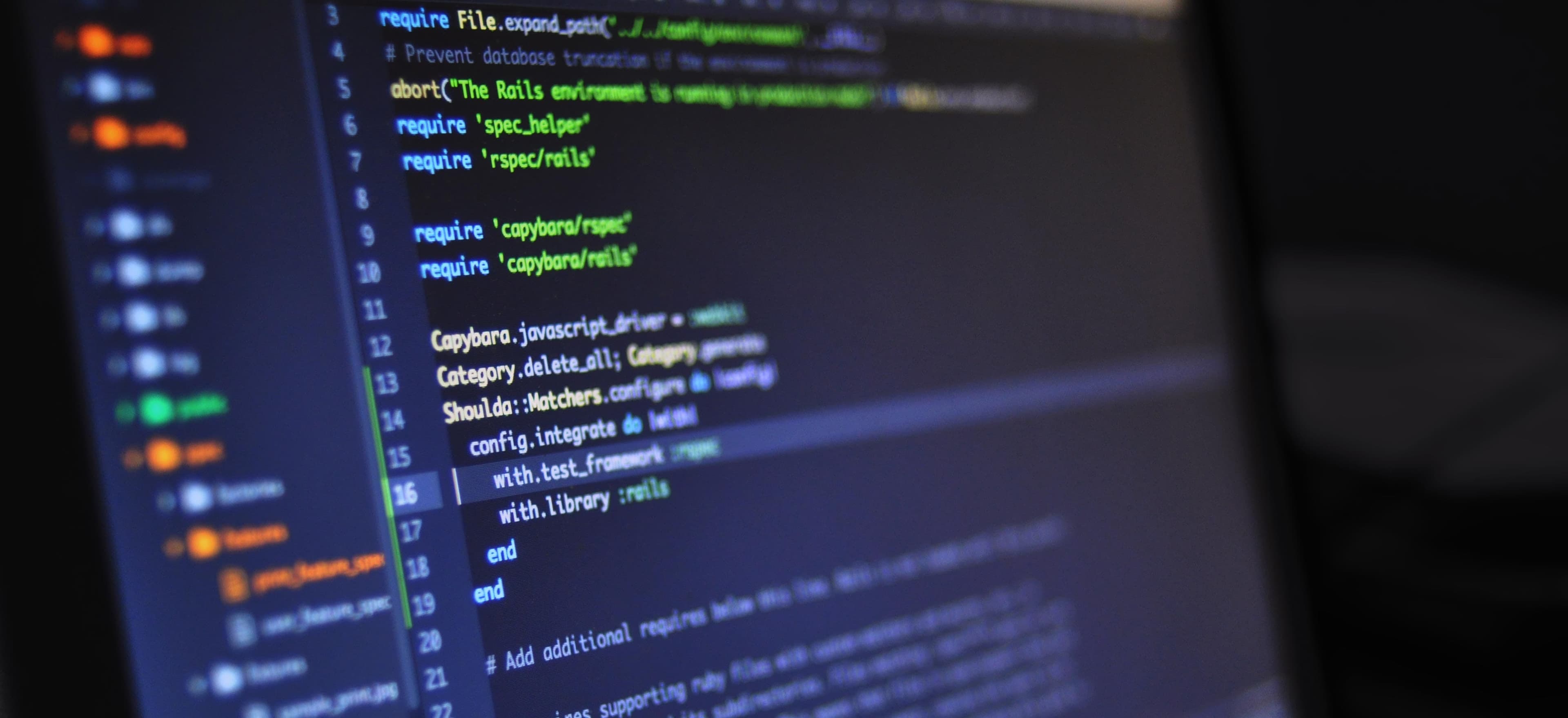
- Published on
Scaling Challenges in Your Java Spring Boot URL Shortener
In today's digital landscape, URL shorteners have become increasingly popular. They make sharing links on social media, emails, and forums much easier. As your application grows, however, you might run into scaling challenges. In this blog, we’ll explore how to tackle those scaling challenges when building a URL shortener using Java and Spring Boot.
Understanding URL Shorteners
A URL shortener takes long URLs and converts them into shorter, manageable versions. For example, the URL https://www.example.com/some/very/long/path
could be transformed into https://short.ly/abc123
. This process not only makes the links convenient but also allows tracking of user engagement.
Key Scaling Challenges
- Database Management
- Caching Solutions
- Load Balancing
- Concurrency Issues
- Monitoring & Logging
1. Database Management
As your URL shortener gains popularity, the number of stored URLs will explode. Consider using a NoSQL database like MongoDB or a highly performant SQL database like PostgreSQL to manage high read-write operations effectively.
Why Use NoSQL?
NoSQL databases, such as MongoDB, are designed to handle large volumes of data and offer scalability through data sharding. For example, here’s how you could define a simple Url
model in Java using Spring Data:
import org.springframework.data.annotation.Id;
import org.springframework.data.mongodb.core.mapping.Document;
@Document(collection = "urls")
public class Url {
@Id
private String id; // Auto-generated ID by MongoDB
private String longUrl;
private String shortUrl;
// Getters and Setters
}
Here, the @Document
annotation signifies that this class is mapped to a MongoDB document, which helps in easy scaling due to its schema-less nature.
2. Caching Solutions
Frequent database calls can slow down your service, particularly with millions of redirects. Implementing a caching layer using technologies like Redis can drastically improve performance.
Why Use Redis?
Redis allows you to store frequently accessed data in memory for quick retrieval. Here’s how you could implement caching:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.stereotype.Service;
@Service
public class UrlService {
@Autowired
private RedisTemplate<String, String> redisTemplate;
public String shortenUrl(String longUrl) {
String shortCode = generateShortCode(longUrl);
redisTemplate.opsForValue().set(shortCode, longUrl);
return shortCode;
}
public String getLongUrl(String shortCode) {
return redisTemplate.opsForValue().get(shortCode);
}
}
In this scenario, the RedisTemplate
allows us to store and retrieve URLs quickly. When a user requests a long URL for a short code, the service checks Redis first, bypassing the database if possible.
3. Load Balancing
As web traffic increases, a single instance of your application may not handle the load effectively. Utilizing a load balancer can distribute incoming traffic across multiple servers, enhancing the resilience and availability of your service.
How to Implement Load Balancing?
You can employ tools like Nginx or AWS Elastic Load Balancing. For Nginx, the configuration could look like this:
upstream url_shortener {
server app_server1.example.com;
server app_server2.example.com;
}
server {
listen 80;
location / {
proxy_pass http://url_shortener;
}
}
This configuration directs incoming requests to either app_server1
or app_server2
, ensuring that no single server is overwhelmed.
4. Concurrency Issues
With an influx of users, you might experience concurrency problems such as race conditions, especially when generating short URLs. Implementing synchronization mechanisms becomes essential.
Synchronization in Java
Here's an example to control access when generating a short code:
public synchronized String generateShortCode(String longUrl) {
// Logic to generate a unique short code
}
By synchronizing the method, we ensure that only one thread can access this logic at a time. While this approach is simple, consider alternatives like distributed locks or optimistic locking for better scalability.
5. Monitoring & Logging
Finally, monitoring is vital in ensuring your application performs optimally under various loads. Integrating logging and monitoring solutions like Spring Actuator, Grafana, or Prometheus can be key to proactive management.
Example of Actuator Implementation
To implement Spring Actuator, add the dependency in your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
In the application.properties
file, enable actuator endpoints:
management.endpoints.web.exposure.include=*
Access health and metrics via http://localhost:8080/actuator/health
and http://localhost:8080/actuator/metrics
.
Bringing It All Together
Building a scalable URL shortener using Java Spring Boot is rewarding, but comes with its unique challenges. By implementing strategic database management, caching solutions, load balancing, concurrency control, and robust monitoring, you can ensure your application remains responsive and reliable as it grows.
For further reading on improving performance in Java applications, consider exploring Java Performance Tuning.
Understanding these principles not only enhances your current project but also equips you with the knowledge to tackle similar issues in future applications. Don’t just build; build to scale. Happy coding!
Checkout our other articles