Unlocking Big Data: Java’s Role in Innovation
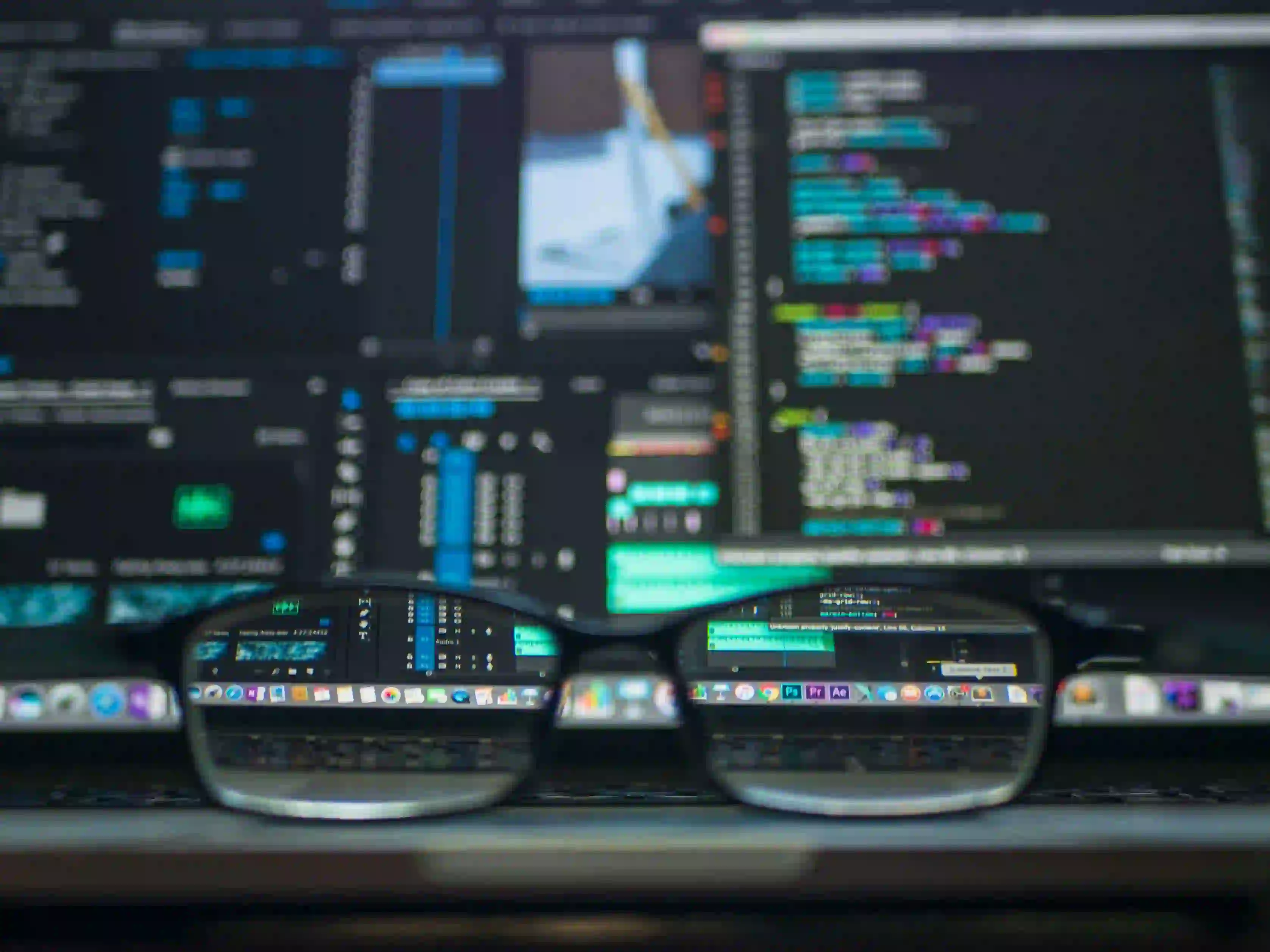
Unlocking Big Data: Java’s Role in Innovation
In today's tech-savvy world, the phrase "Big Data" is no longer confined to the realm of buzzwords; instead, it has become a critical aspect of how businesses operate and grow. Organizations are inundated with massive amounts of data, and leveraging that data effectively can mean the difference between success and failure. Java, a programming language known for its versatility and scalability, plays an essential role in unlocking the potential of Big Data.
Understanding Big Data
Big Data refers to extremely large datasets that cannot be processed efficiently with traditional data management tools. These datasets are often characterized by the "Three Vs": Volume, Velocity, and Variety.
- Volume: Represents the sheer amount of data generated daily.
- Velocity: Refers to the speed at which new data is generated and processed.
- Variety: Encompasses the different types of data, including structured, semi-structured, and unstructured data.
Why Java?
Java has been a mainstay in the software development community for decades. Its "write once, run anywhere" capability has made it a staple for enterprise-grade applications. Here are a few reasons why Java is integral to Big Data innovation:
- Platform Independence: Java code can run on any machine that has a Java Virtual Machine (JVM), enabling seamless deployment.
- Robust Libraries: With libraries like Apache Hadoop and Apache Spark, Java provides significant resources for managing and processing large datasets.
- Strong Community Support: Java boasts a large community of developers, which fosters continuous improvement, bug fixes, and extensive documentation.
- Scalability: The architecture of Java applications can scale with business needs, making it well-suited for Big Data solutions.
Java and Big Data Technologies
Apache Hadoop
Apache Hadoop is a framework that enables distributed storage and processing of large data sets using simple programming models. It utilizes a fault-tolerant, scalable architecture that can handle petabytes of data.
Here's a basic structure of a Hadoop MapReduce job written in Java:
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.Mapper;
import org.apache.hadoop.mapreduce.Reducer;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
import java.io.IOException;
public class WordCount {
public static class TokenizerMapper extends Mapper<Object, Text, Text, IntWritable> {
private final static IntWritable one = new IntWritable(1);
private Text word = new Text();
public void map(Object key, Text value, Context context) throws IOException, InterruptedException {
String[] words = value.toString().split("\\s+");
for (String w : words) {
word.set(w);
context.write(word, one);
}
}
}
public static class IntSumReducer extends Reducer<Text, IntWritable, Text, IntWritable> {
private IntWritable result = new IntWritable();
public void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException {
int sum = 0;
for (IntWritable val : values) {
sum += val.get();
}
result.set(sum);
context.write(key, result);
}
}
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "word count");
job.setJarByClass(WordCount.class);
job.setMapperClass(TokenizerMapper.class);
job.setCombinerClass(IntSumReducer.class);
job.setReducerClass(IntSumReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(IntWritable.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
Commentary on the Code:
- Mapper and Reducer: The
TokenizerMapper
class is responsible for breaking down the input text into words and emitting them as key-value pairs. TheIntSumReducer
processes these pairs, summing up the counts for each unique word. - Configuration: Using
Configuration
ensures the application settings can be easily modified for different environments. - File Handling: Input and output paths are dynamically set, which makes the application flexible.
This example illustrates a simple word count application, a classic introduction to MapReduce. You can find more detailed documentation on Apache Hadoop here.
Apache Spark
While Hadoop is powerful, it often struggles with batch processing speed. This is where Apache Spark, an open-source unified analytics engine, comes into play. Spark provides in-memory data processing capabilities, drastically improving performance over Hadoop’s disk-based approach.
Here's a simple Spark application in Java for counting words:
import org.apache.spark.api.java.JavaPairRDD;
import org.apache.spark.api.java.JavaRDD;
import org.apache.spark.api.java.JavaSparkContext;
import org.apache.spark.api.java.function.Function2;
import org.apache.spark.api.java.function.FlatMapFunction;
import org.apache.spark.api.java.function.PairFunction;
import org.apache.spark.SparkConf;
import java.util.Arrays;
public class SparkWordCount {
public static void main(String[] args) {
SparkConf conf = new SparkConf().setAppName("Word Count").setMaster("local");
JavaSparkContext sc = new JavaSparkContext(conf);
JavaRDD<String> lines = sc.textFile(args[0]);
JavaRDD<String> words = lines.flatMap((FlatMapFunction<String, String>) line -> Arrays.asList(line.split(" ")).iterator());
JavaPairRDD<String, Integer> wordCounts = words
.mapToPair((PairFunction<String, String, Integer>) word -> new Tuple2<>(word, 1))
.reduceByKey((Function2<Integer, Integer, Integer>) Integer::sum);
wordCounts.saveAsTextFile(args[1]);
}
}
Commentary on the Code:
- FlatMap: The
flatMap
function processes each line, splitting it into words, and returns them as an iterable collection. - mapToPair: This function transforms each word into a pair of (word, 1), initially counting each occurrence.
- reduceByKey: The
reduceByKey
function aggregates the counts for identical keys (words in this case), summing their occurrences efficiently.
This simple implementation of a word count application illustrates Spark's power and how it simplifies complex distributed data processing tasks. More information can be found in the Spark documentation here.
The Bottom Line
As businesses increasingly rely on data-driven decision-making, understanding how to harness the power of Big Data is essential. Java, with its array of frameworks and tools like Apache Hadoop and Apache Spark, provides the building blocks for developers to create robust solutions that align with modern data needs.
The significance of Java in the Big Data landscape is undeniable. By providing the tools necessary for efficient data processing and management, Java continues to empower organizations to turn enormous datasets into actionable insights. As Big Data technology evolves, so too will Java's role in delivering innovative solutions to tackle future challenges.
For further reading on Java and Big Data, visit Java Magazine and explore how to implement Java in your own data projects. Understanding these concepts will not only enhance your technical skills but also add significant value to your organization’s data strategies.
Call to Action
Are you ready to unlock the potential of Big Data using Java? Start experimenting with tools like Apache Hadoop and Apache Spark today! Share your experiences and insights in the comments below. Happy coding!