Struggling with Microservices? Tips for a Smooth Transition!
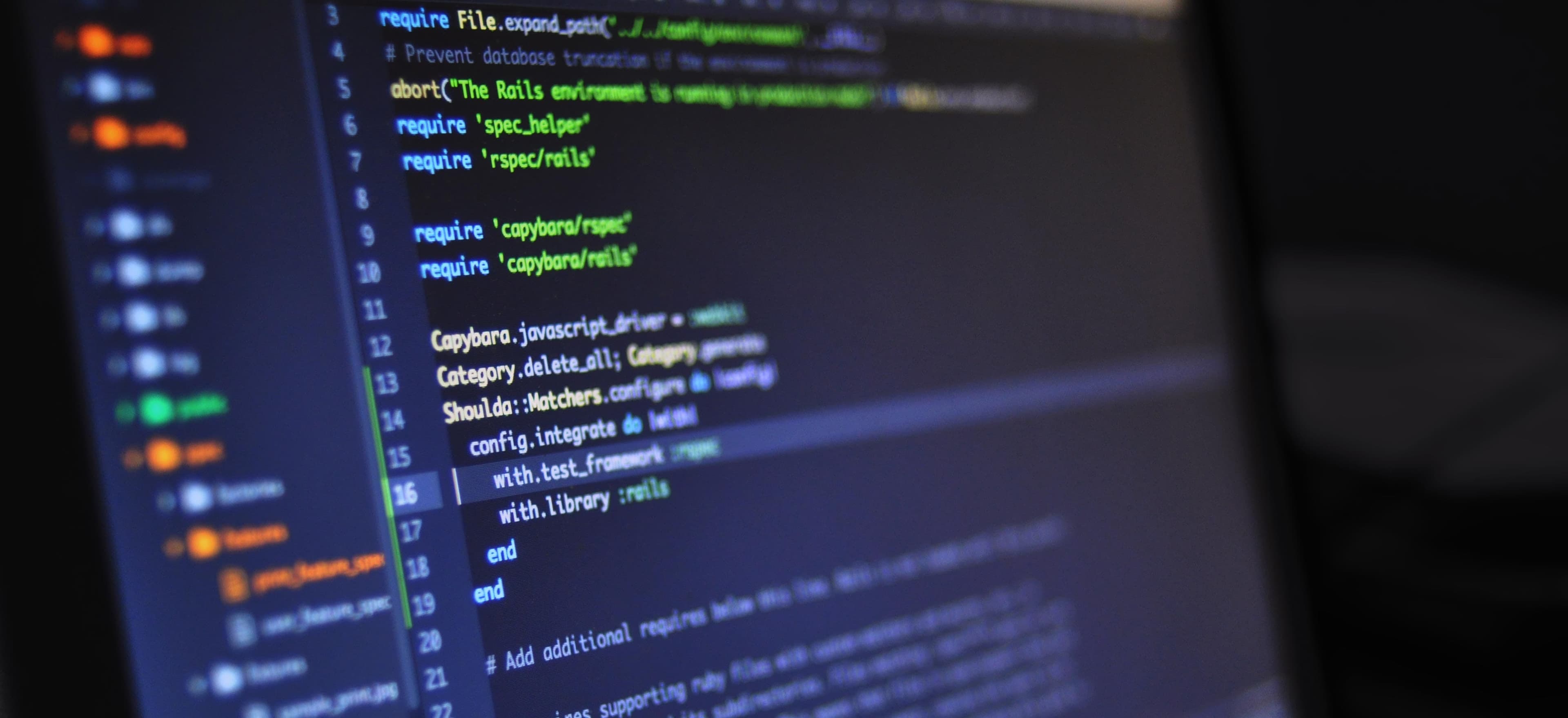
- Published on
Struggling with Microservices? Tips for a Smooth Transition!
The world of software development is experiencing a seismic shift toward microservices architectures. Developers, product owners, and companies alike are exploring the benefits that such systems can provide: flexibility, scalability, and enhanced maintainability. However, transitioning to a microservices architecture can be daunting. In this post, we will explore key strategies for a smooth transition to microservices, along with code examples that highlight important concepts.
What are Microservices?
Before we dive into the transition strategies, it’s essential to understand what microservices are. Microservices are an architectural style that structures an application as a collection of small, independently deployable services. Each service—often built around a specific business capability—communicates with others using APIs. This decentralizes development and allows for teams to work more independently.
Benefits of Microservices
- Scalability: Individual components can be scaled horizontally based on demand, without having to scale the entire application.
- Technology Diversity: Teams can choose different technologies that best suit their service requirements.
- Improved Maintainability: Smaller codebases are easier to manage, allowing for better version control.
Why Transition to Microservices?
While monolithic architectures have served us well, they often lead to tightly coupled systems that are hard to maintain, scale, and deploy. The transition to microservices not only facilitates agility within teams but also ensures that applications are more resilient to failures.
Step 1: Evaluate Your Current Architecture
Before making any changes, assess your existing monolithic architecture. Identify the components that would benefit from being extracted as microservices.
Example Evaluation Code
Here’s a simple example of how to analyze a monolithic application using metrics:
import java.util.HashMap;
import java.util.Map;
public class ServiceMetrics {
private Map<String, Integer> serviceCalls = new HashMap<>();
public void addServiceCall(String service) {
serviceCalls.put(service, serviceCalls.getOrDefault(service, 0) + 1);
}
public void printMetrics() {
serviceCalls.forEach((service, count) ->
System.out.println("Service: " + service + ", Calls: " + count));
}
}
Commentary
The above code provides a basic framework for tracking service usage within a monolithic architecture. By gathering data on individual service calls, you can identify bottlenecks and services that may need to be broken out into microservices.
Step 2: Modularize Your Monolith
Before jumping into full-blown microservices, consider modularizing your monolith. Extract modules that encapsulate specific business functions or capabilities.
Benefits of Modularization
- Risk Mitigation: By testing modules on their own, you can identify potential issues before they become larger problems.
- Gradual Transition: Incrementally breaking the application down makes the transition to microservices manageable.
Step 3: Design Your Microservices Around Business Capabilities
One of the most crucial aspects of building microservices is ensuring they are organized around business capabilities rather than technical functions. Each service should represent a single business domain or capability.
Code Example: Defining a Simple Service
public class OrderService {
public void createOrder(Order order) {
// Logic for creating an order
System.out.println("Order created: " + order);
}
}
Commentary
The OrderService
class here is a complete and cohesive service that manages the order creation process. It encapsulates all the logic required to handle orders, making it easy to maintain.
Step 4: Choose the Right Communication Protocols
Microservices typically require inter-service communication. Choose appropriate protocols like HTTP/REST, gRPC, or message queues (like RabbitMQ or Apache Kafka) based on your needs.
Example of a Simple RESTful Communication
@RestController
@RequestMapping("/orders")
public class OrderController {
private final OrderService orderService;
public OrderController(OrderService orderService) {
this.orderService = orderService;
}
@PostMapping
public ResponseEntity<String> createOrder(@RequestBody Order order) {
orderService.createOrder(order);
return ResponseEntity.status(HttpStatus.CREATED).body("Order created");
}
}
Commentary
This REST controller exposes an endpoint for creating orders. The use of Spring Boot annotations makes it clear and concise, allowing swift communication with front-end applications or other services.
Step 5: Implement Service Discovery
In a microservices architecture, services need to discover each other dynamically. Implement a service discovery solution to maintain a registry of services. Tools like Netflix Eureka, Consul, or Kubernetes can be great options.
Step 6: Monitor and Log
Monitoring becomes even more crucial in a distributed system. Implement logging and monitoring systems (such as ELK Stack, Prometheus, and Grafana) to keep track of service health.
Example: Logging Service Calls
public class LoggingService {
public void logServiceCall(String service) {
System.out.println("Service call to: " + service + " at " + System.currentTimeMillis());
}
}
Commentary
Implementing a logging service will help you track service interactions. This data can be invaluable for debugging and performance tuning.
Step 7: Database Choices
Decide whether each service should have its own database. This often leads to a more resilient architecture, as one service's database failure does not affect others. Be mindful of data consistency issues when using multiple databases.
Step 8: Embrace Continuous Delivery
Adopt Continuous Integration and Continuous Delivery (CI/CD) practices. This ensures each microservice can be deployed independently, thus maintaining the agility of development.
Bringing It All Together
Transitioning to microservices isn’t just a technical move; it's a cultural shift. Teams must embrace collaboration and ownership of services. By following these steps, your organization can smoothly transition to a microservices architecture. Each step requires careful planning and execution, but the benefits of scalability, resilience, and improved maintainability often far outweigh the challenges.
For more information, check out resources on Microservices Architecture and Spring Boot to deepen your understanding of these concepts.
The journey to microservices may be intricate, but with these tips and a clear focus on best practices, you can achieve a microservices architecture that enhances your software development process. Happy coding!
Checkout our other articles