Transforming JPA Annotations to jOOQ: Common Pitfalls
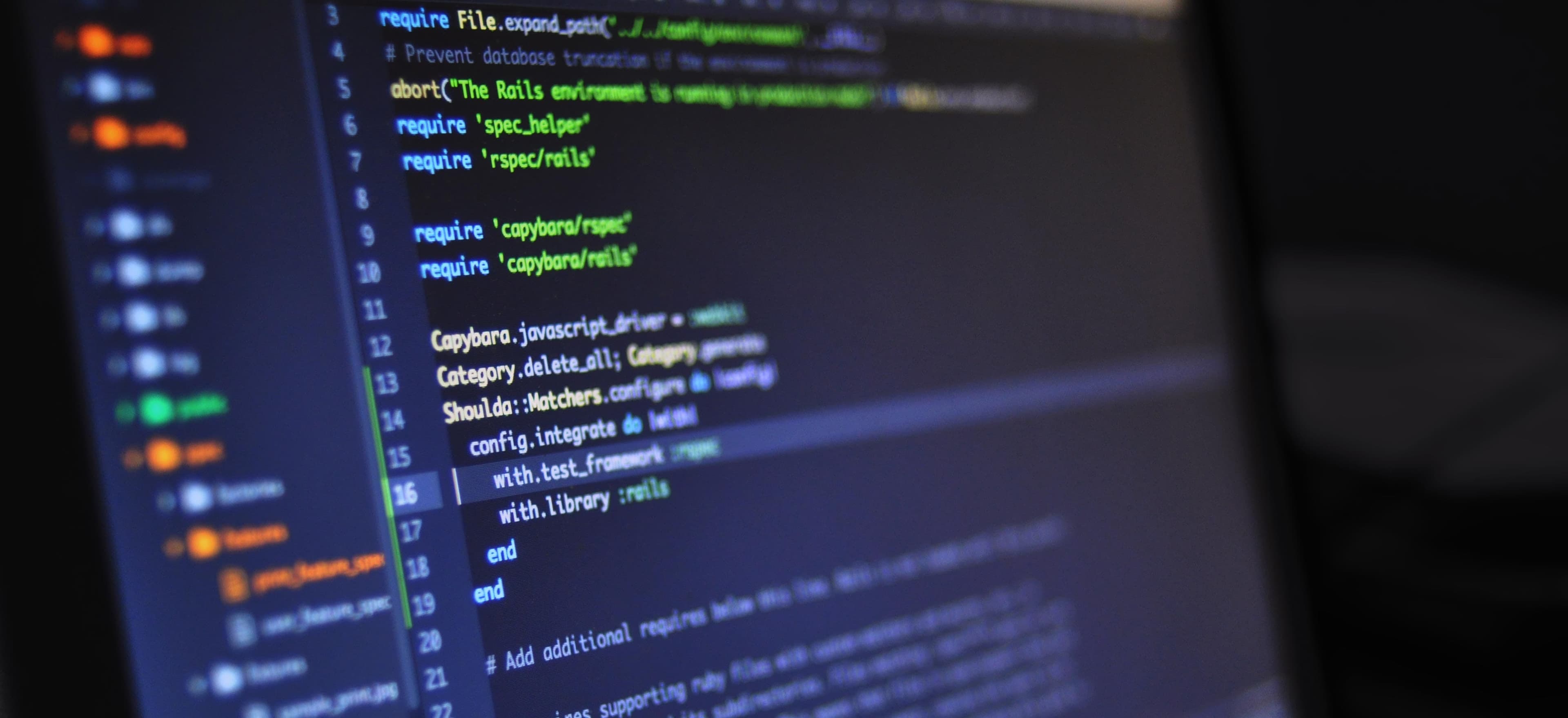
- Published on
Transforming JPA Annotations to jOOQ: Common Pitfalls
As developers, we often find ourselves transitioning between different frameworks and libraries. One common transition is from Java Persistence API (JPA) annotations to jOOQ, a powerful SQL query construction and execution library. While both approaches serve the purpose of interacting with databases, they exhibit distinct philosophies, capabilities, and quirks. Understanding these differences is crucial to avoid common pitfalls and ensure a smooth translation of your code.
In this blog post, we will explore some of these pitfalls and provide strategies for overcoming them. We will also include exemplary code snippets, offering commentary to shed light on why certain approaches work and how they fit into the broader context of data handling in Java. For more insights on jOOQ, you might find jOOQ's official documentation helpful.
Understanding the Differences
Before diving into the common pitfalls, it’s essential to have a firm grasp of the differences between JPA and jOOQ.
1. Abstraction Level
JPA provides a higher level of abstraction over relational database interactions compared to jOOQ. JPA allows developers to map Java objects to database tables. In contrast, jOOQ emphasizes direct SQL execution and gives developers more control over the SQL that is generated and executed.
2. Data Handling vs. Querying
JPA is primarily focused on object-relational mapping (ORM), where it interacts with the database records as objects. jOOQ, on the other hand, is tailored for building and executing SQL queries directly, putting a stronger emphasis on SQL as a first-class citizen.
Common Pitfalls
Pitfall 1: Ignoring SQL Syntax
One of the most frequent mistakes developers make while transitioning from JPA to jOOQ is neglecting the SQL syntax. Unlike JPA, jOOQ does not abstract SQL; instead, it requires a solid understanding of the SQL queries that will be generated.
Example
Here's a JPA entity:
@Entity
public class User {
@Id
@GeneratedValue
private Long id;
private String name;
@Column(name = "email_address")
private String email;
}
When translating it to jOOQ, you will be writing your SQL queries directly.
jOOQ Code
Result<Record3<Long, String, String>> result = DSL.using(configuration)
.select(USER.ID, USER.NAME, USER.EMAIL_ADDRESS)
.from(USER)
.fetch();
Why This Is Important: Neglecting the SQL syntax can lead to inefficient queries, errors, and unexpected behavior. Ensure you understand the SQL your jOOQ code generates and validate it against your expected results.
Pitfall 2: Misunderstanding the Type System
JPA is quite forgiving in terms of type handling due to its ORM principles; however, jOOQ mandates precise type matching. This means that you must ensure type safety when constructing your jOOQ queries.
Example
In JPA, the type conversion happens automatically. With jOOQ, if you are storing numeric values, you need to explicitly specify the type.
jOOQ Code
Long userId = result.getValue(USER.ID).longValue(); // Ensuring type safety
Why This Is Important: Type mismatches can lead to runtime exceptions. Always ensure the types you are leveraging in jOOQ match the database schema.
Pitfall 3: Using an Incorrect DSL Context
jOOQ relies on DSL contexts to execute queries. An incorrect context can either lead to incorrect behavior or performance drawbacks.
Example
DSLContext create = DSL.using(connection, SQLDialect.MYSQL);
Using the wrong SQL dialect can alter how your queries are interpreted and executed.
Why This Is Important: Each SQL dialect has its peculiarities; using the correct one ensures the generated SQL is optimized and executed properly. Make sure to choose the right SQL dialect that aligns with your database.
Pitfall 4: Failing to Manage Transactions
JPA provides built-in transaction management, which can make it easy for developers to manage their database interactions seamlessly. In contrast, with jOOQ, transaction management is often overlooked.
Example
In JPA, you might automatically handle transactions like this:
entityManager.getTransaction().begin();
// Perform operations here
entityManager.getTransaction().commit();
For jOOQ, you should manage transactions more explicitly.
jOOQ Code
try (DSLContext create = DSL.using(connection, SQLDialect.MYSQL)) {
create.transaction(configuration -> {
// Your SQL operations here
});
} catch (Exception e) {
// Handle exceptions
}
Why This Is Important: Not properly managing transactions can result in data inconsistency and integrity issues. Always ensure transactions are handled explicitly when transitioning to jOOQ.
Pitfall 5: Burden of SQL Maintenance
Developers often underestimate the maintenance burden that comes with writing SQL manually. With JPA, the ORM layer abstracts many details for you. When using jOOQ, you need to pay closer attention to SQL updates and changes.
Example
If the database schema changes, the SQL queries need to be adjusted accordingly. Unlike JPA’s annotations, which handle schema evolution for you, SQL in jOOQ must be maintained manually.
Why This Is Important: Regularly review and adapt your SQL queries as your database schema evolves. Proper documentation and version control of your SQL can mitigate maintenance issues in your projects.
The Last Word
Transitioning from JPA annotations to jOOQ can be a rewarding endeavor, leading to more control over your SQL interactions and potentially better performance. However, being aware of the common pitfalls—including SQL syntax, type system, DSL context, transaction management, and SQL maintenance—is crucial for a successful transition.
Incorporating jOOQ into your workflow can empower your projects with significant SQL manipulation capability, but it requires a different skill set compared to using JPA. Master these concepts and avoid these pitfalls, and you will set yourself up for success in your jOOQ endeavors.
For additional resources, you can check out jOOQ's online user manual and the official documentation for JPA.
Happy coding!
Checkout our other articles