Overcoming Common Pitfalls in HL7 Integration with Camel
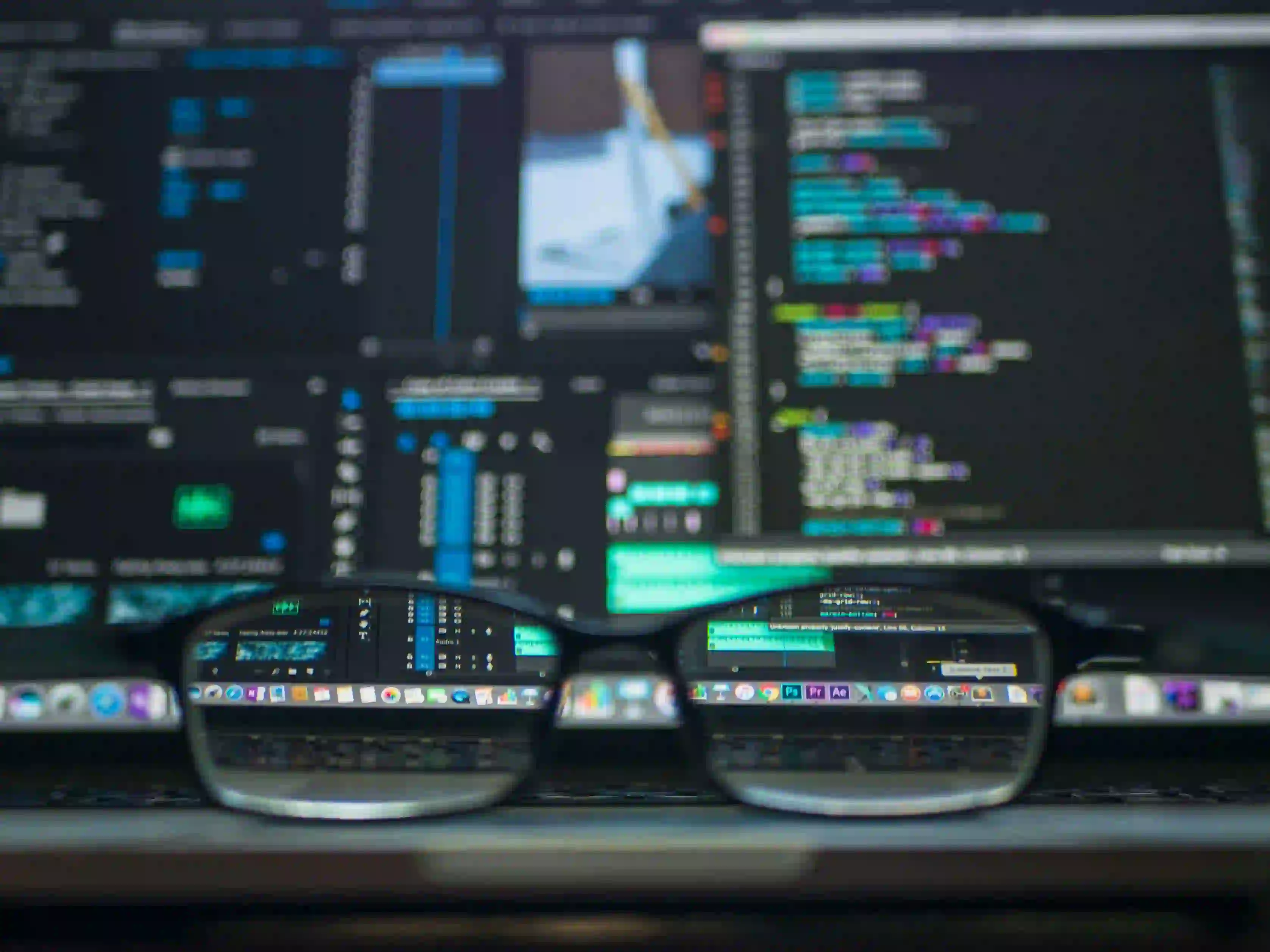
Overcoming Common Pitfalls in HL7 Integration with Camel
The healthcare sector is increasingly leveraging technology to improve patient care and data management. HL7 (Health Level Seven) is an established standard for electronic health information exchange and communication. Apache Camel, an open-source integration framework, provides developers with robust tools to facilitate HL7 integration. However, this integration is not without its challenges. In this blog post, we will discuss common pitfalls encountered during HL7 integration with Camel and how to overcome them effectively.
Understanding HL7 and Apache Camel
Before diving into the pitfalls and solutions, it's essential to understand both HL7 and Apache Camel. HL7 outlines protocols for sharing health information and is widely adopted globally. Apache Camel simplifies integration by offering a data mapping and routing strategy. Learn more about HL7 standards here and read about Apache Camel here.
Common Pitfalls in HL7 Integration with Camel
-
Inadequate HL7 Understanding
- Problem: Many developers lack a deep understanding of HL7 message structures. HL7 messages can be complex, often consisting of various segments that must adhere to specific formats.
- Solution: Familiarize yourself with HL7 basics. Utilize resources like HL7.org and practice with sample messages.
Here's a simple example of an HL7 message:
📄snippet.txtMSH|^~\&|SendingApp|SendingFacility|ReceivingApp|ReceivingFacility|202310021200||ORM^O01|MSG00001|P|2.3 PID|1||123456^^MRN||DOE^JOHN||19680525|M|||123 MAIN ST^^METROPOLIS^IL^12345||(555)555-5555
Each segment, delimited by pipes (
|
), contains specific data elements crucial for the interoperability of systems. -
Improper Use of Data Types
- Problem: The HL7 standard uses various data types (e.g., CN for composite name, DT for date). Using the wrong data type can lead to integration failures.
- Solution: Ensure the Camel route definitions specify the correct data types in message processing.
☕snippet.javafrom("direct:start") .unmarshal().hl7() .process(exchange -> { // Extract patient name using the correct data type (CN) String patientName = exchange.getIn().getBody(org.hl7.fhir.dstu3.model.Patient.class).getName().toString(); exchange.getIn().setBody(patientName); }) .to("log:receivedName");
This code snippet highlights how to unmarshal an HL7 message and extract pertinent patient information while respecting the data types.
-
Ignoring Version Compatibility
- Problem: HL7 has multiple versions, and mixing them can lead to compatibility issues and errors.
- Solution: Stick to one HL7 version throughout your integration project. Utilize Apache Camel's built-in support for HL7 versions.
To specify the HL7 version in your Camel route, configure the unmarshal action:
☕snippet.javafrom("direct:start") .unmarshal().hl7("2.5") // Specify the version here .process(exchange -> { // Process incoming HL7 message }) .to("log:hl7Messages");
-
Poor Error Handling
- Problem: Failing to implement robust error handling can lead to system crashes and data loss when unexpected messages are received.
- Solution: Implement exception handling in Camel routes to catch and log errors.
☕snippet.javafrom("direct:start") .onException(Exception.class) .handled(true) .log("Error processing HL7 message: ${exception.message}") .to("log:errorHandling") .end() .unmarshal().hl7() .process(exchange -> { // Process the HL7 message });
This code ensures that if any error occurs while processing an HL7 message, it logs the error and moves on, preventing a complete failure.
-
Inadequate Testing and Validation
- Problem: Rushing into production without adequate testing can result in faulty integrations and real-time problems.
- Solution: Create thorough test cases for your Camel routes, checking various scenarios, including edge cases and error conditions.
Use JUnit for testing:
☕snippet.java@Test public void testHl7Processing() { String hl7Message = "MSH|..."; // Sample HL7 message template.sendBody("direct:start", hl7Message); // Validate output and ensure expected results }
Setting up robust tests can help identify integration faults early in the process.
-
Performance Inefficiencies
- Problem: Performance can be a significant concern when processing large volumes of HL7 messages. Inefficient routes can lead to bottlenecks.
- Solution: Optimize your Camel routes by minimizing processing time wherever possible.
Consider using a thread pool for concurrent message processing:
☕snippet.javafrom("direct:start") .threads() // Enable multithreading for better performance .unmarshal().hl7() .process(exchange -> { // Process messages }) .to("log:processedMessages");
This enables Apache Camel to handle multiple messages simultaneously, enhancing throughput.
Best Practices for HL7 Integration with Camel
-
Use a Centralized Configuration: Maintain configuration files centrally. This practice simplifies updates and changes to integration settings.
-
Document Your Implementation: Clearly document the purpose and functionality of each Camel route to facilitate future maintenance.
-
Perform Continuous Monitoring: Implement real-time monitoring of your Camel context to catch issues before they escalate into severe problems.
-
Stay Updated: Keep abreast of the latest updates and changes in both HL7 standards and Apache Camel features.
Lessons Learned
Integrating HL7 standards with Apache Camel is not devoid of challenges, but with proper strategies and best practices, developers can substantially mitigate common pitfalls. By understanding HL7 message structures, ensuring version compatibility, handling errors gracefully, and optimizing performance, you can enhance the integration process.
For further reading, check HL7's official documentation and Apache Camel’s user guide. Embrace these practices, and you will improve both the reliability and efficiency of your HL7 integrations.
Your Next Steps
Now that you understand the common pitfalls in HL7 integration with Camel, take the following actions:
- Audit your current integration code against the pitfalls mentioned.
- Update your documentation to reflect the latest integration setup.
- Implement automated tests for your HL7 messaging paths.
By keeping these aspects in mind, you can ensure smoother HL7 integrations moving forward. Happy coding!