Streamline Your File Comparison Process in Java
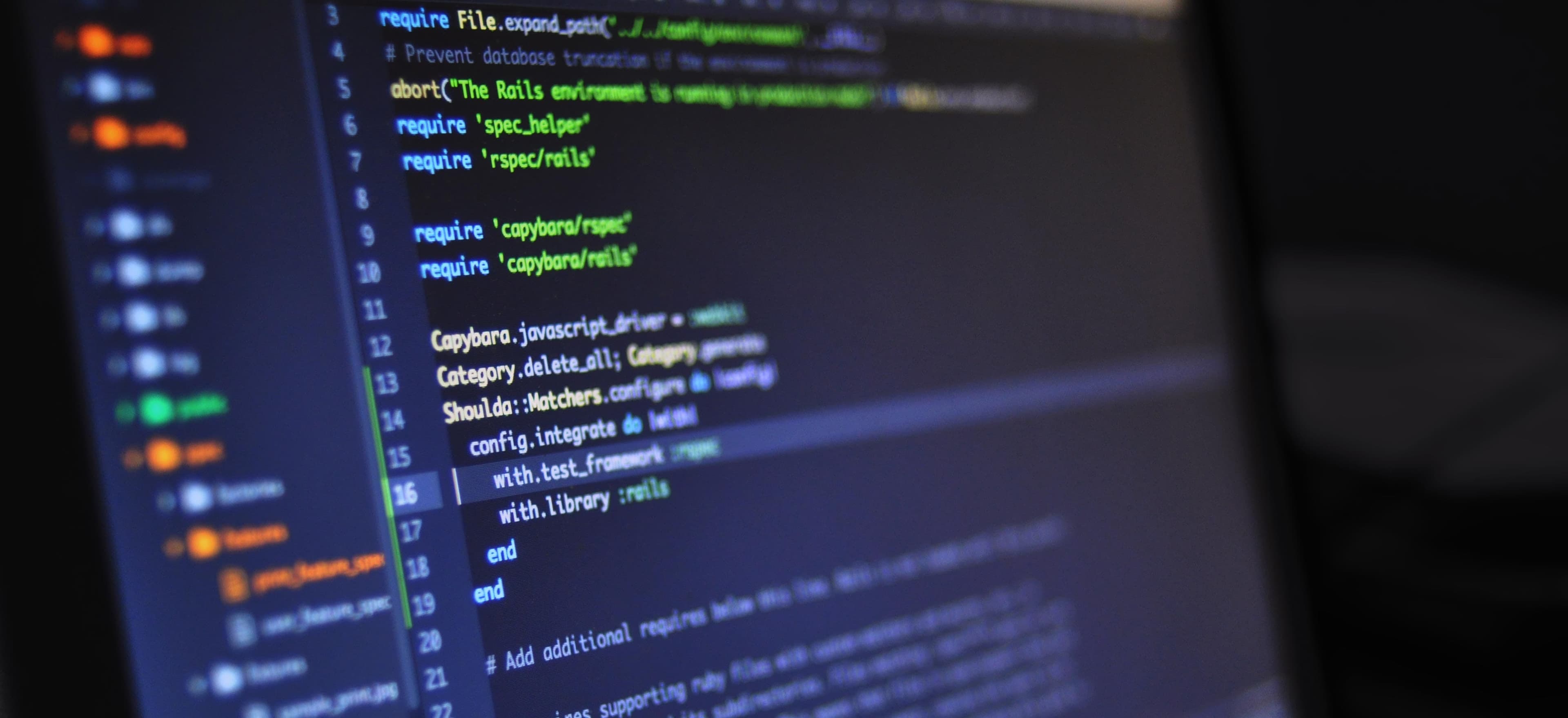
- Published on
Streamline Your File Comparison Process in Java
In today's digital world, managing and comparing files is vital for software development, data migration, and everyday tasks. Java offers powerful tools and libraries for file manipulation, making it an ideal choice for file comparison tasks. In this blog post, we will explore how to streamline the file comparison process in Java, helping you save time and improve efficiency.
Understanding File Comparisons
Before diving into the code, let's first understand what file comparison entails. File comparison typically involves:
- Content Comparison: Checking whether two files have the same content.
- Metadata Comparison: Evaluating attributes like size, timestamp, and permissions.
- Binary Comparison: Looking at files bit by bit, which is crucial for non-text files.
In this article, we will primarily focus on content comparison, as it is the most common requirement.
Java Libraries for File Comparison
Java provides several libraries to facilitate file comparison. Two common ways to achieve this are by reading files into memory and comparing the content line by line or utilizing Java's built-in Files
class for easier file handling.
Using Java NIO
Java NIO (New Input/Output) allows for efficient file handling. The following code snippet demonstrates how to compare two text files using Files
and streams:
import java.nio.file.Files;
import java.nio.file.Paths;
import java.io.IOException;
import java.util.List;
public class FileComparer {
public static void main(String[] args) {
String filePath1 = "path/to/file1.txt";
String filePath2 = "path/to/file2.txt";
try {
boolean areIdentical = compareFiles(filePath1, filePath2);
System.out.println("Files are identical: " + areIdentical);
} catch (IOException e) {
System.err.println("An error occurred while comparing files: " + e.getMessage());
}
}
public static boolean compareFiles(String path1, String path2) throws IOException {
List<String> file1Lines = Files.readAllLines(Paths.get(path1));
List<String> file2Lines = Files.readAllLines(Paths.get(path2));
return file1Lines.equals(file2Lines);
}
}
Explanation of the Code
- Imports: We import classes from
java.nio.file
, which provide access to file handling capabilities. - File Reading: The
Files.readAllLines
method reads all lines from a file into a List. This is efficient for small to moderately sized files. - Comparison: We utilize the
.equals()
method of List, which checks both the size and content of the lists.
This simple method offers a quick way to check if two files are the same. However, if you are dealing with very large files, this approach may consume significant memory. We'll explore a more scalable solution later.
Improved Approach for Large Files
When working with large files, loading them entirely into memory may not be practical. Instead, we can read and compare files line by line. Here’s how you can do that:
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class FileComparer {
public static void main(String[] args) {
String filePath1 = "path/to/largefile1.txt";
String filePath2 = "path/to/largefile2.txt";
try {
boolean areIdentical = compareFilesLineByLine(filePath1, filePath2);
System.out.println("Files are identical: " + areIdentical);
} catch (IOException e) {
System.err.println("An error occurred while comparing files: " + e.getMessage());
}
}
public static boolean compareFilesLineByLine(String path1, String path2) throws IOException {
try (BufferedReader br1 = new BufferedReader(new FileReader(path1));
BufferedReader br2 = new BufferedReader(new FileReader(path2))) {
String line1, line2;
while ((line1 = br1.readLine()) != null & (line2 = br2.readLine()) != null) {
if (!line1.equals(line2)) {
return false; // Lines are not the same
}
}
return br1.readLine() == null && br2.readLine() == null; // Check if both have reached EOF
}
}
}
Explanation of the Buffered Comparison
- BufferedReader: We use
BufferedReader
for reading files efficiently, especially useful for large text files. - Line By Line Comparison: We read two lines from each file and check if they are equal. This reduces memory usage significantly.
- End-of-File Check: The final check ensures that if one file is shorter than the other, the method will return false.
This approach combines efficiency with simplicity, making it suitable for various file sizes.
File Comparison for Binary Files
Often, the files you need to compare may not be in text format. They could be images, PDFs, or binary data where line-based comparisons are not applicable. In such cases, you can use the following method leveraging byte arrays:
import java.io.FileInputStream;
import java.io.IOException;
public class FileComparer {
public static void main(String[] args) {
String filePath1 = "path/to/file1.bin";
String filePath2 = "path/to/file2.bin";
try {
boolean areIdentical = compareBinaryFiles(filePath1, filePath2);
System.out.println("Files are identical: " + areIdentical);
} catch (IOException e) {
System.err.println("An error occurred while comparing files: " + e.getMessage());
}
}
public static boolean compareBinaryFiles(String path1, String path2) throws IOException {
try (FileInputStream fis1 = new FileInputStream(path1);
FileInputStream fis2 = new FileInputStream(path2)) {
int byte1, byte2;
while ((byte1 = fis1.read()) != -1 && (byte2 = fis2.read()) != -1) {
if (byte1 != byte2) {
return false; // Bytes are not the same
}
}
return fis1.read() == -1 && fis2.read() == -1; // Ensure both reach EOF
}
}
}
Explanation of the Binary Comparison
- FileInputStream: This class allows for reading raw bytes from a file, ideal for binary files.
- Byte-by-Byte Comparison: We read each byte and compare them individually.
- End-of-File Check: Similar to the previous examples, we ensure both files reach the end to confirm they are identical.
The Bottom Line
Efficiently comparing files in Java can be achieved through different approaches based on the file types and sizes. From simple line-by-line comparisons to handling binary files, the strategies discussed in this blog can streamline your file comparison process, enhancing productivity.
For a deeper understanding of file handling in Java, consider referring to the official Java documentation on NIO and File Handling.
Armed with these implementations and concepts, you can tackle your file comparison challenges with confidence. Happy coding!