Understanding the Key Differences in Spring Architecture
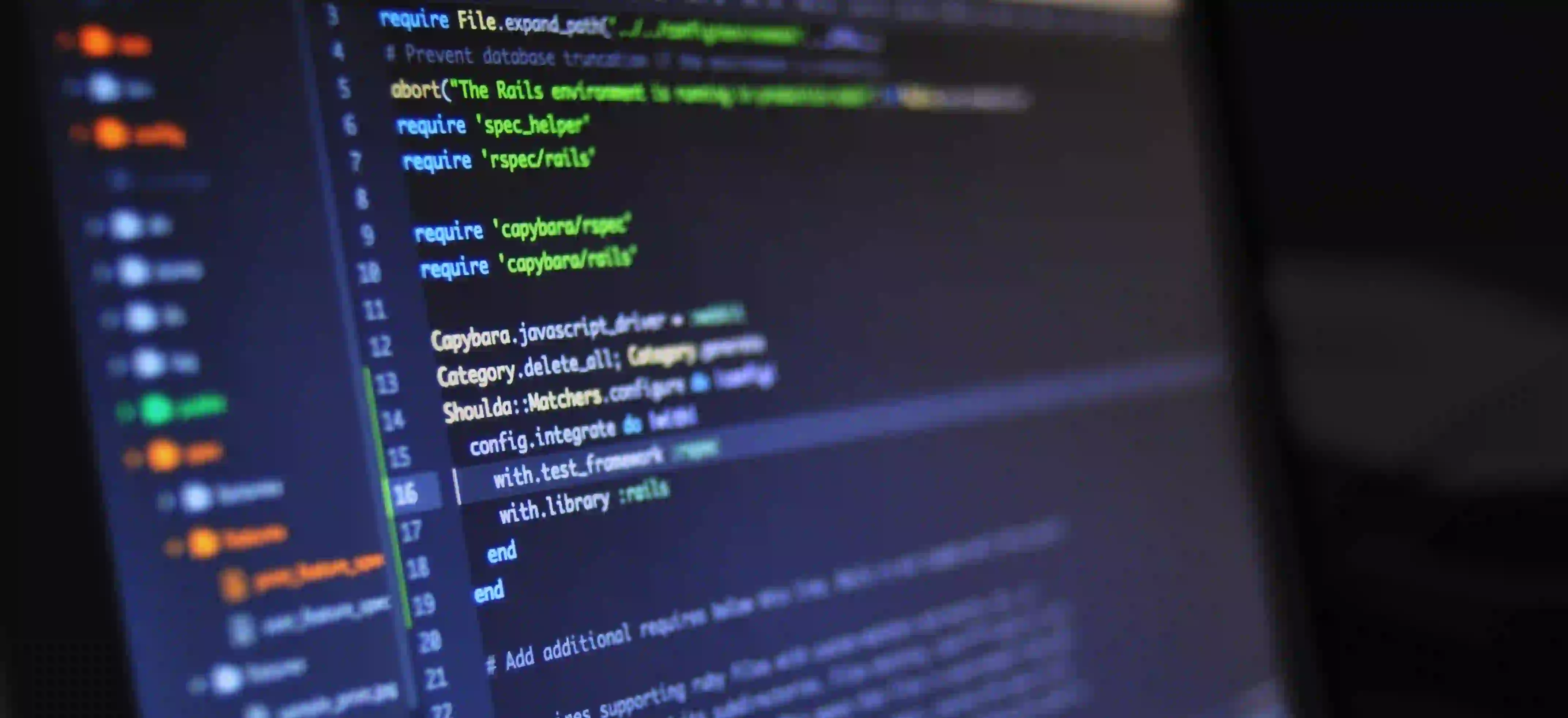
Understanding the Key Differences in Spring Architecture
The Spring Framework has become a cornerstone for modern Java application development. It offers a robust architecture and a plethora of features that streamline the development process. In this blog post, we will explore the key differences in Spring architecture, focusing on its core components and how they interconnect to provide a comprehensive and flexible environment for building enterprise-grade applications.
What is Spring Framework?
Spring is an open-source application framework for Java that provides comprehensive infrastructure support for developing Java applications. It is primarily designed to foster a simpler, more efficient programming model through dependency injection and aspect-oriented programming (AOP).
Key Features of the Spring Framework
- Dependency Injection (DI): Makes your code more modular and testable.
- Aspect-Oriented Programming (AOP): Promotes separation of concerns and code reusability.
- Modularity: Divides the application into independent modules, making it easier to manage and develop.
- Integration: Easily connects with various other frameworks and technologies.
- Flexibility: Offers multiple ways to configure applications.
Core Components of Spring Architecture
The Spring Framework is composed of several modules that each serve a specific purpose. Letβs dive into the major components:
- Spring Core Container
- Spring AOP
- Spring Data Access/Integration
- Spring Web
- Spring Security
- Spring Test
1. Spring Core Container
The Spring Core Container
module is foundational to the Spring Framework. It consists of the following sub-modules:
- Beans: This module is responsible for configuring and managing the beans. It uses Dependency Injection to provide the necessary dependencies and instantiate the classes.
- Core: This is the core of the Spring Framework, containing the fundamental components that define the bean creation and management process.
- Context: This module builds on the Beans module and adds support for internationalization and event propagation.
- Expression Language (SpEL): It provides powerful capabilities to query and manipulate objects at runtime.
Important Code Snippet: Dependency Injection using XML Configuration
<beans>
<bean id="car" class="com.example.Car">
<property name="engine" ref="engine"/>
</bean>
<bean id="engine" class="com.example.Engine"/>
</beans>
In this XML configuration snippet, we've defined two beans: car
and engine
. The car
bean is dependent on the engine
bean, as shown through the ref
attribute in the property
tag. This demonstrates Dependency Injection, where the Spring Framework injects the required dependencies into a class, promoting loose coupling.
2. Spring AOP
AOP enables separation of cross-cutting concerns like logging, security, and transaction management from the core business logic. This architecture improves code modularity and maintainability.
Important Code Snippet: AOP Example
@Aspect
public class LoggingAspect {
@Before("execution(* com.example.service.*.*(..))")
public void logBefore(JoinPoint joinPoint) {
System.out.println("Executing: " + joinPoint.getSignature().getName());
}
}
In this code example, we define a logging aspect that intercepts all method executions within the service
package. The @Before
annotation indicates that logging should occur before method execution. This mechanism enhances our application's monitoring capabilities without cluttering business logic.
3. Spring Data Access/Integration
This module provides seamless integration with various data access technologies such as JDBC and ORM frameworks like Hibernate and JPA. It simplifies database operations and transaction management.
Important Code Snippet: JDBC with Spring
@Autowired
private JdbcTemplate jdbcTemplate;
public List<User> getAllUsers() {
return jdbcTemplate.query("SELECT * FROM users",
(rs, rowNum) -> new User(rs.getInt("id"), rs.getString("name")));
}
In this snippet, we utilize JdbcTemplate
for database interaction. The method getAllUsers
uses a lambda expression to transform ResultSet
to a list of User
objects. This demonstrates Spring's ability to simplify database operations through a cleaner API.
4. Spring Web
The Spring Web module includes tools for building web applications. It supports various technologies such as Spring MVC, REST, and WebFlux for reactive applications.
Important Code Snippet: Basic Spring MVC Controller
@Controller
public class UserController {
@GetMapping("/users")
public String getUsers(Model model) {
List<User> users = userService.getAllUsers();
model.addAttribute("users", users);
return "userList";
}
}
In this code, we demonstrate a basic Spring MVC controller. The @GetMapping
annotation defines a route that retrieves a list of users. Utilizing the Model
object, we pass the users
list to the view. This setup exemplifies how Spring simplifies the MVC paradigm.
5. Spring Security
Spring Security adds a robust layer of security to Java applications. It provides authentication and authorization features, ensuring protection for web applications and REST APIs.
Important Code Snippet: Basic Security Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/", "/home").permitAll()
.anyRequest().authenticated()
.and().formLogin().loginPage("/login").permitAll()
.and().logout().permitAll();
}
}
In this security configuration, we restrict access to certain URLs. The permitAll()
method allows public access, while anyRequest().authenticated()
secures all other routes. This level of configuration ensures your application is protected against unauthorized access.
6. Spring Test
The Spring Test module facilitates the testing of Spring components with JUnit or TestNG. It provides support for integration tests, enabling developers to validate the entire application context.
Important Code Snippet: Simple Unit Test with Spring
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(classes = AppConfig.class)
public class UserServiceTest {
@Autowired
private UserService userService;
@Test
public void testGetAllUsers() {
List<User> users = userService.getAllUsers();
assertNotNull(users);
assertFalse(users.isEmpty());
}
}
In this unit test, we load the Spring context using @ContextConfiguration
. The @Autowired
annotation injects the userService
bean, allowing us to test its functionality effectively. This modular testing guarantees that we can verify behavior without unnecessary dependencies.
In Conclusion, Here is What Matters
The Spring Framework stands out as an essential tool in Java application development due to its modular architecture and powerful features. Understanding the key differences within its architecture is paramount for effectively leveraging its capabilities. From Dependency Injection to Spring Security, each component plays a crucial role in building scalable, maintainable applications.
For those seeking to delve deeper into Spring's richer landscape, consider exploring Spring Official Documentation or Baeldung's Spring Tutorials. These resources will undoubtedly enhance your understanding and skills in Spring development.
Whether you're building a small-scale application or a complex enterprise solution, mastering Spring architecture is your gateway to success in Java development. Embrace these components, and watch your applications flourish.