Mastering Java Reflection: Common Pitfalls to Avoid
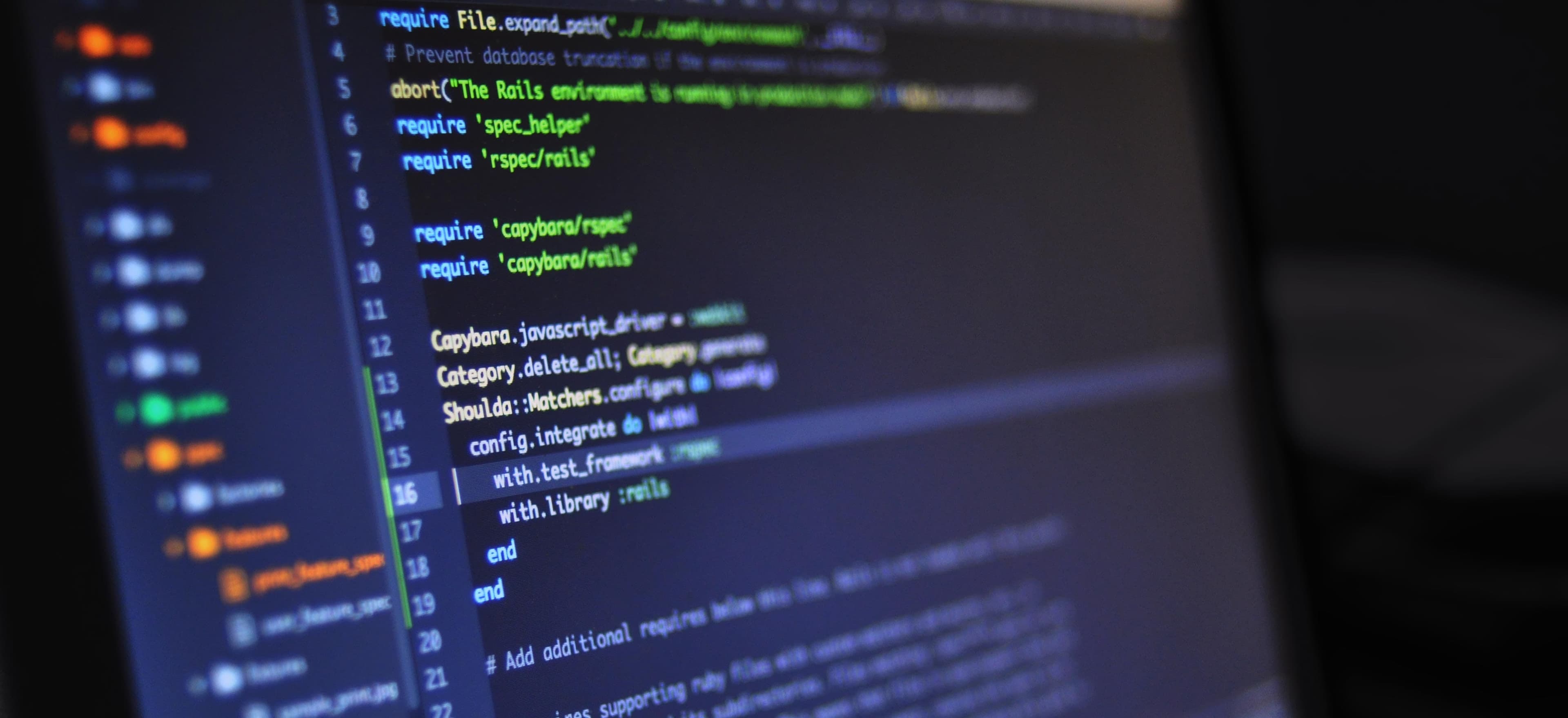
- Published on
Mastering Java Reflection: Common Pitfalls to Avoid
Java Reflection is a powerful feature that allows developers to inspect and manipulate classes, methods, and fields at runtime. Although it provides flexibility and dynamic capabilities, using reflection can lead to a myriad of pitfalls if not approached carefully. This blog post aims to illuminate the common pitfalls developers face when using Java Reflection and how to master it effectively.
What is Java Reflection?
Java Reflection is part of the java.lang.reflect
package, which allows you to:
- Inspect class details (methods, fields, constructors)
- Create instances of classes at runtime
- Invoke methods on any object
- Access private fields and methods
While reflection is undoubtedly useful, it’s crucial to approach it with caution. Let’s dive into common pitfalls and explore how to avoid them.
1. Performance Overhead
The Problem
One of the first things to consider when using reflection is performance. Reflection is generally slower than direct method calls. This occurs because the Java Virtual Machine (JVM) needs to do extra work to resolve classes and methods at runtime.
The Solution
When possible, use reflection judiciously. If you need to access a method or field frequently, cache the reference instead of using reflection each time. For high-performance applications, consider alternatives such as interfaces or direct method calls.
import java.lang.reflect.Method;
public class ReflectionPerformanceExample {
public static void main(String[] args) throws Exception {
MyClass myObject = new MyClass();
Method method = MyClass.class.getDeclaredMethod("myMethod");
// Instead of calling reflection repeatedly,
// call the method once and cache the reference if needed.
method.invoke(myObject);
}
}
class MyClass {
public void myMethod() {
System.out.println("Method called");
}
}
2. Security Restrictions
The Problem
Java's security model protects sensitive information by restricting reflective access to private members of classes. You might encounter a SecurityException
if the code does not have the necessary permissions.
The Solution
Be sure to handle exceptions properly and always check your security context. If reflection is necessary, evaluate if it can be done in a secure environment or with the appropriate permissions.
import java.lang.reflect.Field;
public class SecurityExample {
public static void main(String[] args) {
try {
MySecureClass secureObj = new MySecureClass();
Field privateField = MySecureClass.class.getDeclaredField("secret");
// Set accessible to true if you know it is safe to do so.
privateField.setAccessible(true);
System.out.println("Secret: " + privateField.get(secureObj));
} catch (Exception e) {
e.printStackTrace();
}
}
}
class MySecureClass {
private String secret = "This is a secret";
}
3. Lack of Compile-time Type Safety
The Problem
Reflection operates at runtime, and this can result in ClassCastException
or NoSuchMethodException
errors that are not evident at compile time. This makes debugging and maintaining code more complex.
The Solution
Use reflection with care, and consider using generic types whenever possible. Implement robust error handling and consider using unit tests to catch potential issues early.
import java.lang.reflect.Method;
public class TypeSafetyExample {
public static void main(String[] args) {
try {
Object obj = getInstance("com.example.MyClass");
Method method = obj.getClass().getMethod("myMethod");
// Checked casting ensures that you are aware of the expected type.
MyClass myClassInstance = (MyClass) obj;
method.invoke(myClassInstance);
} catch (Exception e) {
e.printStackTrace();
}
}
private static Object getInstance(String className) throws Exception {
return Class.forName(className).getDeclaredConstructor().newInstance();
}
}
class MyClass {
public void myMethod() {
System.out.println("Method invoked");
}
}
4. Misuse of setAccessible(true)
The Problem
Using setAccessible(true)
can expose private fields and methods, leading to unwanted side effects and reduced encapsulation. Misuse can cause maintenance issues and can make your code harder to read and understand.
The Solution
Limit the use of setAccessible(true)
to cases where it’s absolutely necessary. Always consider the implications of violating encapsulation and how it affects your code’s integrity.
import java.lang.reflect.Method;
public class AccessibleExample {
public static void main(String[] args) {
try {
Class<?> clazz = MyClass.class;
Method method = clazz.getDeclaredMethod("privateMethod");
method.setAccessible(true); // Use with caution!
MyClass myClassInstance = new MyClass();
method.invoke(myClassInstance);
} catch (Exception e) {
e.printStackTrace();
}
}
}
class MyClass {
private void privateMethod() {
System.out.println("Private method called");
}
}
5. Improper Use of Reflection for Frameworks
The Problem
Frameworks and libraries often utilize reflection internally, but using reflection outside of these contexts can lead to potential compatibility issues. For instance, if a library is updated, your reflection-dependent code might break due to method renaming or changes in access modifiers.
The Solution
Whenever possible, rely on public APIs provided by the frameworks rather than digging deeper with reflection. If you absolutely have to use reflection, read the library's documentation to better understand potential pitfalls.
Closing Remarks
Java Reflection is an essential tool in a developer's toolkit, offering significant power and flexibility. However, it comes with a responsibility to manage the complexity and potential pitfalls associated with its use. By understanding and avoiding common mistakes, optimizing for performance, respecting security implications, and maintaining strong typing, you can harness reflection's capabilities effectively.
As you dive deeper into Java Reflection, remember these guiding principles. Always review your code for security concerns, performance overhead, and maintainability. With careful consideration, reflection can enhance your applications rather than complicate them.
For more information on Java Reflection and best practices, visit Oracle's Java Documentation or join the vibrant Java community on forums like Stack Overflow.
Master Java Reflection, avoid the pitfalls, and elevate your coding experience!