Streamlining Stack Exchange Questions with Spring Social
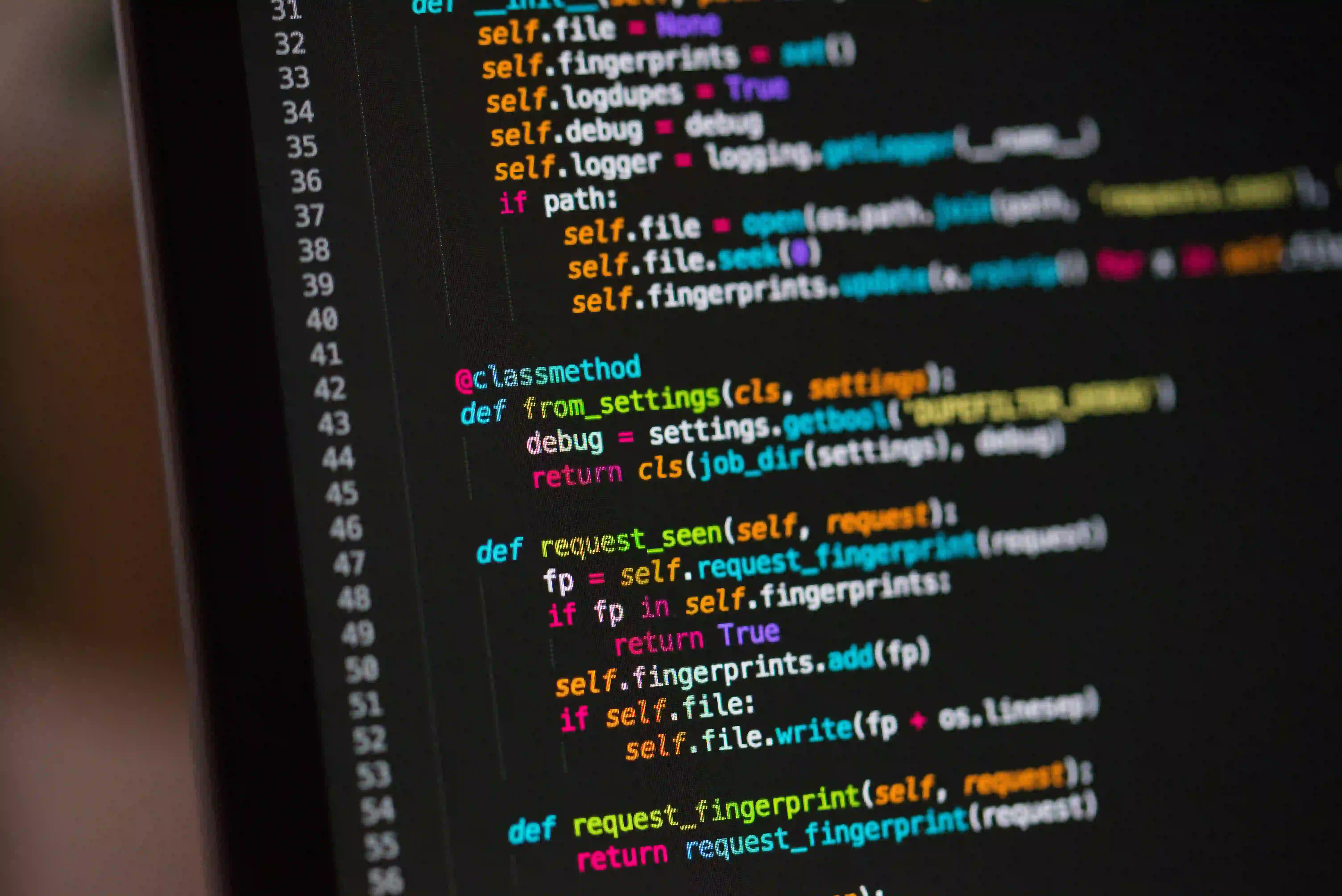
Streamlining Stack Exchange Questions with Spring Social
In today's digital age, engaging with a community of developers and tech enthusiasts has become essential. For many, Stack Exchange serves as a vital resource for problem-solving and knowledge sharing. However, posting a question that resonates with the community requires thoughtful consideration. This is where Spring Social comes into play. In this blog post, we will explore how to streamline the process of asking questions on Stack Exchange using Spring Social, the popular Java framework for integrating with social networks.
Table of Contents
- What is Spring Social?
- Introduction to Stack Exchange API
- Setting Up Your Spring Social Application
- Creating a Seamless User Experience
- Code Example: Posting a Question
- Conclusion
- Further Reading
What is Spring Social?
Spring Social is a powerful framework that simplifies the creation of social applications by providing a direct way to interact with social media APIs. It allows developers to build applications that can connect to various social networks, enabling features such as sharing content, liking posts, and even auto-posting questions and answers.
The framework largely exists to bridge the gap between your application and social media platforms. By leveraging Spring Social, you can streamline the connectivity features that enhance user interactions and community engagement.
Why Spring Social?
- Ease of Integration: It simplifies API connectivity and OAuth authentication.
- Enhanced User Experience: Offers a smooth interaction by connecting with third-party services.
- Robust Documentation: Comprehensive resources are available for developers to get started.
Setting the Scene to Stack Exchange API
The Stack Exchange API provides developers with the ability to interact programmatically with Stack Exchange's many communities. The API supports operations such as retrieving questions, answers, users, and many other aspects of the platform. Here are some key features of the Stack Exchange API:
- Search for Questions: Allows searching across various tags, users, and more.
- Timing Control: Rate limits are in place to control the flow of requests.
- Authentication: Some operations require authentication for user-specific data access.
The access to diverse data points enables developers to create streamlined systems for users wanting to ask questions or retrieve existing Solutions.
Setting Up Your Spring Social Application
Before diving into code examples, we need to set up a Spring Social application.
Step 1: Initialize Your Project
You can use Spring Initializr to bootstrap your Spring application. Here’s how you can set it up:
- Choose Java as your language.
- Select a suitable Spring version (preferably 2.x).
- Add dependencies:
- Spring Web
- Spring Security
- Spring Social Core
- Spring Social Stack Exchange
Step 2: Configure Your Application
After initializing your new project, you need to configure it to integrate with the Stack Exchange API:
# application.yml
spring:
social:
stackexchange:
app-id: your_app_id
app-secret: your_app_secret
Setting app-id
and app-secret
is crucial for OAuth-based authentication with Stack Exchange. You can register your application on the Stack Apps site to obtain these credentials.
Creating a Seamless User Experience
To enhance user experience, provide straightforward onboarding for users, enabling them to authenticate and seamlessly ask questions. By utilizing Spring Social, you can simplify the OAuth flow.
- User Authentication: Allow users to log in with their Stack Exchange credentials.
- Profile Access: Fetch user profiles to personalize questions.
Benefits
- Reduced Friction: Users can leverage existing accounts.
- Increase Participation: Easier access can lead to more questions being posted.
Code Example: Posting a Question
Let’s look at how to post a question to Stack Exchange using Spring Social.
Step 1: Create a Service for Posting Questions
Implement a service to interact with the Stack Exchange API.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.social.stackexchange.api.StackExchange;
import org.springframework.social.stackexchange.api.Question;
import org.springframework.stereotype.Service;
@Service
public class StackExchangeService {
@Autowired
private StackExchange stackExchange;
public Question postQuestion(String title, String body) {
Question question = new Question();
question.setTitle(title);
question.setBody(body);
// Making the API call to post the question
return stackExchange.getQuestionOperations().postQuestion(question);
}
}
Commentary
- Service Annotation: By marking the class as a @Service, Spring manages its lifecycle and allows for easy dependency injection.
- @Autowired: Automatically inject the StackExchange API, making it ready to use.
- Question Object: Encapsulation of question data that will be sent to the API.
Step 2: Configure a Controller
Next, create a REST controller to manage user requests:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/api/questions")
public class QuestionController {
@Autowired
private StackExchangeService stackExchangeService;
@PostMapping
public ResponseEntity<Question> createQuestion(@RequestParam String title,
@RequestParam String body) {
Question createdQuestion = stackExchangeService.postQuestion(title, body);
return ResponseEntity.ok(createdQuestion);
}
}
Commentary
- @RestController: Indicates that the class will handle HTTP requests and responses.
- Endpoint: The
@PostMapping
annotation creates an endpoint to post a new question, making it easily accessible to client applications.
By following these steps, you enable your users to post questions directly to Stack Exchange with just a few clicks, increasing their engagement with the platform.
Wrapping Up
Integrating Spring Social with the Stack Exchange API offers an efficient solution for developers looking to streamline the question-asking process. By leveraging Spring's capabilities, we can create an engaging and user-friendly way to encourage participation in community discussions.
This framework not only allows for smooth interactions but also enhances user experiences through familiar social login mechanisms.
Further Reading
- Spring Social Documentation
- Stack Exchange API Documentation
- Spring Framework Guide
Implement these practices to enhance your interaction with Stack Exchange and other platforms. Happy coding!