Struggling to Find Peak Elements in Java? Here's How!
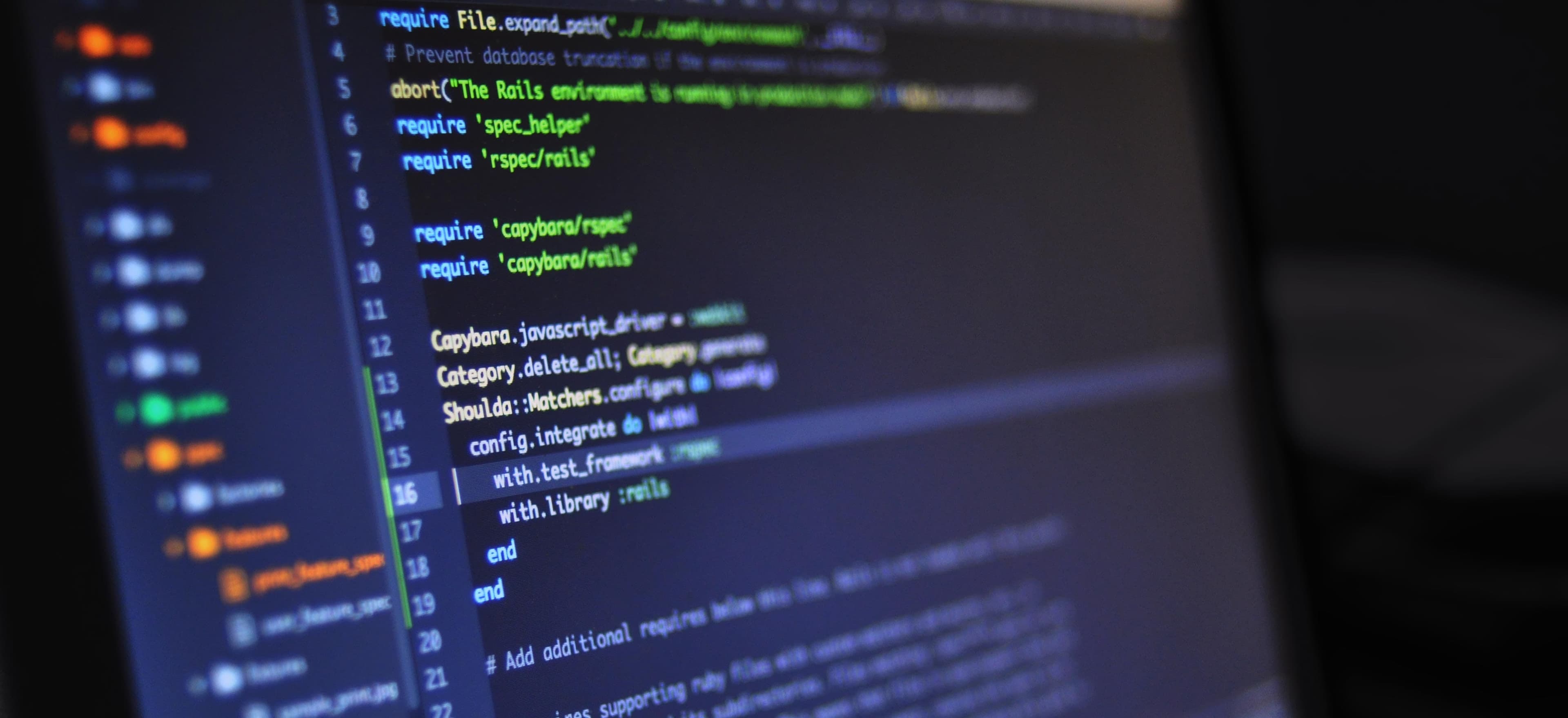
- Published on
Struggling to Find Peak Elements in Java? Here's How!
When traversing through arrays, you might encounter various challenges, one of which is identifying peak elements. A peak element is defined as an element that is greater than or equal to its neighbors. In a one-dimensional array, the elements at the ends only need to be greater than their one neighbor.
In this blog post, we will explore:
- What a peak element is.
- A concise algorithm to find peak elements in Java.
- Sample implementations with explanations.
- Tips for optimizing your search.
Let’s dive deep into this!
Understanding Peak Elements
To clarify, for an element arr[i]
to be a peak, the following conditions must hold:
- If
i
is0
(the first element), it should be greater than or equal toarr[1]
. - If
i
isn-1
(the last element), it should be greater than or equal toarr[n-2]
. - For elements in between (i.e.,
1 ≤ i ≤ n-2
), the conditions arearr[i] >= arr[i-1]
andarr[i] >= arr[i+1]
.
This definition implies that there can be multiple peak elements, or even if there's only one peak, it can be found using various methods.
The Algorithm: Linear Search
A straightforward way to find a peak element is through linear search. This involves iterating through the array and checking the necessary conditions for each element.
Implementation: Linear Search Method
Let's implement this method in Java.
public class PeakElementFinder {
public static int findPeak(int[] arr) {
int n = arr.length;
// Handle edge case for the first element
if (n == 1 || arr[0] >= arr[1]) {
return 0; // The first element is a peak
}
// Handle edge case for the last element
if (arr[n - 1] >= arr[n - 2]) {
return n - 1; // The last element is a peak
}
// Iterate through the array
for (int i = 1; i < n - 1; i++) {
if (arr[i] >= arr[i - 1] && arr[i] >= arr[i + 1]) {
return i; // Peak element found
}
}
return -1; // No peak element found (shouldn't happen in valid array)
}
public static void main(String[] args) {
int[] arr = {1, 3, 20, 4, 1, 0};
int peakIndex = findPeak(arr);
if (peakIndex != -1) {
System.out.println("Peak Element: " + arr[peakIndex] + " at index " + peakIndex);
} else {
System.out.println("No peak element found.");
}
}
}
Explanation
- Edge Cases: The algorithm first checks if the first element or the last element is the peak. This prevents out-of-bounds errors.
- Looping Through Elements: It then loops through all elements from the second to the second-to-last. For each element, it checks if it is greater than or equal to its neighbors.
- Return the Index: When a peak is found, the index is returned immediately.
Complexity Analysis
- Time Complexity: O(n) - requires a single pass through the array.
- Space Complexity: O(1) - no additional space usage apart from variables.
The More Efficient Approach: Binary Search
While the linear search method is intuitive, it's not the most efficient for large datasets. Instead, we can use a binary search technique, which reduces the time complexity to O(log n).
Implementation: Binary Search Method
Here’s how the binary search algorithm for peak finding looks in Java:
public class PeakElementFinder {
public static int findPeakUsingBinarySearch(int[] arr) {
return findPeakUtil(arr, 0, arr.length - 1);
}
private static int findPeakUtil(int[] arr, int left, int right) {
int mid = left + (right - left) / 2;
// Check peak condition for mid
if ((mid == 0 || arr[mid] >= arr[mid - 1]) &&
(mid == arr.length - 1 || arr[mid] >= arr[mid + 1])) {
return mid; // Mid is a peak
}
// Move towards the larger neighbor
if (mid > 0 && arr[mid - 1] > arr[mid]) {
return findPeakUtil(arr, left, mid - 1); // Search left
} else {
return findPeakUtil(arr, mid + 1, right); // Search right
}
}
public static void main(String[] args) {
int[] arr = {1, 3, 20, 4, 1, 0};
int peakIndex = findPeakUsingBinarySearch(arr);
System.out.println("Peak Element: " + arr[peakIndex] + " at index " + peakIndex);
}
}
Explanation
- Binary Search Splitting: This method recursively searches through half of the array based on the value of the middle element.
- Base Case: It checks if the middle element is a peak.
- Decision Making: If the left neighbor is greater than the middle element, it recursively searches the left half; otherwise, it searches the right half.
Complexity Analysis
- Time Complexity: O(log n) - because it halves the number of elements to search.
- Space Complexity: O(log n) - due to the recursive stack (not iterative).
A Final Look and Further Considerations
Finding peak elements in an array is a quintessential programming challenge that enhances your understanding of algorithms. We covered two methods: linear search and binary search.
- Use linear search for its simplicity and ease of implementation on small arrays.
- Opt for binary search when working with larger datasets for efficiency.
Explore further details about searching algorithms on GeeksforGeeks.
Final Thoughts
Through both methods, you have learned how to effectively find peak elements in a Java array. Consider which algorithm fits your needs based on the size of your data and specific requirements. Try to implement these functions, tweak them, and see how they perform in different scenarios.
Happy coding!