Overcoming Common Pitfalls in Java Command-Line Execution
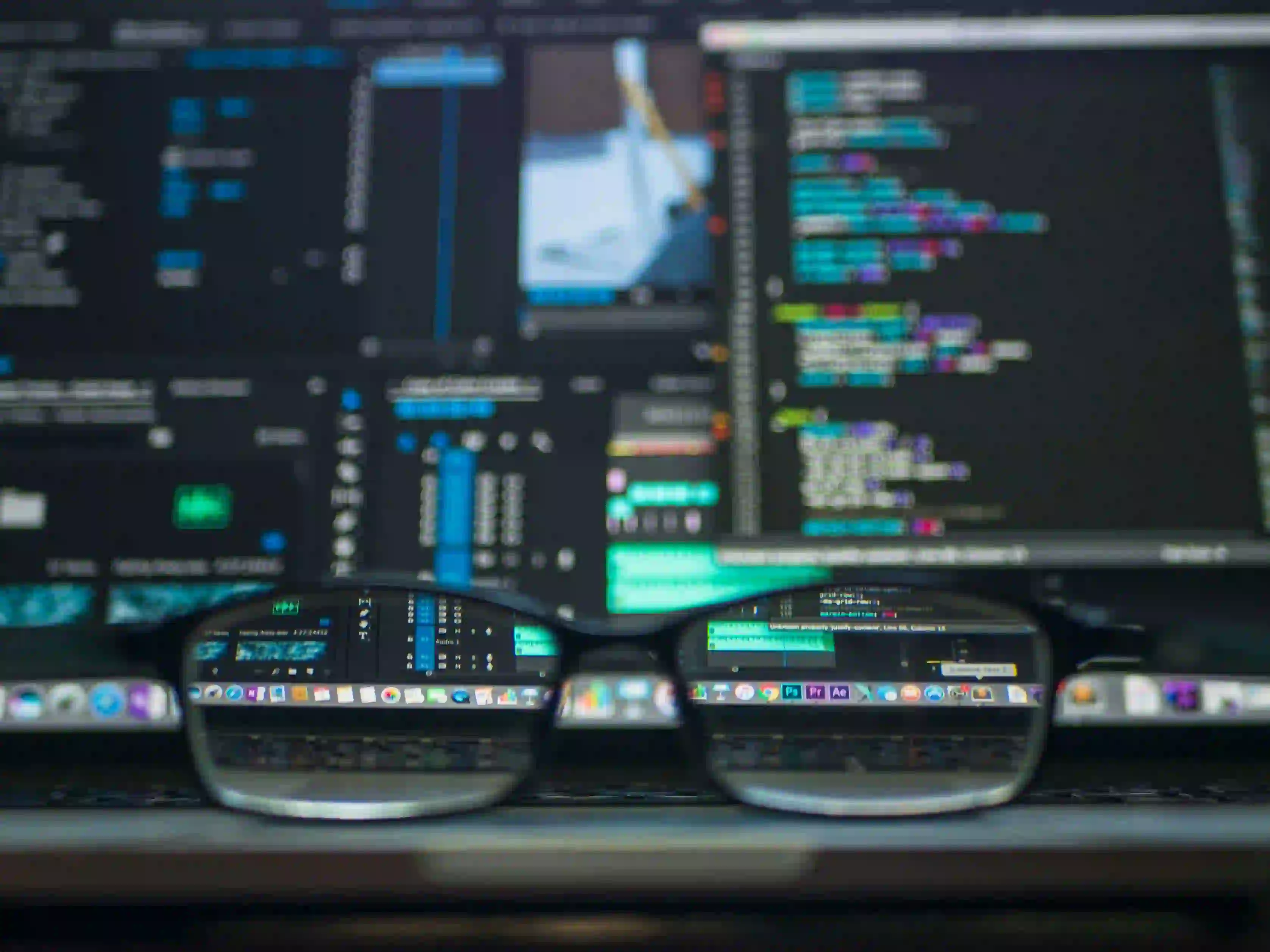
Overcoming Common Pitfalls in Java Command-Line Execution
Java is a powerful and versatile programming language that has been a staple in software development for decades. One aspect of Java that often trips up even seasoned developers is executing Java applications from the command line. This blog post will help you navigate common pitfalls associated with Java command-line execution, ensuring you can run your applications smoothly and efficiently.
Understanding the Java Command-Line Environment
Before diving into the common pitfalls, let's lay the groundwork on how the Java command-line environment works. Java is designed to be platform-independent. The Java compiler (javac
) compiles your Java source files, and the Java Virtual Machine (JVM) executes the compiled bytecode.
Here's a brief overview of the commands you'll commonly encounter:
javac
- Compiles Java source files.java
- Executes Java applications.
To execute your Java application, you generally follow these steps:
- Write your code in a file (e.g.,
HelloWorld.java
). - Compile the file:
🔧snippet.sh
javac HelloWorld.java
- Run the compiled bytecode:
🔧snippet.sh
java HelloWorld
Now, let's look at common pitfalls while executing Java applications from the command line.
Pitfall 1: Incorrect Java Installation
The Issue
Sometimes, developers encounter problems stemming from an incorrect installation of the Java Development Kit (JDK).
The Solution
Before running any Java commands, ensure that the JDK is installed correctly. You can verify the installation by opening your command-line interface (CLI) and typing:
java -version
This command should return the version of Java that is installed, confirming it is set up correctly. If you get an error or an unexpected version, consider reinstalling or adjusting your Java installation.
Why This Matters
Having a proper setup will not only streamline your development process but will also prevent numerous errors when running commands later.
Pitfall 2: Class Not Found
The Issue
A common error is the "Class not found" exception when attempting to run a Java program. This usually happens due to issues with the classpath configuration.
The Solution
Ensure that you are compiling and executing your Java files from the correct directory and using the correct fully qualified class name. If your file structure looks like this:
src/
└── com/
└── example/
└── HelloWorld.java
You should navigate to the src
directory before compiling:
cd src
javac com/example/HelloWorld.java
java com.example.HelloWorld
Why This Matters
Understanding how classpaths work in Java can save you a lot of headaches. It is essential to know the structure of your project and how to navigate it efficiently in order to avoid these types of errors.
Pitfall 3: Missing Main Method
The Issue
Receiving a "No main method found" error can be frustrating, especially for beginners. Java applications require an entry point, which is designated by the main
method.
The Solution
Ensure your Java class contains the following main
method:
public static void main(String[] args) {
System.out.println("Hello, World!");
}
This method serves as the starting point. If it’s missing, you will encounter an error when trying to run your program.
Why This Matters
The main
method is crucial for any standalone Java application. Without it, the JVM has no clue where to start, which leads directly to the runtime error.
Pitfall 4: Working Directory Issues
The Issue
Users might find that their program runs correctly in an IDE but fails from the command line due to discrepancies between the working directory.
The Solution
Always be conscious of the working directory. Use the pwd
command (print working directory) in Unix-based systems or cd
in Windows to check where you currently are within the file system before running your command.
Example:
pwd # Unix/Linux
cd # Windows
And ensure you're running your commands from the appropriate directory.
Why This Matters
The working directory dictates where the JVM looks for resources. If your resources (like properties files, images, or other Java classes) aren't situated where expected, your program will fail to locate them.
Pitfall 5: Classpath Issues
The Issue
Classpath issues manifest as runtime errors. If you’re using external libraries, you might run into ClassNotFoundException
.
The Solution
Setting the classpath is vital to finding your classes and libraries. Use the -cp
or -classpath
option to specify your paths correctly:
Example:
java -cp ".;path/to/library.jar" com.example.HelloWorld
On UNIX systems, use a colon :
instead of a semicolon ;
.
Why This Matters
Using libraries efficiently can greatly enhance your capabilities as a developer. The proper setup of the classpath allows you to utilize external functionalities without running into missing library errors.
Final Thoughts
Navigating the command line in Java may initially seem daunting, but understanding these common pitfalls will empower you to run your applications confidently. Always verify your setup, maintain awareness of your working directory, ensure your classpath is set, and don't forget the main
method.
For more in-depth discussions on Java execution and best practices, feel free to check out Oracle's official Java documentation and Baeldung for various tutorials and examples.
Equipped with this knowledge, you will spend less time troubleshooting and more time creating robust Java applications. Happy coding!