Mastering Java: Create Mappers Without Underlying Objects!
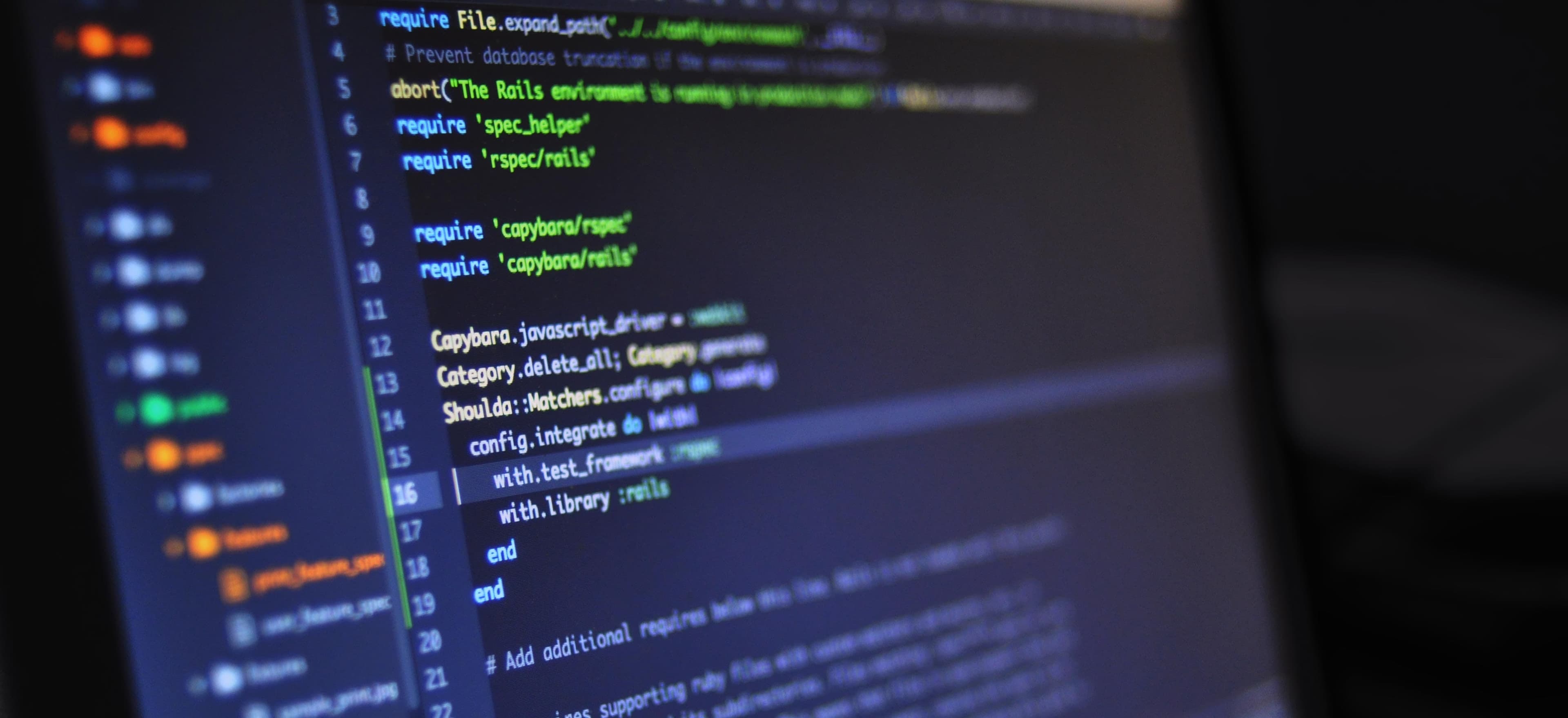
- Published on
Mastering Java: Create Mappers Without Underlying Objects!
In the world of Java development, efficiently managing data transformations between different layers of an application is crucial. A common approach is to use mappers, which convert data between various formats or structures. In this blog post, we will focus on how to create mappers without the need for underlying objects, allowing for a more streamlined process.
Table of Contents
- Understanding Mappers
- Why Use Mappers?
- Creating Mappers Without Underlying Objects
- Example: Simple Data Transformation
- Best Practices
- Conclusion
- Further Reading
Understanding Mappers
Mappers are software constructs that facilitate the conversion of data from one representation to another. In Java, they typically translate domain models to DTOs (Data Transfer Objects) and vice versa. This process is often necessary when interfacing with web services, databases, or significant internal application components.
Theoretically, the presence of an underlying object (the original data structure) can often add complexity. What if we could create a mapping solution directly from raw data? This is where mappers without underlying objects shine.
Why Use Mappers?
Before diving into the implementation, let's discuss the advantages of employing mappers:
-
Separation of Concerns: Mappers allow you to decouple different layers of your application, like service and presentation layers.
-
Simplification: Creating mappers without an underlying object can simplify data transformation. This approach can help remove the intricacies associated with maintaining additional classes or models.
-
Flexibility: By using a lightweight mapper, you can easily handle dynamic datasets, especially in applications that involve external data sources.
Creating Mappers Without Underlying Objects
In typical Java applications, mappers work by converting between classes. However, you can streamline the process by utilizing Java's built-in capabilities, such as Map
, List
, and functional interfaces. Let's see how to achieve this.
Using Maps for Mapping
You can use a Map<String, Object>
to represent key-value pairs. Here's a basic structure:
import java.util.HashMap;
import java.util.Map;
public class MapMapper {
public static void main(String[] args) {
// Sample source data
Map<String, Object> sourceData = new HashMap<>();
sourceData.put("firstName", "John");
sourceData.put("lastName", "Doe");
sourceData.put("age", 30);
// Map to transform data
Map<String, Object> transformedData = mapData(sourceData);
// Output the transformed data
System.out.println(transformedData);
}
public static Map<String, Object> mapData(Map<String, Object> source) {
Map<String, Object> target = new HashMap<>();
// Transforming the data (e.g., combining first and last name)
String fullName = source.get("firstName") + " " + source.get("lastName");
target.put("fullName", fullName);
target.put("age", source.get("age"));
return target;
}
}
Code Explanation
- Source Data: We create a sample
Map
to represent incoming data. This is essential for testing how the mapping works. - mapData() Method: This method takes the source
Map
, performs transformations, and returns a newMap
. - Transformation Logic: Here, we create a full name and retain the age. You can expand this logic as needed.
This approach avoids the need for additional classes and focuses solely on the mapping logic.
Example: Simple Data Transformation
Let's build on our previous example by implementing a more complex transformation. Suppose we want to convert data for a user registration process.
public class UserMapper {
public static void main(String[] args) {
// Sample registration data
Map<String, Object> registrationData = new HashMap<>();
registrationData.put("username", "johndoe");
registrationData.put("email", "john.doe@example.com");
registrationData.put("password", "securePassword");
// Data mapped to DTO format
Map<String, Object> userDTO = mapRegistrationData(registrationData);
// Output the transformed data
System.out.println(userDTO);
}
public static Map<String, Object> mapRegistrationData(Map<String, Object> source) {
Map<String, Object> userDTO = new HashMap<>();
// Example combining, encrypting, and formatting data
userDTO.put("userId", source.get("username")); // Using username as userID
userDTO.put("emailAddress", source.get("email"));
userDTO.put("hashedPassword", hashPassword((String) source.get("password"))); // Simulate a hashing process
return userDTO;
}
public static String hashPassword(String password) {
// Simple hash simulation (don't use this in production)
return "hashed_" + password;
}
}
Code Explanation
- Registration Data: Another
Map
holds user registration information. - mapRegistrationData() Method: This method maps registration data to a user DTO format, such as changing names and hashing passwords.
- Password Hashing: A mock password hashing function demonstrates how to incorporate additional logic within your mapping method.
Best Practices
Creating mappers without underlying objects can yield clear advantages, but it is essential to adhere to some best practices to maintain code quality and ease of use:
-
Keep It Simple: Overcomplicating mappers can lead to maintenance issues. Aim for straightforward and clean mappings.
-
Reuse Logic: If you have mapping logic that applies in multiple places, consider encapsulating that logic in methods for reuse.
-
JSON Support: Java has libraries such as Jackson or GSON that can assist in transforming data structures. For complex conversions, consider enhancing your mappers with serialization capabilities. Learn more about Jackson.
-
Validation: Include validation checks to handle cases where expected data might be missing.
The Closing Argument
Mappers provide powerful tools to ease data transformations without the burden of additional underlying classes. By leveraging Java’s built-in data structures like Maps and Lists, you can create flexible, efficient mappers that meet your application’s needs.
In this blog post, we've explored practical implementations and best practices for creating mappers without underlying objects. Whether you are a novice or a seasoned developer, applying these concepts can yield clean and maintainable code.
Further Reading
- Java Collections Framework
- Understanding Data Transfer Objects (DTOs)
By mastering these techniques, you can enhance your Java applications, making them more robust and adaptable. Happy coding!