Struggling with Mock Injection in Spock Spring Tests?
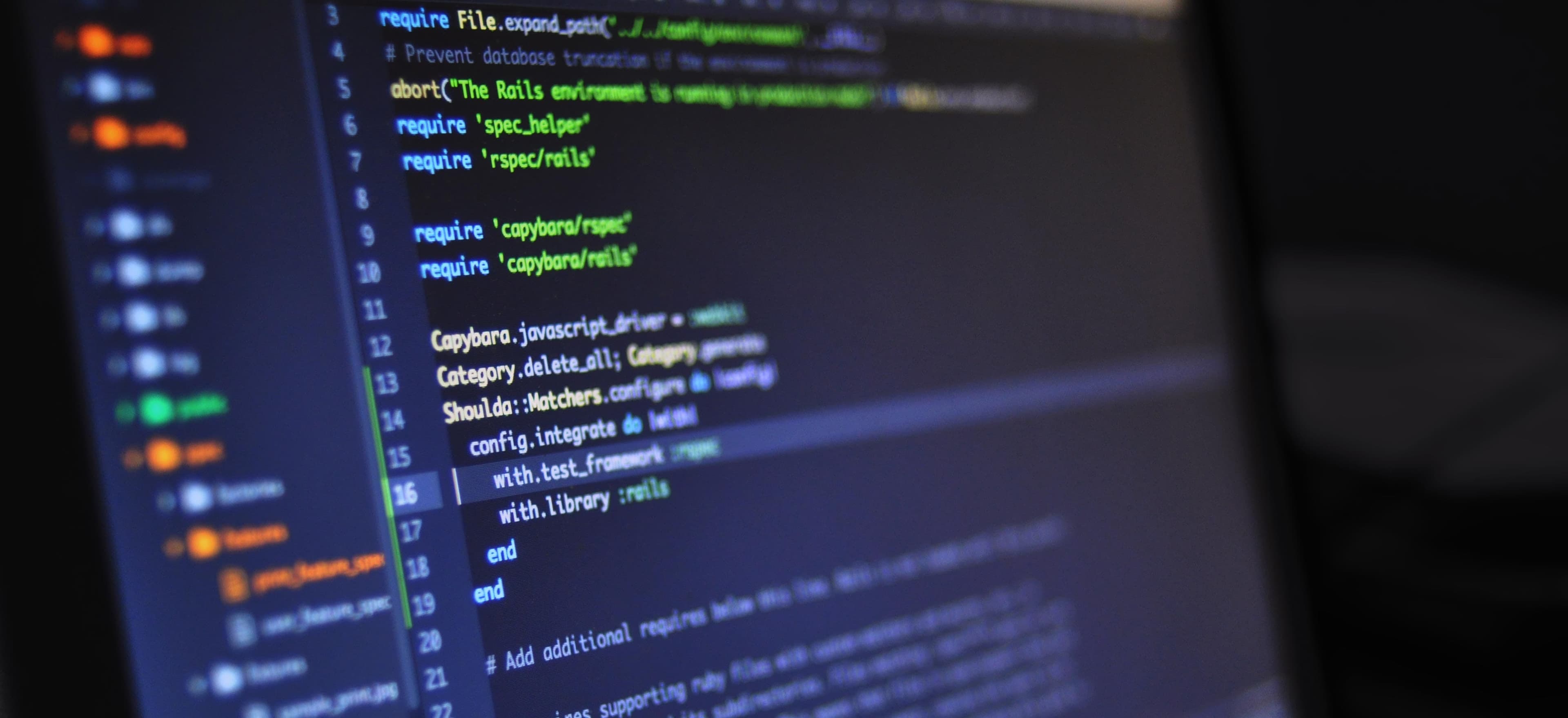
- Published on
Struggling with Mock Injection in Spock Spring Tests?
If you are a developer working with Java, Spring, and Spock, you might have encountered the intricacies of mocking and dependency injection in your tests. Mock injection is a powerful feature that allows you to isolate units of your application, making your tests more reliable and easier to maintain. In this blog post, we will explore how to effectively use mock injection with Spock in your Spring tests.
What is Spock?
Spock is a testing and specification framework for Java and Groovy applications. It is particularly well-suited for testing Spring applications due to its concise syntax and expressive features. The framework uses JUnit as its underlying engine, making it compatible with existing JUnit tests.
Why Mock Injection?
Mocking is a technique where real dependencies are replaced with mock objects. This allows for:
- Isolation of the unit being tested.
- Faster tests since mocks do not require a real implementation.
- Control over the behavior of dependencies.
Mock injection is essential for unit tests, especially for managing external services or databases that you may not want to include in every test scenario.
Setting Up Your Environment
Before diving into code examples, make sure you have the following dependencies in your Maven or Gradle project.
Maven
<dependency>
<groupId>org.spockframework</groupId>
<artifactId>spock-core</artifactId>
<version>2.3-groovy-3.0</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
Gradle
testImplementation 'org.spockframework:spock-core:2.3-groovy-3.0'
testImplementation 'org.springframework.boot:spring-boot-starter-test'
Basic Mock Injection with Spock
Let's take a look at a simple example. We have a service class that depends on a repository interface. We want to test this service without hitting the database.
Example Service Class
@Service
public class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User getUserById(Long id) {
return userRepository.findById(id).orElse(null);
}
}
Example Repository Interface
public interface UserRepository extends JpaRepository<User, Long> {
}
Spock Test Class
import org.springframework.beans.factory.annotation.Autowired
import org.springframework.boot.test.context.SpringBootTest
import org.springframework.boot.test.mock.mockito.MockBean
import spock.lang.Specification
import static org.mockito.Mockito.when
@SpringBootTest
class UserServiceTest extends Specification {
@MockBean
UserRepository userRepository
@Autowired
UserService userService
def "test getUserById with existing user"() {
given:
Long userId = 1L
User user = new User(id: userId, name: "John Doe")
// Mock repository behavior
when(userRepository.findById(userId)).thenReturn(Optional.of(user))
when:
User result = userService.getUserById(userId)
then:
result.name == "John Doe"
}
def "test getUserById with non-existing user"() {
given:
Long userId = 2L
// Mock repository to return empty Optional
when(userRepository.findById(userId)).thenReturn(Optional.empty())
when:
User result = userService.getUserById(userId)
then:
result == null
}
}
Explanation of the Code
- @SpringBootTest: This annotation tells Spring to start the ApplicationContext for the test. It's handy for loading the whole application context.
- @MockBean: This annotation is used to create a mock of the
UserRepository
. When theUserService
is autowired, it will use this mock instead of a concrete implementation. - when-then syntax: The
when
clause defines the behavior of the mock while thethen
clause verifies the outcome.
In the first test case, we check if the getUserById
method returns the expected user when an existing user ID is provided. In the second case, we ensure it returns null for a non-existent user.
Advanced Mock Injection with Spock
In many cases, you might need more flexibility in your mocked service responses. Let's expand our initial setup to include a scenario where the mock can throw an exception.
Updated UserService
public class UserService {
// Other methods...
public User getUserByIdWithException(Long id) {
return userRepository.findById(id)
.orElseThrow(() -> new UserNotFoundException("User not found"));
}
}
Updated Spock Test Class
def "test getUserById with exception"() {
given:
Long userId = 3L
// Mock repository to throw an exception
when(userRepository.findById(userId)).thenThrow(new UserNotFoundException("User not found"))
when:
userService.getUserByIdWithException(userId)
then:
thrown(UserNotFoundException)
}
Explanation of Advanced Test
In this new test, we are validating that the UserService
properly handles the case where a user is not found by throwing a custom exception.
- thenThrow: This method tells the mock behavior to throw an exception upon being called with a specific parameter.
- thrown: This Spock method asserts that the expected exception was thrown during the execution of the method.
Final Thoughts
Mock injection in Spock tests for Spring applications offers a robust way to isolate and test your services without the need for real implementations. By using mocking effectively, you can run fast, reliable, and meaningful tests.
For further reading on Spock and testing practices, consider the following resources:
By mastering these techniques, you will not only streamline your testing process but also enhance the overall quality of your code. Happy testing!