Common Pitfalls in Scaling Enterprise Systems Successfully
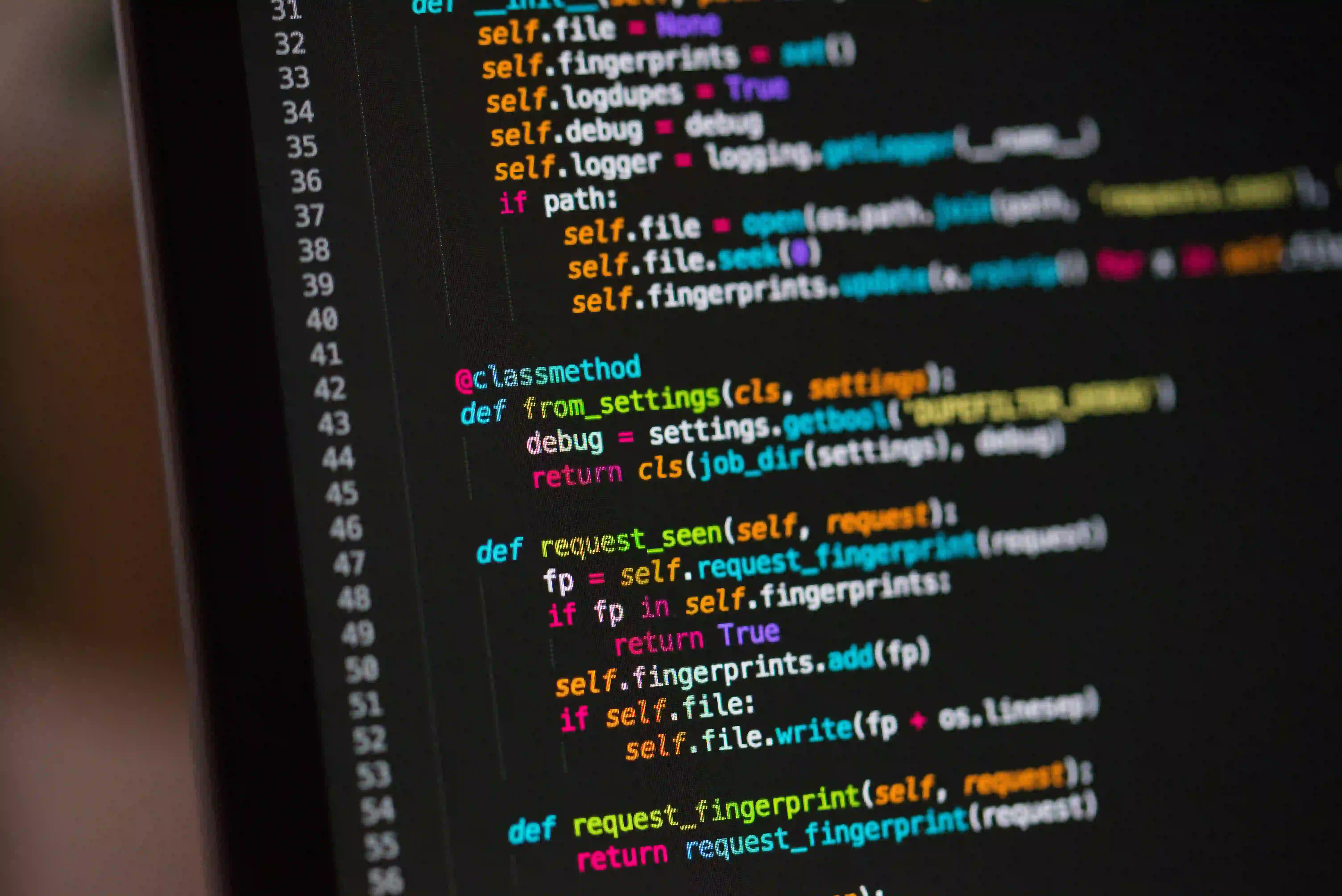
Common Pitfalls in Scaling Enterprise Systems Successfully
In today's fast-paced digital world, scaling enterprise systems is a crucial endeavor for organizations aiming to remain competitive and responsive to market demands. However, scaling is not just about increasing capacity or adding resources; it involves a holistic approach that incorporates architecture, operations, and culture. Without careful planning and execution, organizations can encounter significant pitfalls. This blog will discuss some of the most common pitfalls associated with scaling enterprise systems and provide insights on avoiding them.
1. Underestimating Technical Debt
What is Technical Debt?
Technical debt refers to the implied cost of additional rework caused by choosing an easy solution today instead of using a better approach that would take longer. As systems scale, the complexity increases, and existing technical debt can spiral out of control, leading to reduced agility and increased risks of failure.
Why This is a Pitfall
When scaling, teams often prioritize new features over addressing technical debt. Ignoring this can lead to more complicated systems and longer development cycles.
How to Avoid It
Conduct Regular Assessments: Schedule periodic reviews of the codebase to identify and address technical vulnerabilities.
Create a Balanced Roadmap: Allocate time for both new development and technical debt repayment.
// Example of refactoring a piece of code to avoid technical debt
public class OrderService {
// Old method - tightly coupled and hard to maintain
public void processOrder(Order order) {
// Processing logic here...
}
// New method - more modular and testable
public void processOrder(Order order) {
validateOrder(order);
chargeCustomer(order);
notifyCustomer(order);
}
private void validateOrder(Order order) {
// Validation logic here
}
private void chargeCustomer(Order order) {
// Charging logic here
}
private void notifyCustomer(Order order) {
// Notification logic here
}
}
In the refactored example, breaking down the processOrder
method into smaller, modular components enhances maintainability and readability, paving the way for easier scaling.
2. Lack of Clear Architectural Vision
The Importance of Architecture
A well-defined architecture lays the groundwork for scaling. It ensures that systems can handle increased loads without failure. Many enterprises overlook this aspect, diving straight into scaling efforts.
Consequences of Poor Architecture
Without a clear architectural vision, systems may face bottlenecks that lead to performance issues. This can result in downtime, frustrating users, and tarnishing the brand's reputation.
How to Establish a Strong Architectural Vision
Document Architectural Decisions: Create a repository of architectural choices, justifications, and consequences.
Adopt Microservices: Consider transitioning to a microservices architecture to enhance scalability and flexibility.
3. Insufficient Testing and Monitoring
The Necessity of Robust Testing
When scaling, it is vital to ensure that the systems are thoroughly tested at every stage. Many organizations fail to implement comprehensive testing strategies, leading to serious unforeseen issues.
The Role of Monitoring
Proper monitoring tools can provide insights into system performance. Without them, anomalies may go unnoticed until significant damage occurs.
Strategies for Effective Testing and Monitoring
Implement CI/CD Pipelines: Continuous Integration and Continuous Deployment practices can catch bugs early in the development cycle.
Utilize APM Tools: Application Performance Management (APM) tools like New Relic or AppDynamics can provide visibility into system performance.
// Example of a simple health check in a Spring Boot application
@RestController
public class HealthCheckController {
@GetMapping("/health")
public ResponseEntity<String> checkHealth() {
// This could include more complex checks
return ResponseEntity.ok("System is healthy");
}
}
By implementing a health check endpoint, operations teams can continuously monitor the system's state and quickly identify issues.
4. Overloading Teams and Resources
The Reality of Resource Management
Scaling often brings the temptation to push teams beyond their limits in hopes of faster results. Overloading teams can lead to burnout and decreased productivity.
Recognizing Limits
Ignoring the limits of your developers or infrastructure can result in diminished returns as quality suffers.
Best Practices for Resource Management
Set Realistic Goals: Understand what your team can accomplish in a given timeframe and set targets accordingly.
Invest in Training: Provide training and development opportunities to ensure that teams are equipped with the latest skills and knowledge.
5. Neglecting Data Management
Data: The Lifeblood of Enterprises
As systems scale, the volume of data they handle often grows exponentially. Without proper data management practices, organizations can face challenges related to data integrity, accessibility, and security.
Potential Complications
Poor data management can lead to slow queries, data collisions, and security vulnerabilities. These issues can severely affect user experience and trust.
Strategies for Effective Data Management
Implement Data Governance: Establish protocols for data quality, privacy, and security.
Utilize High-Performance Databases: Make use of databases that support sharding and replication to handle increased data loads efficiently.
// Example of using JPA with Hibernate for scalable data management
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false, unique = true)
private String username;
@Column(nullable = false)
private String password;
}
// Creating the User Repository
public interface UserRepository extends JpaRepository<User, Long> {
Optional<User> findByUsername(String username);
}
Using JPA with Hibernate for user data management allows for scalable, secure, and straightforward access to user information.
6. Failing to Foster a Culture of Innovation
Culture as a Catalyst
A culture that embraces innovation encourages team members to propose new ideas and challenge the status quo. Unfortunately, many organizations focus solely on processes and neglect this vital aspect.
Cultural Barriers to Scaling
A lack of innovation can stall scaling efforts, making it difficult to adapt to new technologies and methodologies.
How to Cultivate Innovation
Encourage Experimentation: Allow team members to experiment and share their findings without fear of failure.
Celebrate Successes and Learn from Failures: Create a safe environment for discussing both achievements and setbacks.
To Wrap Things Up
Scaling enterprise systems successfully requires a multifaceted approach. While technical aspects such as architecture, testing, and data management are essential, an organization's culture and resourcing are equally paramount. By understanding and mitigating common pitfalls, businesses can create resilient systems equipped to handle future growth.
Further Reading
- Microservices Architecture
- Continuous Integration and Continuous Deployment
- Effective Data Governance
By carefully addressing these factors, organizations can ensure smooth scaling, better performance, and improved customer satisfaction. Happy scaling!