Mastering Phone Number Validation in Java: Avoid Common Errors
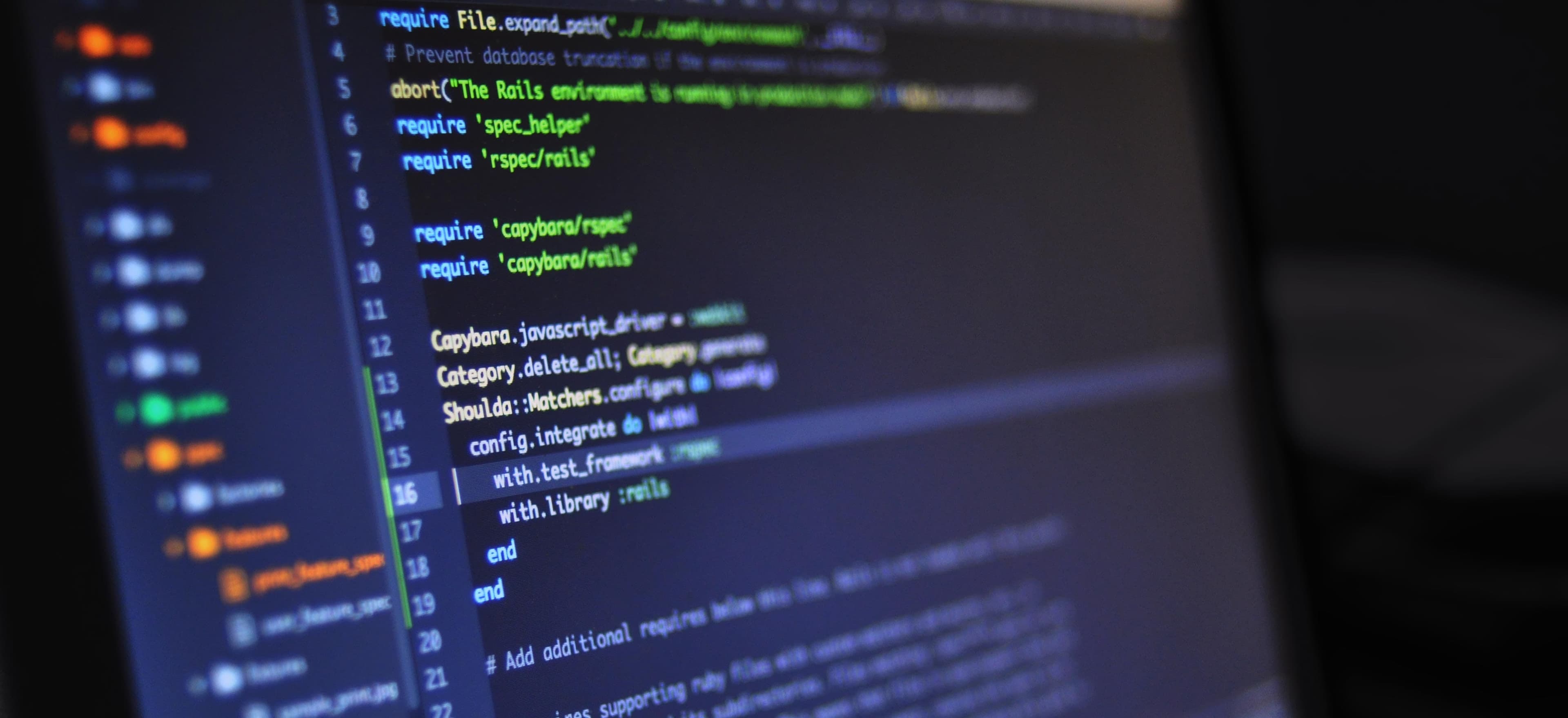
- Published on
Mastering Phone Number Validation in Java: Avoid Common Errors
Phone number validation is a crucial task in software development. An invalid phone number can lead to user frustration, miscommunication, and other downstream errors. In this blog post, we will explore effective techniques for validating phone numbers in Java. Through clear examples, you'll gain a deeper understanding of the common pitfalls and best practices for accurate phone number validation.
Why Validate Phone Numbers?
Validating phone numbers helps ensure data accuracy and consistency. Without proper validation, your application might:
- Allow formatting errors.
- Accept invalid numbers.
- Cause issues during user communication.
For instance, if a user inputs (123) 456-7890 but we store it as 1234567890 without understanding the context, we lose the ability to format or interpret it correctly later. Thus, validation is essential.
Common Phone Number Formats
Understanding different phone number formats is critical. Here are some common formats:
- North American Numbering Plan (NANP): (123) 456-7890 / 123-456-7890 / 1234567890
- International Format: +1 123 456 7890 / +44 20 7946 0958
- Local Formats: 0123 456 7890 (depending on regional needs)
Keeping these formats in mind, we can create a robust validation strategy.
Regex for Phone Number Validation
One of the most prevalent methods for validating phone numbers is using regular expressions (regex). Regex allows us to match strings against defined patterns. Below is an example of how to use regex for this purpose in Java.
Basic Regex Pattern
Let's start with a basic regex pattern for North American phone numbers.
String regex = "^\\(?\\d{3}\\)?[-\\s]?\\d{3}[-\\s]?\\d{4}$";
Code Example
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class PhoneNumberValidator {
private static final String PHONE_REGEX = "^\\(?\\d{3}\\)?[-\\s]?\\d{3}[-\\s]?\\d{4}$";
public static boolean isValidPhoneNumber(String phoneNumber) {
Pattern pattern = Pattern.compile(PHONE_REGEX);
Matcher matcher = pattern.matcher(phoneNumber);
return matcher.matches();
}
public static void main(String[] args) {
String[] testNumbers = {
"(123) 456-7890",
"123-456-7890",
"1234567890",
"123 456 7890",
"invalidNumber"
};
for (String number : testNumbers) {
System.out.println(number + ": " + isValidPhoneNumber(number));
}
}
}
Explanation
- Regex Breakdown:
^
and$
define the start and end of the string.\\(?\\d{3}\\)?
matches three digits, optionally enclosed in parentheses.[-\\s]?
allows for either a dash or space after the area code.\\d{3}[-\\s]?\\d{4}
completes the pattern for the main number.
- Code Walkthrough:
- The
isValidPhoneNumber
method takes a phone number as input and checks it against the defined regex. - The
main
method tests several numbers to see which are valid, printing results accordingly.
- The
This simple method will catch several format errors, but we should also consider edge cases and varying requirements.
Advanced Validation Techniques
1. International Numbers
For international numbers, we can modify our regex or utilize a library like Google’s libphonenumber. This library provides comprehensive validation and formatting rules for billions of phone numbers around the world.
Example Using Libphonenumber
import com.google.i18n.phonenumbers.PhoneNumberUtil;
import com.google.i18n.phonenumbers.Phonenumber;
public class AdvancedPhoneNumberValidator {
public static boolean isValidInternationalPhoneNumber(String phoneNumber, String regionCode) {
PhoneNumberUtil phoneUtil = PhoneNumberUtil.getInstance();
try {
Phonenumber.PhoneNumber numberProto = phoneUtil.parse(phoneNumber, regionCode);
return phoneUtil.isValidNumber(numberProto);
} catch (Exception e) {
return false;
}
}
public static void main(String[] args) {
String internationalNumber = "+1 202-555-0176";
boolean isValid = isValidInternationalPhoneNumber(internationalNumber, "US");
System.out.println("Is valid international number: " + isValid);
}
}
Explanation
- The
isValidInternationalPhoneNumber
function uses thePhoneNumberUtil
to parse and validate the phone number based on the specified region code. - The method catches exceptions to handle invalid inputs gracefully, returning false in such cases.
2. Integrating with User Interfaces
When working with user interfaces, consider providing feedback to users. Here are some tips:
- Real-time Validation: Provide users with immediate feedback on the validity of their input.
- Formatting Suggestions: Suggest correct formats as users type.
Common Errors to Avoid
- Ignoring Formatting: Users input numbers in various formats. Have your validation logic flexible enough to accommodate.
- Over-restrictive Validation: Your regex might be too strict, leading to false negatives (valid numbers marked as invalid).
- Not Considering Regions: Phone numbers vary by region. Ensure your validation logic considers this.
Wrapping Up
Phone number validation in Java is an essential task that can enhance user experience and data integrity. By utilizing regex for simple formats, and advanced libraries for international formats, you can create robust validation mechanisms.
Remember to keep your code clean, avoid common pitfalls, and always provide user-friendly feedback.
For more detailed discussions on this topic, check Java Documentation and explore related libraries on Maven Repository.
Happy coding!
Checkout our other articles