Common Pitfalls When Starting with Jakarta Faces
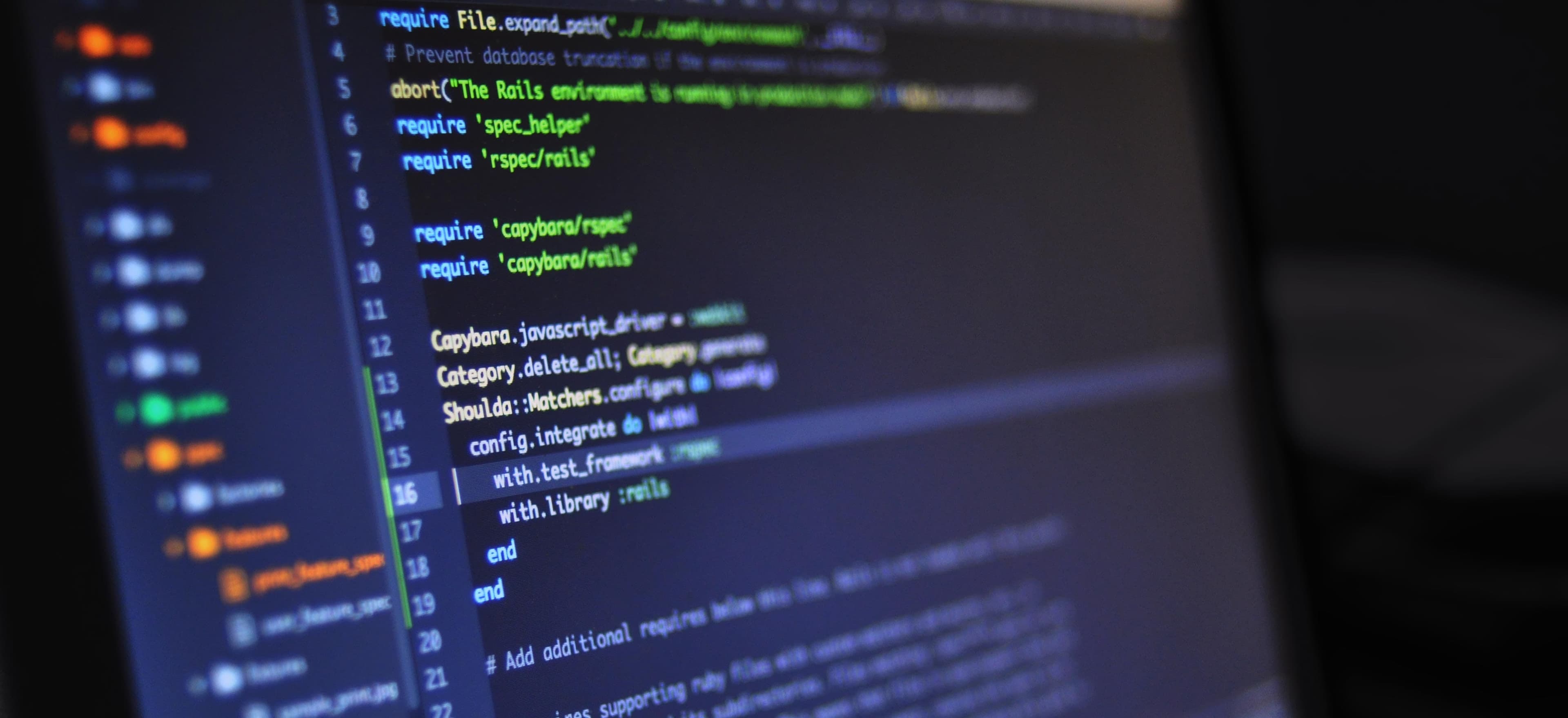
- Published on
Common Pitfalls When Starting with Jakarta Faces
In the world of Java web applications, Jakarta Faces, formerly known as JavaServer Faces (JSF), plays a pivotal role in facilitating component-based user interfaces. While it offers a robust environment for building web applications, many newcomers encounter challenges that can hinder their productivity and learning curve. This blog post addresses common pitfalls when starting with Jakarta Faces and provides tips to navigate these challenges effectively.
Understanding Jakarta Faces
Jakarta Faces simplifies the development of user interfaces for web applications by using a component-oriented approach. It abstracts many complexities associated with HTTP and HTML, allowing developers to focus on business logic rather than the intricacies of server-client interactions.
To better navigate Jakarta Faces, here's a brief overview of its core concepts:
- Components: UI elements such as buttons, text fields, and data tables.
- Managed Beans: Java classes that back the UI components, holding the application state.
- Navigation: Manage the flow of the web application between views.
For a deep dive into Jakarta Faces and its architecture, check out the official Jakarta Faces documentation.
Pitfall 1: Misunderstanding the Lifecycle
One of the most common missteps in Jakarta Faces is not understanding its lifecycle. The Faces lifecycle consists of several phases, including Restore View, Apply Request Values, Process Validations, Update Model Values, Invoke Application, and Render Response.
Why It Matters
Each phase has a specific role, and failing to grasp this can lead to unintended behaviors, such as data not being updated correctly or validation errors not firing.
Example
Here's a simple managed bean demonstrating a misunderstanding of lifecycles:
@ManagedBean
@SessionScoped
public class UserBean {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
// Incorrectly assuming this will update the UI immediately
this.name = name;
// Not considering the lifecycle phases
}
}
In the above code, the setName
method does not account for when the component gets rendered again. To manage the state properly, utilize the right lifecycle phase.
Tip
Familiarize yourself with the Jakarta Faces lifecycle documentation to understand how data flows from the UI to your managed beans.
Pitfall 2: Overcomplicating Navigation
Jakarta Faces provides a built-in navigation system, but leveraging it incorrectly is another common pitfall. New developers often design complex navigation rules without making effective use of the framework's capabilities.
Why It Matters
An overly complex navigation configuration can lead to confusion, making code harder to maintain and debug.
Example
Consider the following navigation rules defined in faces-config.xml
:
<navigation-rule>
<from-view-id>/home.xhtml</from-view-id>
<navigation-case>
<from-outcome>next</from-outcome>
<to-view-id>/next.xhtml</to-view-id>
</navigation-case>
<!-- More navigation cases -->
</navigation-rule>
While this defines a navigation rule, excessive navigation cases can complicate logic unnecessarily. A simpler approach, like using implicit navigation (directly returning a string from an action method), enhances maintainability.
Tip
Utilize implicit navigation whenever possible. For instance:
public String goToNext() {
return "next"; // Implicitly navigates to 'next.xhtml'
}
This approach reduces complexity and makes the code easier to read.
Pitfall 3: Not Leveraging Component Libraries
Many developers start using Jakarta Faces without leveraging powerful libraries such as PrimeFaces, OmniFaces, or MyFaces. These libraries provide a rich set of UI components and extend the functionality of standard Jakarta Faces.
Why It Matters
By not taking advantage of these libraries, you risk reinventing the wheel and missing out on enhanced features, responsive components, and improved user experiences.
Example
Here's an illustration of the difference between using basic components and leveraging PrimeFaces:
<!-- Basic Jakarta Faces component -->
<h:inputText value="#{userBean.name}" />
<!-- PrimeFaces InputText with additional features -->
<p:inputText value="#{userBean.name}" required="true" placeholder="Enter your name"/>
The PrimeFaces InputText
component has built-in attributes for validation and styling, which reduces the need for additional coding efforts.
Tip
Explore the features provided by component libraries to improve productivity and enhance user experience. A good starting point is the PrimeFaces Showcase.
Pitfall 4: Ignoring Validation and Error Handling
Error handling and validation are critical aspects of any user interface. However, newcomers often overlook implementing effective validation and error handling strategies in their Jakarta Faces applications.
Why It Matters
Neglecting these aspects can lead to a poor user experience and data integrity issues.
Example
A simple managed bean demonstrating a lack of validation:
@ManagedBean
@ViewScoped
public class UserBean {
private String email;
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
// Not validating email format here
}
}
While setters are convenient, they do not facilitate validation for critical fields like email.
Tip
Incorporate built-in validation components or create custom validators. Here's how to implement a basic validation:
<h:inputText id="email" value="#{userBean.email}" required="true">
<f:validateRegex pattern="^[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\\.[A-Z|a-z]{2,}$" />
<f:message for="email" />
</h:inputText>
This input field validates the email format before submission, enhancing data quality.
Pitfall 5: Not Structuring Applications Properly
Application structure plays a crucial role in maintainability. Many beginners tend to place all managed beans and resources in a single package without proper organization.
Why It Matters
Poorly organized code can lead to confusion and maintenance challenges as the application grows.
Tip
Organize your application structure logically. Separate managed beans, services, and models into different packages. Follow a nomenclature that reflects your business logic, making it easier to navigate through code.
Closing Remarks
Embarking on your journey with Jakarta Faces can be exhilarating yet challenging. Understanding the framework's core concepts, familiarizing yourself with its lifecycle, leveraging existing libraries, implementing effective validation, and maintaining a well-structured codebase can help you avoid common pitfalls.
For further reading, consult the following resources:
By addressing these pitfalls early on, you will lay a robust foundation for developing intuitive and powerful web applications using Jakarta Faces. Happy coding!