Understanding JSR 107 Caching Annotations Misuse
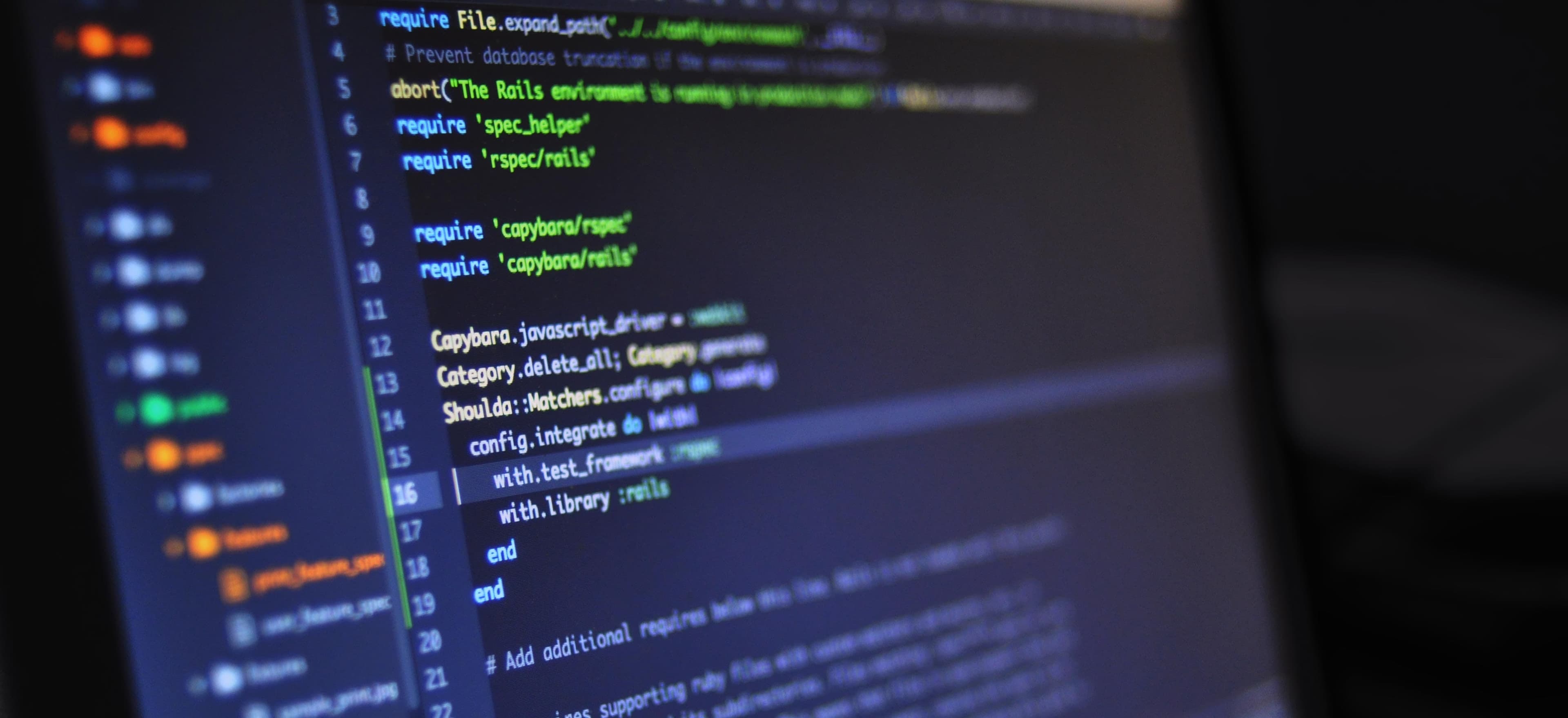
- Published on
Understanding JSR 107 Caching Annotations Misuse
Caching is a key performance enhancement strategy in modern application development. It reduces latency and improves throughput by storing frequently accessed data in memory rather than fetching it from a slower data source. Java provides several mechanisms to implement caching, and one of the most standardized approaches is represented by JSR 107, also known as JCache.
In this blog, we will delve into JSR 107's caching annotations while highlighting common misuses. By the end, you will have a clear understanding of how to utilize these annotations effectively to avoid pitfalls in your applications.
What is JSR 107?
Java Specification Request (JSR) 107 defines a standard caching API for Java, which is designed to provide a consistent way to cache data across different implementations. The key benefits of JSR 107 include:
- Interoperability: Multiple caching solutions can easily plug into this standard, allowing developers to switch providers without significant code changes.
- Annotations: JSR 107 introduces a set of annotations that simplify the usage of caching.
- Flexibility: It allows configuration of caches without modifying code, making management easier.
For detailed specifications of JSR 107, refer to the official document here.
Caching Annotations Overview
JSR 107 provides a handful of annotations:
@Cacheable
: Use this annotation to cache the output of a method.@CachePut
: It updates the cache with the result of a method.@CacheEvict
: It removes entries from the cache.
Example of proper usage of JSR 107 annotations
Let’s walk through a typical use case by creating a service that fetches user data, implementing caching using JSR 107 annotations.
import javax.cache.CacheManager;
import javax.cache.annotation.CacheResult;
import javax.inject.Inject;
public class UserService {
@Inject
private CacheManager cacheManager;
@CacheResult(cacheName = "userCache")
public User getUserById(int id) {
// Simulate a slow DB call
return database.getUser(id);
}
}
Why this code works well
@CacheResult
: It caches the result ofgetUserById
for a specified cache name. If the method is called with the same user ID, the cached result is returned.- Performance: This reduces database hits, improving application performance.
Common Misuses of Caching Annotations
Despite the benefits, many developers fall into common traps when using caching annotations. Below are some of these pitfalls, accompanied by examples of misuses.
1. Over-caching
Developers may cache results that do not require caching, leading to excessive memory use. For instance:
@Cacheable(cacheName = "temporaryDataCache")
public int calculateSum(int a, int b) {
return a + b; // Cached unnecessarily
}
Why this is a problem
Caching this method is unneeded since it generates the same result based on inputs every time. This can waste resources and complicate cache management.
Tip: Cache only data that is expensive to retrieve or compute. Use caching selectively to ensure that your application remains efficient.
2. Inefficient Cache Eviction
Improper usage of @CacheEvict
can lead to stale data being served or cache thrashing.
@CacheEvict(cacheName = "userCache", allEntries = true)
public void updateUser(User user) {
database.update(user);
}
Explanation
Using allEntries = true
will clear the entire cache every time a user is updated. This might be inefficient; for example, if you have a large user base and are only updating a single user, caching every user will lead to unnecessary cache misses.
Tip: Evict only the specific entries that are outdated or related to the update, using key-based eviction.
@CacheEvict(cacheName = "userCache", key = "#user.id")
public void updateUser(User user) {
database.update(user);
}
3. Lazy Loading Misinterpretations
Some developers may misunderstand the necessity of fresh data and thus lazily cache results without clear strategies.
@Cacheable(cacheName = "stockDataCache", condition = "#stockId > 0")
public Stock getStockPrice(int stockId) {
return stockService.getPrice(stockId);
}
Why this could lead to issues
Cached values will remain in memory for as long as the cache is accessible unless evicted. If your stock prices change often, a cache may hold stale data.
Tip: Implement a time-to-live (TTL) policy or configure the @Cacheable
annotation properly to ensure cache consistency.
Best Practices for Using JSR 107 Annotations
To ensure effectiveness when utilizing JSR 107 caching annotations, adhere to the following best practices:
-
Use Caching Judiciously: Analyze performance needs before deciding to cache. Cache only results from expensive computations or slow database calls.
-
Manage Cache Size: Implement cache policies to avoid memory overflow. Consider limits on sizes or entries.
-
Regularly Review Cache Usage: Establish logging to monitor what’s being cached. Regular reviews can help identify ineffective caching strategies.
-
Be Mindful of Concurrency: Concurrent access can lead to race conditions. Ensure that your caching strategies account for multi-threading behavior.
Closing Remarks
Through careful application and understanding of JSR 107 Caching Annotations, Java developers can enhance application performance and create clean, maintainable code. Avoiding common misuses is essential to leverage the full potential of caching without incurring unnecessary costs or complications.
For more in-depth discussion on caching strategies in Java, check out this comprehensive guide on caching in Java.
By adhering to best practices and learning from common pitfalls, you can markedly improve the performance and reliability of your Java applications. Happy coding!
Checkout our other articles