Improving Exception Handling with @ControllerAdvice in Spring 4
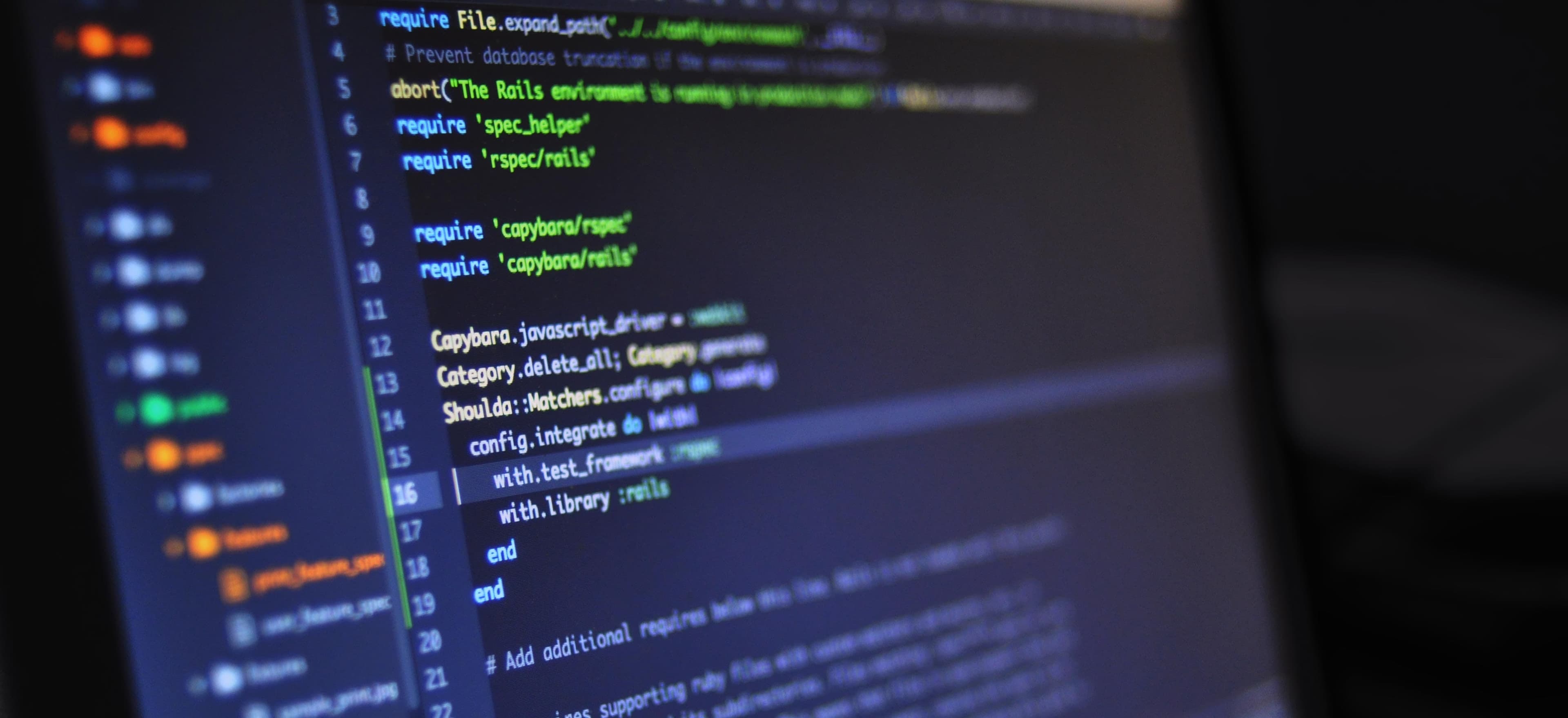
- Published on
Improving Exception Handling with @ControllerAdvice in Spring 4
Exception handling is a crucial part of any web application. Proper exception management can lead to better user experience and easier debugging. In Spring 4, the introduction of @ControllerAdvice
made it easier to handle exceptions globally across all controllers. In this blog post, we will explore how to utilize @ControllerAdvice
for enhanced exception handling in your Spring MVC applications.
What is @ControllerAdvice?
@ControllerAdvice
is a special annotation in the Spring Framework that allows you to define global exception handlers. It provides a way to handle exceptions at a global level, rather than handling them individually in each controller. This centralizes your error handling logic, promotes code reusability, and improves the maintainability of your application.
Advantages of Using @ControllerAdvice
- Centralized Error Handling: Instead of repeating error handling logic in every controller, you can define it once in your
@ControllerAdvice
class. - Cleaner Code: It enhances the readability of your code by keeping the controller logic separate from error handling.
- Custom Response Structure: You can define custom error responses that can include more information, such as error codes and messages.
Setting Up an Example
Before we dive deeper, let’s set up a quick example of a Spring Boot application to demonstrate how @ControllerAdvice
works.
Step 1: Create a Basic Spring Boot Application
If you don't have one set up, create a Spring Boot application using Spring Initializr at start.spring.io.
Make sure to include the following dependencies:
- Spring Web
- Spring Boot DevTools (optional, for development)
Step 2: Create a Simple Controller
Next, we will create a basic controller that throws an exception.
package com.example.demo.controller;
import com.example.demo.exception.ResourceNotFoundException;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class UserController {
@GetMapping("/users/{id}")
public String getUserById(@PathVariable String id) {
if (id.equals("1")) {
return "User found!";
} else {
throw new ResourceNotFoundException("User not found!");
}
}
}
In this example, we have a simple UserController
that returns a user if they exist or throws a ResourceNotFoundException
otherwise.
Step 3: Create the Custom Exception
Now, let's create a custom exception.
package com.example.demo.exception;
public class ResourceNotFoundException extends RuntimeException {
public ResourceNotFoundException(String message) {
super(message);
}
}
The ResourceNotFoundException
extends RuntimeException
, allowing it to be thrown from our controller without being caught or declared.
Step 4: Define the Global Exception Handler
Now it’s time to create the @ControllerAdvice
class that will handle exceptions.
package com.example.demo.advice;
import com.example.demo.exception.ResourceNotFoundException;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<String> handleResourceNotFoundException(ResourceNotFoundException ex) {
return new ResponseEntity<>(ex.getMessage(), HttpStatus.NOT_FOUND);
}
}
Explanation of the Code
-
@ControllerAdvice
: This annotation indicates that the class will contain global exception handling methods. -
@ExceptionHandler(ResourceNotFoundException.class)
: This method will handle any exceptions of typeResourceNotFoundException
. -
ResponseEntity: We are creating a ResponseEntity to return a custom response with an HTTP status code. In this case, a
404 NOT FOUND
along with the error message.
Step 5: Testing the Exception Handling
Now, let’s test our application. Start your Spring Boot application and access the endpoint:
- Valid User:
GET /users/1
- Invalid User:
GET /users/2
When you access GET /users/1
, you will receive "User found!".
When accessing GET /users/2
, you should receive a response with a 404 NOT FOUND
status and an error message "User not found!".
Advanced Customization
Custom Error Response Structure
While the above example returns a simple error message, you might want to return a more structured error response. To do this, you can create a custom error response class.
package com.example.demo.response;
public class ErrorResponse {
private String message;
private int status;
public ErrorResponse(String message, int status) {
this.message = message;
this.status = status;
}
// Getters and setters
}
Update Global Exception Handler
Now, modify the GlobalExceptionHandler
to return this structured response.
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<ErrorResponse> handleResourceNotFoundException(ResourceNotFoundException ex) {
ErrorResponse errorResponse = new ErrorResponse(ex.getMessage(), HttpStatus.NOT_FOUND.value());
return new ResponseEntity<>(errorResponse, HttpStatus.NOT_FOUND);
}
Multiple Exception Handlers
You can handle multiple exceptions by simply adding more methods to your @ControllerAdvice
class.
@ExceptionHandler(NullPointerException.class)
public ResponseEntity<ErrorResponse> handleNullPointerException(NullPointerException ex) {
ErrorResponse errorResponse = new ErrorResponse("Null pointer exception occurred.", HttpStatus.INTERNAL_SERVER_ERROR.value());
return new ResponseEntity<>(errorResponse, HttpStatus.INTERNAL_SERVER_ERROR);
}
Closing the Chapter
By using @ControllerAdvice
in Spring 4, you can streamline your exception handling process. It leads to improved code organization, making your application easier to maintain and evolve.
The showcased patterns can be further expanded to handle various scenarios, such as validating input data, processing other types of exceptions, or customizing the response structure based on your needs.
For more on exception handling in Spring, refer to the official Spring documentation.
Happy coding!