How to Safely Remove Elements from a Java Map
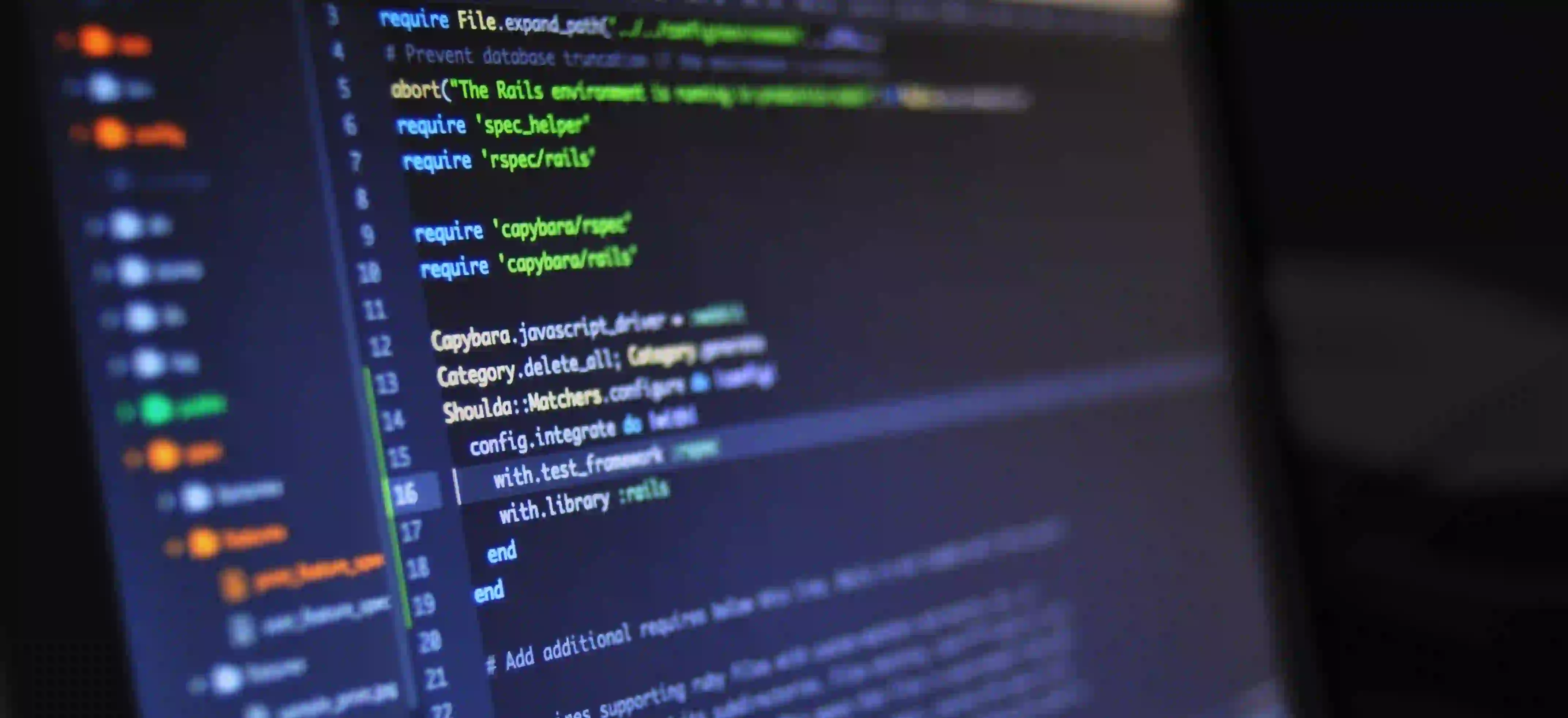
How to Safely Remove Elements from a Java Map
In Java, maps are widely used data structures that associate keys with values. While they provide efficient methods for retrieving, updating, and removing elements, caution must be exercised when removing entries, especially during iteration. This blog post will delve into the various approaches for safely removing elements from a Java map while ensuring that the integrity of the map remains intact.
Understanding Java Maps
Java provides several implementations of the Map
interface, including HashMap
, TreeMap
, and LinkedHashMap
. Each of these implementations has its benefits and drawbacks:
- HashMap: Offers average constant time complexity for operations but does not maintain any order.
- TreeMap: Keeps entries sorted by key, offering logarithmic time complexity for operations.
- LinkedHashMap: Maintains the insertion order, which provides near constant-time performance.
Example of a Java Map
Here's a simple example illustrating a HashMap
usage:
import java.util.HashMap;
import java.util.Map;
public class MapExample {
public static void main(String[] args) {
Map<String, Integer> students = new HashMap<>();
students.put("Alice", 22);
students.put("Bob", 23);
students.put("Charlie", 21);
System.out.println("Students Map: " + students);
}
}
In this example, we create a HashMap
called students
that maps student names to their ages. Now, let's explore how to safely remove elements from this map.
Methods for Removing Elements from a Map
Removing elements from a map can be straightforward, yet doing it during iteration can lead to a ConcurrentModificationException
. Java provides different ways to avoid this issue.
1. Using Iterator
The most effective method is to use an Iterator
. This allows you to safely remove elements while iterating through the map.
Here’s how to do this:
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
public class RemoveWithIterator {
public static void main(String[] args) {
Map<String, Integer> students = new HashMap<>();
students.put("Alice", 22);
students.put("Bob", 23);
students.put("Charlie", 21);
// Use Iterator to remove elements safely
Iterator<Map.Entry<String, Integer>> iterator = students.entrySet().iterator();
while (iterator.hasNext()) {
Map.Entry<String, Integer> entry = iterator.next();
if (entry.getValue() < 22) {
iterator.remove(); // Safe remove
}
}
System.out.println("Updated Students Map: " + students);
}
}
Why Use an Iterator?
Using an Iterator
prevents ConcurrentModificationException
. The remove
method on the Iterator
is specifically designed to safely eliminate entries during iteration, maintaining the state of the map.
2. Using remove
Method
If you know the key of the element you want to remove, you can simply use the remove
method of the map:
public class RemoveByKey {
public static void main(String[] args) {
Map<String, Integer> students = new HashMap<>();
students.put("Alice", 22);
students.put("Bob", 23);
students.put("Charlie", 21);
students.remove("Bob");
System.out.println("Students Map after removal: " + students);
}
}
Why Use the remove
Method?
This method is straightforward and efficient when you have the key readily available. It effectively removes the entry associated with that key.
3. Using removeIf
Method
If you are using Java 8 or later, the removeIf
method can be an elegant solution. Here’s how it works:
import java.util.HashMap;
import java.util.Map;
public class RemoveIfExample {
public static void main(String[] args) {
Map<String, Integer> students = new HashMap<>();
students.put("Alice", 22);
students.put("Bob", 23);
students.put("Charlie", 21);
// Use removeIf with a lambda expression
students.entrySet().removeIf(entry -> entry.getValue() < 22);
System.out.println("Students Map after removeIf: " + students);
}
}
Why Use removeIf
?
This approach allows for succinct code. You can specify a condition in a lambda expression, and all entries matching this condition get removed. This is a clear and effective way to manage map entries in Java 8 and beyond.
4. Deferred Removal with Collection Views
Another technique is to collect entries to remove and remove them after finishing the iteration:
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class DeferredRemoval {
public static void main(String[] args) {
Map<String, Integer> students = new HashMap<>();
students.put("Alice", 22);
students.put("Bob", 23);
students.put("Charlie", 21);
// Collect keys to remove
List<String> keysToRemove = new ArrayList<>();
for (Map.Entry<String, Integer> entry : students.entrySet()) {
if (entry.getValue() < 22) {
keysToRemove.add(entry.getKey());
}
}
// Remove collected keys
for (String key : keysToRemove) {
students.remove(key);
}
System.out.println("Students Map after deferred removal: " + students);
}
}
Why Defer Removal?
Deferring removal allows you to iterate without any special handling, reducing complexity. However, it requires extra memory for storing keys to be removed.
A Final Look
Removing elements from a Java map requires attention to detail, particularly when iterating over the entries. Use the approach that best suits your needs:
- Use
Iterator
when you want to remove elements during the iteration process. - Employ the
remove
method for known keys. - Take advantage of
removeIf
for clearer code from Java 8 onward. - Consider deferred removal when complexity needs to be minimized.
For further reading and a deeper understanding of Java Collections, check out Oracle's Java Collections Documentation.
By adhering to these practices, you will ensure that your map operations are both safe and efficient, ultimately leading to better performance in your Java applications.
Happy coding!