Overcoming Microservices Complexity in Real-Time Platforms
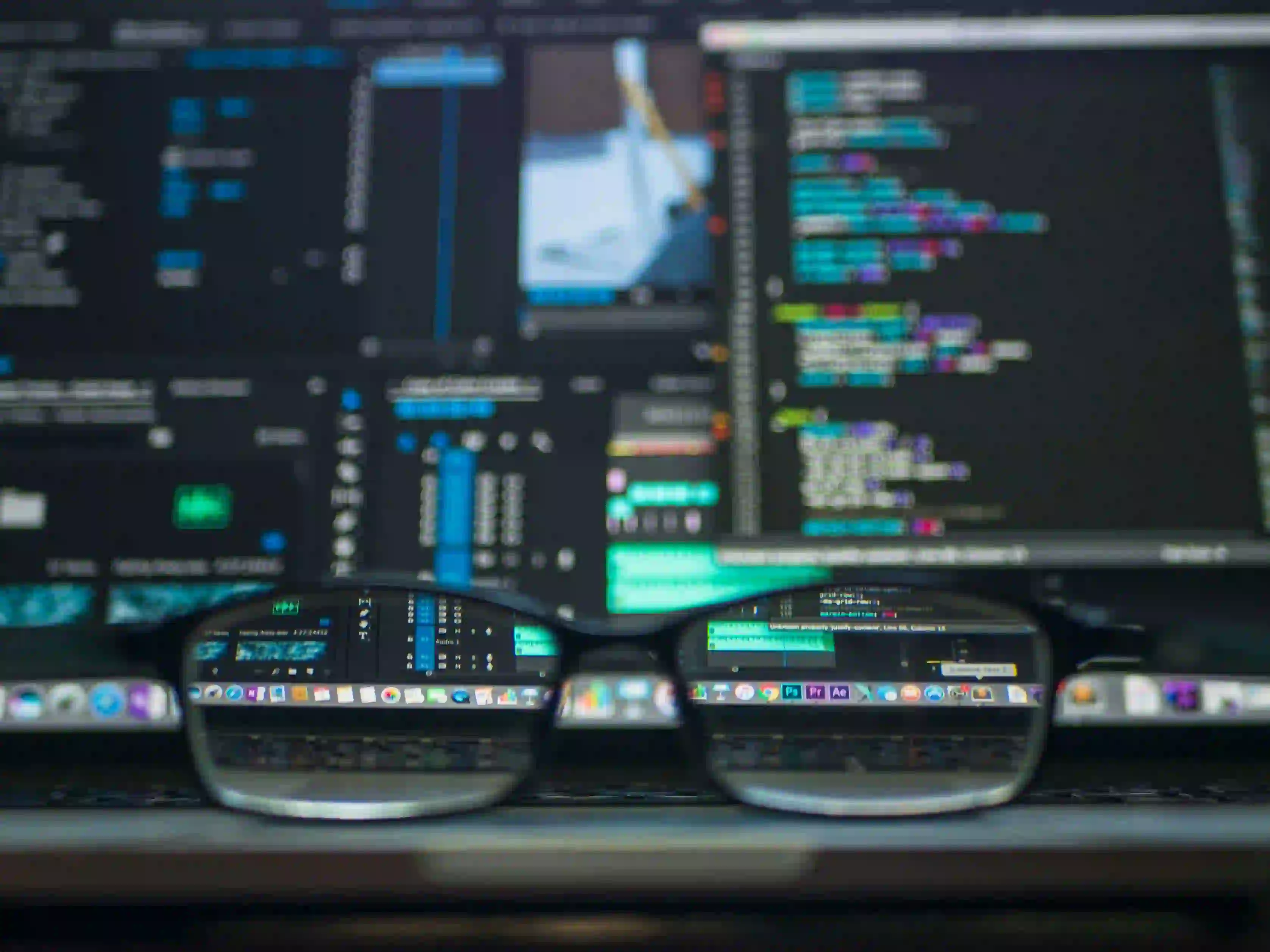
Overcoming Microservices Complexity in Real-Time Platforms
In recent years, microservices architecture has gained immense popularity among developers looking to build scalable and flexible applications. However, while microservices can simplify some aspects of system design, they can also add layers of complexity, particularly in real-time platforms. The challenges include inter-service communication, data consistency, and deployment difficulties. In this blog post, we will explore these challenges and provide practical strategies for overcoming them.
Understanding Microservices and Real-Time Systems
Microservices are small, independent services that communicate over a network. Each service represents a specific business capability and can be developed, deployed, and scaled independently.
Real-time systems, on the other hand, require immediate processing and action. Examples include online gaming, financial trading platforms, and IoT systems. The demand for real-time data processing can significantly complicate the microservices landscape.
Why Microservices?
Microservices offer several advantages, including:
- Scalability: Each service can be scaled independently based on demand.
- Flexibility: Developers can use various technologies for different services.
- Resilience: A failure in one service does not affect the entire system.
However, the benefits come with significant challenges, particularly in real-time applications.
Key Challenges with Microservices in Real-Time Platforms
-
Latency: Microservices often involve multiple network calls. This can lead to increased latency, which is unacceptable in a real-time context.
-
Data Consistency: In a distributed architecture, maintaining data consistency across services can be complicated.
-
Complex Deployments: Coordinating deployments of multiple services can be complex. Any misalignment can lead to system failures.
-
Monitoring and Debugging: Identifying the source of bottlenecks or failures across multiple services can be challenging.
-
Transaction Management: Handling transactions that span multiple services requires careful design to ensure success or rollback.
Given these challenges, how can we effectively manage microservices in a real-time environment?
Strategies to Overcome Microservices Complexity
1. Optimize Communication Patterns
Microservices communicate over a network, which can introduce latency. To optimize this, consider the following:
-
Asynchronous Communication: Use message brokers like Kafka or RabbitMQ to facilitate asynchronous communication. This allows services to send messages without waiting for an immediate response.
☕snippet.java// Example of sending an asynchronous message using Kafka Producer<String, String> producer = new KafkaProducer<>(props); producer.send(new ProducerRecord<>("my-topic", "key", "value"), (metadata, exception) -> { if (exception != null) { // Handle exception System.err.println("Error sending message: " + exception.getMessage()); } else { System.out.printf("Message sent to topic %s partition %d%n", metadata.topic(), metadata.partition()); } });
-
Service Mesh: Implement a service mesh (e.g., Istio or Linkerd) to manage service-to-service communication. This allows for better traffic management, including retries, load balancing, and circuit breaking.
2. Implement Event Sourcing and CQRS
Event Sourcing can improve data consistency in microservices. Instead of directly modifying the database, services emit events that represent state changes. This provides a log of actions taken in the system.
Command Query Responsibility Segregation (CQRS) can be combined with event sourcing to separate read and write operations:
- The command side handles actions that update the state.
- The query side deals with fetching data without affecting the system's state.
By using these patterns, you can build a robust real-time platform that maintains consistency while allowing for scalability.
3. Simplify Deployment with CI/CD
Continuous Integration and Continuous Deployment (CI/CD) pipelines can help automate the deployment process. Tools like Jenkins, GitLab CI, or AWS CodePipeline can facilitate seamless deployment across multiple services. Here’s a simple CI/CD pipeline example using GitHub Actions:
name: CI/CD Pipeline
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout Code
uses: actions/checkout@v2
- name: Build with Maven
run: mvn clean install
- name: Deploy to Kubernetes
run: kubectl apply -f k8s/deployment.yaml
Using CI/CD pipelines reduces the complexity of managing multiple deployments and enables you to roll back changes easily if necessary.
4. Monitoring and Observability
To effectively monitor and debug microservices, implement an observability strategy using tools like Prometheus, Grafana, or ELK Stack (Elasticsearch, Logstash, and Kibana). This will help you visualize metrics, logs, and traces across services.
For example, using Spring Boot and Spring Cloud Sleuth, you can automatically add trace IDs to logs for better tracking:
@RestController
public class MyController {
@GetMapping("/api/resource")
public String getResource() {
// The trace ID will be added to logs automatically by Sleuth
return "Resource data";
}
}
Having a robust monitoring system allows you to trace issues quickly, reducing downtime in critical real-time applications.
5. Implement Distributed Transactions
Managing transactions that span multiple microservices can be complex. Consider using the Saga pattern, which involves breaking down a transaction into a series of smaller, manageable transactions.
For instance, when creating an order, you might first reserve stock in one service, then charge a payment in another. If one service fails, you can execute compensating transactions in others to rollback.
Choreography vs. Orchestration: You can implement Sagas using either choreography (where each service communicates directly) or orchestration (a centralized service manages the entire process). Choose the method that best fits your platform's architecture.
The Closing Argument
While microservices offer great scalability and flexibility, integrating them into real-time platforms brings significant complexity. By adopting optimized communication patterns, event sourcing, CI/CD pipelines, observability, and managing distributed transactions effectively, you can overcome these challenges.
Microservices can enable you to build responsive, resilient, and highly agile systems if approached wisely. As the trend continues to grow, staying informed about best practices can help you effectively harness their power in real-time applications.
For further reading, check out the following resources:
- Microservices Architecture
- Event Sourcing with CQRS
- Service Mesh Overview
Explore these strategies and enhance your microservices architecture to meet the challenges of real-time platforms. Happy coding!