Overcoming Common Java Project Challenges for Beginners
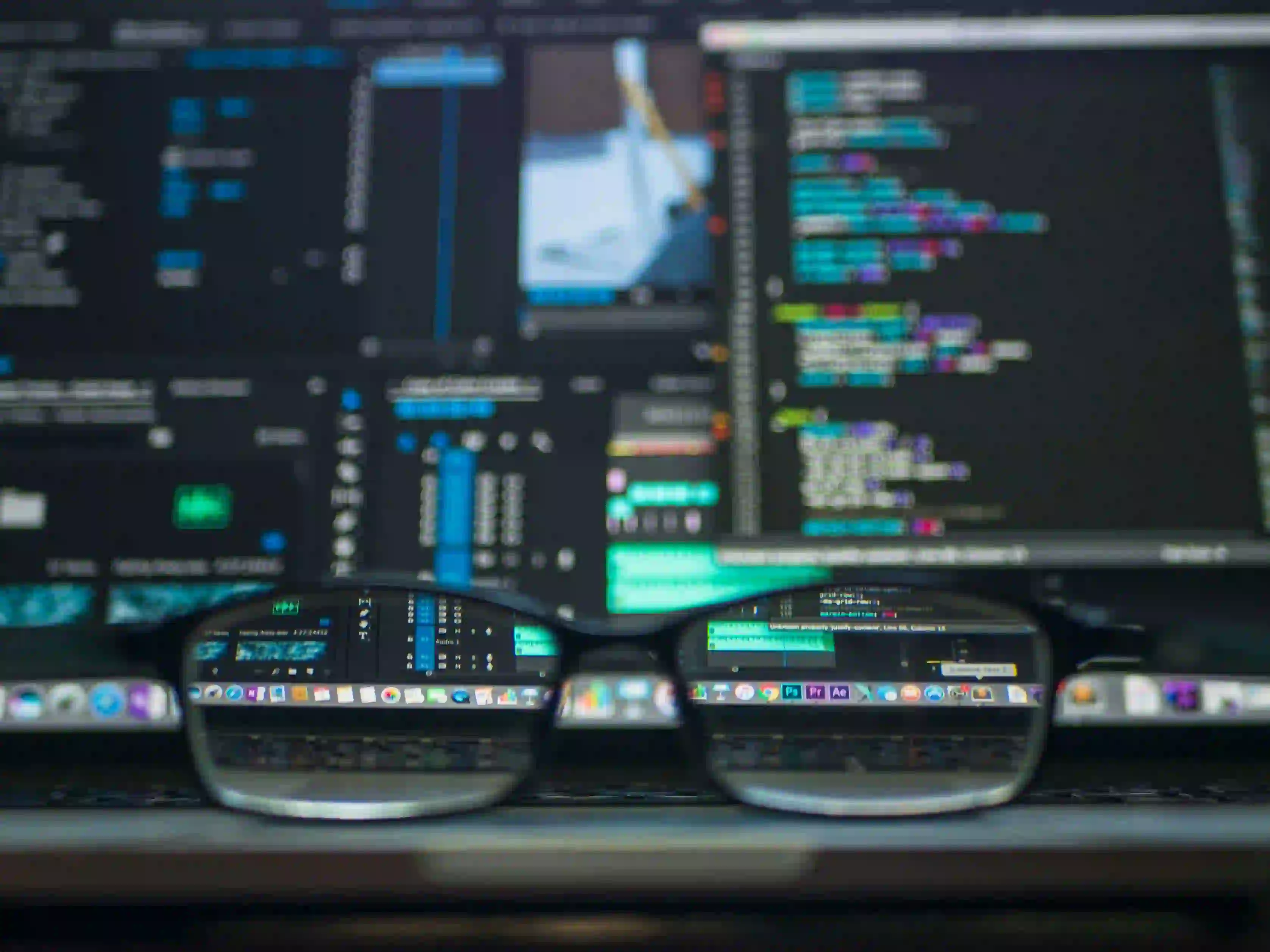
Overcoming Common Java Project Challenges for Beginners
Java is often recommended for beginners due to its simplicity and extensive community support. However, as with any programming language, new Java developers can encounter several challenges when embarking on their first projects. In this blog post, we'll delve into some of these common challenges and provide effective strategies for overcoming them.
Understanding Java Basics
Before diving into project-specific challenges, it's vital to grasp the fundamentals of Java. Knowing how to effectively use classes, objects, interfaces, and exception handling can significantly ease the learning curve. If you need a refresher, consider checking out the Java Tutorials from Oracle.
Key Java Concepts to Familiarize Yourself With
-
Object-Oriented Programming (OOP): Java is an object-oriented language, which means understanding concepts such as inheritance, encapsulation, and polymorphism is essential.
-
Data Types and Control Structures: Knowing the difference between primitive types and objects, as well as control flow structures (if, switch, loops), provides a strong foundation.
-
Collections Framework: Familiarity with Java's Collections Framework, including Lists, Sets, and Maps, is crucial for managing groups of objects efficiently.
Challenge 1: Setting up the Development Environment
Solution: Use IDEs like IntelliJ IDEA or Eclipse
Setting up a Java development environment can be daunting. However, using Integrated Development Environments (IDEs) like IntelliJ IDEA or Eclipse can greatly simplify this process. These environments come with built-in tools that facilitate coding, debugging, and project management.
Here is a brief setup guide for IntelliJ IDEA:
- Download IntelliJ IDEA: Visit the JetBrains website and download the Community edition.
- Install the JDK: Ensure you have the Java Development Kit (JDK) installed. You can download it from the Oracle official site or use OpenJDK.
After installing, you can start a new project by:
File > New Project > Java
Challenge 2: Understanding Java Syntax and Semantics
Solution: Practice Coding Regularly
Java syntax can seem verbose for those used to languages like Python. New developers often struggle with semicolons, curly braces, and data types. The best approach to mastering this is continuous practice.
Consider the following simple Java program:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Why this code?
- Public Class: Defines a class called HelloWorld.
- Main Method: The
main
method serves as the entry point for any Java application. - Print Statement:
System.out.println
outputs the string to the console.
By regularly writing simple Java applications, you'll become more comfortable with the syntax.
Challenge 3: Debugging Issues
Solution: Master Debugging Techniques
Every developer faces bugs. However, understanding how to debug effectively is what distinguishes a proficient programmer from the rest.
-
Using Debugger Tools: IDEs like IntelliJ and Eclipse provide built-in debugging tools. Learn how to set breakpoints, step through the code, and inspect variable values.
-
Reading Stack Traces: When exceptions occur, Java generates stack traces. Familiarize yourself with reading these to diagnose issues.
Consider the following code:
public class MathUtils {
public static int divide(int numerator, int denominator) {
return numerator / denominator;
}
public static void main(String[] args) {
System.out.println(divide(10, 0));
}
}
The error: This will throw an ArithmeticException
when attempting to divide by zero. Understanding stack traces will guide you in resolving such errors effectively.
Challenge 4: Working with External Libraries
Solution: Leverage Maven or Gradle
When starting your first Java project, you may find a need for external libraries. Handling these dependencies manually can be tedious, but tools like Maven or Gradle can automate this process.
Maven Example:
-
Create a Maven Project:
- Use the command line or your chosen IDE to create a new Maven project.
-
Add Dependencies: Open your
pom.xml
file and add necessary libraries:
<dependencies>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.12.0</version>
</dependency>
</dependencies>
Why Maven?
Maven resolves dependencies automatically and ensures that your project has everything it needs to compile and run.
Challenge 5: Understanding Java Exceptions
Solution: Learn to Handle Exceptions Properly
One challenge that new developers face is dealing with exceptions. Java provides a robust mechanism for error handling, but it can be overwhelming at first.
To effectively handle exceptions, follow these best practices:
- Try-Catch Blocks: Use try-catch blocks to gracefully recover from errors.
public class ExceptionDemo {
public static void main(String[] args) {
try {
System.out.println(divide(10, 0));
} catch (ArithmeticException e) {
System.out.println("Cannot divide by zero.");
}
}
}
Why handle exceptions?
Handling exceptions allows your program to run smoothly without crashing, providing a better user experience.
Challenge 6: Version Control
Solution: Utilize Git
Managing project files can be overwhelming. Implementing version control systems like Git can simplify this process significantly.
- Install Git: Download and install Git from the official site.
- Initialize a Git Repository:
git init my-java-project
cd my-java-project
- Add files: Use
git add
andgit commit
commands to track your changes.
Why use Git?
Git helps you manage file changes, collaborate with others, and revert to previous versions of your code, thereby making your project management more organized.
Challenge 7: Best Practices and Design Patterns
Solution: Learn and Implement Best Practices
One of the most effective ways to avoid common pitfalls is to learn best practices and design patterns. This enhances your code quality and maintainability.
-
Code Quality: Write clean, readable code by following naming conventions and keeping functions focused.
-
Design Patterns: Familiarize yourself with design patterns such as Singleton, Factory, and Observer. These patterns provide tested solutions to common problems.
To Wrap Things Up
Embarking on your first Java project can undoubtedly be a challenging experience. However, by knowing the common pitfalls and understanding the solutions, you can streamline the learning process significantly.
Remember to set up your environment properly, debug effectively, and utilize version control systems. With practice and patience, you will find yourself overcoming these challenges and building robust Java applications.
For a deeper dive into Java programming, check out resources like GeeksforGeeks and Java Code Geeks, which provide a wealth of knowledge on various Java topics.
Happy coding!