Common Pitfalls When Using Spring Quartz with JavaMail
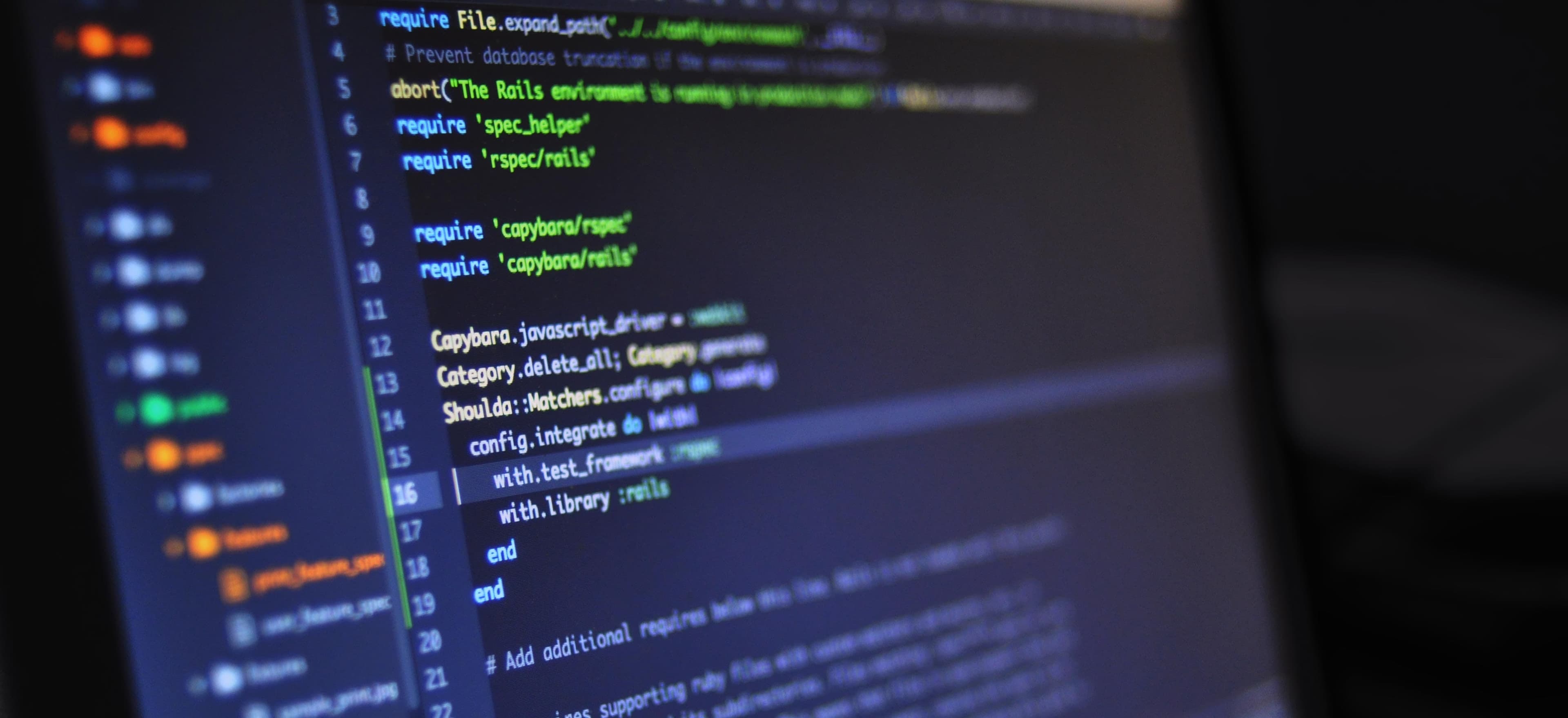
- Published on
Common Pitfalls When Using Spring Quartz with JavaMail
When building Java applications, integrating scheduling tasks and email functionalities can be challenging. Spring's Quartz, a powerful scheduling library, combined with JavaMail, enables developers to send emails at specified intervals or based on specific events. However, there are common pitfalls that developers encounter when using these two powerful features together. In this blog post, we will dive into some common issues, ways to avoid them, and best practices for using Spring Quartz with JavaMail effectively.
What is Spring Quartz?
Spring Quartz is a module in the Spring framework that allows developers to easily schedule tasks in a Spring application. It provides a robust way to schedule jobs, allowing for various configurations, including cron-style expressions.
Example of a Simple Quartz Job
To get started, let’s create a simple Quartz job that sends an email. Here’s a Java class for our job using Spring:
import org.quartz.Job;
import org.quartz.JobExecutionContext;
import org.quartz.JobExecutionException;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.mail.SimpleMailMessage;
import org.springframework.mail.javamail.JavaMailSender;
public class EmailJob implements Job {
@Autowired
private JavaMailSender emailSender;
@Override
public void execute(JobExecutionContext context) throws JobExecutionException {
sendEmail();
}
private void sendEmail() {
SimpleMailMessage message = new SimpleMailMessage();
message.setTo("recipient@example.com");
message.setSubject("Scheduled Email");
message.setText("This is a scheduled email sent using Spring Quartz and JavaMail.");
emailSender.send(message);
}
}
Why Use This Structure?
- Separation of Concerns: The job focuses only on the email-sending logic. It keeps your application modular and easy to test.
- Dependency Injection: Utilizing
@Autowired
means Spring handles the lifecycle of theJavaMailSender
bean, making your code cleaner.
Common Pitfalls
1. Configuration Issues
Problem
Failing to configure the Quartz Scheduler correctly can lead to jobs not running at all.
Solution
Make sure to register your Quartz Scheduler in your Spring configuration. Below is an example configuration:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.scheduling.annotation.EnableScheduling;
import org.springframework.scheduling.quartz.SchedulerFactoryBean;
@Configuration
@EnableScheduling
public class QuartzConfig {
@Bean
public SchedulerFactoryBean schedulerFactoryBean() {
SchedulerFactoryBean factoryBean = new SchedulerFactoryBean();
// additional customizations here
return factoryBean;
}
}
2. Job Execution Context
Problem
Sometimes, developers forget to manage the JobExecutionContext. This context is crucial for passing data between jobs.
Solution
You can store data in the context using the JobDataMap
. For instance:
@Override
public void execute(JobExecutionContext context) throws JobExecutionException {
String emailToSend = context.getMergedJobDataMap().getString("emailToSend");
// use emailToSend variable accordingly
}
This way, you can customize the execution based on the context.
3. Email Throttling
Problem
Sending too many emails in a short period can cause your application to be rate-limited by email servers.
Solution
Implementing a throttling mechanism is important. Instead of sending email directly in your job, consider queuing them and sending them in batches.
4. Exception Handling
Problem
Not handling exceptions adequately can lead to job failures and potential loss of critical information.
Solution
Use try-catch blocks and consider implementing logging for exceptions:
@Override
public void execute(JobExecutionContext context) throws JobExecutionException {
try {
sendEmail();
} catch (Exception e) {
// Log the error properly
System.err.println("Email sending failed: " + e.getMessage());
throw new JobExecutionException(e);
}
}
5. Use Cron Expressions Wisely
Problem
Incorrect cron expressions can lead to jobs not executing at the expected intervals.
Solution
Double-check your cron expressions. Here’s a quick reference for cron expressions:
0 0 12 * * ?
= Every day at noon0 15 10 ? * *
= Every day at 10:15 AM
Testing cron expressions can be done using external tools or local environments to avoid mistakes.
Best Practices
Configure Retry Mechanisms
It's beneficial to configure retry mechanisms for your email-sending jobs. This way, if an email fails while sending, it can retry a set number of times.
property.put("retry.count", "3"); // Maximum retry count can be configured
Use Email Templates
For professional applications, avoiding hardcoding email content is wise. Email templates can be managed externally or in resources, making it easier to adjust the content without modifying code.
Using frameworks like Thymeleaf can improve maintainability.
Document Scheduling Logic
When you have multiple scheduled jobs, keep a documentation of their purposes, cron expressions, and the impact they have on the system. Clear documentation ensures that future developers can understand and maintain the scheduling logic easily.
Test Locally Before Production Deployment
Always test your Quartz jobs locally before deploying them to production. This includes both unit testing and integration testing. Writing unit tests for your Quartz jobs can help catch potential issues early.
Bringing It All Together
Using Spring Quartz with JavaMail offers a powerful way to manage scheduled email sending in your Java applications. However, as we've discussed, it is essential to be aware of common pitfalls, such as configuration mishaps, improper handling of the execution context, and failure management.
By adhering to best practices like using retry mechanisms, implementing email templates, and documenting your scheduling logic, you can make your application more reliable and easy to manage.
For more information on Spring Quartz, consider visiting the Spring Documentation, and for JavaMail best practices, check out the JavaMail API Documentation.
By mastering these components, you can significantly enhance the functionality and reliability of your applications.
Happy coding!
Checkout our other articles