Common Pitfalls in Using Parameterized Tests with JUnit
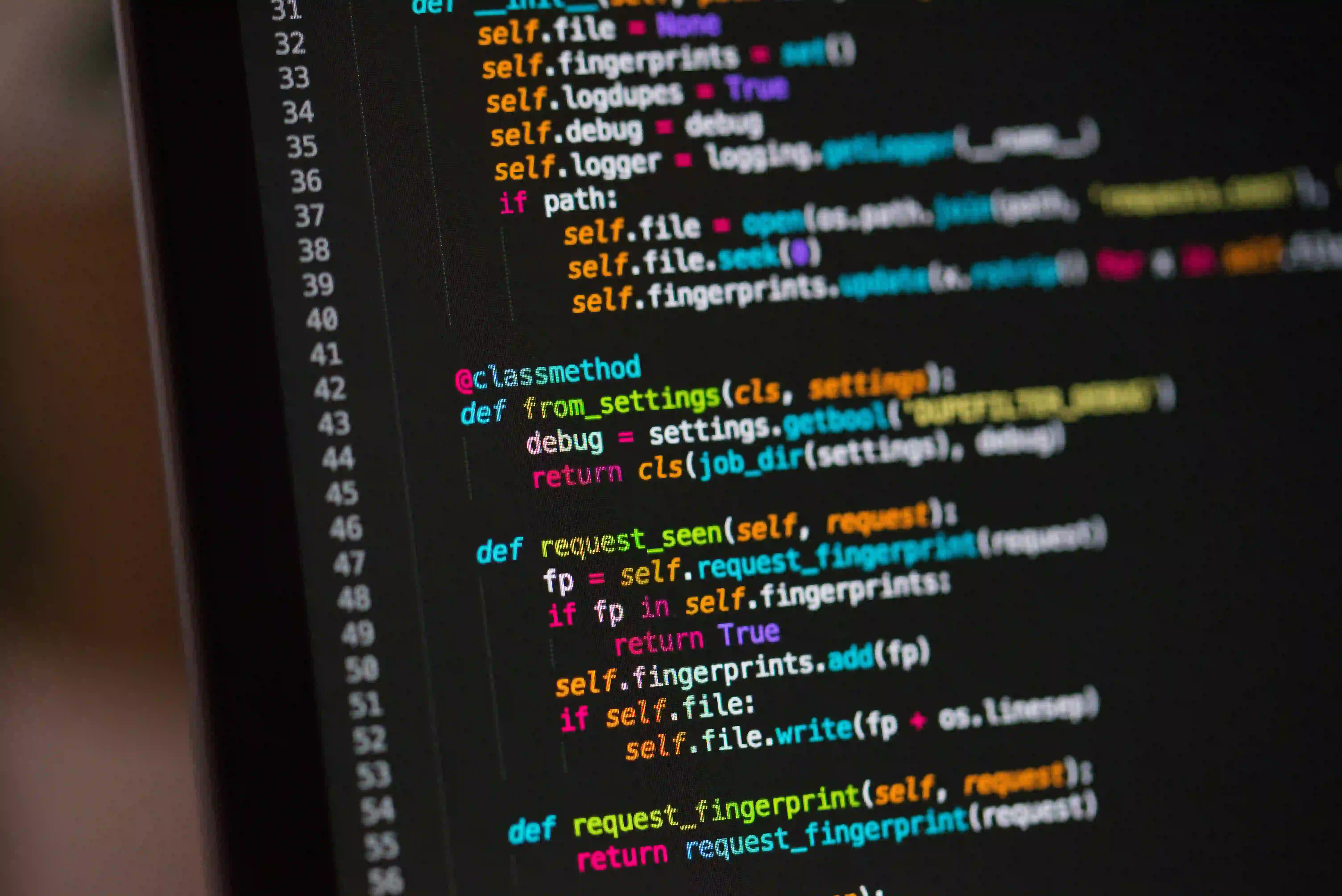
Common Pitfalls in Using Parameterized Tests with JUnit
JUnit has become the de facto standard for unit testing in Java. One of its powerful features is the ability to perform parameterized tests, which allows developers to run the same test with different inputs. This not only streamlines the testing process but also increases code reusability. However, while parameterized tests can be highly beneficial, they also come with their own set of pitfalls if not handled correctly.
In this blog post, we’ll discuss common pitfalls encountered when using parameterized tests in JUnit and how to avoid them, ensuring that your tests remain clean, efficient, and effective.
What are Parameterized Tests?
Parameterized tests allow you to run the same test multiple times with different data. Rather than writing multiple test methods (which can be verbose and repetitive), you can define a single test that takes a set of parameters.
Example
Here’s a simple example of using parameterized tests in JUnit:
import org.junit.Test;
import org.junit.runner.RunWith;
import org.junit.runners.Parameterized;
import java.util.Arrays;
import java.util.Collection;
@RunWith(Parameterized.class)
public class CalculatorTest {
private int a;
private int b;
private int expected;
public CalculatorTest(int a, int b, int expected) {
this.a = a;
this.b = b;
this.expected = expected;
}
@Parameterized.Parameters
public static Collection<Object[]> data() {
return Arrays.asList(new Object[][] {
{ 1, 1, 2 },
{ 2, 3, 5 },
{ 5, 5, 10 }
});
}
@Test
public void testAdd() {
Calculator calculator = new Calculator();
assertEquals(expected, calculator.add(a, b));
}
}
In the above code, we define a CalculatorTest
class that runs the testAdd
method multiple times with different sets of parameters. This approach makes it clearer and easier to maintain.
Common Pitfalls
Despite the benefits of parameterized tests, there are several pitfalls that can hinder their effectiveness. Let’s dive into some of these common issues:
1. Lack of Clarity
Issue
One common pitfall is sacrificing clarity for brevity. When tests are overly compact or too many parameters are included, the intent of the test can become obscured.
Solution
Keep It Simple: Use descriptive parameter names and maintain simplicity in your parameters. Avoid overwhelming the reader with too much data. For example:
@Parameterized.Parameters
public static Collection<Object[]> data() {
return Arrays.asList(new Object[][] {
// {input1, input2, expectedOutput}
{ 1, 1, 2 },
{ 2, 3, 5 },
{ 5, 5, 10 }
});
}
Here, even though the data set is brief, it tells us precisely what the inputs and expectations are.
2. Poor Test Data Management
Issue
Using hard-coded data can lead to repetitive code, making it difficult to update test cases. If you want to add or modify test cases, you may find it tedious to edit the source code frequently.
Solution
Externalize Test Data: Consider using external files or databases to manage test data. This way, you can easily change test inputs without modifying the code. Libraries like JUnitParams can help with this.
3. Incorrect Assumptions About Input Data
Issue
Another common pitfall is assuming that all tested inputs will behave the same way. This misguidance can result in tests that pass when they should fail and vice versa if the test logic does not account for edge cases.
Solution
Thoroughly Analyze Your Inputs: Always include edge cases in your data sets. For instance, tests should account for negative values, zeros, and large numbers.
public static Collection<Object[]> data() {
return Arrays.asList(new Object[][] {
{ 1, 1, 2 },
{ -1, -1, -2 }, // Edge case
{ 0, 0, 0 }, // Edge case
{ Integer.MAX_VALUE, 0, Integer.MAX_VALUE } // Edge case
});
}
4. Making Tests Too Complex
Issue
As your testing needs grow, you might find yourself trying to combine too many types of inputs into a single parameterized test. This can lead to complicated test methods that are difficult to read and maintain.
Solution
Break Down Complex Tests: Ensure each test remains focused on a specific case. If a test starts to feel complex, analyze whether it could be split into multiple tests. Each test should validate a single behavior.
5. Not Utilizing Descriptive Names for Tests
Issue
By default, JUnit names test methods in a generic way. When running parameterized tests, the problem compounds because the test name may not describe the scenario effectively.
Solution
Use Custom Method Names: Use the @Rule
annotation with the TestName
class to dynamically create recognizable test names. Not only does this aid in debugging, but it also helps other developers understand what each test does at a glance.
import org.junit.Rule;
import org.junit.rules.TestName;
public class CalculatorTest {
@Rule
public TestName testName = new TestName();
@Test
public void testAdd() {
System.out.println("Running test: " + testName.getMethodName() +
" with parameters " + a + ", " + b + " expecting " + expected);
assertEquals(expected, calculator.add(a, b));
}
}
6. Not Isolating Tests
Issue
When you use stateful tests that rely on external resources (like databases or files), other tests might unintentionally affect your parameterized test, leading to inaccurate results.
Solution
Separate Tests in Isolation: Ensure that each test runs in a clean environment. Utilize the @Before
and @After
annotations to set up and teardown any necessary states.
@Before
public void setUp() {
calculator = new Calculator(); // Always start with a fresh Calculator instance.
}
@After
public void tearDown() {
// Clean up resources if necessary.
}
Key Takeaways
Parameterized tests in JUnit can be an exceptionally potent tool for ensuring your code behaves as expected across various scenarios. Nevertheless, to harness their full potential, you must be wary of common pitfalls. By ensuring clarity, properly managing test data, analyzing assumptions, limiting complexity, providing descriptive names, and keeping tests isolated, you can write effective parameterized tests that make your codebase robust.
Would you like to learn more about parameterized tests in JUnit? Explore the official JUnit documentation for in-depth examples and guidelines. And if you want to dive deeper into Java testing methodologies, you can find valuable insights on Baeldung's Java Testing Guide as well.
Happy testing!