Troubleshooting Arquillian Integration in Java EE Projects
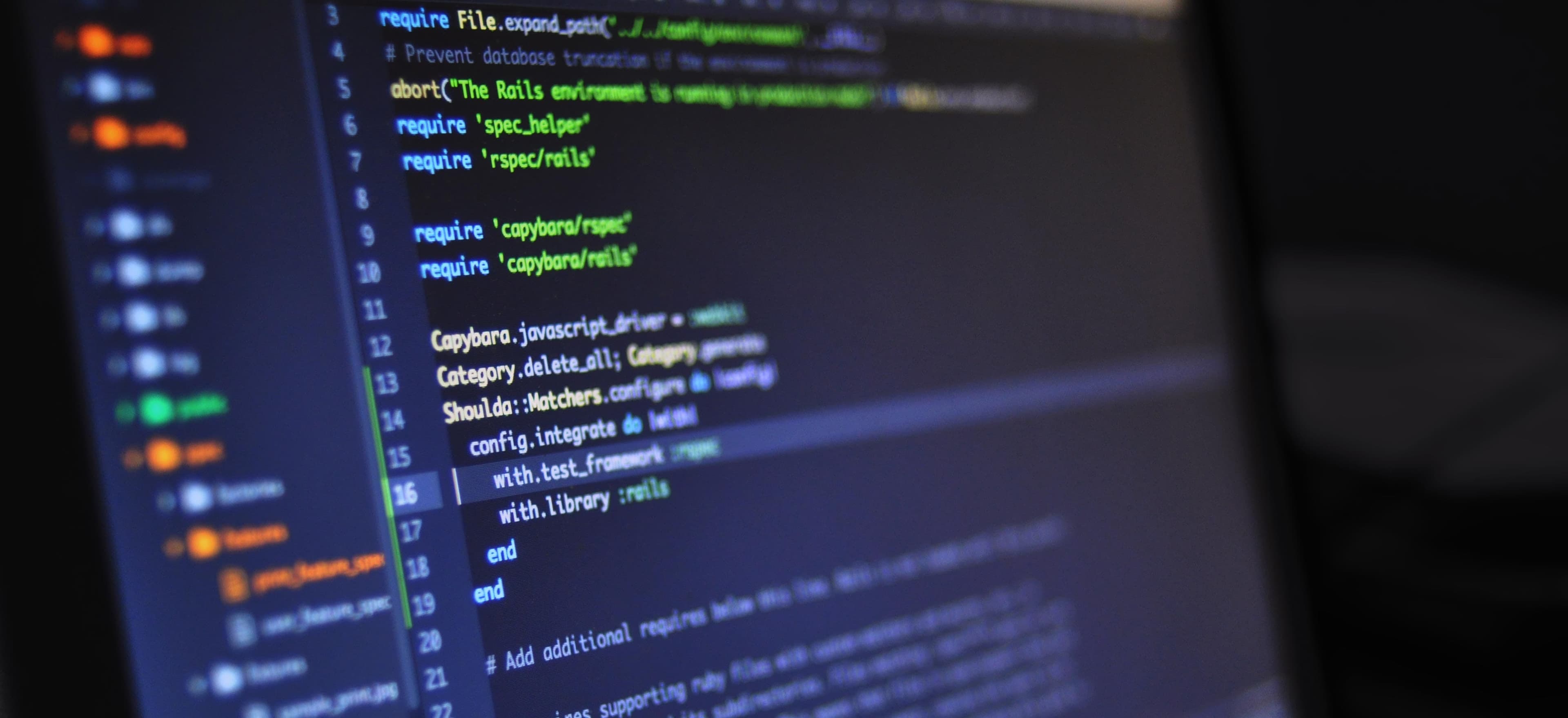
- Published on
Troubleshooting Arquillian Integration in Java EE Projects
When it comes to testing Java EE applications, Arquillian stands out as a powerful framework that simplifies the integration testing process. However, even seasoned developers may face challenges in setting it up and making it work as intended. In this comprehensive guide, we will explore common issues that arise during Arquillian integration and offer practical solutions to help you troubleshoot effectively.
What is Arquillian?
Arquillian is an open-source testing framework that allows you to run your tests inside the container where your application runs. This means you can use the same environment for your tests as you do in production, resulting in more accurate verifications of your application's behavior.
Benefits of using Arquillian
- Container-managed testing: Reduces discrepancies between test and production environments.
- Simplicity: Simple API for testing various Java EE technologies.
- Integration: Works seamlessly with popular build tools like Maven and Gradle.
Getting Started with Arquillian
Before diving into troubleshooting, it’s vital to ensure that you have Arquillian set up correctly. Generally, you will need:
- An application server or container (like WildFly, Payara).
- Dependencies for Arquillian in your project's
pom.xml
orbuild.gradle
.
Sample Maven Configuration
Below is an example of a Maven configuration for Arquillian, which will help validate if your setup is correct.
<dependency>
<groupId>org.jboss.arquillian.junit</groupId>
<artifactId>arquillian-junit-container</artifactId>
<version>1.8.0.Final</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.jboss.arquillian.container</groupId>
<artifactId>arquillian-wildfly-embedded-1.0</artifactId>
<version>1.0.0.Final</version>
<scope>test</scope>
</dependency>
Ensure you update the versions based on the latest releases to benefit from improvements and bug fixes.
Common Issues and Troubleshooting Steps
Now that you have Arquillian set up, let's dive into some common issues you might encounter:
1. Dependency Conflicts
Issue: One of the first hurdles developers face is dependency conflicts, especially if you're using multiple libraries.
Solution: Use the mvn dependency:tree
command to identify conflicting dependencies. Once you do so, you might want to exclude specific transitive dependencies to solve the conflict.
Example exclusion in pom.xml
:
<dependency>
<groupId>com.example</groupId>
<artifactId>conflicting-lib</artifactId>
<exclusions>
<exclusion>
<groupId>conflict-group</groupId>
<artifactId>conflict-artifact</artifactId>
</exclusion>
</exclusions>
</dependency>
2. Misconfigured Arquillian Container
Issue: Another common error occurs when the Arquillian container is not configured correctly, which might lead to runtime errors or tests not being executed.
Solution: Check your arquillian.xml
configuration file.
Example arquillian.xml
for WildFly:
<arquillian>
<container qualifier="wildfly" default="true">
<configuration>
<property name="jboss.home.dir">/path/to/wildfly</property>
</configuration>
</container>
</arquillian>
Make sure the jboss.home.dir
points to the correct directory. Also, ensure the qualifier
matches your container definitions.
3. Testing Class Not Found
Issue: Getting an error about the testing class not being found can indicate issues with package structures or misnamed classes.
Solution: Confirm the package structure of your tests. If you have a class named MyTest
, verify it's in the right folder corresponding to its package syntax:
src/test/java/com/example/MyTest.java
Also, make sure your test classes are public and have a proper constructor.
4. Deployment Errors
Issue: Deployment errors can occur if your application module is misconfigured or if required resources are not found.
Solution: Check the methods annotated with @Deployment
. A typical deployment method looks like this:
@Deployment
public static Archive<?> createDeployment() {
return ShrinkWrap.create(WebArchive.class, "test.war")
.addClasses(MyBean.class)
.addAsWebInfResource(EmptyAsset.INSTANCE, "beans.xml");
}
Ensure that all classes are correctly referenced. Dependency injection issues can also be attributed to missing beans.xml
.
5. Runtime Exceptions or Timeouts
Issue: Encountering runtime exceptions or timeouts during tests is a sign your business logic might have flaws, or external dependencies are acting up.
Solution: Use logging and breakpoints to diagnose issues. Implement retries and gradually increase timeouts:
@Timeout(20)
public void testLongRunningOperation() {
// Test code...
}
Monitor for specific exceptions and errors in the server logs for additional context.
6. Environment-Specific Behaviors
Issue: Sometimes, tests pass locally but fail on CI/CD pipelines due to discrepancies between environments.
Solution: Ensure the container on your CI/CD matches your local setup as closely as possible. Verify Java versions, configuration files, and container settings.
In Conclusion, Here is What Matters and Additional Resources
Troubleshooting Arquillian can be challenging but is often manageable with a systematic approach. Errors can often be broken down into steps, allowing for incremental debugging to identify the root causes.
If you experience ongoing issues, take advantage of the community and resources. Refer back to the Arquillian Documentation and community forums for further insights and assistance.
Integrating Arquillian into your Java EE testing framework can significantly enhance test reliability and provide insights that manual testing could overlook. With proper configuration and knowledge of troubleshooting steps, you can streamline your development workflow and achieve better software quality.
By employing these strategies, you’ll be well-prepared to tackle any Arquillian integration issues that may arise in your Java EE projects, ensuring successful deployment and high-quality application performance.