Common Pitfalls for Java Developers in Elasticsearch Integration
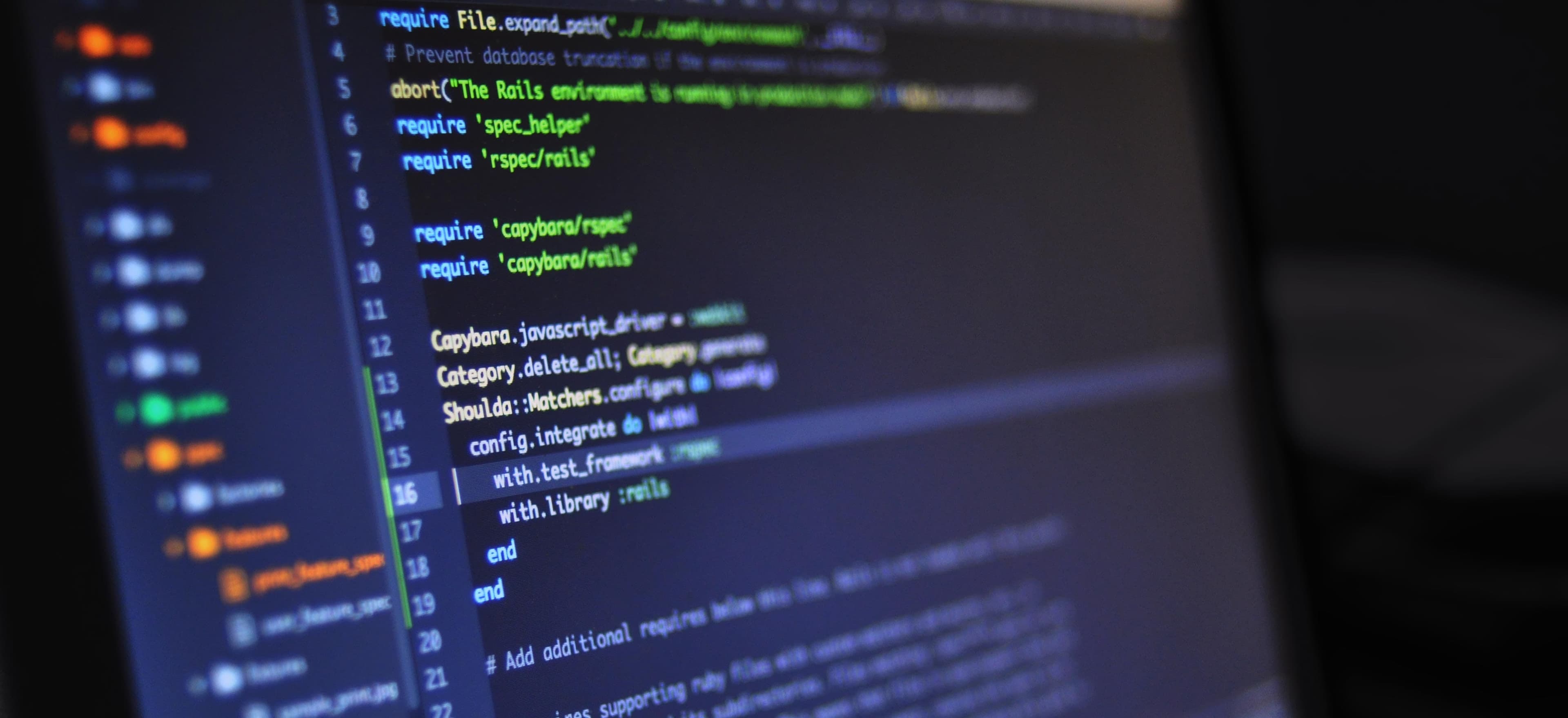
- Published on
Common Pitfalls for Java Developers in Elasticsearch Integration
Elasticsearch has become a go-to solution for applications requiring powerful search and analytical capabilities. For Java developers looking to integrate Elasticsearch into their applications, the process can be both rewarding and challenging. In this post, we will explore common pitfalls experienced by Java developers while integrating Elasticsearch, along with best practices to help you avoid these issues.
Understanding Elasticsearch and Its Client
Before diving into the pitfalls, let’s briefly recap what Elasticsearch is and the significance of its Java client. Elasticsearch is a distributed, RESTful search and analytics engine capable of handling structured and unstructured data. The official Java client library facilitates seamless interaction between Java applications and an Elasticsearch cluster.
To get started, ensure you include the Elasticsearch Java client library in your Maven pom.xml
:
<dependency>
<groupId>org.elasticsearch.client</groupId>
<artifactId>elasticsearch-rest-high-level-client</artifactId>
<version>8.2.2</version> <!-- Use the version appropriate for your needs -->
</dependency>
Common Pitfalls
1. Ignoring Connection Management
One of the most prominent mistakes is improper connection management. Many developers instantiate new client connections for each request, leading to exhaustion of resources and performance bottlenecks.
Solution: Use a singleton pattern for your Elasticsearch client. Here’s a simple implementation:
import org.elasticsearch.client.RestHighLevelClient;
import org.elasticsearch.client.RestClient;
import org.elasticsearch.client.RestClientBuilder;
public class ElasticsearchClientFactory {
private static RestHighLevelClient client;
private ElasticsearchClientFactory() {}
public static RestHighLevelClient getClient() {
if (client == null) {
client = new RestHighLevelClient(
RestClient.builder(new HttpHost("localhost", 9200, "http"))
);
}
return client;
}
}
In this implementation, we ensure that only one instance of the RestHighLevelClient
is created and reused throughout the application. This approach fosters resource efficiency.
2. not Using Bulk API for Indexing
When dealing with a large volume of data, some developers index each document individually. This approach leads to increased load times and negatively impacts performance due to high network latency.
Solution: Employ the Bulk API to send multiple index requests in a single HTTP request. Here’s how to do this:
import org.elasticsearch.action.bulk.BulkRequest;
import org.elasticsearch.action.index.IndexRequest;
import org.elasticsearch.client.RequestOptions;
public void bulkIndexDocuments(List<YourDocument> documents) throws IOException {
BulkRequest bulkRequest = new BulkRequest();
for (YourDocument doc : documents) {
IndexRequest indexRequest = new IndexRequest("your_index")
.id(doc.getId().toString())
.source("field1", doc.getField1(), "field2", doc.getField2());
bulkRequest.add(indexRequest);
}
client.bulk(bulkRequest, RequestOptions.DEFAULT);
}
By using the Bulk API, the performance can significantly improve, especially in high-load scenarios.
3. Mishandling Exceptions
Elasticsearch throws various exceptions, which can lead to unhandled scenarios in the application. Ignoring these exceptions can lead to data inconsistencies or application crashes.
Solution: Adopt a comprehensive exception handling strategy tailored to catch potential Elasticsearch-specific exceptions. Here’s a sample:
try {
// Your Elasticsearch operation here
} catch (IOException e) {
// Handle connection errors, timeouts, etc.
logger.error("Connection error: {}", e.getMessage());
} catch (ElasticsearchException e) {
// Handle document indexing issues
logger.error("Elasticsearch error: {}", e.getDetailedMessage());
} catch (Exception e) {
logger.error("Unexpected error: {}", e.getMessage());
}
This pattern will allow you to handle various error scenarios gracefully, improving your application's robustness.
4. Poor Indexing Strategy
Another common pitfall is adopting a one-size-fits-all approach to indexing. Improper mapping of field types or not using the correct analyzers can lead to suboptimal performance and inaccurate search results.
Solution: Plan your index structure in advance. Understand the types of queries you will run, and design your index mappings accordingly. Here’s an example mapping:
PUT /your_index
{
"mappings": {
"properties": {
"name": {
"type": "text",
"analyzer": "standard"
},
"age": {
"type": "integer"
}
}
}
}
Ensure that your fields are defined with the appropriate types and settings. This can heavily impact both indexing performance and search accuracy.
5. Neglecting Efficient Queries
In many scenarios, developers fail to optimize their queries, either by sending insufficient queries or using too many resources on complex queries. This can slow down application response time significantly.
Solution: Always evaluate your queries. Leverage features like filters to narrow down your search results effectively. For example:
SearchRequest searchRequest = new SearchRequest("your_index");
SearchSourceBuilder searchSourceBuilder = new SearchSourceBuilder();
// Using filter to limit results
searchSourceBuilder.query(QueryBuilders.boolQuery()
.must(QueryBuilders.matchQuery("field1", "search term"))
.filter(QueryBuilders.rangeQuery("age").gte(18)));
searchRequest.source(searchSourceBuilder);
In this query, we combine both must and filter conditions, optimizing performance by limiting the number of documents examined.
6. Not Monitoring Cluster Health
Elasticsearch clusters can experience unplanned failures. Failing to monitor the health of your cluster leaves you vulnerable to downtime or data loss.
Solution: Utilize monitoring tools available in the Elasticsearch ecosystem or integrate APIs to check cluster health within your application:
ClusterHealthResponse healthResponse = client.cluster().health(request, RequestOptions.DEFAULT);
if (healthResponse.getStatus() != ClusterHealthStatus.GREEN) {
logger.warn("Cluster health is not optimal: {}", healthResponse.getStatus());
}
By routinely checking the cluster's health, you can proactively address issues before they escalate.
Key Takeaways
Integrating Elasticsearch into your Java applications can enhance functionality and provide critical search capabilities. However, being aware of common pitfalls is essential to ensuring a successful integration. From managing connections efficiently to implementing a proper indexing strategy, each step can contribute to the overall performance and scalability of your application.
Embrace these best practices, conduct thorough testing, and always stay informed about the latest updates and features in Elasticsearch. Happy coding!
For more insights on Elasticsearch and its client, check out the official documentation.
For further reading on Java development and integrations, feel free to explore other Java-related articles on best practices and common pitfalls.