Choosing Between EasyMock and Mockito: The Common Dilemma
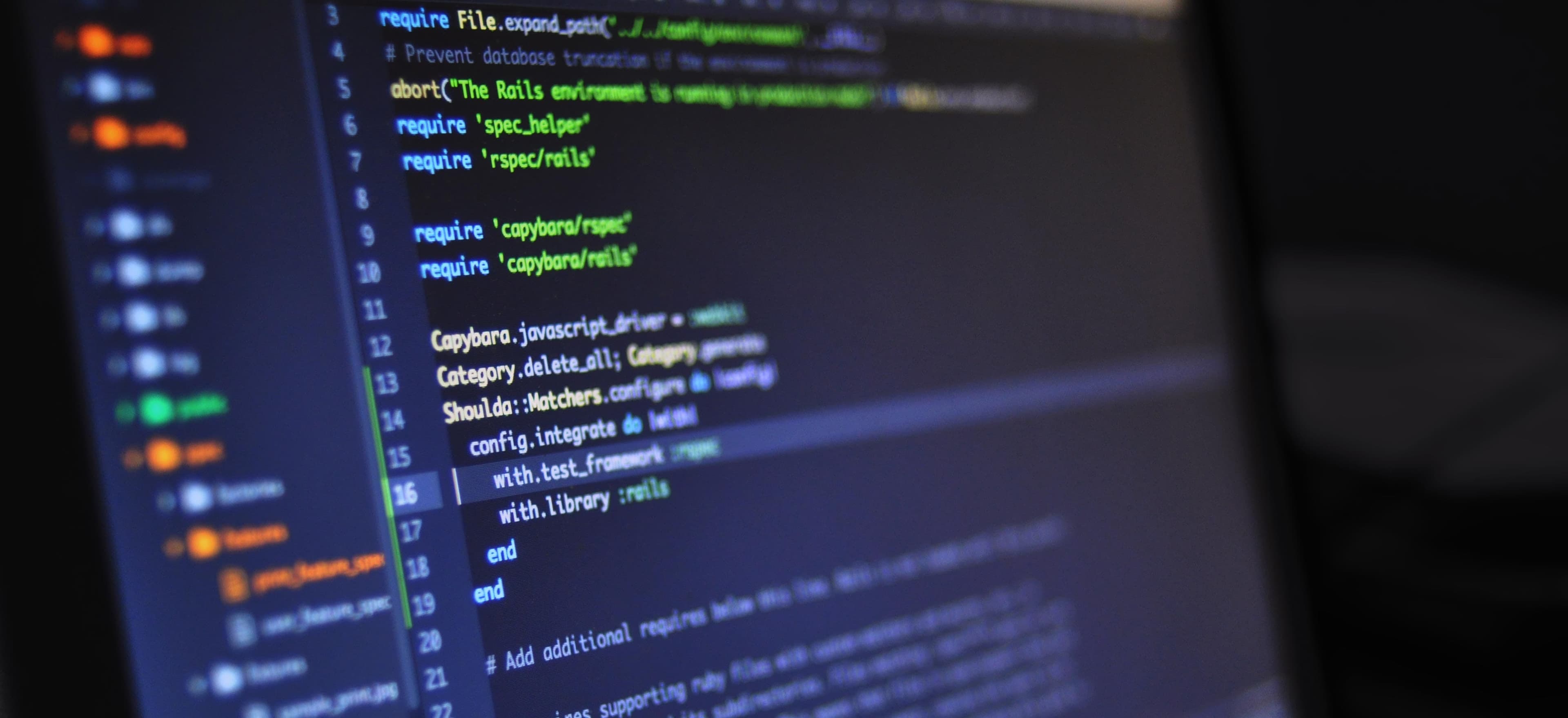
- Published on
Choosing Between EasyMock and Mockito: The Common Dilemma
When working with Java, unit testing is an essential practice that enhances code reliability. This is where mocking frameworks like EasyMock and Mockito come into play. Each tool has its own strengths and weaknesses, leading developers to face a common dilemma: which one should they choose?
In this blog post, we'll delve into both frameworks, examining their features, usability, and the contexts in which they excel. By the end, you will be better equipped to choose the right mocking framework for your next Java project.
Understanding Mocking Frameworks
Mocking frameworks allow developers to create 'mock' objects in unit tests. These mocks simulate the behavior of real objects, making it easier to isolate code under test. This is crucial when you're dealing with code that interacts with databases, web services, or even complex business logic.
What are EasyMock and Mockito?
-
EasyMock: Created in 2004, EasyMock focuses on creating mock objects using Java reflection to set expectations and validate interactions with mocks.
-
Mockito: Launched in 2007, Mockito has gained popularity due to its user-friendly syntax and powerful features. It allows the creation of mock objects and mocking of final classes and methods.
Key Differences Between EasyMock and Mockito
To make an informed decision, we need to compare various aspects of both frameworks:
1. Syntax and Usability
EasyMock Syntax
EasyMock relies heavily on the use of explicit expectations. This can make tests appear lengthy and harder to read at times.
import org.easymock.*;
import org.junit.*;
public class UserServiceTest {
private UserService userService;
private UserDAO userDAOMock;
@Before
public void setUp() {
userDAOMock = EasyMock.createMock(UserDAO.class);
userService = new UserService(userDAOMock);
}
@Test
public void testGetUser() {
EasyMock.expect(userDAOMock.findUserById(1)).andReturn(new User("John"));
EasyMock.replay(userDAOMock);
User user = userService.getUser(1);
assertEquals("John", user.getName());
EasyMock.verify(userDAOMock);
}
}
In this example, you see how EasyMock requires setting expectations up front, then switching to replay mode to test your function.
Mockito Syntax
Mockito uses a more natural, less verbose syntax, which leads to cleaner and more readable tests.
import org.junit.*;
import static org.mockito.Mockito.*;
public class UserServiceTest {
private UserService userService;
private UserDAO userDAOMock;
@Before
public void setUp() {
userDAOMock = mock(UserDAO.class);
userService = new UserService(userDAOMock);
}
@Test
public void testGetUser() {
when(userDAOMock.findUserById(1)).thenReturn(new User("John"));
User user = userService.getUser(1);
assertEquals("John", user.getName());
verify(userDAOMock).findUserById(1);
}
}
Mockito allows you to define the behavior of the mock directly inline, removing the need for explicit declaration of replay mode and making your tests less cluttered.
2. Mocking Final Classes and Methods
Another significant point of contention is the ability to mock final classes and methods. This is particularly important when dealing with legacy code, which is often littered with final classes.
- EasyMock does not support mocking final classes or methods.
- Mockito introduced support for final classes and methods starting from version 2.0. Thus, it provides far more flexibility when it comes to legacy systems.
3. Parameterized Tests
Parameterized tests allow developers to run the same test with different inputs.
- EasyMock requires additional configuration to facilitate this feature.
- Mockito integrates seamlessly with JUnit and other testing frameworks to simplify the setup for parameterized tests.
4. Stubbing and Verification
Stubbing or setting mock behavior can be done differently in both frameworks.
-
In EasyMock, you must set expectations before calling the code under test, making these tests somewhat rigid and less intuitive.
-
In Mockito, the stubbing is more fluid, as you can set up mocks and then verify interactions later, making it easier to follow and read the flow.
When to Use Each Framework
The final choice often boils down to your project requirements, team familiarity, and preferences. Here's a quick guide:
-
Use EasyMock if:
- Your team is already experienced with it
- You prefer its approach to setting expectations
- Your project has fewer dependencies and is not likely to need mocking final classes
-
Use Mockito if:
- You want cleaner and more readable tests
- The project contains final classes, or you anticipate needing to mock them
- You want more intuitive stubbing and straightforward verification
Hands-On Comparisons
Let’s further illustrate the differences through real-world scenarios.
Mocking a Simple Service
Let’s say we have a UserService
that interacts with a UserDAO
to fetch user details.
EasyMock Example
public User getUser(int id) {
User user = userDAO.findUserById(id);
return user != null ? user : new User("Guest");
}
Test with EasyMock:
@Test
public void testGetUserWithNull() {
EasyMock.expect(userDAOMock.findUserById(99)).andReturn(null);
EasyMock.replay(userDAOMock);
User user = userService.getUser(99);
assertEquals("Guest", user.getName());
EasyMock.verify(userDAOMock);
}
Mockito Example
Test with Mockito:
@Test
public void testGetUserWithNull() {
when(userDAOMock.findUserById(99)).thenReturn(null);
User user = userService.getUser(99);
assertEquals("Guest", user.getName());
verify(userDAOMock).findUserById(99);
}
Summary: The Right Choice for You
In the end, both EasyMock and Mockito have their strengths. If you value clarity and simplicity, Mockito is the way to go. It interacts smoothly with modern Java and reduces verbosity in your tests.
On the other hand, if your project has been built around EasyMock or you have specific compliance requirements, it may remain the better choice for your team.
Regardless of your choice, the important part is that you're committed to unit testing and delivering quality software.
For more insights on using Mockito effectively, check out the Mockito documentation and for EasyMock, their official site. Happy testing!
Checkout our other articles