Why Most Beginners Fail with ACID Transactions
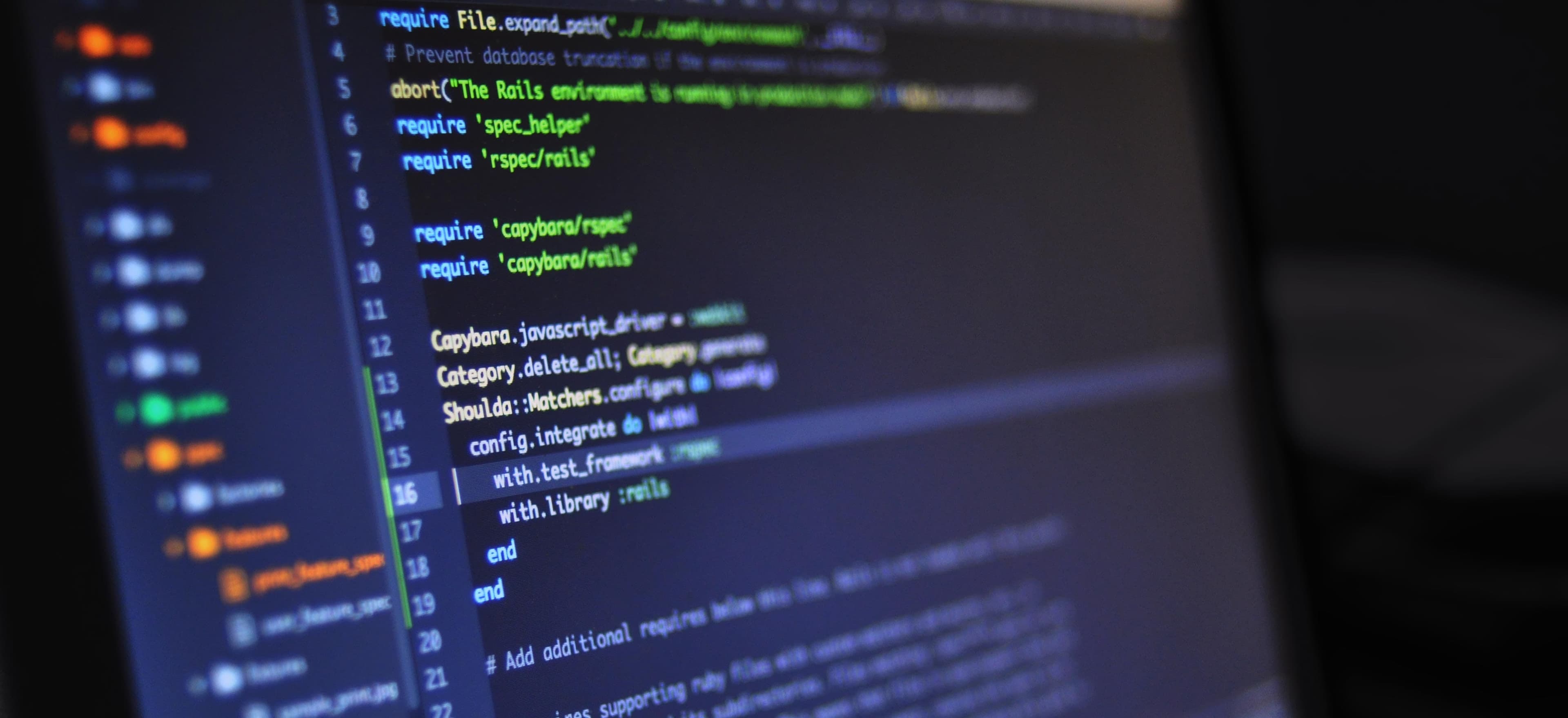
- Published on
Why Most Beginners Fail with ACID Transactions
When diving into database management, the concept of ACID (Atomicity, Consistency, Isolation, Durability) transactions often surfaces as a fundamental principle. Yet, many beginners struggle to grasp its importance and implementation. This blog post seeks to elucidate why most beginners fail with ACID transactions and provide a clear framework to avoid common pitfalls.
Understanding ACID Properties
Before we delve into the reasons for failure, let’s take a moment to understand the ACID properties:
-
Atomicity: This ensures that a transaction is treated as a single, indivisible unit. If one part fails, the entire transaction fails. This prevents partial data updates.
-
Consistency: The database must always transition from one valid state to another. All constraints, such as primary keys or user-defined rules, must be adhered to during a transaction.
-
Isolation: To ensure that transactions are executed independently and without interference from one another, isolation guarantees that concurrent transactions do not affect each other's execution.
-
Durability: Once a transaction has been committed, it will remain so, even in the event of system failures. This guarantees that completed transactions are saved.
Understanding these properties is crucial for anyone working with relational databases. However, their implementation can be taxing for newcomers.
Common Pitfalls for Beginners
Let's explore the common reasons why beginners run into issues with ACID transactions.
1. Lack of Understanding of Transaction Management
Many beginners skip over the concept of transaction management, assuming that the database engine will handle it all. While modern databases indeed have robust management systems, it still requires a firm understanding of how transactions work, what commits and rollbacks do, and how to handle errors effectively.
Example:
Connection connection = null;
try {
connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "user", "password");
connection.setAutoCommit(false); // Begin transaction
// Perform some database operations
connection.commit(); // Commit transaction
} catch (SQLException e) {
if (connection != null) {
connection.rollback(); // Rollback on error
}
e.printStackTrace();
} finally {
if (connection != null) {
connection.close(); // Always close connection
}
}
Why? The above code illustrates the importance of explicitly starting a transaction (setAutoCommit(false)
) and handling exceptions effectively. Beginners often forget these components, leading to unintended data corruption.
2. Overlooking Concurrency Issues
Concurrency is another challenging aspect of ACID transactions. Beginners may not appreciate that multiple transactions can be executed simultaneously, causing race conditions if not handled properly.
Example:
Statement stmt1 = connection.createStatement();
Statement stmt2 = connection.createStatement();
stmt1.executeUpdate("UPDATE accounts SET balance = balance - 100 WHERE account_id = 1");
stmt2.executeUpdate("UPDATE accounts SET balance = balance + 100 WHERE account_id = 2");
Why? The above code doesn’t take isolation into account. If both statements are executed at the same time, the integrity of the accounts may be compromised. This highlights the need for proper transaction isolation levels.
3. Misunderstanding Isolation Levels
Many beginners have a shallow understanding of transaction isolation levels. While it's integral, they often miss configuring the appropriate isolation level for their transactions.
- Read Uncommitted: Allows dirty reads.
- Read Committed: Prevents dirty reads but allows non-repeatable reads.
- Repeatable Read: Prevents both dirty and non-repeatable reads.
- Serializable: The strictest level that simulates transactions as if they were executed one after another.
Example:
connection.setTransactionIsolation(Connection.TRANSACTION_SERIALIZABLE);
Why? Beginners frequently stick to the default isolation level, unaware of its implications. Understanding when to apply higher isolation levels can protect data integrity, albeit at the cost of performance.
4. Neglecting Error Handling and Recovery
Error handling is a crucial component of any transactional system. A common mistake involves assuming that everything will go right during execution, leading to poorly designed error handling.
Example:
try {
// Perform transaction
} catch (Exception e) {
connection.rollback(); // Ensure rollback is handled correctly
System.err.println("Transaction failed: " + e.getMessage());
}
Why? In this case, a flawed transaction results in rollback. Beginners can often neglect this aspect, leading to undetected data inconsistencies across database states.
5. Skipping the Testing Phase
Testing is the backbone of ensuring that your implementation of ACID transactions works as intended. Beginners frequently underestimate the significance of rigorous testing.
Why? Without a proper testing phase, errors may go unnoticed until they manifest in production, resulting in potential data losses or corruption.
6. Inadequate Knowledge of Database Tools and Features
Lastly, beginners often overlook the features and tools provided by modern databases that ensure ACID compliance. Whether it's using prepared statements, ORM frameworks, or advanced database configurations, understanding the full capability of your selected database technology is essential.
Best Practices for Working with ACID Transactions
To avoid these pitfalls, consider adopting the following best practices:
-
Learn the Basics: Familiarize yourself completely with how transactions work and the associated mechanisms in your chosen database.
-
Use Proper Isolation Levels: Understand and apply appropriate isolation levels based on your application's requirements.
-
Implement Structured Error Handling: Always include error handling in your code to catch exceptions and ensure that rollbacks are applied where necessary.
-
Establish a Rigorous Testing Protocol: Include unit testing and integration testing to confirm that transactions behave as expected.
-
Utilize Database Tools Effectively: Leverage the features offered by your database management system, as they can significantly ease the implementation of ACID transactions.
-
Focus on Learning Resources: Leverage online resources, documentation, and communities. Tutorials or courses focused on transactions will give you a broader perspective.
For further resources on database management, check out:
- The ACID Properties in Database Systems
- Understanding Database Transactions
A Final Look
ACID transactions are essential for maintaining data integrity in applications. Beginners often fail due to various pitfalls: lack of understanding, overlooking concurrency, inadequate error handling, and more. By recognizing these pitfalls and implementing the best practices discussed, it is possible to navigate the complexities of ACID transactions with confidence. With diligence and study, you’ll ensure that your applications are robust against data corruption and maintain high reliability.
By approaching ACID transactions in a methodical manner and embracing continuous learning, beginners can not only avoid failure but also become adept at managing their data effectively. Happy coding!