Common Pitfalls When Attaching to Docker Containers
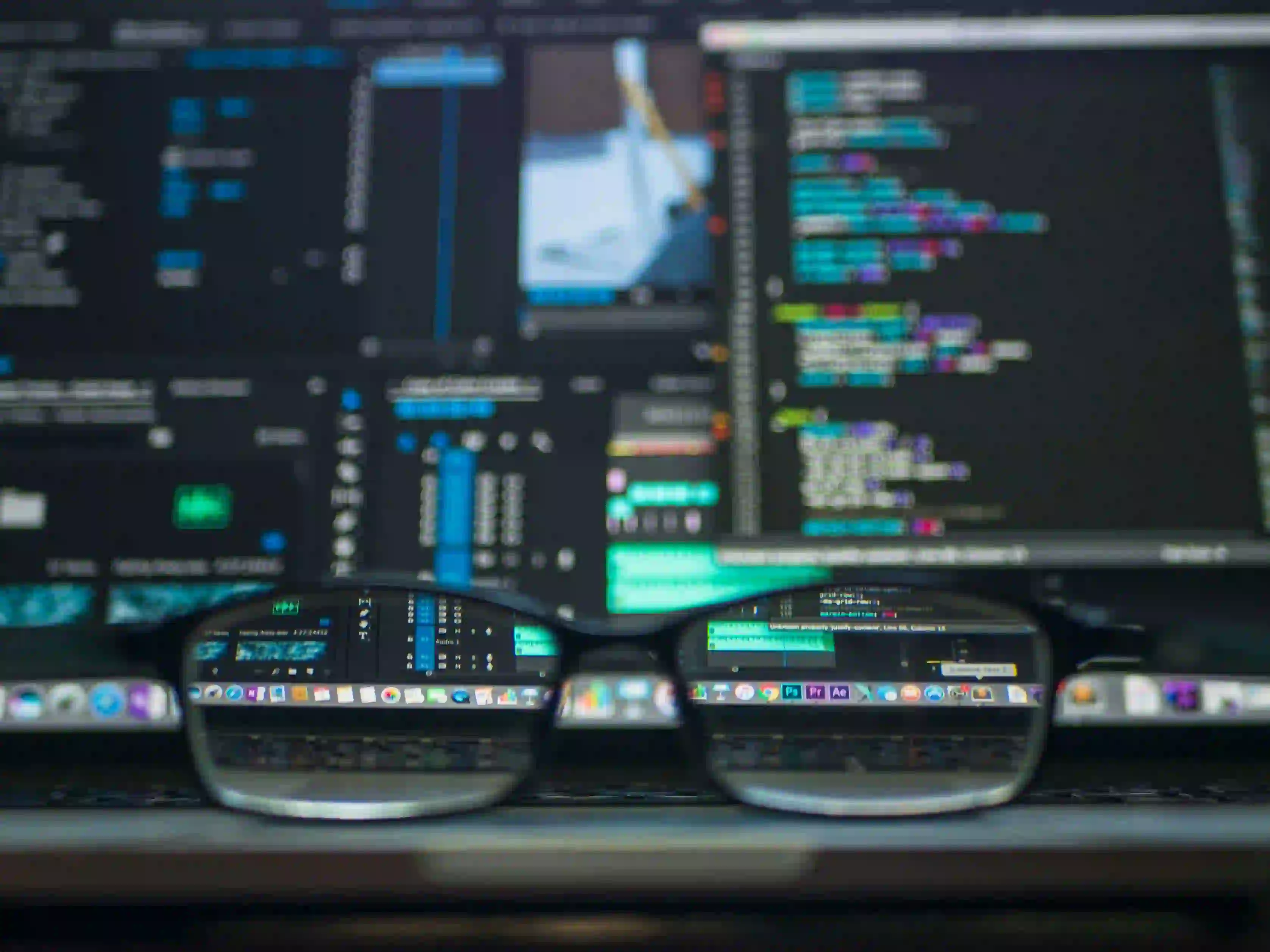
Common Pitfalls When Attaching to Docker Containers
Docker has revolutionized the way developers create, deploy, and manage applications. Whether you are testing locally or running complex microservices architectures, Docker provides a convenient way to encapsulate your application with all its dependencies. However, a common area of confusion for many developers revolves around attaching to Docker containers. This blog post will explore some of the common pitfalls that developers face and how to avoid them.
Understanding Docker Attach vs Execute
Before diving into pitfalls, it's vital to clarify the difference between docker attach
and docker exec
.
-
docker attach: This command allows you to connect to a running container's standard input, output, and error streams. However, this can be misleading since it replays any output that has already occurred which may make it unclear what the current state of the container is.
-
docker exec: This command creates a new process inside a running container. This is generally the preferred method for interacting with containers as it provides a more intuitive experience.
Understanding these commands is crucial because choosing the wrong one can lead to confusion.
Pitfall 1: Not Using the Right Command
One of the most common mistakes is using docker attach
when docker exec
would be more appropriate.
Why This Matters
When you use docker attach
, you essentially connect to the main process of the container. If that process terminates, your session is also terminated. On the other hand, docker exec
allows you to run new processes in the container without affecting the main application.
Code Snippet
Here’s an example illustrating this difference:
# Wrong approach: using docker attach
docker attach my_container
# Correct approach: using docker exec to run a shell
docker exec -it my_container /bin/bash
The first command can be problematic since detaching can complicate your access. The second command is safer; it opens a new shell without impacting the application.
Pitfall 2: Not Using Interactive Mode
When you execute commands in a container, forgetting to include interactive mode can lead to frustrating experiences.
Why This Matters
Interactive mode (-it
) is crucial for commands that require user input, like shells. Without this mode, you might not be able to see your command’s output or provide any input.
Code Snippet
# Missing interactive mode
docker exec my_container /bin/bash
# Correct with interactive mode
docker exec -it my_container /bin/bash
The first command simply runs the shell but does not allow for any interaction. The latter opens an interactive shell, allowing for dynamic input.
Pitfall 3: Network Misconfigurations
Another common pitfall is neglecting to consider network configurations when attaching to Docker containers, especially in multi-container environments.
Why This Matters
Docker containers communicate through networks. If you have not properly configured a network, attempting to connect to services via command line can lead to connectivity issues.
Code Snippet
# Create a custom network
docker network create my_network
# Run containers on the custom network
docker run -d --name webapp --network my_network my_webapp_image
docker run -d --name database --network my_network my_db_image
Creating a custom network helps in isolated communication between containers. Always make sure that your containers interact within the right network environment.
Pitfall 4: Ignoring Permissions
Another common issue is overlooking user permissions when attaching to containers.
Why This Matters
Many Docker images are configured to run processes as non-root users for security. If you attempt to attach as root without adjusting user permissions, you might run into permission errors.
Code Snippet
# This might fail due to permission issues
docker exec my_container /usr/bin/some_command
# Better approach
docker exec -u non_root_user my_container /usr/bin/some_command
By specifying -u non_root_user
, you ensure that the command executes with the correct permissions.
Pitfall 5: Forgetting to Clean Up
After interacting with a container, it's easy to overlook cleanup, which can leave behind unnecessary processes or dangling containers.
Why This Matters
Forgetting to clean up can lead to resource exhaustion, making your development environment slow and difficult to manage.
Code Snippet
# List all stopped containers
docker ps -a
# Remove a stopped container
docker rm my_stopped_container
# Clean up dangling images
docker image prune
Regularly reviewing and cleaning up containers and images ensures that your environment remains efficient.
Pitfall 6: Attempting to Attach to a Stopped Container
A common oversight is trying to attach to a container that has already stopped.
Why This Matters
You cannot attach to a container that isn’t running. This can lead to confusion about container status or errors in your workflow.
Code Snippet
# Check the status of a container
docker ps -a
# If stopped, restart before attaching
docker start my_stopped_container
docker attach my_stopped_container
It's always good practice to check the status of your containers before attempting to interact with them.
Final Thoughts
Understanding the ins and outs of attaching to Docker containers can significantly enhance your productivity and efficiency. By being aware of these common pitfalls—choosing the right commands, enabling interactive mode, managing network configurations, respecting permissions, and keeping your environment clean—you can streamline your workflow and avoid common frustrations.
For further reading on Docker commands, explore the official Docker documentation. Additionally, consider checking out Docker for Beginners, a curated tutorial that can help solidify your understanding of Docker's capabilities.
Whether you are a seasoned professional or just starting in containerization, avoiding these pitfalls will ensure a smoother experience in your development journey. Happy Dockering!