Troubleshooting Gmail SMTP Issues in Spring Boot Apps
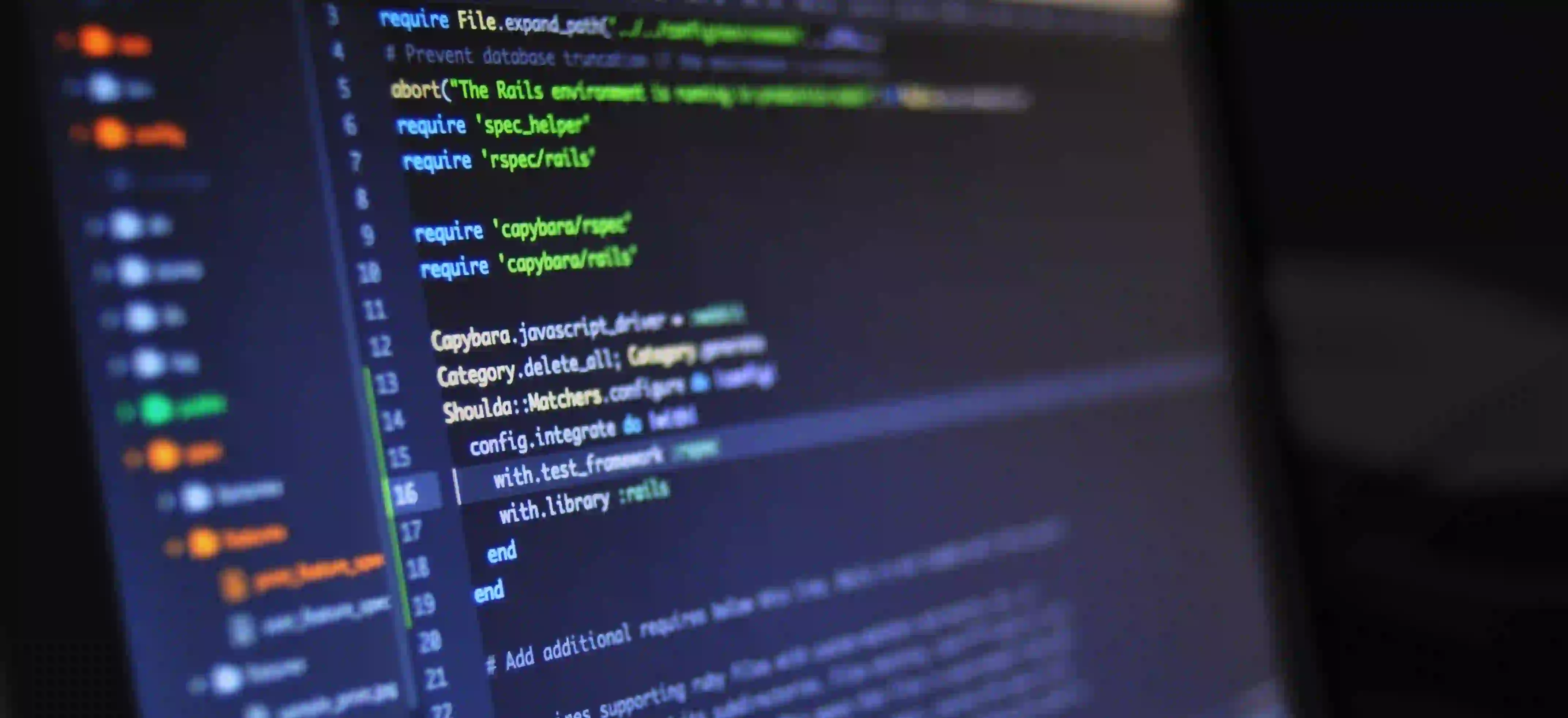
Troubleshooting Gmail SMTP Issues in Spring Boot Apps
When developing applications with Spring Boot, integrating email services can significantly enhance the functionality. Gmail stands out as a popular choice due to its reliability and widespread use. However, developers often encounter SMTP issues when attempting to send emails. In this blog post, we will explore common problems with Gmail SMTP in Spring Boot applications and provide effective solutions to resolve them.
Understanding SMTP and Gmail
SMTP (Simple Mail Transfer Protocol) is a protocol used to send email messages between servers. To send emails using Gmail's SMTP server, developers often require specific configurations and permissions. Knowing how to correctly configure these and what prevalent problems can arise will save you time and frustration.
Basic Configuration
Before diving into troubleshooting, let's set up a basic Spring Boot project to send emails using Gmail SMTP.
Dependency
First, add the following dependency in your pom.xml
file to use JavaMailSender:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
Application Properties
Next, you need to configure your email properties in the application.properties
file:
spring.mail.host=smtp.gmail.com
spring.mail.port=587
spring.mail.username=your-email@gmail.com
spring.mail.password=your-password
spring.mail.properties.mail.smtp.auth=true
spring.mail.properties.mail.smtp.starttls.enable=true
Replace your-email@gmail.com
and your-password
with your actual Gmail credentials. Please note that using plain text is not secure.
Sending an Email
Here’s a simple service to send an email:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.mail.SimpleMailMessage;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.stereotype.Service;
@Service
public class EmailService {
@Autowired
private JavaMailSender emailSender;
public void sendSimpleEmail(String to, String subject, String body) {
SimpleMailMessage message = new SimpleMailMessage();
message.setTo(to);
message.setSubject(subject);
message.setText(body);
emailSender.send(message);
}
}
Common SMTP Issues & Solutions
Despite the simplicity, you may encounter several issues. Let's examine them:
1. App Password Required
Problem: If you have two-factor authentication (2FA) enabled on your Gmail account, direct login with username and password won't work.
Solution: Instead, you need to create an app password.
- Go to your Google Account settings.
- Navigate to "Security."
- Under "Signing in to Google," select "App passwords."
- Generate a new app password and replace your actual password in
application.properties
with this app password.
2. Less Secure App Access
Problem: If you do not have 2FA enabled, but "Less Secure App access" is disabled, you won’t be able to authenticate using just your password.
Solution: Allow less secure apps to access your account.
- Go to your Google Account settings.
- Navigate to "Security."
- Find the section "Less secure app access" and enable it.
Learn more about less secure apps.
3. Firewall / Network Restrictions
Problem: If you are behind a corporate firewall or using certain networks, outgoing connections to SMTP ports may be blocked.
Solution: Check with your network administrator to ensure that outgoing connections on port 587 are allowed. If not, consider using a different network or a VPN.
4. SMTP Authentication Failed
Problem: The error "SMTP authentication failed" can arise from incorrect credentials or not switching to a secure connection.
Solution: Double-check your email and app password. Ensure you’ve set spring.mail.properties.mail.smtp.starttls.enable=true
.
Debugging with Logs
If you are still encountering issues, enabling debug logging can provide additional context. Add the following line to your application.properties
file:
logging.level.org.springframework.mail=DEBUG
It will help in identifying the exact issue with more granularity.
Testing with Postman
As an additional troubleshooting step, you can test emailing functionality using tools like Postman or Telnet to simulate and diagnose SMTP connections independently of your Spring Boot application.
- Postman: Go to the "Body" tab and configure your request to send emails via SMTP.
- Telnet: Run commands to simulate SMTP operations, testing the connection to
smtp.gmail.com
.
Best Practices
-
Environment Variables: Store sensitive information like email and password in environment variables instead of hardcoding them.
-
Error Handling: Implement robust error handling in your email service. Always catch exceptions and log detailed error messages.
-
Email Format: Use HTML format and structured emails to ensure better rendering in various email clients.
Example Sending an HTML Email
If you want to send a more advanced email, here's an example to send an HTML formatted email:
import javax.mail.MessagingException;
import org.springframework.mail.javamail.MimeMessageHelper;
import org.springframework.mail.javamail.JavaMailSender;
public void sendHtmlEmail(String to, String subject, String body) throws MessagingException {
MimeMessage message = emailSender.createMimeMessage();
MimeMessageHelper helper = new MimeMessageHelper(message, true);
helper.setTo(to);
helper.setSubject(subject);
helper.setText(body, true); // true indicates that it's HTML
emailSender.send(message);
}
The Bottom Line
Sending emails via Gmail SMTP in a Spring Boot application can seem daunting, but understanding the common pitfalls simplifies the process. Always make sure to comply with best practices to ensure security and reliability, particularly when it involves sensitive data like email credentials.
By following the suggestions and solutions outlined above, you should be well on your way to successfully integrating Gmail SMTP into your Spring Boot applications. For further reading on email sending in Spring, check out Spring Documentation on Email.
If you have any additional troubleshooting tips or experiences, feel free to share in the comments! Happy coding!