Common Pitfalls in Automated Testing and How to Avoid Them
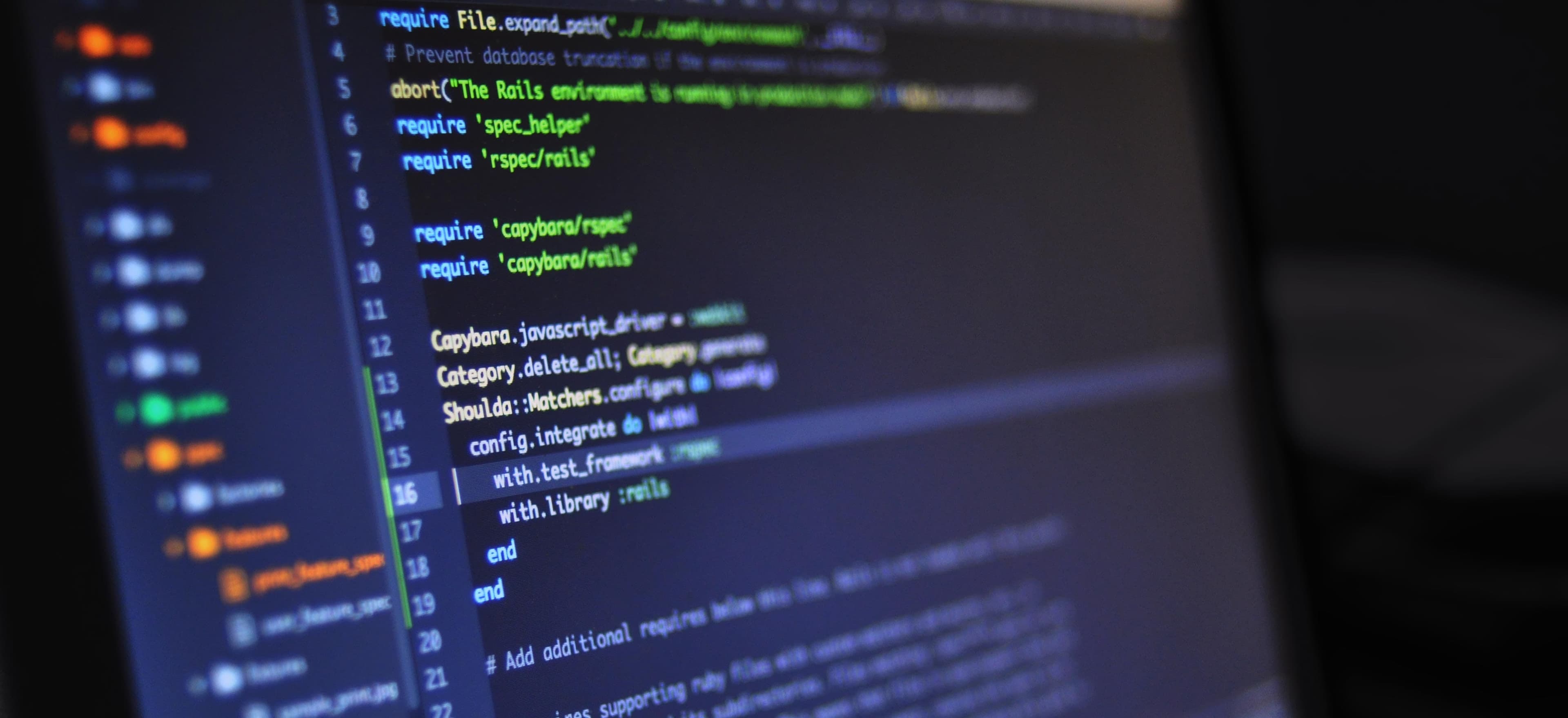
- Published on
Common Pitfalls in Automated Testing and How to Avoid Them
Automated testing is a critical component of software development in today's fast-paced tech landscape. It increases efficiency, ensures greater test coverage, and minimizes human error. However, despite its advantages, it's not without pitfalls. This post will explore common pitfalls in automated testing and provide actionable strategies to avoid them.
1. Lack of Clear Objectives
The Problem: One of the most common pitfalls in automated testing is the absence of clear objectives. Without understanding what you're aiming to achieve, your test automation framework can quickly devolve into chaos.
Solution: Before automating any tests, define clear objectives and scope. What do you want to achieve with your automated tests? Are you looking to validate performance under load or ensure functionality across multiple browsers? Document these objectives to provide a roadmap for your testing efforts.
public class TestObjectives {
public static void main(String[] args) {
String objectives = "1. Validate functionality" +
"\n2. Ensure performance" +
"\n3. Cross-browser compatibility" +
"\n4. Regression testing";
System.out.println("Test Objectives: " + objectives);
}
}
Commentary:
This code snippet demonstrates how to systematically lay out your testing objectives. Clearly defined goals will serve as a guide for your testing process.
2. Choosing the Wrong Tools
The Problem: Selecting the wrong testing tools can lead to inefficiencies and complicate the testing process. Some tools might not support the technologies used in your project, or they may not fit your team's skill set.
Solution: Evaluate your project requirements and team expertise before choosing testing tools. Focus on tools that are compatible with your tech stack. Popular tools in the Java ecosystem include:
- JUnit: A widely used framework for unit testing Java applications.
- Selenium: Ideal for browser-based tests.
- TestNG: Provides advanced features such as parallel execution and data-driven testing.
Example Code Snippet:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class SimpleTest {
@Test
public void testAddition() {
assertEquals(5, 2 + 3);
}
}
Commentary:
Using JUnit for simple test cases helps you get started with automated testing in Java. Ensure your team is comfortable with the tools you choose.
3. Over-Automating Tests
The Problem: It's tempting to automate every test case, but not every test is a candidate for automation. This often leads to time wasted on maintaining tests that do not provide significant value.
Solution: Focus on automating tests that are likely to be run frequently or are critical to application functionality. Manual testing is still essential for exploratory tests and one-off scenarios.
Example Code Snippet:
public class AutomationCandidate {
public static void main(String[] args) {
String testScenario = "1. Login functionality" +
"\n2. Data validation" +
"\n3. Constantly evolving features";
System.out.println("Good candidates for automation: " + testScenario);
}
}
Commentary:
In this code, we've identified the types of tests suitable for automation. Prioritizing high-value test cases mitigates the risks associated with over-automation.
4. Lack of Test Maintenance
The Problem: As the software evolves, tests become outdated or irrelevant. Failing to maintain your test suite can lead to flaky tests, which undermine the entire automated testing effort.
Solution: Regularly review and update your test cases. Establish a schedule for refactoring or removing outdated tests to ensure that your suite remains relevant and reliable.
Example Code Snippet:
public class TestMaintenance {
public static void main(String[] args) {
String maintenanceSchedule = "1. Monthly review of test cases" +
"\n2. Remove flaky tests" +
"\n3. Refactor for new features";
System.out.println("Test Maintenance Schedule: " + maintenanceSchedule);
}
}
Commentary:
This snippet emphasizes the importance of a routine maintenance schedule for your test suite. Consistent upkeep ensures the efficacy of your automated tests.
5. Ignoring Test Results
The Problem: Ignoring the results of automated tests is a common mistake. If you don't analyze test failures, you could miss critical issues in your application.
Solution: Establish a process for regularly reviewing test results. Use reporting tools to track test performance over time and make informed decisions about your code.
Example Code Snippet:
import org.junit.Test;
public class ResultAnalysis {
@Test
public void analyzeResults() {
boolean testPassed = false; // Simulated test result
if (!testPassed) {
System.out.println("Test failed. Immediate review required!");
} else {
System.out.println("Test passed successfully!");
}
}
}
Commentary:
This simple test shows how you can incorporate result analysis into your automated tests. Regular reviews will help catch problems early.
6. Poorly Defined Test Cases
The Problem: Automated tests based on poorly defined test cases can produce unreliable results and require extensive maintenance.
Solution: Invest time in creating clear, well-structured test cases. Use clear naming conventions, and ensure that each test case has a defined purpose and expected outcome.
Example Code Snippet:
public class WellDefinedTest {
public static void main(String[] args) {
String testCase = "Verify User Authentication" +
"\nStep 1: Input correct username and password" +
"\nStep 2: Validate successful login";
System.out.println("Test Case: " + testCase);
}
}
Commentary:
This snippet illustrates how to articulate a test case clearly. Properly defined tests will lead to more effective automation.
Bringing It All Together
Automated testing can significantly improve your software development lifecycle. However, avoiding common pitfalls is essential for maximizing its potential. Remember to set clear objectives, choose the right tools, avoid over-automation, maintain your tests properly, analyze results, and define test cases thoroughly. By following these best practices, your automated testing journey will be smoother and more efficient.
For further reading, check out The Importance of Automated Testing as well as Best Practices in Test Automation.