Why Your Java Regex Performance May Be Slowing You Down
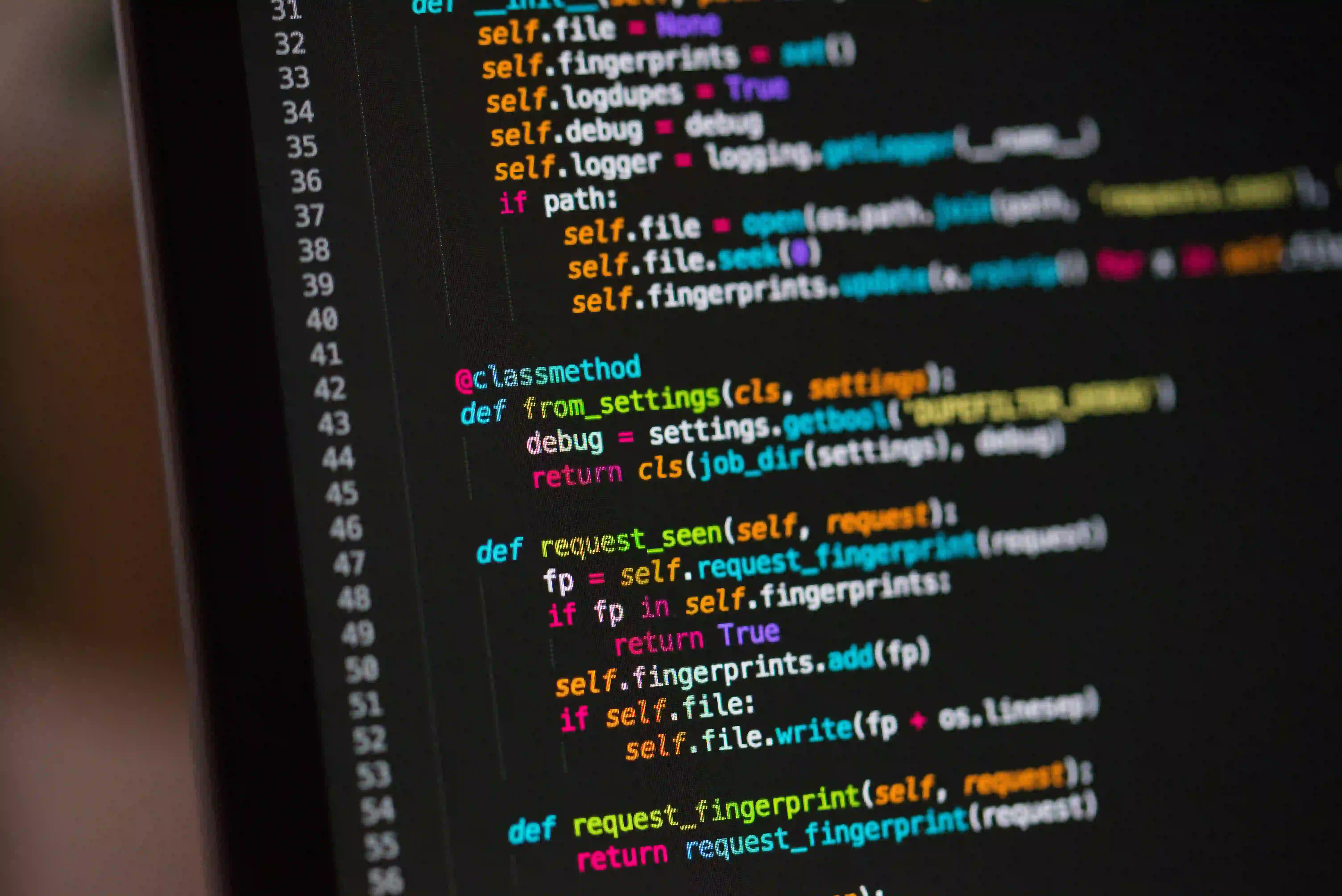
Why Your Java Regex Performance May Be Slowing You Down
Regular expressions (regex) are an indispensable tool in Java, providing a flexible method for string matching and manipulation. They enable you to search, replace, and parse text efficiently. However, when regexes are improperly implemented, they can seriously hinder application performance. In this blog post, we’ll dive deep into the reasons why your Java regex performance may be slowing you down and provide strategies to optimize regex usage.
Understanding Regular Expressions
A regular expression is essentially a sequence of characters that define a search pattern. In Java, regex is handled by the java.util.regex
package, which includes classes like Pattern
and Matcher
.
Here's a simple example of regex in Java:
import java.util.regex.Pattern;
import java.util.regex.Matcher;
public class RegexExample {
public static void main(String[] args) {
String input = "Hello, World!";
String regex = "Hello.*";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(input);
if (matcher.find()) {
System.out.println("Match found: " + matcher.group());
} else {
System.out.println("No match found.");
}
}
}
In this code snippet, the regex Hello.*
matches any string that starts with "Hello". The .*
part is a wildcard that can match any number of characters.
Why Regex Performance Matters
When you perform regex operations, especially on large datasets, the performance can become a bottleneck. Issues can arise from several factors. Here are some reasons why regex performance may falter:
1. Complex Pattern Matching
Complex regex patterns can take a long time to process. Expressions with multiple quantifiers, alternations, or backreferences can create catastrophic backtracking situations.
Example of Catastrophic Backtracking
Consider the following regex pattern:
String regex = "(a|aa|aaa|aaaa|aaaaa)b";
If given a long string filled with "a" characters, this regex may cause significant delays. The regex engine will try numerous combinations, eventually leading to performance degradation.
2. Greedy vs. Lazy Matching
Greedy matching attempts to match as much text as possible, while lazy matching does the opposite, trying to match the smallest possible string. Misusing greediness can lead to excessive backtracking.
Greedy Example
String regex = ".*bad.*";
In this case, using .*
can also consume the entire input text before identifying a match.
3. Inefficient Character Classes
Character classes can also complicate regex performance. Using overly broad classes can slow down the search, as the engine tries to check against multiple characters.
Inefficient Example
String regex = "[a-zA-Z0-9]{4,}";
If you are only dealing with specific characters, optimize the ranges you use.
4. Repeated Invocations
Compiling regex patterns repeatedly can lead to performance losses. Compiling a regex pattern is an expensive operation. Take advantage of the Pattern
class’s caching mechanism by compiling your pattern once and reusing it.
Compiling Once Example
Pattern pattern = Pattern.compile("your-regex-here");
// Use the same pattern as many times as needed
Matcher matcher = pattern.matcher(yourString);
5. Using String Methods for Simple Tasks
Regular expressions are powerful, but they can be overkill for straightforward string manipulation. Using Java's built-in string methods like contains()
, startsWith()
, and endsWith()
can significantly improve performance for simple checks.
Simple String Check Example
String str = "Hello, World!";
if (str.startsWith("Hello")) {
// Do something
}
This is faster than using regex and achieves the same goal.
Techniques for Optimizing Regex in Java
Understanding the pitfalls mentioned above can help in fine-tuning your regex usage. Here are a few techniques for optimizing regex performance:
1. Avoid Catastrophic Backtracking
Keep regex patterns as simple and specific as possible. Avoid nested quantifiers:
Instead of:
String regex = "(a+)+b";
Opt for:
String regex = "a+b";
This modification eliminates the nested quantifiers, leading to better performance.
2. Use Non-Greedy Matching Appropriately
If you find yourself in a situation where you need to match an arbitrary string within a set boundary, consider using non-greedy modifiers.
Non-Greedy Example
String regex = "<.*?>"; // This matches HTML tags non-greedily.
This ensures that the smallest possible match is made, reducing the possibility of backtracking.
3. Profile and Benchmark
Regularly profile your application to identify where regex patterns cause delays. Use tools like Java VisualVM or YourKit for monitoring.
4. Leverage Precompiled Patterns
Cache or precompile your regex patterns to boost performance:
private static final Pattern COMPILED_PATTERN = Pattern.compile("your-regex-here");
// Method using the compiled pattern
public void performRegexMatch(String input) {
Matcher matcher = COMPILED_PATTERN.matcher(input);
// Process matches
}
5. Consider Alternatives to Regex
In some cases, using a combination of Java string methods and libraries like Apache Commons Lang can yield efficiency boosts. Libraries provide relevant utilities that might replace a complex regex.
Additional Resources
- Java Regular Expressions Documentation
- Regex Performance Patterns
- Java Pattern and Matcher
Wrapping Up
Regular expressions are a critical part of Java programming, offering powerful string manipulation capabilities. However, their performance can be hindered by complex patterns, greedy matching, inefficiencies, and repetitive compilations. By refining your regex practices, leveraging non-greedy matching, avoiding catastrophic backtracking, and profiling your application, you can significantly enhance the performance of regex in your Java applications.
Regex should serve as a tool for efficiency, not an obstacle. Implement these strategies and watch your performance soar!