Why ESBs Fall Short in Modern Microservices Architecture
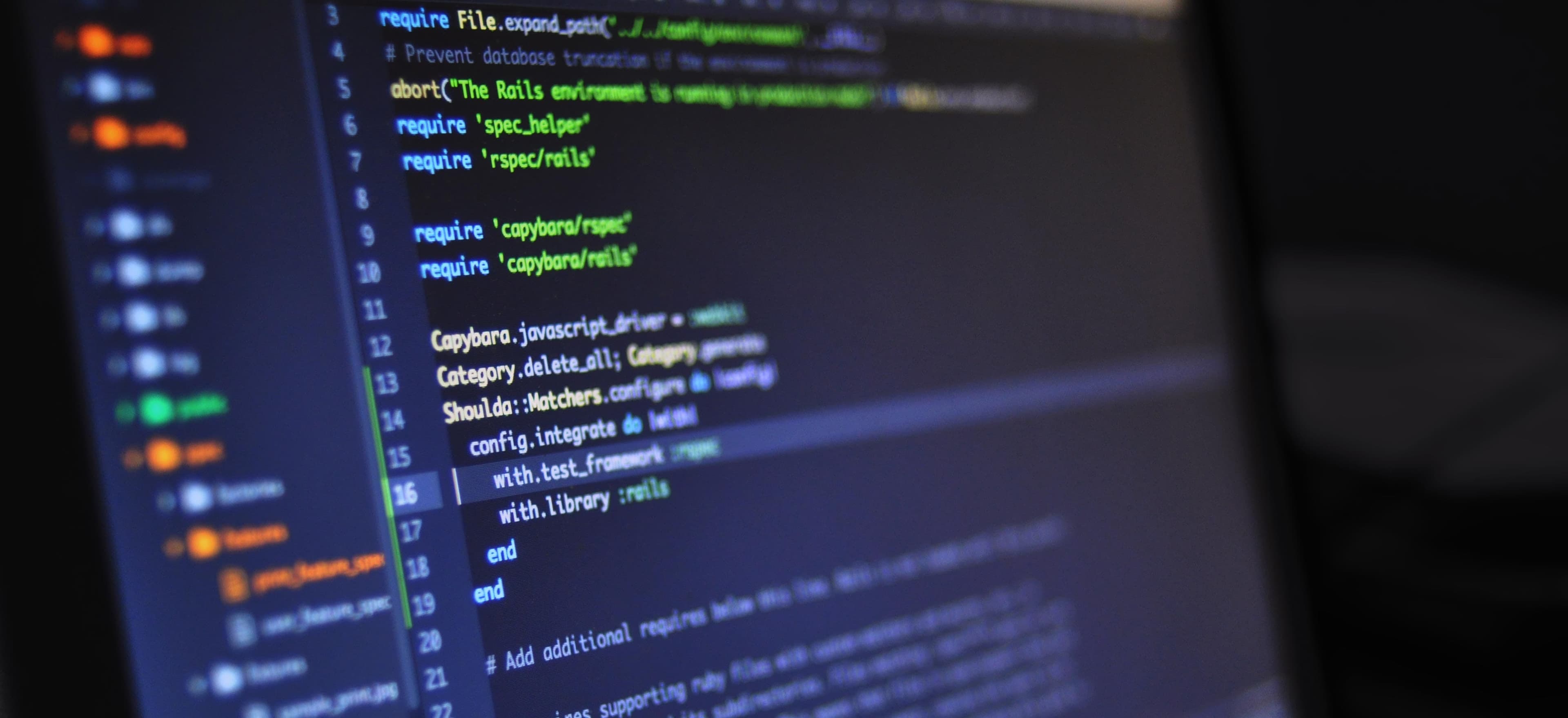
- Published on
Why ESBs Fall Short in Modern Microservices Architecture
The rise of microservices architecture (MSA) has transformed the software development landscape. As applications evolve into granular services to improve scalability and agility, the traditional approaches to integration, particularly Enterprise Service Buses (ESBs), are often found lacking. This post explores why ESBs do not fit well into the modern microservices paradigm, focusing on flexibility, complexity, and performance.
Understanding Microservices Architecture
Before diving into the shortcomings of ESBs, it's essential to understand what microservices architecture entails. At its core, microservices is an architectural style that structures an application as a collection of loosely coupled services. Each service is:
- Independent: Each microservice can be developed, deployed, and scaled independently.
- Focused: Designed around specific business capabilities, serving a single purpose effectively.
- Decentralized: Data management is distributed, typically favoring polyglot persistence.
This independence allows development teams to enhance productivity and adapt quickly to changing requirements. However, the way we connect these independent services necessitates a different approach than the centralized model offered by ESBs.
The Traditional Role of ESBs
Enterprise Service Buses were designed to facilitate communication between different applications, particularly in Service-Oriented Architecture (SOA). They provide features such as:
- Message routing: Determining the correct destination for outbound messages.
- Protocol translation: Converting messages between various protocols.
- Data transformation: Modifying message formats to ensure interoperability.
While these features were essential in monolithic or SOA applications, the modern landscape has evolved in ways that render ESBs less effective.
1. Tightly Coupled Architecture
One of the critical issues with using an ESB in a microservices architecture is that ESBs lead to tightly coupled systems. In a traditional ESB setup:
- Centralized Dependency: All messages pass through the ESB, creating a single point of failure.
- Inherent Latency: Service calls require communication back and forth through the central bus, which increases response times.
In contrast, microservices thrive on the principle of loose coupling. Services communicate directly with each other, minimizing dependencies.
Example: Direct Communication
@Service
public class OrderService {
private final InventoryService inventoryService;
public OrderService(InventoryService inventoryService) {
this.inventoryService = inventoryService;
}
public void createOrder(Order order) {
if (inventoryService.isProductAvailable(order.getProductId())) {
// Create order logic...
}
}
}
In this example, OrderService
calls InventoryService
directly. This allows quicker service modifications without needing changes in the ESB or affecting other services.
2. Increased Complexity
The integration of an ESB introduces additional complexity to microservices architecture:
- Configuration Overhead: Setting up and maintaining an ESB can require significant operational overhead.
- Learning Curve: Developers must learn the ESB's intricacies, convoluting the development process.
Microservices benefit from simplicity. Each service can understand and communicate with the services they depend on without additional layers of abstraction.
Keeping It Simple with Spring Cloud
Spring Cloud offers various tools to simplify service communication without introducing the complexity of an ESB.
@RestController
public class UserController {
@HystrixCommand
@GetMapping("/users/{id}")
public User getUser(@PathVariable String id) {
return userService.getUser(id);
}
}
Hystrix is a library that enables fault tolerance for microservices without a central bus. It makes communication more straightforward and resilient.
For more on Spring Cloud and its solutions for microservices architecture, check the Spring Cloud documentation.
3. Performance Bottlenecks
Performance is crucial in a microservices architecture. However, ESBs can create significant performance bottlenecks:
- Single Point of Failure: If the ESB becomes slow or fails, it directly impacts all services relying on it.
- Overhead: The additional processing required for data transformations and routing can lead to latency issues.
Microservices aim for optimal performance through direct, lightweight communication. A study by Martin Fowler emphasizes that microservices should communicate using lightweight protocols, like REST or gRPC, to maintain the system's responsiveness.
Lightweight Communication with REST
@RestController
@RequestMapping("/api/inventory")
public class InventoryController {
@Autowired
private InventoryService inventoryService;
@GetMapping("/check/{productId}")
public ResponseEntity<Boolean> checkProduct(@PathVariable String productId) {
boolean isAvailable = inventoryService.checkAvailability(productId);
return ResponseEntity.ok(isAvailable);
}
}
In the example above, the InventoryController
checks product availability via a RESTful API, establishing a lightweight communication channel that preserves performance.
4. Flexibility and Adaptability
Microservices require flexibility to adapt to changing business needs rapidly. ESBs lack this agility:
- Versioning Issues: Changes to an ESB configuration can lead to complicated versioning problems, affecting all dependant services.
- Rigid Governance: Overhead from governance can stifle innovation, contrary to the convenience of agile frameworks.
By enabling each service to evolve independently, microservices foster innovation. Development teams can easily update services without realigning with an overarching governance structure.
Gradual Transition
Development teams can also take a gradual approach to microservices, often referred to as the strangler pattern, where the existing monolith can be incrementally moved to a microservices architecture over time, minimizing risks and dependencies that ESBs would typically entrench.
Key Takeaways
In conclusion, while ESBs have historically served an essential role in application integration, they fall short in modern microservices architecture. The preferences lean towards decentralized communication models, lightweight protocols, and independent service capabilities. Adopting a microservices approach means embracing the principles of loose coupling, simplicity, and performance—which ESBs often compromise.
As teams transition to microservices, it's essential to leverage technology stacks that foster seamless integration and robust communication without the burdens tied to traditional ESB architectures. Choices such as REST APIs, event-driven architectures, and frameworks like Spring Cloud can crucially empower development teams to harness the agile potential of microservices architecture without the constraints of ESB.
With this understanding, organizations can make informed decisions that align with their architectural vision, ensuring that their technological frameworks meet modern demands effectively.
Checkout our other articles