Common Mistakes Beginners Make with Hazelcast
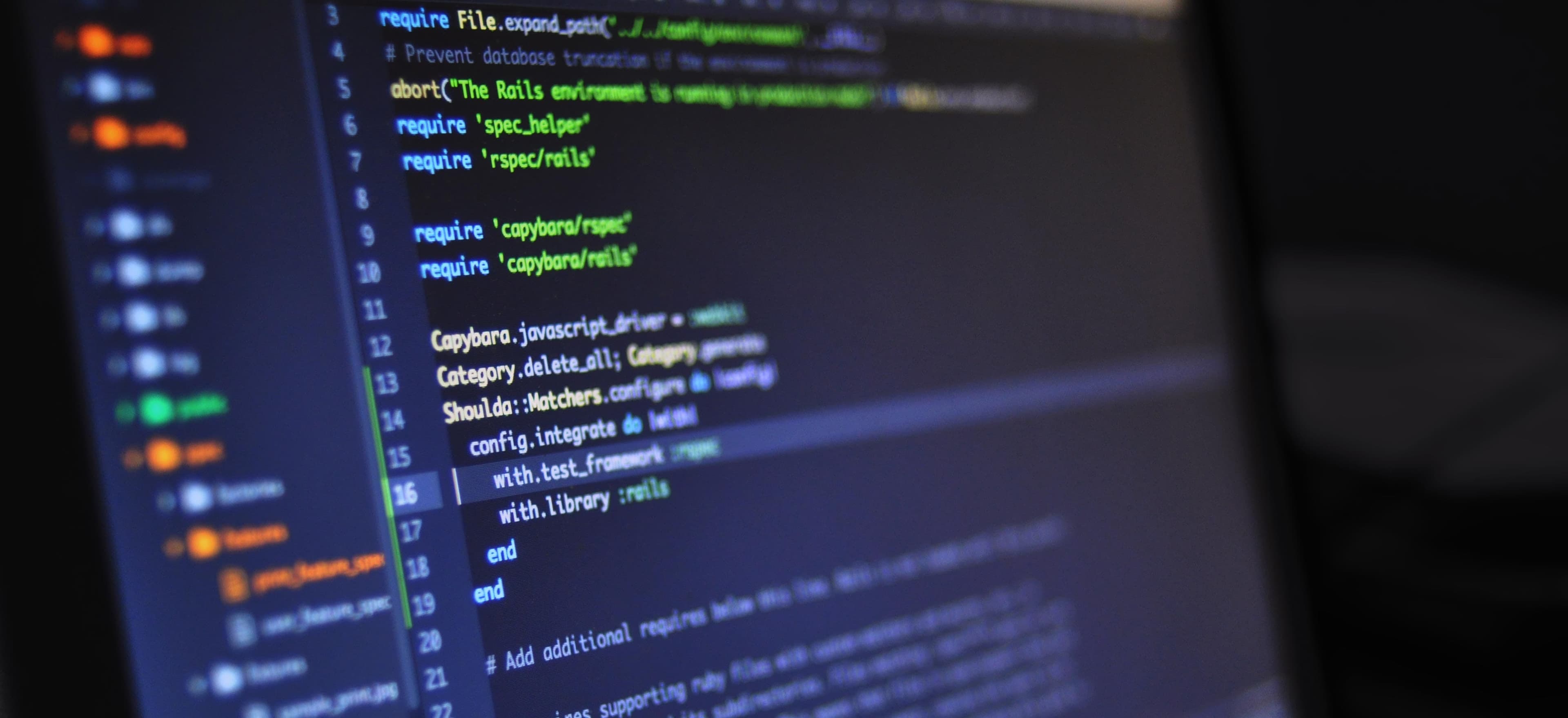
- Published on
Common Mistakes Beginners Make with Hazelcast
Hazelcast is an in-memory data grid (IMDG) that provides a scalable and reliable way to manage data across various applications. It is a powerful tool for distributed computing, making it easier to build high-performance applications. However, beginners often encounter several pitfalls when starting with Hazelcast. In this blog post, we will discuss some of the most common mistakes that new users make and how to avoid them.
1. Ignoring Cluster Configuration
One of the first mistakes beginners make is not paying attention to the cluster configuration. Hazelcast operates using a cluster of nodes, which means that each node needs to communicate effectively with the others.
Solution
Before diving into code, ensure that your hazelcast.xml
or programmatic configuration is set up correctly. Here's a basic example of what your XML configuration might look like:
<hazelcast>
<network>
<port>5701</port>
<join>
<multicast enabled="false"/>
<TcpIp enabled="true">
<member>127.0.0.1:5701</member>
<member>127.0.0.1:5702</member>
</TcpIp>
</join>
</network>
</hazelcast>
In this configuration:
- Port: Specifies the port for node communication.
- Join: Configures how nodes discover each other; in this case, TCP/IP is enabled.
Ensuring correct configuration of these parameters will prevent many connectivity issues you might face later.
2. Not Understanding Partitioning
Another common error is the lack of understanding of data partitioning in Hazelcast. Hazelcast partitions your data across different nodes, and each partition is owned by a single node at any given time.
Why Partitioning Matters
By not taking partitioning into account when designing your application, you may end up creating hotspots or uneven loads on your nodes.
Example of Correct Partitioning
When you store data in a map, Hazelcast automatically manages partitioning. However, if you define custom partitioning logic, ensure that your partitionKey
is correctly implemented. Here’s an example:
public class MyValue implements HazelcastInstance {
@Override
public int getPartitionKey() {
return yourCustomHashFunction();
}
}
In this snippet, getPartitionKey
uses a hash function to ensure a balanced distribution of data across partitions.
3. Not Properly Handling Serialization
Serialization is a critical component of Hazelcast. If objects are not properly serialized, you may run into issues when you retrieve data from your data grid.
Solution
Always make sure that the objects being stored in Hazelcast implement Serializable
. Here's a sample class:
import java.io.Serializable;
public class UserData implements Serializable {
private String name;
private int age;
public UserData(String name, int age) {
this.name = name;
this.age = age;
}
// Getters and setters
}
By making your UserData
class implement Serializable
, you ensure that it can be correctly stored and retrieved from the Hazelcast cluster.
4. Not Leveraging Hazelcast Features
Hazelcast comes packed with features like distributed computing, near-cache, and IMap. Beginners often use only the basic features when ample functionality is available.
Example of Using IMap
Here’s how you can utilize an IMap
for distributed caching:
HazelcastInstance hazelcastInstance = Hazelcast.newHazelcastInstance();
IMap<String, UserData> userMap = hazelcastInstance.getMap("user");
UserData user = new UserData("Alice", 30);
userMap.put("user1", user);
With IMap
, you gain advantages of distributed data storage along with near-cache capabilities, reducing latency and improving performance.
5. Neglecting Monitoring and Management
Ignoring the monitoring and management capabilities of Hazelcast can lead to missed performance issues or bottlenecks. Hazelcast provides tools for monitoring cluster health and performance metrics.
Solution
Use Hazelcast Management Center to monitor your cluster. You can set it up as follows:
- Download Management Center: Get the latest version from the Hazelcast website.
- Run Management Center: Use Docker or run it as a standalone application.
- Connect to hazelcast instance: Configure the management center to connect to your Hazelcast instance for real-time statistics.
This will help you quickly identify issues and optimize performance.
6. Poor Resource Management
Hazelcast relies heavily on memory and network resources. Beginners might not monitor these resources properly and could face performance issues as a result.
Optimizing Resource Use
Using Hazelcast’s configurations, you can manage memory more effectively:
<map name="user">
<in-memory-format>OBJECT</in-memory-format>
<eviction>
<max-size policy="PER_NODE">1000</max-size>
</eviction>
</map>
In this configuration:
- In-memory-format: Selects the format of the data in memory (OBJECT or BINARY).
- Eviction: Sets limits to prevent memory overflow.
Proper resource management can significantly enhance application performance and stability.
7. Lack of Testing Distributed Scenarios
Many beginners often build applications without adequately testing distributed scenarios. Given that Hazelcast is inherently a distributed system, your application should be tested in a clustered environment.
Solution
Always set up a testing cluster that mirrors your production environment as closely as possible. Utilize integration tests with multiple client instances to cover various edge cases, and always profile your applications under these conditions.
Example of a Simple Test Configuration
Using JUnit, a simple test could look like this:
@Test
public void testDistributedMap() {
HazelcastInstance instance1 = Hazelcast.newHazelcastInstance();
HazelcastInstance instance2 = Hazelcast.newHazelcastInstance();
IMap<String, String> map = instance1.getMap("testMap");
map.put("key", "value");
Assert.assertEquals("value", instance2.getMap("testMap").get("key"));
}
This test verifies that data put into the map is accessible from different nodes, ensuring that the distributed map is functioning as expected.
Closing Remarks
Getting started with Hazelcast can be a rewarding journey, but it comes with its challenges. By being aware of common beginner mistakes and employing best practices, you can harness the full potential of Hazelcast.
For further learning, I recommend additional reading on Hazelcast Documentation and checking out the official Hazelcast GitHub Repository for practical examples and updates.
By avoiding these pitfalls and honing your familiarity with Hazelcast, you'll be well on your way to creating resilient, scale-out applications that benefit from in-memory performance. Happy coding!
Checkout our other articles