Mastering Maven: Fixing Failsafe Issues with Test Separation
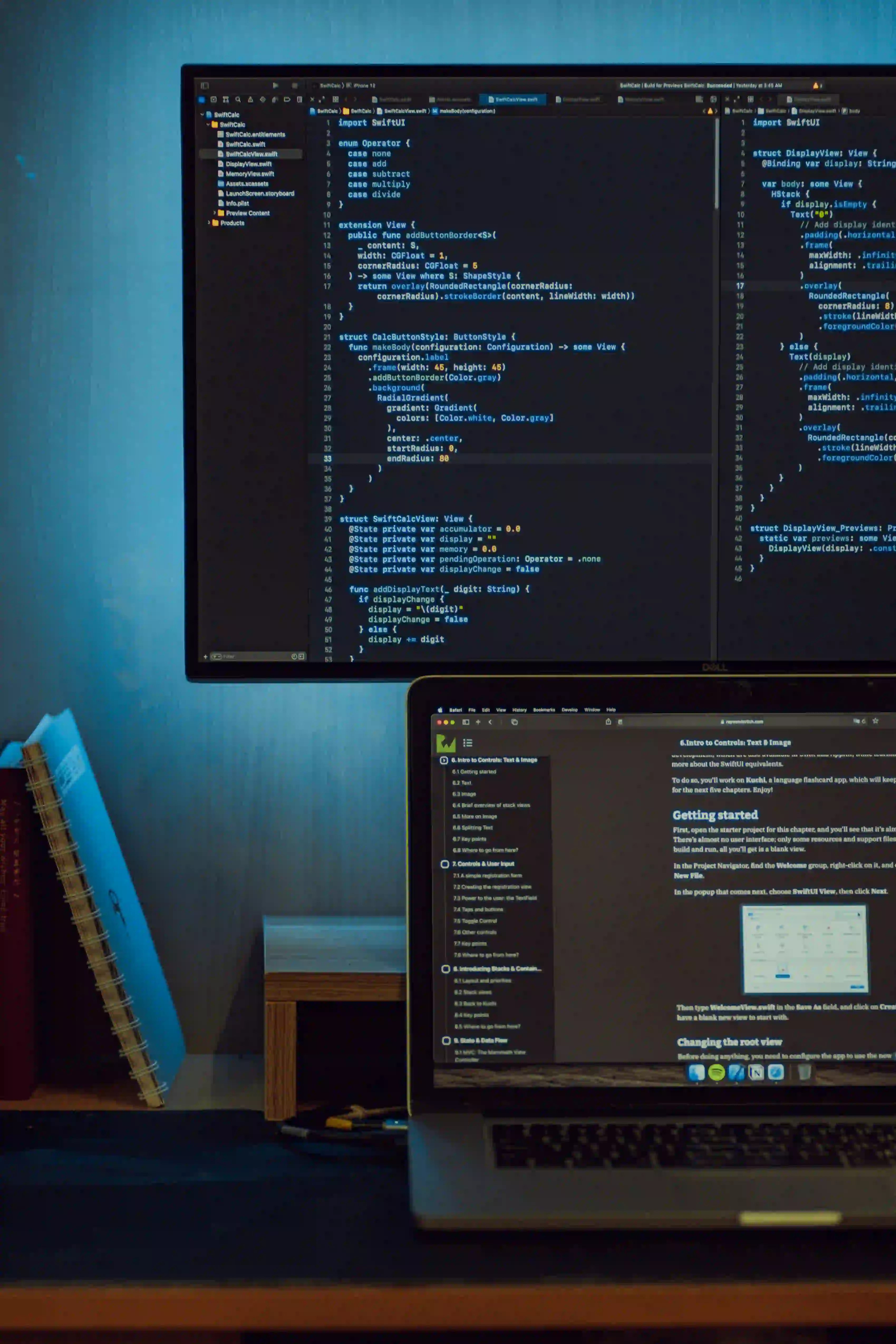
Mastering Maven: Fixing Failsafe Issues with Test Separation
In the world of Java development, Maven's Failsafe Plugin is a powerful tool designed to handle integration testing. However, dealing with Failsafe issues can be tricky, especially when tests do not run as expected. This post will guide you through troubleshooting Failsafe issues, emphasizing test separation and management practices.
What is the Maven Failsafe Plugin?
The Maven Failsafe Plugin is responsible for running integration tests in your Maven project. Unlike the Surefire Plugin, which is primarily used for unit tests, Failsafe focuses on tests that require a deployed environment or multiple components working together.
The primary benefit of using Failsafe is its ability to handle both success and failure cases during testing, ultimately aiding in the creation of a robust application. However, if not configured properly, Failsafe can cause headaches during the build process.
Understanding Test Separation
When working on a large Java project, it's prudent to separate unit tests from integration tests. Here's why:
-
Clarity: Unit tests validate individual components in isolation, while integration tests check how several parts work together.
-
Efficiency: Running unit tests is generally faster than running integration tests since they do not involve deployment or setup overhead.
-
Continuous Integration (CI): Separating these tests allows teams to quickly identify issues in CI environments.
Maven Project Structure
To effectively use the Failsafe Plugin, ensure your Maven project is structured appropriately. Here's a typical project layout:
my-java-project/
βββ pom.xml
βββ src/
β βββ main/
β β βββ java/
β βββ test/
β β βββ java/
β βββ integration-test/
β βββ java/
In this structure:
- Place your unit tests in
src/test/java/
. - Place your integration tests in
src/integration-test/java/
.
This explicit separation allows Maven to manage the tests correctly and ensures the Failsafe Plugin only evaluates integration tests.
Setting Up Failsafe Plugin in POM
Hereβs a sample Maven pom.xml
configuration for integrating the Failsafe Plugin:
<project>
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-java-project</artifactId>
<version>1.0-SNAPSHOT</version>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-failsafe-plugin</artifactId>
<version>3.0.0-M5</version>
<executions>
<execution>
<goals>
<goal>integration-test</goal>
<goal>verify</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
</project>
In this example:
- The
<version>
specifies which version of the Failsafe plugin to use; adjust this according to your project's requirements. - The
<goals>
includes bothintegration-test
andverify
, which are necessary for executing and validating integration tests respectively.
Creating Integration Tests
Coherently writing integration tests is essential. Hereβs an example using JUnit:
package com.example.integration;
import org.junit.Test;
import static org.junit.Assert.assertTrue;
public class SampleIntegrationTest {
@Test
public void testServiceIntegration() {
// Mock setting up services and dependencies
Service service = new Service();
// Execution of the service method
boolean result = service.performAction();
// Validating the outcome
assertTrue("Service action should return true", result);
}
}
Commentary
In this code snippet:
- We define a simple integration test with JUnit.
- The test simulates a service integration scenario, ensuring that the
performAction()
method returnstrue
. - Clear assertions follow lifecycle events, effectively creating a communicable story to stakeholders.
Common Failsafe Issues and Solutions
Letβs explore some common issues you might encounter when using the Failsafe Plugin and how to address them.
1. Tests Not Found
If your integration tests are not being picked up, ensure they follow the correct naming convention. The Failsafe Plugin looks for classes with names that end in IT
, IntegrationTest
, or follow the pattern *IT*.java
.
2. Failsafe Not Running Tests
Often, developers might forget to run the Failsafe Plugin during the build lifecycle. To ensure it runs, always execute:
mvn clean verify
This command triggers the Failsafe Plugin, executing the integration-test
and verify
phases.
3. Integration Test Failures
Integration tests can fail for many reasons, such as:
- Environment issues.
- Dependency misconfigurations.
To diagnose these failures, check the logs generated during the build:
mvn clean verify | tee build.log
Reading through build.log
will provide insights into what went wrong and why.
4. Test Timeout Issues
Sometimes, tests can timeout due to the integration waiting for services to respond. To mitigate timeouts, you can configure your Failsafe Plugin with timeout settings, for example:
<configuration>
<forkCount>1</forkCount>
<reuseForks>true</reuseForks>
<timeout>30000</timeout>
<timeoutSuite>60000</timeoutSuite>
</configuration>
These configurations help to increase the timeout for both tests and suites, accommodating slower integrations.
Best Practices
- Maintain Isolation: Use mocks and avoid external dependencies where possible in integration tests.
- Focus on Failure: Write tests that can provide clear failure messages to simplify debugging.
- Regular Reviews: Regularly check and refactor tests to maintain test quality and relevance.
- Version Control: Keep your Maven and Failsafe Plugin versions up to date. For the latest features and performance improvements, regularly check Maven Failsafe Plugin on Maven.io.
My Closing Thoughts on the Matter
Mastering the Maven Failsafe Plugin and understanding test separation can significantly enhance your Java development process. While integration testing may initially seem challenging, following best practices and utilizing the right configurations can streamline your workflow.
By implementing the strategies discussed above, you will be better equipped to handle Failsafe issues, ensuring your software is stable and reliable. Happy testing!