Common JavaFX Mistakes Beginners Make and How to Fix Them
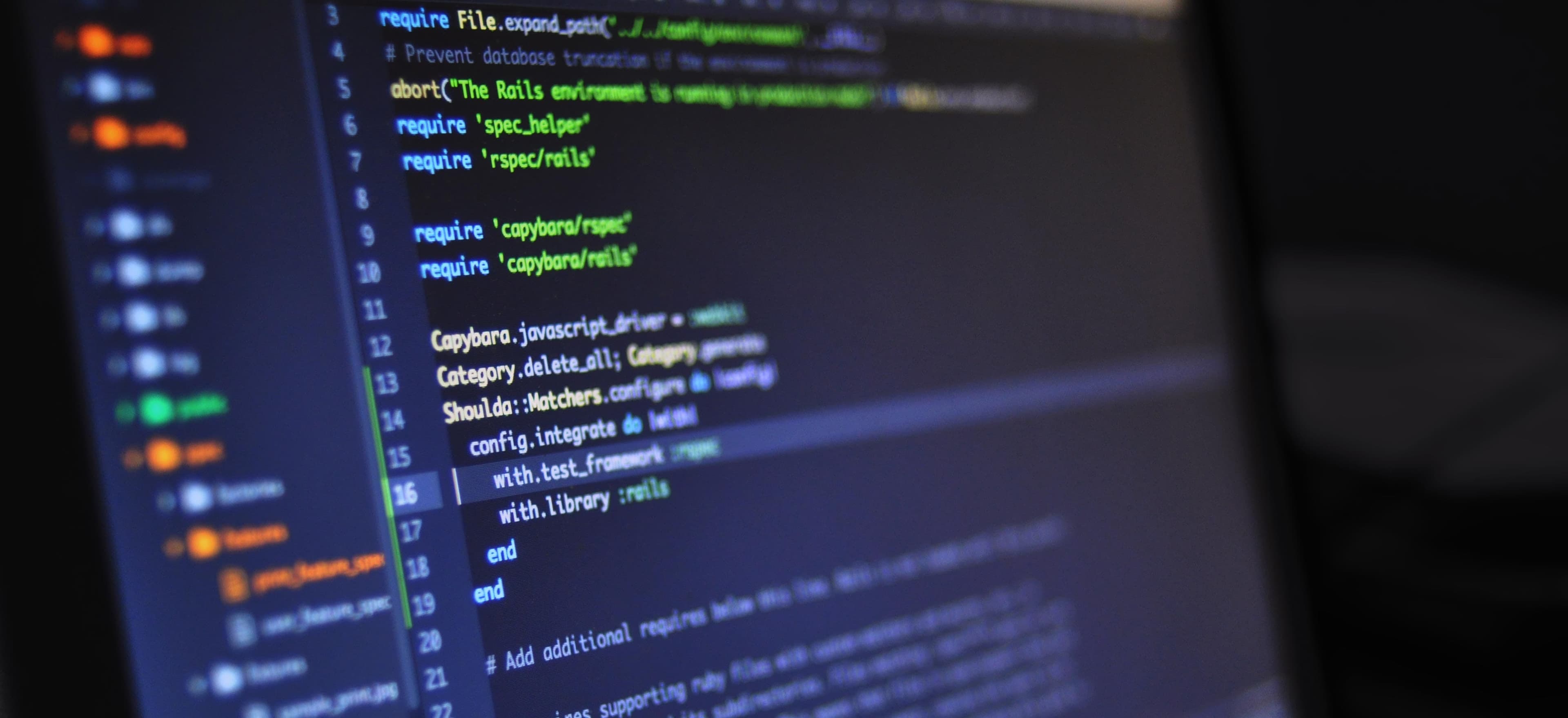
- Published on
Common JavaFX Mistakes Beginners Make and How to Fix Them
JavaFX is a powerful framework for building rich desktop applications in Java. While its capabilities are vast and impressive, beginners often stumble due to lack of experience with the framework's intricacies. In this blog post, we will delve into some common mistakes that JavaFX beginners frequently make and offer actionable solutions for each.
1. Ignoring the JavaFX Application Thread
One of the most critical aspects of JavaFX is its single-threaded architecture. JavaFX UI components can only be modified on the JavaFX Application Thread. A common mistake is attempting to update UI elements from background threads, leading to runtime exceptions or unexpected behavior.
Example:
Thread backgroundThread = new Thread(() -> {
// Performing a long-running task
String result = performLongTask();
// ERROR: This will throw an IllegalStateException
myLabel.setText(result); // Incorrect: updating UI from a non-JavaFX thread
});
backgroundThread.start();
Fix:
Use Platform.runLater()
to ensure UI updates occur on the JavaFX Application Thread.
Thread backgroundThread = new Thread(() -> {
String result = performLongTask();
// CORRECT: This will run on the JavaFX Application Thread
Platform.runLater(() -> myLabel.setText(result));
});
backgroundThread.start();
Using Platform.runLater()
encapsulates the UI update in a runnable, which JavaFX executes safely on the correct thread. This simple yet crucial practice helps prevent crashes and bugs in your application.
2. Forgetting to Use FXML
FXML is an XML-based language used to define the user interface in JavaFX applications. Beginners often neglect it, leading them to create UI components purely in Java code. This can result in messy code that is hard to maintain.
Example:
public class MainApp extends Application {
@Override
public void start(Stage primaryStage) {
Label label = new Label("Hello, JavaFX!");
Scene scene = new Scene(new VBox(label), 300, 200);
primaryStage.setScene(scene);
primaryStage.show();
}
}
While this works fine, managing complex UIs directly in code becomes cumbersome.
Fix:
Utilize FXML to separate your UI from your application logic.
- Create a FXML file:
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.scene.control.*?>
<?import javafx.scene.layout.*?>
<VBox xmlns:fx="http://javafx.com/fxml/1" fx:controller="sample.Controller">
<Label fx:id="myLabel" text="Hello, JavaFX!" />
<Button text="Click Me" onAction="#handleButtonClick"/>
</VBox>
- Load the FXML in your MainApp:
@Override
public void start(Stage primaryStage) throws Exception {
FXMLLoader loader = new FXMLLoader(getClass().getResource("myLayout.fxml"));
Parent root = loader.load();
primaryStage.setScene(new Scene(root, 300, 200));
primaryStage.show();
}
By adopting FXML, you maintain cleaner, more readable code and separate concerns, making it easier to update UI without altering the logic.
3. Overlooking Event Handling
Many beginners either forget to handle user inputs or over-complicate event handling. Neglecting events can lead to an unresponsive application, while convoluted event systems can lead to bugs and degraded performance.
Example:
Button button = new Button("Click Me");
button.setOnAction(event -> {
System.out.println("Button Clicked");
});
This snippet effectively responds to button clicks, but many beginners tend to misuse lambdas or do not encapsulate logic properly.
Fix:
Make sure to create separate methods for complex event handling.
Button button = new Button("Click Me");
button.setOnAction(this::handleButtonClick);
// Event handler method
private void handleButtonClick(ActionEvent event) {
System.out.println("Button Clicked");
// Additional logic goes here
}
Separating event logic improves readability and maintainability, clears up the UI code, and makes debugging easier.
4. Not Taking Advantage of CSS for Styling
JavaFX supports rich styling capabilities through CSS, but beginners often stick to Java code for styling or neglect styling altogether. This can lead to inconsistent and unattractive UIs.
Example:
button.setStyle("-fx-background-color: blue; -fx-text-fill: white;");
This method works for simple scenarios, but it's inflexible for complex styles.
Fix:
Create a CSS file and link it to your JavaFX application:
- Create a styles.css file:
.button {
-fx-background-color: blue;
-fx-text-fill: white;
}
- Link it in your application:
Scene scene = new Scene(root, 300, 200);
scene.getStylesheets().add(getClass().getResource("styles.css").toExternalForm());
By using CSS, you take full control of your application's aesthetic, offering a cleaner approach for styling and saving time when adjusting UI appearance.
5. Neglecting Scene Lifecycle Management
JavaFX utilizes a scene graph to manage various states of stages and scenes. A common mistake that beginners make is failing to efficiently transition between different scenes or stages.
Fix:
Use an efficient scene-switching technique, preferably with a dedicated method for transitioning between scenes. For example:
private void switchToScene1() throws IOException {
Parent scene1 = FXMLLoader.load(getClass().getResource("Scene1.fxml"));
Stage stage = (Stage) myButton.getScene().getWindow();
stage.setScene(new Scene(scene1));
}
By creating dedicated methods to manage scene transitions, your application retains clarity, makes transitions fluid, and is easier to manage.
Bringing It All Together
Stepping into JavaFX development can seem daunting, but by understanding and avoiding these common pitfalls, beginners can build robust and responsive applications with ease. The JavaFX community is thriving, and there’s an abundance of resources available online for further learning—check out the OpenJFX community for tutorials and documentation.
By adhering to best practices like threading, using FXML, managing events, adopting CSS for styling, and effectively handling scene lifecycles, developers can unlock JavaFX's full potential. Happy coding!